` element with a non-editable question value within it.\n *\n * - `textRenderMode`: `\"input\"` (default) | `\"div\"`\\\n * Specifies how to render the input field of [Text](https://surveyjs.io/form-library/documentation/api-reference/text-entry-question-model) questions in [read-only](https://surveyjs.io/form-library/documentation/api-reference/text-entry-question-model#readOnly) mode: as a disabled `
` element with a non-editable question value within it.\n */\n readOnly: {\n enableValidation: false,\n commentRenderMode: \"textarea\",\n textRenderMode: \"input\"\n },\n //#region readOnly section, Obsolete properties\n get readOnlyCommentRenderMode() { return this.readOnly.commentRenderMode; },\n set readOnlyCommentRenderMode(val) { this.readOnly.commentRenderMode = val; },\n get readOnlyTextRenderMode() { return this.readOnly.textRenderMode; },\n set readOnlyTextRenderMode(val) { this.readOnly.textRenderMode = val; },\n //#endregion\n /**\n * An object with properties that configure question numbering.\n *\n * Nested properties:\n *\n * - `includeQuestionsWithHiddenNumber`: `boolean`\\\n * Specifies whether to number questions whose [`hideNumber`](https://surveyjs.io/form-library/documentation/api-reference/question#hideNumber) property is enabled. Default value: `false`.\n *\n * - `includeQuestionsWithHiddenTitle`: `boolean`\\\n * Specifies whether to number questions whose [`titleLocation`](https://surveyjs.io/form-library/documentation/api-reference/question#titleLocation) property is set to `\"hidden\"`. Default value: `false`.\n */\n numbering: {\n includeQuestionsWithHiddenNumber: false,\n includeQuestionsWithHiddenTitle: false\n },\n //#region numbering section, Obsolete properties\n get setQuestionVisibleIndexForHiddenTitle() { return this.numbering.includeQuestionsWithHiddenTitle; },\n set setQuestionVisibleIndexForHiddenTitle(val) { this.numbering.includeQuestionsWithHiddenTitle = val; },\n get setQuestionVisibleIndexForHiddenNumber() { return this.numbering.includeQuestionsWithHiddenNumber; },\n set setQuestionVisibleIndexForHiddenNumber(val) { this.numbering.includeQuestionsWithHiddenNumber = val; },\n //#endregion\n /**\n * Specifies an action to perform when users press the Enter key within a survey.\n *\n * Possible values:\n *\n * - `\"moveToNextEditor\"` - Moves focus to the next editor.\n * - `\"loseFocus\"` - Removes focus from the current editor.\n * - `\"default\"` - Behaves as a standard `
` element.\n */\n enterKeyAction: \"default\",\n /**\n * An object that configures string comparison.\n *\n * Nested properties:\n *\n * - `trimStrings`: `boolean`\\\n * Specifies whether to remove whitespace from both ends of a string before the comparison. Default value: `true`.\n *\n * - `caseSensitive`: `boolean`\\\n * Specifies whether to differentiate between capital and lower-case letters. Default value: `false`.\n */\n comparator: {\n trimStrings: true,\n caseSensitive: false,\n normalizeTextCallback: function (str, reason) { return str; }\n },\n expressionDisableConversionChar: \"#\",\n get commentPrefix() { return settings.commentSuffix; },\n set commentPrefix(val) { settings.commentSuffix = val; },\n /**\n * A suffix added to the name of the property that stores comments.\n *\n * Default value: \"-Comment\"\n *\n * You can specify this setting for an individual survey: [`commentSuffix`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#commentSuffix).\n */\n commentSuffix: \"-Comment\",\n /**\n * A separator used in a shorthand notation that specifies a value and display text for an [`ItemValue`](https://surveyjs.io/form-library/documentation/api-reference/itemvalue) object: `\"value|text\"`.\n *\n * Default value: `\"|\"`\n */\n itemValueSeparator: \"|\",\n /**\n * A maximum number of rate values in a [Rating](https://surveyjs.io/form-library/documentation/api-reference/rating-scale-question-model) question.\n *\n * Default value: 20\n */\n ratingMaximumRateValueCount: 20,\n /**\n * Specifies whether to close the drop-down menu of a [TagBox](https://surveyjs.io/form-library/examples/how-to-create-multiselect-tag-box/) question after a user selects a value.\n *\n * This setting applies to all TagBox questions on a page. You can use the [closeOnSelect](https://surveyjs.io/form-library/documentation/api-reference/dropdown-tag-box-model#closeOnSelect) property to specify the same setting for an individual TagBox question.\n */\n tagboxCloseOnSelect: false,\n /**\n * A function that activates a browser confirm dialog.\n *\n * Use the following code to execute this function:\n *\n * ```js\n * import { settings } from \"survey-core\";\n *\n * // `result` contains `true` if the action was confirmed or `false` otherwise\n * const result = settings.confirmActionFunc(\"Are you sure?\");\n * ```\n *\n * You can redefine the `confirmActionFunc` function if you want to display a custom dialog window. Your function should return `true` if a user confirms an action or `false` otherwise.\n * @param message A message to be displayed in the confirm dialog window.\n */\n confirmActionFunc: function (message) {\n return confirm(message);\n },\n /**\n * A function that activates a proprietary SurveyJS confirm dialog.\n *\n * Use the following code to execute this function:\n *\n * ```js\n * import { settings } from \"survey-core\";\n *\n * settings.confirmActionAsync(\"Are you sure?\", (confirmed) => {\n * if (confirmed) {\n * // ...\n * // Proceed with the action\n * // ...\n * } else {\n * // ...\n * // Cancel the action\n * // ...\n * }\n * });\n * ```\n *\n * You can redefine the `confirmActionAsync` function if you want to display a custom dialog window. Your function should return `true` to be enabled; otherwise, a survey executes the [`confirmActionFunc`](#confirmActionFunc) function. Pass the dialog result as the `callback` parameter: `true` if a user confirms an action, `false` otherwise.\n * @param message A message to be displayed in the confirm dialog window.\n * @param callback A callback function that should be called with `true` if a user confirms an action or `false` otherwise.\n */\n confirmActionAsync: function (message, callback, applyTitle, locale, rootElement) {\n return Object(_utils_utils__WEBPACK_IMPORTED_MODULE_1__[\"showConfirmDialog\"])(message, callback, applyTitle, locale, rootElement);\n },\n /**\n * A minimum width value for all survey elements.\n *\n * Default value: `\"300px\"`\n *\n * You can override this setting for individual elements: [`minWidth`](https://surveyjs.io/form-library/documentation/api-reference/surveyelement#minWidth).\n */\n minWidth: \"300px\",\n /**\n * A maximum width value for all survey elements.\n *\n * Default value: `\"100%\"`\n *\n * You can override this setting for individual elements: [`maxWidth`](https://surveyjs.io/form-library/documentation/api-reference/surveyelement#maxWidth).\n */\n maxWidth: \"100%\",\n /**\n * Specifies how many times surveys can re-evaluate expressions when a question value changes. This limit helps avoid recursions in expressions.\n *\n * Default value: 10\n */\n maxConditionRunCountOnValueChanged: 10,\n /**\n * An object that configures notifications.\n *\n * Nested properties:\n *\n * - `lifetime`: `number`\\\n * Specifies a time period during which a notification is displayed; measured in milliseconds.\n */\n notifications: {\n lifetime: 2000\n },\n /**\n * Specifies how many milliseconds a survey should wait before it automatically switches to the next page. Applies only when [auto-advance](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#goNextPageAutomatic) is enabled.\n *\n * Default value: 300\n */\n autoAdvanceDelay: 300,\n /**\n * Specifies the direction in which to lay out Checkbox and Radiogroup items. This setting affects the resulting UI when items are arranged in [more than one column](https://surveyjs.io/form-library/documentation/api-reference/checkbox-question-model#colCount).\n *\n * Possible values:\n *\n * - `\"row\"` (default) - Items fill the current row, then move on to the next row.\n * - `\"column\"` - Items fill the current column, then move on to the next column.\n */\n showItemsInOrder: \"default\",\n /**\n * A value to save in survey results when respondents select the \"None\" choice item.\n *\n * Default value: `\"none\"`\n */\n noneItemValue: \"none\",\n /**\n * A value to save in survey results when respondents select the \"Refuse to answer\" choice item.\n *\n * Default value: `\"refused\"`\n */\n refuseItemValue: \"refused\",\n /**\n * A value to save in survey results when respondents select the \"Don't know\" choice item.\n *\n * Default value: `\"dontknow\"`\n */\n dontKnowItemValue: \"dontknow\",\n /**\n * An object whose properties specify the order of the special choice items (\"None\", \"Other\", \"Select All\", \"Refuse to answer\", \"Don't know\") in select-based questions.\n *\n * Default value: `{ selectAllItem: [-1], noneItem: [1], otherItem: [2], dontKnowItem: [3], otherItem: [4] }`\n *\n * Use this object to reorder special choices. Each property accepts an array of integer numbers. Negative numbers place a special choice item above regular choice items, positive numbers place it below them. For instance, the code below specifies the following order of choices: None, Select All, regular choices, Other.\n *\n * ```js\n * import { settings } from \"survey-core\";\n *\n * settings.specialChoicesOrder.noneItem = [-2];\n * settings.specialChoicesOrder.selectAllItem = [-1];\n * settings.specialChoicesOrder.otherItem = [1];\n * ```\n *\n * If you want to duplicate a special choice item above and below other choices, add two numbers to the corresponding array:\n *\n * ```js\n * settings.specialChoicesOrder.selectAllItem = [-1, 3] // Displays Select All above and below other choices\n * ```\n */\n specialChoicesOrder: {\n selectAllItem: [-1],\n noneItem: [1],\n refuseItem: [2],\n dontKnowItem: [3],\n otherItem: [4]\n },\n /**\n * A list of supported validators by question type.\n */\n supportedValidators: {\n question: [\"expression\"],\n comment: [\"text\", \"regex\"],\n text: [\"numeric\", \"text\", \"regex\", \"email\"],\n checkbox: [\"answercount\"],\n imagepicker: [\"answercount\"],\n },\n /**\n * Specifies a minimum date that users can enter into a [Text](https://surveyjs.io/form-library/documentation/api-reference/text-entry-question-model) question with [`inputType`](https://surveyjs.io/form-library/documentation/api-reference/text-entry-question-model#inputType) set to `\"date\"` or `\"datetime-local\"`. Set this property to a string with the folllowing format: `\"yyyy-mm-dd\"`.\n */\n minDate: \"\",\n /**\n * Specifies a maximum date that users can enter into a [Text](https://surveyjs.io/form-library/documentation/api-reference/text-entry-question-model) question with [`inputType`](https://surveyjs.io/form-library/documentation/api-reference/text-entry-question-model#inputType) set to `\"date\"` or `\"datetime-local\"`. Set this property to a string with the folllowing format: `\"yyyy-mm-dd\"`.\n */\n maxDate: \"\",\n showModal: undefined,\n showDialog: undefined,\n supportCreatorV2: false,\n showDefaultItemsInCreatorV2: true,\n /**\n * An object that specifies icon replacements. Object keys are built-in icon names. To use a custom icon, assign its name to the key of the icon you want to replace:\n *\n * ```js\n * import { settings } from \"survey-core\";\n *\n * settings.customIcons[\"icon-redo\"] = \"custom-redo-icon\";\n * ```\n *\n * For more information about icons in SurveyJS, refer to the following help topic: [UI Icons](https://surveyjs.io/form-library/documentation/icons).\n */\n customIcons: {},\n /**\n * Specifies which part of a choice item responds to a drag gesture in Ranking questions.\n *\n * Possible values:\n *\n * - `\"entireItem\"` (default) - Users can use the entire choice item as a drag handle.\n * - `\"icon\"` - Users can only use the choice item icon as a drag handle.\n */\n rankingDragHandleArea: \"entireItem\",\n environment: defaultEnvironment,\n /**\n * Allows you to hide the maximum length indicator in text input questions.\n *\n * If you specify a question's [`maxLength`](https://surveyjs.io/form-library/documentation/api-reference/text-entry-question-model#maxLength) property or a survey's [`maxTextLength`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#maxTextLength) property, text input questions indicate the number of entered characters and the character limit. Assign `false` to the `settings.showMaxLengthIndicator` property if you want to hide this indicator.\n *\n * Default value: `true`\n */\n showMaxLengthIndicator: true,\n /**\n * Specifies whether to animate survey elements.\n *\n * Default value: `true`\n */\n animationEnabled: true,\n /**\n * An object that specifies heading levels (`
`, ``, etc.) to use when rendering survey, page, panel, and question titles.\n *\n * Default value: `{ survey: \"h3\", page: \"h4\", panel: \"h4\", question: \"h5\" }`\n *\n * If you want to modify heading levels for individual titles, handle `SurveyModel`'s [`onGetTitleTagName`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#onGetTitleTagName) event.\n */\n titleTags: {\n survey: \"h3\",\n page: \"h4\",\n panel: \"h4\",\n question: \"h5\",\n },\n questions: {\n inputTypes: [\n \"color\",\n \"date\",\n \"datetime-local\",\n \"email\",\n \"month\",\n \"number\",\n \"password\",\n \"range\",\n \"tel\",\n \"text\",\n \"time\",\n \"url\",\n \"week\",\n ],\n dataList: [\n \"\",\n \"name\",\n \"honorific-prefix\",\n \"given-name\",\n \"additional-name\",\n \"family-name\",\n \"honorific-suffix\",\n \"nickname\",\n \"organization-title\",\n \"username\",\n \"new-password\",\n \"current-password\",\n \"organization\",\n \"street-address\",\n \"address-line1\",\n \"address-line2\",\n \"address-line3\",\n \"address-level4\",\n \"address-level3\",\n \"address-level2\",\n \"address-level1\",\n \"country\",\n \"country-name\",\n \"postal-code\",\n \"cc-name\",\n \"cc-given-name\",\n \"cc-additional-name\",\n \"cc-family-name\",\n \"cc-number\",\n \"cc-exp\",\n \"cc-exp-month\",\n \"cc-exp-year\",\n \"cc-csc\",\n \"cc-type\",\n \"transaction-currency\",\n \"transaction-amount\",\n \"language\",\n \"bday\",\n \"bday-day\",\n \"bday-month\",\n \"bday-year\",\n \"sex\",\n \"url\",\n \"photo\",\n \"tel\",\n \"tel-country-code\",\n \"tel-national\",\n \"tel-area-code\",\n \"tel-local\",\n \"tel-local-prefix\",\n \"tel-local-suffix\",\n \"tel-extension\",\n \"email\",\n \"impp\",\n ]\n },\n legacyProgressBarView: false,\n /**\n * An object with properties that configure input masks.\n *\n * Nested properties:\n *\n * - `patternPlaceholderChar`: `string`\\\n * A symbol used as a placeholder for characters to be entered in [pattern masks](https://surveyjs.io/form-library/documentation/api-reference/inputmaskpattern). Default value: `\"_\"`.\n *\n * - `patternEscapeChar`: `string`\\\n * A symbol used to insert literal representations of special characters in [pattern masks](https://surveyjs.io/form-library/documentation/api-reference/inputmaskpattern). Default value: `\"\\\\\"`.\n *\n * - `patternDefinitions`: `<{ [key: string]: RegExp }>`\\\n * An object that maps placeholder symbols to regular expressions in [pattern masks](https://surveyjs.io/form-library/documentation/api-reference/inputmaskpattern). Default value: `{ \"9\": /[0-9]/, \"a\": /[a-zA-Z]/, \"#\": /[a-zA-Z0-9]/ }`.\n */\n maskSettings: {\n patternPlaceholderChar: \"_\",\n patternEscapeChar: \"\\\\\",\n patternDefinitions: {\n \"9\": /[0-9]/,\n \"a\": /[a-zA-Z]/,\n \"#\": /[a-zA-Z0-9]/\n }\n }\n};\n\n\n/***/ }),\n\n/***/ \"./src/stylesmanager.ts\":\n/*!******************************!*\\\n !*** ./src/stylesmanager.ts ***!\n \\******************************/\n/*! exports provided: modernThemeColors, defaultThemeColors, orangeThemeColors, darkblueThemeColors, darkroseThemeColors, stoneThemeColors, winterThemeColors, winterstoneThemeColors, StylesManager */\n/***/ (function(module, __webpack_exports__, __webpack_require__) {\n\n\"use strict\";\n__webpack_require__.r(__webpack_exports__);\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"modernThemeColors\", function() { return modernThemeColors; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"defaultThemeColors\", function() { return defaultThemeColors; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"orangeThemeColors\", function() { return orangeThemeColors; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"darkblueThemeColors\", function() { return darkblueThemeColors; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"darkroseThemeColors\", function() { return darkroseThemeColors; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"stoneThemeColors\", function() { return stoneThemeColors; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"winterThemeColors\", function() { return winterThemeColors; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"winterstoneThemeColors\", function() { return winterstoneThemeColors; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"StylesManager\", function() { return StylesManager; });\n/* harmony import */ var _defaultCss_defaultV2Css__WEBPACK_IMPORTED_MODULE_0__ = __webpack_require__(/*! ./defaultCss/defaultV2Css */ \"./src/defaultCss/defaultV2Css.ts\");\n/* harmony import */ var _global_variables_utils__WEBPACK_IMPORTED_MODULE_1__ = __webpack_require__(/*! ./global_variables_utils */ \"./src/global_variables_utils.ts\");\n/* harmony import */ var _settings__WEBPACK_IMPORTED_MODULE_2__ = __webpack_require__(/*! ./settings */ \"./src/settings.ts\");\n/* harmony import */ var _utils_utils__WEBPACK_IMPORTED_MODULE_3__ = __webpack_require__(/*! ./utils/utils */ \"./src/utils/utils.ts\");\n\n\n\n\nvar modernThemeColors = {\n \"$main-color\": \"#1ab394\",\n \"$add-button-color\": \"#1948b3\",\n \"$remove-button-color\": \"#ff1800\",\n \"$disable-color\": \"#dbdbdb\",\n \"$progress-text-color\": \"#9d9d9d\",\n \"$disabled-label-color\": \"rgba(64, 64, 64, 0.5)\",\n \"$slider-color\": \"white\",\n \"$disabled-slider-color\": \"#cfcfcf\",\n \"$error-color\": \"#d52901\",\n \"$text-color\": \"#404040\",\n \"$light-text-color\": \"#fff\",\n \"$checkmark-color\": \"#fff\",\n \"$progress-buttons-color\": \"#8dd9ca\",\n \"$inputs-background-color\": \"transparent\",\n \"$main-hover-color\": \"#9f9f9f\",\n \"$body-container-background-color\": \"#f4f4f4\",\n \"$text-border-color\": \"#d4d4d4\",\n \"$disabled-text-color\": \"rgba(64, 64, 64, 0.5)\",\n \"$border-color\": \"rgb(64, 64, 64, 0.5)\",\n \"$header-background-color\": \"#e7e7e7\",\n \"$answer-background-color\": \"rgba(26, 179, 148, 0.2)\",\n \"$error-background-color\": \"rgba(213, 41, 1, 0.2)\",\n \"$radio-checked-color\": \"#404040\",\n \"$clean-button-color\": \"#1948b3\",\n \"$body-background-color\": \"#ffffff\",\n \"$foreground-light\": \"#909090\",\n \"$font-family\": \"Raleway\",\n};\nvar defaultThemeColors = {\n \"$header-background-color\": \"#e7e7e7\",\n \"$body-container-background-color\": \"#f4f4f4\",\n \"$main-color\": \"#1ab394\",\n \"$main-hover-color\": \"#0aa384\",\n \"$body-background-color\": \"white\",\n \"$inputs-background-color\": \"white\",\n \"$text-color\": \"#6d7072\",\n \"$text-input-color\": \"#6d7072\",\n \"$header-color\": \"#6d7072\",\n \"$border-color\": \"#e7e7e7\",\n \"$error-color\": \"#ed5565\",\n \"$error-background-color\": \"#fcdfe2\",\n \"$progress-text-color\": \"#9d9d9d\",\n \"$disable-color\": \"#dbdbdb\",\n \"$disabled-label-color\": \"rgba(64, 64, 64, 0.5)\",\n \"$slider-color\": \"white\",\n \"$disabled-switch-color\": \"#9f9f9f\",\n \"$disabled-slider-color\": \"#cfcfcf\",\n \"$foreground-light\": \"#909090\",\n \"$foreground-disabled\": \"#161616\",\n \"$background-dim\": \"#f3f3f3\",\n \"$progress-buttons-color\": \"#8dd9ca\",\n \"$progress-buttons-line-color\": \"#d4d4d4\"\n};\nvar orangeThemeColors = {\n \"$header-background-color\": \"#4a4a4a\",\n \"$body-container-background-color\": \"#f8f8f8\",\n \"$main-color\": \"#f78119\",\n \"$main-hover-color\": \"#e77109\",\n \"$body-background-color\": \"white\",\n \"$inputs-background-color\": \"white\",\n \"$text-color\": \"#4a4a4a\",\n \"$text-input-color\": \"#4a4a4a\",\n \"$header-color\": \"#f78119\",\n \"$border-color\": \"#e7e7e7\",\n \"$error-color\": \"#ed5565\",\n \"$error-background-color\": \"#fcdfe2\",\n \"$progress-text-color\": \"#9d9d9d\",\n \"$disable-color\": \"#dbdbdb\",\n \"$disabled-label-color\": \"rgba(64, 64, 64, 0.5)\",\n \"$slider-color\": \"white\",\n \"$disabled-switch-color\": \"#9f9f9f\",\n \"$disabled-slider-color\": \"#cfcfcf\",\n \"$foreground-light\": \"#909090\",\n \"$foreground-disabled\": \"#161616\",\n \"$background-dim\": \"#f3f3f3\",\n \"$progress-buttons-color\": \"#f7b781\",\n \"$progress-buttons-line-color\": \"#d4d4d4\"\n};\nvar darkblueThemeColors = {\n \"$header-background-color\": \"#d9d8dd\",\n \"$body-container-background-color\": \"#f6f7f2\",\n \"$main-color\": \"#3c4f6d\",\n \"$main-hover-color\": \"#2c3f5d\",\n \"$body-background-color\": \"white\",\n \"$inputs-background-color\": \"white\",\n \"$text-color\": \"#4a4a4a\",\n \"$text-input-color\": \"#4a4a4a\",\n \"$header-color\": \"#6d7072\",\n \"$border-color\": \"#e7e7e7\",\n \"$error-color\": \"#ed5565\",\n \"$error-background-color\": \"#fcdfe2\",\n \"$progress-text-color\": \"#9d9d9d\",\n \"$disable-color\": \"#dbdbdb\",\n \"$disabled-label-color\": \"rgba(64, 64, 64, 0.5)\",\n \"$slider-color\": \"white\",\n \"$disabled-switch-color\": \"#9f9f9f\",\n \"$disabled-slider-color\": \"#cfcfcf\",\n \"$foreground-light\": \"#909090\",\n \"$foreground-disabled\": \"#161616\",\n \"$background-dim\": \"#f3f3f3\",\n \"$progress-buttons-color\": \"#839ec9\",\n \"$progress-buttons-line-color\": \"#d4d4d4\"\n};\nvar darkroseThemeColors = {\n \"$header-background-color\": \"#ddd2ce\",\n \"$body-container-background-color\": \"#f7efed\",\n \"$main-color\": \"#68656e\",\n \"$main-hover-color\": \"#58555e\",\n \"$body-background-color\": \"white\",\n \"$inputs-background-color\": \"white\",\n \"$text-color\": \"#4a4a4a\",\n \"$text-input-color\": \"#4a4a4a\",\n \"$header-color\": \"#6d7072\",\n \"$border-color\": \"#e7e7e7\",\n \"$error-color\": \"#ed5565\",\n \"$error-background-color\": \"#fcdfe2\",\n \"$progress-text-color\": \"#9d9d9d\",\n \"$disable-color\": \"#dbdbdb\",\n \"$disabled-label-color\": \"rgba(64, 64, 64, 0.5)\",\n \"$slider-color\": \"white\",\n \"$disabled-switch-color\": \"#9f9f9f\",\n \"$disabled-slider-color\": \"#cfcfcf\",\n \"$foreground-light\": \"#909090\",\n \"$foreground-disabled\": \"#161616\",\n \"$background-dim\": \"#f3f3f3\",\n \"$progress-buttons-color\": \"#c6bed4\",\n \"$progress-buttons-line-color\": \"#d4d4d4\"\n};\nvar stoneThemeColors = {\n \"$header-background-color\": \"#cdccd2\",\n \"$body-container-background-color\": \"#efedf4\",\n \"$main-color\": \"#0f0f33\",\n \"$main-hover-color\": \"#191955\",\n \"$body-background-color\": \"white\",\n \"$inputs-background-color\": \"white\",\n \"$text-color\": \"#0f0f33\",\n \"$text-input-color\": \"#0f0f33\",\n \"$header-color\": \"#0f0f33\",\n \"$border-color\": \"#e7e7e7\",\n \"$error-color\": \"#ed5565\",\n \"$error-background-color\": \"#fcdfe2\",\n \"$progress-text-color\": \"#9d9d9d\",\n \"$disable-color\": \"#dbdbdb\",\n \"$disabled-label-color\": \"rgba(64, 64, 64, 0.5)\",\n \"$slider-color\": \"white\",\n \"$disabled-switch-color\": \"#9f9f9f\",\n \"$disabled-slider-color\": \"#cfcfcf\",\n \"$foreground-light\": \"#909090\",\n \"$foreground-disabled\": \"#161616\",\n \"$background-dim\": \"#f3f3f3\",\n \"$progress-buttons-color\": \"#747491\",\n \"$progress-buttons-line-color\": \"#d4d4d4\"\n};\nvar winterThemeColors = {\n \"$header-background-color\": \"#82b8da\",\n \"$body-container-background-color\": \"#dae1e7\",\n \"$main-color\": \"#3c3b40\",\n \"$main-hover-color\": \"#1e1d20\",\n \"$body-background-color\": \"white\",\n \"$inputs-background-color\": \"white\",\n \"$text-color\": \"#000\",\n \"$text-input-color\": \"#000\",\n \"$header-color\": \"#000\",\n \"$border-color\": \"#e7e7e7\",\n \"$error-color\": \"#ed5565\",\n \"$error-background-color\": \"#fcdfe2\",\n \"$disable-color\": \"#dbdbdb\",\n \"$progress-text-color\": \"#9d9d9d\",\n \"$disabled-label-color\": \"rgba(64, 64, 64, 0.5)\",\n \"$slider-color\": \"white\",\n \"$disabled-switch-color\": \"#9f9f9f\",\n \"$disabled-slider-color\": \"#cfcfcf\",\n \"$foreground-light\": \"#909090\",\n \"$foreground-disabled\": \"#161616\",\n \"$background-dim\": \"#f3f3f3\",\n \"$progress-buttons-color\": \"#d1c9f5\",\n \"$progress-buttons-line-color\": \"#d4d4d4\"\n};\nvar winterstoneThemeColors = {\n \"$header-background-color\": \"#323232\",\n \"$body-container-background-color\": \"#f8f8f8\",\n \"$main-color\": \"#5ac8fa\",\n \"$main-hover-color\": \"#06a1e7\",\n \"$body-background-color\": \"white\",\n \"$inputs-background-color\": \"white\",\n \"$text-color\": \"#000\",\n \"$text-input-color\": \"#000\",\n \"$header-color\": \"#000\",\n \"$border-color\": \"#e7e7e7\",\n \"$error-color\": \"#ed5565\",\n \"$error-background-color\": \"#fcdfe2\",\n \"$disable-color\": \"#dbdbdb\",\n \"$progress-text-color\": \"#9d9d9d\",\n \"$disabled-label-color\": \"rgba(64, 64, 64, 0.5)\",\n \"$slider-color\": \"white\",\n \"$disabled-switch-color\": \"#9f9f9f\",\n \"$disabled-slider-color\": \"#cfcfcf\",\n \"$foreground-light\": \"#909090\",\n \"$foreground-disabled\": \"#161616\",\n \"$background-dim\": \"#f3f3f3\",\n \"$progress-buttons-color\": \"#acdcf2\",\n \"$progress-buttons-line-color\": \"#d4d4d4\"\n};\nfunction setCssVariables(vars, element) {\n Object.keys(vars || {}).forEach(function (sassVarName) {\n var name = sassVarName.substring(1);\n element.style.setProperty(\"--\" + name, vars[sassVarName]);\n });\n}\nvar StylesManager = /** @class */ (function () {\n function StylesManager() {\n StylesManager.autoApplyTheme();\n }\n StylesManager.autoApplyTheme = function () {\n if (_defaultCss_defaultV2Css__WEBPACK_IMPORTED_MODULE_0__[\"surveyCss\"].currentType === \"bootstrap\" || _defaultCss_defaultV2Css__WEBPACK_IMPORTED_MODULE_0__[\"surveyCss\"].currentType === \"bootstrapmaterial\") {\n return;\n }\n var includedThemeCss = StylesManager.getIncludedThemeCss();\n if (includedThemeCss.length === 1) {\n StylesManager.applyTheme(includedThemeCss[0].name);\n }\n };\n StylesManager.getAvailableThemes = function () {\n var themeMapper = _defaultCss_defaultV2Css__WEBPACK_IMPORTED_MODULE_0__[\"surveyCss\"].getAvailableThemes()\n .filter(function (themeName) { return [\"defaultV2\", \"default\", \"modern\"].indexOf(themeName) !== -1; })\n .map(function (themeName) { return { name: themeName, theme: _defaultCss_defaultV2Css__WEBPACK_IMPORTED_MODULE_0__[\"surveyCss\"][themeName] }; });\n return themeMapper;\n };\n StylesManager.getIncludedThemeCss = function () {\n if (typeof _settings__WEBPACK_IMPORTED_MODULE_2__[\"settings\"].environment === \"undefined\")\n return [];\n var rootElement = _settings__WEBPACK_IMPORTED_MODULE_2__[\"settings\"].environment.rootElement;\n var themeMapper = StylesManager.getAvailableThemes();\n var element = Object(_utils_utils__WEBPACK_IMPORTED_MODULE_3__[\"isShadowDOM\"])(rootElement) ? rootElement.host : rootElement;\n if (!!element) {\n var styles_1 = getComputedStyle(element);\n if (styles_1.length) {\n return themeMapper.filter(function (item) { return item.theme.variables && styles_1.getPropertyValue(item.theme.variables.themeMark); });\n }\n }\n return [];\n };\n StylesManager.findSheet = function (styleSheetId) {\n if (typeof _settings__WEBPACK_IMPORTED_MODULE_2__[\"settings\"].environment === \"undefined\")\n return null;\n var styleSheets = _settings__WEBPACK_IMPORTED_MODULE_2__[\"settings\"].environment.root.styleSheets;\n for (var i = 0; i < styleSheets.length; i++) {\n if (!!styleSheets[i].ownerNode && styleSheets[i].ownerNode[\"id\"] === styleSheetId) {\n return styleSheets[i];\n }\n }\n return null;\n };\n StylesManager.createSheet = function (styleSheetId) {\n var stylesSheetsMountContainer = _settings__WEBPACK_IMPORTED_MODULE_2__[\"settings\"].environment.stylesSheetsMountContainer;\n var style = _global_variables_utils__WEBPACK_IMPORTED_MODULE_1__[\"DomDocumentHelper\"].createElement(\"style\");\n style.id = styleSheetId;\n // Add a media (and/or media query) here if you'd like!\n // style.setAttribute(\"media\", \"screen\")\n // style.setAttribute(\"media\", \"only screen and (max-width : 1024px)\")\n style.appendChild(new Text(\"\"));\n Object(_utils_utils__WEBPACK_IMPORTED_MODULE_3__[\"getElement\"])(stylesSheetsMountContainer).appendChild(style);\n if (!!StylesManager.Logger) {\n StylesManager.Logger.log(\"style sheet \" + styleSheetId + \" created\");\n }\n return style.sheet;\n };\n StylesManager.applyTheme = function (themeName, themeSelector) {\n if (themeName === void 0) { themeName = \"default\"; }\n if (typeof _settings__WEBPACK_IMPORTED_MODULE_2__[\"settings\"].environment === \"undefined\")\n return;\n var rootElement = _settings__WEBPACK_IMPORTED_MODULE_2__[\"settings\"].environment.rootElement;\n var element = Object(_utils_utils__WEBPACK_IMPORTED_MODULE_3__[\"isShadowDOM\"])(rootElement) ? rootElement.host : rootElement;\n _defaultCss_defaultV2Css__WEBPACK_IMPORTED_MODULE_0__[\"surveyCss\"].currentType = themeName;\n if (StylesManager.Enabled) {\n if (themeName !== \"bootstrap\" && themeName !== \"bootstrapmaterial\") {\n setCssVariables(StylesManager.ThemeColors[themeName], element);\n if (!!StylesManager.Logger) {\n StylesManager.Logger.log(\"apply theme \" + themeName + \" completed\");\n }\n return;\n }\n var themeCss_1 = StylesManager.ThemeCss[themeName];\n if (!themeCss_1) {\n _defaultCss_defaultV2Css__WEBPACK_IMPORTED_MODULE_0__[\"surveyCss\"].currentType = \"defaultV2\";\n return;\n }\n StylesManager.insertStylesRulesIntoDocument();\n var currentThemeSelector_1 = themeSelector || StylesManager.ThemeSelector[themeName] || StylesManager.ThemeSelector[\"default\"];\n var styleSheetId = (themeName + currentThemeSelector_1).trim();\n var sheet_1 = StylesManager.findSheet(styleSheetId);\n if (!sheet_1) {\n sheet_1 = StylesManager.createSheet(styleSheetId);\n var themeColors_1 = StylesManager.ThemeColors[themeName] || StylesManager.ThemeColors[\"default\"];\n Object.keys(themeCss_1).forEach(function (selector) {\n var cssRuleText = themeCss_1[selector];\n Object.keys(themeColors_1 || {}).forEach(function (colorVariableName) { return (cssRuleText = cssRuleText.replace(new RegExp(\"\\\\\" + colorVariableName, \"g\"), themeColors_1[colorVariableName])); });\n try {\n if (selector.indexOf(\"body\") === 0) {\n sheet_1.insertRule(selector + \" { \" + cssRuleText + \" }\", 0);\n }\n else {\n sheet_1.insertRule(currentThemeSelector_1 + selector + \" { \" + cssRuleText + \" }\", 0);\n }\n }\n catch (e) { }\n });\n }\n }\n if (!!StylesManager.Logger) {\n StylesManager.Logger.log(\"apply theme \" + themeName + \" completed\");\n }\n };\n StylesManager.insertStylesRulesIntoDocument = function () {\n if (StylesManager.Enabled) {\n var sheet_2 = StylesManager.findSheet(StylesManager.SurveyJSStylesSheetId);\n if (!sheet_2) {\n sheet_2 = StylesManager.createSheet(StylesManager.SurveyJSStylesSheetId);\n }\n if (Object.keys(StylesManager.Styles).length) {\n Object.keys(StylesManager.Styles).forEach(function (selector) {\n try {\n sheet_2.insertRule(selector + \" { \" + StylesManager.Styles[selector] + \" }\", 0);\n }\n catch (e) { }\n });\n }\n if (Object.keys(StylesManager.Media).length) {\n Object.keys(StylesManager.Media).forEach(function (selector) {\n try {\n sheet_2.insertRule(StylesManager.Media[selector].media +\n \" { \" +\n selector +\n \" { \" +\n StylesManager.Media[selector].style +\n \" } }\", 0);\n }\n catch (e) { }\n });\n }\n }\n };\n StylesManager.SurveyJSStylesSheetId = \"surveyjs-styles\";\n StylesManager.Styles = {};\n StylesManager.Media = {};\n StylesManager.ThemeColors = {\n \"modern\": modernThemeColors,\n \"default\": defaultThemeColors,\n \"orange\": orangeThemeColors,\n \"darkblue\": darkblueThemeColors,\n \"darkrose\": darkroseThemeColors,\n \"stone\": stoneThemeColors,\n \"winter\": winterThemeColors,\n \"winterstone\": winterstoneThemeColors,\n };\n StylesManager.ThemeCss = {};\n StylesManager.ThemeSelector = {\n \"default\": \".sv_main \",\n \"modern\": \".sv-root-modern \"\n };\n StylesManager.Enabled = true;\n return StylesManager;\n}());\n\n\n\n/***/ }),\n\n/***/ \"./src/survey-element.ts\":\n/*!*******************************!*\\\n !*** ./src/survey-element.ts ***!\n \\*******************************/\n/*! exports provided: SurveyElementCore, DragTypeOverMeEnum, SurveyElement */\n/***/ (function(module, __webpack_exports__, __webpack_require__) {\n\n\"use strict\";\n__webpack_require__.r(__webpack_exports__);\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"SurveyElementCore\", function() { return SurveyElementCore; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"DragTypeOverMeEnum\", function() { return DragTypeOverMeEnum; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"SurveyElement\", function() { return SurveyElement; });\n/* harmony import */ var _jsonobject__WEBPACK_IMPORTED_MODULE_0__ = __webpack_require__(/*! ./jsonobject */ \"./src/jsonobject.ts\");\n/* harmony import */ var _base__WEBPACK_IMPORTED_MODULE_1__ = __webpack_require__(/*! ./base */ \"./src/base.ts\");\n/* harmony import */ var _actions_adaptive_container__WEBPACK_IMPORTED_MODULE_2__ = __webpack_require__(/*! ./actions/adaptive-container */ \"./src/actions/adaptive-container.ts\");\n/* harmony import */ var _helpers__WEBPACK_IMPORTED_MODULE_3__ = __webpack_require__(/*! ./helpers */ \"./src/helpers.ts\");\n/* harmony import */ var _settings__WEBPACK_IMPORTED_MODULE_4__ = __webpack_require__(/*! ./settings */ \"./src/settings.ts\");\n/* harmony import */ var _actions_container__WEBPACK_IMPORTED_MODULE_5__ = __webpack_require__(/*! ./actions/container */ \"./src/actions/container.ts\");\n/* harmony import */ var _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_6__ = __webpack_require__(/*! ./utils/cssClassBuilder */ \"./src/utils/cssClassBuilder.ts\");\n/* harmony import */ var _utils_animation__WEBPACK_IMPORTED_MODULE_7__ = __webpack_require__(/*! ./utils/animation */ \"./src/utils/animation.ts\");\n/* harmony import */ var _utils_utils__WEBPACK_IMPORTED_MODULE_8__ = __webpack_require__(/*! ./utils/utils */ \"./src/utils/utils.ts\");\n/* harmony import */ var _global_variables_utils__WEBPACK_IMPORTED_MODULE_9__ = __webpack_require__(/*! ./global_variables_utils */ \"./src/global_variables_utils.ts\");\nvar __extends = (undefined && undefined.__extends) || (function () {\n var extendStatics = function (d, b) {\n extendStatics = Object.setPrototypeOf ||\n ({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) ||\n function (d, b) { for (var p in b) if (Object.prototype.hasOwnProperty.call(b, p)) d[p] = b[p]; };\n return extendStatics(d, b);\n };\n return function (d, b) {\n if (typeof b !== \"function\" && b !== null)\n throw new TypeError(\"Class extends value \" + String(b) + \" is not a constructor or null\");\n extendStatics(d, b);\n function __() { this.constructor = d; }\n d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());\n };\n})();\nvar __decorate = (undefined && undefined.__decorate) || function (decorators, target, key, desc) {\n var c = arguments.length, r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc, d;\n if (typeof Reflect === \"object\" && typeof Reflect.decorate === \"function\") r = Reflect.decorate(decorators, target, key, desc);\n else for (var i = decorators.length - 1; i >= 0; i--) if (d = decorators[i]) r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;\n return c > 3 && r && Object.defineProperty(target, key, r), r;\n};\n\n\n\n\n\n\n\n\n\n\n/**\n * A base class for the [`SurveyElement`](https://surveyjs.io/form-library/documentation/surveyelement) and [`SurveyModel`](https://surveyjs.io/form-library/documentation/surveymodel) classes.\n */\nvar SurveyElementCore = /** @class */ (function (_super) {\n __extends(SurveyElementCore, _super);\n function SurveyElementCore() {\n var _this = _super.call(this) || this;\n _this.createLocTitleProperty();\n return _this;\n }\n SurveyElementCore.prototype.createLocTitleProperty = function () {\n return this.createLocalizableString(\"title\", this, true);\n };\n Object.defineProperty(SurveyElementCore.prototype, \"title\", {\n /**\n * A title for the survey element. If `title` is undefined, the `name` property value is displayed instead.\n *\n * Empty pages and panels do not display their titles or names.\n *\n * @see [Configure Question Titles](https://surveyjs.io/form-library/documentation/design-survey-question-titles)\n */\n get: function () {\n return this.getLocalizableStringText(\"title\", this.getDefaultTitleValue());\n },\n set: function (val) {\n this.setLocalizableStringText(\"title\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElementCore.prototype, \"locTitle\", {\n get: function () {\n return this.getLocalizableString(\"title\");\n },\n enumerable: false,\n configurable: true\n });\n SurveyElementCore.prototype.getDefaultTitleValue = function () { return undefined; };\n SurveyElementCore.prototype.updateDescriptionVisibility = function (newDescription) {\n var showPlaceholder = false;\n if (this.isDesignMode) {\n var property_1 = _jsonobject__WEBPACK_IMPORTED_MODULE_0__[\"Serializer\"].findProperty(this.getType(), \"description\");\n showPlaceholder = !!(property_1 === null || property_1 === void 0 ? void 0 : property_1.placeholder);\n }\n this.hasDescription = !!newDescription || (showPlaceholder && this.isDesignMode);\n };\n Object.defineProperty(SurveyElementCore.prototype, \"locDescription\", {\n get: function () {\n return this.getLocalizableString(\"description\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElementCore.prototype, \"titleTagName\", {\n get: function () {\n var titleTagName = this.getDefaultTitleTagName();\n var survey = this.getSurvey();\n return !!survey ? survey.getElementTitleTagName(this, titleTagName) : titleTagName;\n },\n enumerable: false,\n configurable: true\n });\n SurveyElementCore.prototype.getDefaultTitleTagName = function () {\n return _settings__WEBPACK_IMPORTED_MODULE_4__[\"settings\"].titleTags[this.getType()];\n };\n Object.defineProperty(SurveyElementCore.prototype, \"hasTitle\", {\n get: function () { return this.title.length > 0; },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElementCore.prototype, \"hasTitleActions\", {\n get: function () { return false; },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElementCore.prototype, \"hasTitleEvents\", {\n get: function () {\n return this.hasTitleActions;\n },\n enumerable: false,\n configurable: true\n });\n SurveyElementCore.prototype.getTitleToolbar = function () { return null; };\n SurveyElementCore.prototype.getTitleOwner = function () { return undefined; };\n Object.defineProperty(SurveyElementCore.prototype, \"isTitleOwner\", {\n get: function () { return !!this.getTitleOwner(); },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElementCore.prototype, \"isTitleRenderedAsString\", {\n get: function () { return this.getIsTitleRenderedAsString(); },\n enumerable: false,\n configurable: true\n });\n SurveyElementCore.prototype.toggleState = function () { return undefined; };\n Object.defineProperty(SurveyElementCore.prototype, \"cssClasses\", {\n get: function () { return {}; },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElementCore.prototype, \"cssTitle\", {\n get: function () { return \"\"; },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElementCore.prototype, \"ariaTitleId\", {\n get: function () { return undefined; },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElementCore.prototype, \"ariaDescriptionId\", {\n get: function () { return undefined; },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElementCore.prototype, \"titleTabIndex\", {\n get: function () { return undefined; },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElementCore.prototype, \"titleAriaExpanded\", {\n get: function () { return undefined; },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElementCore.prototype, \"titleAriaRole\", {\n get: function () { return undefined; },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElementCore.prototype, \"ariaLabel\", {\n get: function () {\n return this.locTitle.renderedHtml;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElementCore.prototype, \"titleAriaLabel\", {\n get: function () {\n return this.ariaLabel;\n },\n enumerable: false,\n configurable: true\n });\n SurveyElementCore.prototype.getIsTitleRenderedAsString = function () { return !this.isTitleOwner; };\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_0__[\"property\"])()\n ], SurveyElementCore.prototype, \"hasDescription\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_0__[\"property\"])({\n localizable: true,\n onSet: function (newDescription, self) {\n self.updateDescriptionVisibility(newDescription);\n }\n })\n ], SurveyElementCore.prototype, \"description\", void 0);\n return SurveyElementCore;\n}(_base__WEBPACK_IMPORTED_MODULE_1__[\"Base\"]));\n\n// TODO: rename\nvar DragTypeOverMeEnum;\n(function (DragTypeOverMeEnum) {\n DragTypeOverMeEnum[DragTypeOverMeEnum[\"InsideEmptyPanel\"] = 1] = \"InsideEmptyPanel\";\n DragTypeOverMeEnum[DragTypeOverMeEnum[\"MultilineRight\"] = 2] = \"MultilineRight\";\n DragTypeOverMeEnum[DragTypeOverMeEnum[\"MultilineLeft\"] = 3] = \"MultilineLeft\";\n DragTypeOverMeEnum[DragTypeOverMeEnum[\"Top\"] = 4] = \"Top\";\n DragTypeOverMeEnum[DragTypeOverMeEnum[\"Right\"] = 5] = \"Right\";\n DragTypeOverMeEnum[DragTypeOverMeEnum[\"Bottom\"] = 6] = \"Bottom\";\n DragTypeOverMeEnum[DragTypeOverMeEnum[\"Left\"] = 7] = \"Left\";\n})(DragTypeOverMeEnum || (DragTypeOverMeEnum = {}));\n/**\n * A base class for all survey elements.\n */\nvar SurveyElement = /** @class */ (function (_super) {\n __extends(SurveyElement, _super);\n function SurveyElement(name) {\n var _this = _super.call(this) || this;\n _this.selectedElementInDesignValue = _this;\n _this.disableDesignActions = SurveyElement.CreateDisabledDesignElements;\n _this.parentQuestionValue = null;\n _this.isContentElement = false;\n _this.isEditableTemplateElement = false;\n _this.isInteractiveDesignElement = true;\n _this.isSingleInRow = true;\n _this._renderedIsExpanded = true;\n _this._isAnimatingCollapseExpand = false;\n _this.animationCollapsed = new _utils_animation__WEBPACK_IMPORTED_MODULE_7__[\"AnimationBoolean\"](_this.getExpandCollapseAnimationOptions(), function (val) {\n _this._renderedIsExpanded = val;\n if (_this.animationAllowed) {\n if (val) {\n _this.isAnimatingCollapseExpand = true;\n }\n else {\n _this.updateElementCss(false);\n }\n }\n }, function () { return _this.renderedIsExpanded; });\n _this.animationAllowedValue = true;\n _this.name = name;\n _this.createNewArray(\"errors\");\n _this.createNewArray(\"titleActions\");\n _this.registerPropertyChangedHandlers([\"isReadOnly\"], function () { _this.onReadOnlyChanged(); });\n _this.registerPropertyChangedHandlers([\"errors\"], function () { _this.updateVisibleErrors(); });\n _this.registerPropertyChangedHandlers([\"isSingleInRow\"], function () { _this.updateElementCss(false); });\n return _this;\n }\n SurveyElement.getProgressInfoByElements = function (children, isRequired) {\n var info = _base__WEBPACK_IMPORTED_MODULE_1__[\"Base\"].createProgressInfo();\n for (var i = 0; i < children.length; i++) {\n if (!children[i].isVisible)\n continue;\n var childInfo = children[i].getProgressInfo();\n info.questionCount += childInfo.questionCount;\n info.answeredQuestionCount += childInfo.answeredQuestionCount;\n info.requiredQuestionCount += childInfo.requiredQuestionCount;\n info.requiredAnsweredQuestionCount +=\n childInfo.requiredAnsweredQuestionCount;\n }\n if (isRequired && info.questionCount > 0) {\n if (info.requiredQuestionCount == 0)\n info.requiredQuestionCount = 1;\n if (info.answeredQuestionCount > 0)\n info.requiredAnsweredQuestionCount = 1;\n }\n return info;\n };\n SurveyElement.ScrollElementToTop = function (elementId, scrollIfVisible) {\n var root = _settings__WEBPACK_IMPORTED_MODULE_4__[\"settings\"].environment.root;\n if (!elementId || typeof root === \"undefined\")\n return false;\n var el = root.getElementById(elementId);\n return SurveyElement.ScrollElementToViewCore(el, false, scrollIfVisible);\n };\n SurveyElement.ScrollElementToViewCore = function (el, checkLeft, scrollIfVisible) {\n if (!el || !el.scrollIntoView)\n return false;\n var elTop = scrollIfVisible ? -1 : el.getBoundingClientRect().top;\n var needScroll = elTop < 0;\n var elLeft = -1;\n if (!needScroll && checkLeft) {\n elLeft = el.getBoundingClientRect().left;\n needScroll = elLeft < 0;\n }\n if (!needScroll && _global_variables_utils__WEBPACK_IMPORTED_MODULE_9__[\"DomWindowHelper\"].isAvailable()) {\n var height = _global_variables_utils__WEBPACK_IMPORTED_MODULE_9__[\"DomWindowHelper\"].getInnerHeight();\n needScroll = height > 0 && height < elTop;\n if (!needScroll && checkLeft) {\n var width = _global_variables_utils__WEBPACK_IMPORTED_MODULE_9__[\"DomWindowHelper\"].getInnerWidth();\n needScroll = width > 0 && width < elLeft;\n }\n }\n if (needScroll) {\n el.scrollIntoView();\n }\n return needScroll;\n };\n SurveyElement.GetFirstNonTextElement = function (elements, removeSpaces) {\n if (removeSpaces === void 0) { removeSpaces = false; }\n if (!elements || !elements.length || elements.length == 0)\n return null;\n if (removeSpaces) {\n var tEl = elements[0];\n if (tEl.nodeName === \"#text\")\n tEl.data = \"\";\n tEl = elements[elements.length - 1];\n if (tEl.nodeName === \"#text\")\n tEl.data = \"\";\n }\n for (var i = 0; i < elements.length; i++) {\n if (elements[i].nodeName != \"#text\" && elements[i].nodeName != \"#comment\")\n return elements[i];\n }\n return null;\n };\n SurveyElement.FocusElement = function (elementId) {\n if (!elementId || !_global_variables_utils__WEBPACK_IMPORTED_MODULE_9__[\"DomDocumentHelper\"].isAvailable())\n return false;\n var res = SurveyElement.focusElementCore(elementId);\n if (!res) {\n setTimeout(function () {\n SurveyElement.focusElementCore(elementId);\n }, 10);\n }\n return res;\n };\n SurveyElement.focusElementCore = function (elementId) {\n var root = _settings__WEBPACK_IMPORTED_MODULE_4__[\"settings\"].environment.root;\n if (!root)\n return false;\n var el = root.getElementById(elementId);\n // https://stackoverflow.com/questions/19669786/check-if-element-is-visible-in-dom\n if (el && !el[\"disabled\"] && el.style.display !== \"none\" && el.offsetParent !== null) {\n SurveyElement.ScrollElementToViewCore(el, true, false);\n el.focus();\n return true;\n }\n return false;\n };\n SurveyElement.prototype.onPropertyValueChanged = function (name, oldValue, newValue) {\n _super.prototype.onPropertyValueChanged.call(this, name, oldValue, newValue);\n if (name === \"state\") {\n this.updateElementCss(false);\n this.notifyStateChanged(oldValue);\n if (this.stateChangedCallback)\n this.stateChangedCallback();\n }\n };\n SurveyElement.prototype.getSkeletonComponentNameCore = function () {\n if (this.survey) {\n return this.survey.getSkeletonComponentName(this);\n }\n return \"\";\n };\n Object.defineProperty(SurveyElement.prototype, \"parentQuestion\", {\n /**\n * A Dynamic Panel, Dynamic Matrix, or Dropdown Matrix that includes the current question.\n *\n * This property is `null` for standalone questions.\n */\n get: function () {\n return this.parentQuestionValue;\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.setParentQuestion = function (val) {\n this.parentQuestionValue = val;\n this.onParentQuestionChanged();\n };\n SurveyElement.prototype.onParentQuestionChanged = function () { };\n Object.defineProperty(SurveyElement.prototype, \"skeletonComponentName\", {\n get: function () {\n return this.getSkeletonComponentNameCore();\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"state\", {\n /**\n * Gets and sets the survey element's expand state.\n *\n * Possible values:\n *\n * - `\"default\"` (default) - The survey element is displayed in full and cannot be collapsed in the UI.\n * - `\"expanded\"` - The survey element is displayed in full and can be collapsed in the UI.\n * - `\"collapsed\"` - The survey element displays only `title` and `description` and can be expanded in the UI.\n *\n * @see toggleState\n * @see collapse\n * @see expand\n * @see isCollapsed\n * @see isExpanded\n */\n get: function () {\n return this.getPropertyValue(\"state\");\n },\n set: function (val) {\n this.setPropertyValue(\"state\", val);\n this.renderedIsExpanded = !(this.state === \"collapsed\" && !this.isDesignMode);\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.notifyStateChanged = function (prevState) {\n if (this.survey) {\n this.survey.elementContentVisibilityChanged(this);\n }\n };\n Object.defineProperty(SurveyElement.prototype, \"isCollapsed\", {\n /**\n * Returns `true` if the survey element is collapsed.\n * @see state\n * @see toggleState\n * @see collapse\n * @see expand\n * @see isExpanded\n */\n get: function () {\n return this.state === \"collapsed\" && !this.isDesignMode;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"isExpanded\", {\n /**\n * Returns `true` if the survey element is expanded.\n * @see state\n * @see toggleState\n * @see collapse\n * @see expand\n * @see isCollapsed\n */\n get: function () {\n return this.state === \"expanded\";\n },\n enumerable: false,\n configurable: true\n });\n /**\n * Collapses the survey element.\n *\n * In collapsed state, the element displays only `title` and `description`.\n * @see title\n * @see description\n * @see state\n * @see toggleState\n * @see expand\n * @see isCollapsed\n * @see isExpanded\n */\n SurveyElement.prototype.collapse = function () {\n if (this.isDesignMode)\n return;\n this.state = \"collapsed\";\n };\n /**\n * Expands the survey element.\n * @see state\n * @see toggleState\n * @see collapse\n * @see isCollapsed\n * @see isExpanded\n */\n SurveyElement.prototype.expand = function () {\n this.state = \"expanded\";\n };\n /**\n * Toggles the survey element's `state` between collapsed and expanded.\n * @see state\n * @see collapse\n * @see expand\n * @see isCollapsed\n * @see isExpanded\n */\n SurveyElement.prototype.toggleState = function () {\n if (this.isCollapsed) {\n this.expand();\n return true;\n }\n if (this.isExpanded) {\n this.collapse();\n return false;\n }\n return true;\n };\n Object.defineProperty(SurveyElement.prototype, \"hasStateButton\", {\n get: function () {\n return this.isExpanded || this.isCollapsed;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"shortcutText\", {\n get: function () {\n return this.title || this.name;\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.getTitleToolbar = function () {\n if (!this.titleToolbarValue) {\n this.titleToolbarValue = this.createActionContainer(true);\n this.titleToolbarValue.containerCss = (this.isPanel ? this.cssClasses.panel.titleBar : this.cssClasses.titleBar) || \"sv-action-title-bar\";\n this.titleToolbarValue.setItems(this.getTitleActions());\n }\n return this.titleToolbarValue;\n };\n SurveyElement.prototype.createActionContainer = function (allowAdaptiveActions) {\n var actionContainer = allowAdaptiveActions ? new _actions_adaptive_container__WEBPACK_IMPORTED_MODULE_2__[\"AdaptiveActionContainer\"]() : new _actions_container__WEBPACK_IMPORTED_MODULE_5__[\"ActionContainer\"]();\n if (this.survey && !!this.survey.getCss().actionBar) {\n actionContainer.cssClasses = this.survey.getCss().actionBar;\n }\n return actionContainer;\n };\n Object.defineProperty(SurveyElement.prototype, \"titleActions\", {\n get: function () {\n return this.getPropertyValue(\"titleActions\");\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.getTitleActions = function () {\n if (!this.isTitleActionRequested) {\n this.updateTitleActions();\n this.isTitleActionRequested = true;\n }\n return this.titleActions;\n };\n SurveyElement.prototype.getDefaultTitleActions = function () {\n return [];\n };\n SurveyElement.prototype.updateTitleActions = function () {\n var actions = this.getDefaultTitleActions();\n if (!!this.survey) {\n actions = this.survey.getUpdatedElementTitleActions(this, actions);\n }\n this.setPropertyValue(\"titleActions\", actions);\n };\n Object.defineProperty(SurveyElement.prototype, \"hasTitleActions\", {\n get: function () {\n return this.getTitleActions().length > 0;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"hasTitleEvents\", {\n get: function () {\n return this.state !== undefined && this.state !== \"default\";\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"titleTabIndex\", {\n get: function () {\n return !this.isPage && this.state !== \"default\" ? 0 : undefined;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"titleAriaExpanded\", {\n get: function () {\n if (this.isPage || this.state === \"default\")\n return undefined;\n return this.state === \"expanded\" ? \"true\" : \"false\";\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"titleAriaRole\", {\n get: function () {\n if (this.isPage || this.state === \"default\")\n return undefined;\n return \"button\";\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.setSurveyImpl = function (value, isLight) {\n this.surveyImplValue = value;\n if (!this.surveyImplValue) {\n this.setSurveyCore(null);\n this.surveyDataValue = null;\n }\n else {\n this.surveyDataValue = this.surveyImplValue.getSurveyData();\n this.setSurveyCore(this.surveyImplValue.getSurvey());\n this.textProcessorValue = this.surveyImplValue.getTextProcessor();\n this.onSetData();\n }\n if (!!this.survey) {\n this.updateDescriptionVisibility(this.description);\n this.clearCssClasses();\n }\n };\n SurveyElement.prototype.canRunConditions = function () {\n return _super.prototype.canRunConditions.call(this) && !!this.data;\n };\n SurveyElement.prototype.getDataFilteredValues = function () {\n return !!this.data ? this.data.getFilteredValues() : {};\n };\n SurveyElement.prototype.getDataFilteredProperties = function () {\n var props = !!this.data ? this.data.getFilteredProperties() : {};\n props.question = this;\n return props;\n };\n Object.defineProperty(SurveyElement.prototype, \"surveyImpl\", {\n get: function () {\n return this.surveyImplValue;\n },\n enumerable: false,\n configurable: true\n });\n /* You shouldn't use this method ever */\n SurveyElement.prototype.__setData = function (data) {\n if (_settings__WEBPACK_IMPORTED_MODULE_4__[\"settings\"].supportCreatorV2) {\n this.surveyDataValue = data;\n }\n };\n Object.defineProperty(SurveyElement.prototype, \"data\", {\n get: function () {\n return this.surveyDataValue;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"survey\", {\n /**\n * Returns the survey object.\n */\n get: function () {\n return this.getSurvey();\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.getSurvey = function (live) {\n if (live === void 0) { live = false; }\n if (!!this.surveyValue)\n return this.surveyValue;\n if (!!this.surveyImplValue) {\n this.setSurveyCore(this.surveyImplValue.getSurvey());\n }\n return this.surveyValue;\n };\n SurveyElement.prototype.setSurveyCore = function (value) {\n this.surveyValue = value;\n if (!!this.surveyChangedCallback) {\n this.surveyChangedCallback();\n }\n };\n Object.defineProperty(SurveyElement.prototype, \"isInternal\", {\n get: function () {\n return this.isContentElement;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"areInvisibleElementsShowing\", {\n get: function () {\n return (!!this.survey &&\n this.survey.areInvisibleElementsShowing &&\n !this.isContentElement);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"isVisible\", {\n get: function () {\n return true;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"isReadOnly\", {\n /**\n * Returns `true` if the survey element or its parent element is read-only.\n *\n * If you want to switch a survey element to the read-only state based on a condition, specify the [`enableIf`](https://surveyjs.io/form-library/documentation/question#enableIf) property. Refer to the following help topic for information: [Conditional Visibility](https://surveyjs.io/form-library/documentation/design-survey-conditional-logic#conditional-visibility).\n * @see readOnly\n */\n get: function () {\n return this.readOnly;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"readOnly\", {\n /**\n * Makes the survey element read-only.\n *\n * If you want to switch a survey element to the read-only state based on a condition, specify the [`enableIf`](https://surveyjs.io/form-library/documentation/question#enableIf) property. Refer to the following help topic for information: [Conditional Visibility](https://surveyjs.io/form-library/documentation/design-survey-conditional-logic#conditional-visibility).\n * @see isReadOnly\n */\n get: function () {\n return this.getPropertyValue(\"readOnly\");\n },\n set: function (val) {\n if (this.readOnly == val)\n return;\n this.setPropertyValue(\"readOnly\", val);\n if (!this.isLoadingFromJson) {\n this.setPropertyValue(\"isReadOnly\", this.isReadOnly);\n }\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.onReadOnlyChanged = function () {\n if (!!this.readOnlyChangedCallback) {\n this.readOnlyChangedCallback();\n }\n };\n Object.defineProperty(SurveyElement.prototype, \"css\", {\n get: function () {\n return !!this.survey ? this.survey.getCss() : {};\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"cssClassesValue\", {\n get: function () {\n return this.getPropertyValueWithoutDefault(\"cssClassesValue\");\n },\n set: function (val) {\n this.setPropertyValue(\"cssClassesValue\", val);\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.ensureCssClassesValue = function () {\n if (!this.cssClassesValue) {\n this.cssClassesValue = this.calcCssClasses(this.css);\n this.updateElementCssCore(this.cssClassesValue);\n }\n };\n Object.defineProperty(SurveyElement.prototype, \"cssClasses\", {\n /**\n * Returns an object in which keys are UI elements and values are CSS classes applied to them.\n *\n * Use the following events of the [`SurveyModel`](https://surveyjs.io/form-library/documentation/surveymodel) object to override CSS classes:\n *\n * - [`onUpdateQuestionCssClasses`](https://surveyjs.io/form-library/documentation/surveymodel#onUpdateQuestionCssClasses)\n * - [`onUpdatePanelCssClasses`](https://surveyjs.io/form-library/documentation/surveymodel#onUpdatePanelCssClasses)\n * - [`onUpdatePageCssClasses`](https://surveyjs.io/form-library/documentation/surveymodel#onUpdatePageCssClasses)\n * - [`onUpdateChoiceItemCss`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#onUpdateChoiceItemCss)\n */\n get: function () {\n var _dummy = this.cssClassesValue;\n if (!this.survey)\n return this.calcCssClasses(this.css);\n this.ensureCssClassesValue();\n return this.cssClassesValue;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"cssTitleNumber\", {\n get: function () {\n var css = this.cssClasses;\n if (css.number)\n return css.number;\n return css.panel ? css.panel.number : undefined;\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.calcCssClasses = function (css) { return undefined; };\n SurveyElement.prototype.updateElementCssCore = function (cssClasses) { };\n Object.defineProperty(SurveyElement.prototype, \"cssError\", {\n get: function () { return \"\"; },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.updateElementCss = function (reNew) {\n this.clearCssClasses();\n };\n SurveyElement.prototype.clearCssClasses = function () {\n this.cssClassesValue = undefined;\n };\n SurveyElement.prototype.getIsLoadingFromJson = function () {\n if (_super.prototype.getIsLoadingFromJson.call(this))\n return true;\n return this.surveyValue ? this.surveyValue.isLoadingFromJson : false;\n };\n Object.defineProperty(SurveyElement.prototype, \"name\", {\n /**\n * A survey element identifier.\n *\n * > Question names must be unique.\n */\n get: function () {\n return this.getPropertyValue(\"name\", \"\");\n },\n set: function (val) {\n var oldValue = this.name;\n this.setPropertyValue(\"name\", this.getValidName(val));\n if (!this.isLoadingFromJson && !!oldValue) {\n this.onNameChanged(oldValue);\n }\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.getValidName = function (name) {\n return name;\n };\n SurveyElement.prototype.onNameChanged = function (oldValue) { };\n SurveyElement.prototype.updateBindingValue = function (valueName, value) {\n if (!!this.data &&\n !this.isTwoValueEquals(value, this.data.getValue(valueName))) {\n this.data.setValue(valueName, value, false);\n }\n };\n Object.defineProperty(SurveyElement.prototype, \"errors\", {\n /**\n * Validation errors. Call the `validate()` method to validate survey element data.\n * @see validate\n */\n get: function () {\n return this.getPropertyValue(\"errors\");\n },\n set: function (val) {\n this.setPropertyValue(\"errors\", val);\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.updateVisibleErrors = function () {\n var counter = 0;\n for (var i = 0; i < this.errors.length; i++) {\n if (this.errors[i].visible)\n counter++;\n }\n this.hasVisibleErrors = counter > 0;\n };\n Object.defineProperty(SurveyElement.prototype, \"containsErrors\", {\n /**\n * Returns `true` if the survey element or its child elements have validation errors.\n *\n * This property contains the result of the most recent validation. This result may be outdated. Call the `validate` method to get an up-to-date value.\n * @see errors\n */\n get: function () {\n return this.getPropertyValue(\"containsErrors\", false);\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.updateContainsErrors = function () {\n this.setPropertyValue(\"containsErrors\", this.getContainsErrors());\n };\n SurveyElement.prototype.getContainsErrors = function () {\n return this.errors.length > 0;\n };\n Object.defineProperty(SurveyElement.prototype, \"selectedElementInDesign\", {\n get: function () {\n return this.selectedElementInDesignValue;\n },\n set: function (val) {\n this.selectedElementInDesignValue = val;\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.updateCustomWidgets = function () { };\n SurveyElement.prototype.onSurveyLoad = function () { };\n Object.defineProperty(SurveyElement.prototype, \"wasRendered\", {\n get: function () { return !!this.wasRenderedValue; },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.onFirstRendering = function () {\n this.wasRenderedValue = true;\n this.ensureCssClassesValue();\n };\n SurveyElement.prototype.endLoadingFromJson = function () {\n _super.prototype.endLoadingFromJson.call(this);\n if (!this.survey) {\n this.onSurveyLoad();\n }\n this.updateDescriptionVisibility(this.description);\n };\n SurveyElement.prototype.setVisibleIndex = function (index) {\n return 0;\n };\n Object.defineProperty(SurveyElement.prototype, \"isPage\", {\n /**\n * Returns `true` if the survey element is a page.\n * @see Base.getType\n */\n get: function () {\n return false;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"isPanel\", {\n /**\n * Returns `true` if the survey element is a panel.\n * @see Base.getType\n */\n get: function () {\n return false;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"isQuestion\", {\n /**\n * Returns `true` if the survey element is a question.\n * @see Base.getType\n */\n get: function () {\n return false;\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.delete = function (doDispose) { };\n /**\n * Returns the survey's [locale](https://surveyjs.io/form-library/documentation/surveymodel#locale).\n *\n * If a default locale is used, this method returns an empty string. To get the applied locale in this case, use the following code:\n *\n * ```js\n * import { surveyLocalization } from 'survey-core';\n * const defaultLocale = surveyLocalization.defaultLocale;\n * ```\n *\n * @see [Localization & Globalization](https://surveyjs.io/form-library/documentation/localization)\n */\n SurveyElement.prototype.getLocale = function () {\n return this.survey\n ? this.survey.getLocale()\n : this.locOwner\n ? this.locOwner.getLocale()\n : \"\";\n };\n SurveyElement.prototype.getMarkdownHtml = function (text, name) {\n return this.survey\n ? this.survey.getSurveyMarkdownHtml(this, text, name)\n : this.locOwner\n ? this.locOwner.getMarkdownHtml(text, name)\n : undefined;\n };\n SurveyElement.prototype.getRenderer = function (name) {\n return this.survey && typeof this.survey.getRendererForString === \"function\"\n ? this.survey.getRendererForString(this, name)\n : this.locOwner && typeof this.locOwner.getRenderer === \"function\"\n ? this.locOwner.getRenderer(name)\n : null;\n };\n SurveyElement.prototype.getRendererContext = function (locStr) {\n return this.survey && typeof this.survey.getRendererContextForString === \"function\"\n ? this.survey.getRendererContextForString(this, locStr)\n : this.locOwner && typeof this.locOwner.getRendererContext === \"function\"\n ? this.locOwner.getRendererContext(locStr)\n : locStr;\n };\n SurveyElement.prototype.getProcessedText = function (text) {\n if (this.isLoadingFromJson)\n return text;\n if (this.textProcessor)\n return this.textProcessor.processText(text, this.getUseDisplayValuesInDynamicTexts());\n if (this.locOwner)\n return this.locOwner.getProcessedText(text);\n return text;\n };\n SurveyElement.prototype.getUseDisplayValuesInDynamicTexts = function () { return true; };\n SurveyElement.prototype.removeSelfFromList = function (list) {\n if (!list || !Array.isArray(list))\n return;\n var index = list.indexOf(this);\n if (index > -1) {\n list.splice(index, 1);\n }\n };\n Object.defineProperty(SurveyElement.prototype, \"textProcessor\", {\n get: function () {\n return this.textProcessorValue;\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.getProcessedHtml = function (html) {\n if (!html || !this.textProcessor)\n return html;\n return this.textProcessor.processText(html, true);\n };\n SurveyElement.prototype.onSetData = function () { };\n Object.defineProperty(SurveyElement.prototype, \"parent\", {\n get: function () {\n return this.getPropertyValue(\"parent\", null);\n },\n set: function (val) {\n this.setPropertyValue(\"parent\", val);\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.getPage = function (parent) {\n while (parent && parent.parent)\n parent = parent.parent;\n if (parent && parent.getType() == \"page\")\n return parent;\n return null;\n };\n SurveyElement.prototype.moveToBase = function (parent, container, insertBefore) {\n if (insertBefore === void 0) { insertBefore = null; }\n if (!container)\n return false;\n parent.removeElement(this);\n var index = -1;\n if (_helpers__WEBPACK_IMPORTED_MODULE_3__[\"Helpers\"].isNumber(insertBefore)) {\n index = parseInt(insertBefore);\n }\n if (index == -1 && !!insertBefore && !!insertBefore.getType) {\n index = container.indexOf(insertBefore);\n }\n container.addElement(this, index);\n return true;\n };\n SurveyElement.prototype.setPage = function (parent, newPage) {\n var oldPage = this.getPage(parent);\n //fix for the creator v1: https://github.com/surveyjs/survey-creator/issues/1744\n if (typeof newPage === \"string\") {\n var survey = this.getSurvey();\n survey.pages.forEach(function (page) {\n if (newPage === page.name)\n newPage = page;\n });\n }\n if (oldPage === newPage)\n return;\n if (parent)\n parent.removeElement(this);\n if (newPage) {\n newPage.addElement(this, -1);\n }\n };\n SurveyElement.prototype.getSearchableLocKeys = function (keys) {\n keys.push(\"title\");\n keys.push(\"description\");\n };\n Object.defineProperty(SurveyElement.prototype, \"isDefaultV2Theme\", {\n get: function () {\n return this.survey && this.survey.getCss().root == \"sd-root-modern\";\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"hasParent\", {\n get: function () {\n return (this.parent && !this.parent.isPage && (!this.parent.originalPage)) || (this.parent === undefined);\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.shouldAddRunnerStyles = function () {\n return !this.isDesignMode && this.isDefaultV2Theme;\n };\n Object.defineProperty(SurveyElement.prototype, \"isCompact\", {\n get: function () {\n return this.survey && this.survey[\"isCompact\"];\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.canHaveFrameStyles = function () {\n return (this.parent !== undefined && (!this.hasParent || this.parent && this.parent.showPanelAsPage));\n };\n SurveyElement.prototype.getHasFrameV2 = function () {\n return this.shouldAddRunnerStyles() && this.canHaveFrameStyles();\n };\n SurveyElement.prototype.getIsNested = function () {\n return this.shouldAddRunnerStyles() && !this.canHaveFrameStyles();\n };\n SurveyElement.prototype.getCssRoot = function (cssClasses) {\n var isExpanadable = !!this.isCollapsed || !!this.isExpanded;\n return new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_6__[\"CssClassBuilder\"]()\n .append(cssClasses.withFrame, this.getHasFrameV2() && !this.isCompact)\n .append(cssClasses.compact, this.isCompact && this.getHasFrameV2())\n .append(cssClasses.collapsed, !!this.isCollapsed)\n .append(cssClasses.expandableAnimating, isExpanadable && this.isAnimatingCollapseExpand)\n .append(cssClasses.expanded, !!this.isExpanded && this.renderedIsExpanded)\n .append(cssClasses.expandable, isExpanadable)\n .append(cssClasses.nested, this.getIsNested())\n .toString();\n };\n Object.defineProperty(SurveyElement.prototype, \"width\", {\n /**\n * Sets survey element width in CSS values.\n *\n * Default value: \"\"\n * @see minWidth\n * @see maxWidth\n */\n get: function () {\n return this.getPropertyValue(\"width\", \"\");\n },\n set: function (val) {\n this.setPropertyValue(\"width\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"minWidth\", {\n /**\n * Gets or sets minimum survey element width in CSS values.\n *\n * Default value: \"300px\" (taken from [`settings.minWidth`](https://surveyjs.io/form-library/documentation/settings#minWidth))\n * @see maxWidth\n * @see renderWidth\n * @see width\n */\n get: function () {\n return this.getPropertyValue(\"minWidth\");\n },\n set: function (val) {\n this.setPropertyValue(\"minWidth\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"maxWidth\", {\n /**\n * Gets or sets maximum survey element width in CSS values.\n *\n * Default value: \"100%\" (taken from [`settings.maxWidth`](https://surveyjs.io/form-library/documentation/settings#maxWidth))\n * @see minWidth\n * @see renderWidth\n * @see width\n */\n get: function () {\n return this.getPropertyValue(\"maxWidth\");\n },\n set: function (val) {\n this.setPropertyValue(\"maxWidth\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"renderWidth\", {\n /**\n * Returns a calculated width of the rendered survey element in CSS values.\n * @see width\n * @see minWidth\n * @see maxWidth\n */\n get: function () {\n return this.getPropertyValue(\"renderWidth\", \"\");\n },\n set: function (val) {\n this.setPropertyValue(\"renderWidth\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"indent\", {\n /**\n * Increases or decreases an indent of survey element content from the left edge. Accepts positive integer values and 0. Does not apply in the Default V2 theme.\n * @see rightIndent\n */\n get: function () {\n return this.getPropertyValue(\"indent\");\n },\n set: function (val) {\n this.setPropertyValue(\"indent\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"rightIndent\", {\n /**\n * Increases or decreases an indent of survey element content from the right edge. Accepts positive integer values and 0. Does not apply in the Default V2 theme.\n * @see indent\n */\n get: function () {\n return this.getPropertyValue(\"rightIndent\", 0);\n },\n set: function (val) {\n this.setPropertyValue(\"rightIndent\", val);\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.getRootStyle = function () {\n var style = {};\n if (!!this.paddingLeft) {\n style[\"--sv-element-add-padding-left\"] = this.paddingLeft;\n }\n if (!!this.paddingRight) {\n style[\"--sv-element-add-padding-right\"] = this.paddingRight;\n }\n return style;\n };\n Object.defineProperty(SurveyElement.prototype, \"paddingLeft\", {\n get: function () {\n return this.getPropertyValue(\"paddingLeft\", \"\");\n },\n set: function (val) {\n this.setPropertyValue(\"paddingLeft\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"paddingRight\", {\n get: function () {\n return this.getPropertyValue(\"paddingRight\", \"\");\n },\n set: function (val) {\n this.setPropertyValue(\"paddingRight\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"rootStyle\", {\n get: function () {\n var style = {};\n var minWidth = this.minWidth;\n if (minWidth != \"auto\")\n minWidth = \"min(100%, \" + this.minWidth + \")\";\n if (this.allowRootStyle && this.renderWidth) {\n // style[\"width\"] = this.renderWidth;\n style[\"flexGrow\"] = 1;\n style[\"flexShrink\"] = 1;\n style[\"flexBasis\"] = this.renderWidth;\n style[\"minWidth\"] = minWidth;\n style[\"maxWidth\"] = this.maxWidth;\n }\n return style;\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.isContainsSelection = function (el) {\n var elementWithSelection = undefined;\n var _document = _global_variables_utils__WEBPACK_IMPORTED_MODULE_9__[\"DomDocumentHelper\"].getDocument();\n if (_global_variables_utils__WEBPACK_IMPORTED_MODULE_9__[\"DomDocumentHelper\"].isAvailable() && !!_document && _document[\"selection\"]) {\n elementWithSelection = _document[\"selection\"].createRange().parentElement();\n }\n else {\n var selection = _global_variables_utils__WEBPACK_IMPORTED_MODULE_9__[\"DomWindowHelper\"].getSelection();\n if (!!selection && selection.rangeCount > 0) {\n var range = selection.getRangeAt(0);\n if (range.startOffset !== range.endOffset) {\n elementWithSelection = range.startContainer.parentNode;\n }\n }\n }\n return elementWithSelection == el;\n };\n Object.defineProperty(SurveyElement.prototype, \"clickTitleFunction\", {\n get: function () {\n var _this = this;\n if (this.needClickTitleFunction()) {\n return function (event) {\n if (!!event && _this.isContainsSelection(event.target)) {\n return;\n }\n return _this.processTitleClick();\n };\n }\n return undefined;\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.needClickTitleFunction = function () {\n return this.state !== \"default\";\n };\n SurveyElement.prototype.processTitleClick = function () {\n if (this.state !== \"default\") {\n this.toggleState();\n }\n };\n Object.defineProperty(SurveyElement.prototype, \"additionalTitleToolbar\", {\n get: function () {\n return this.getAdditionalTitleToolbar();\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.getAdditionalTitleToolbar = function () {\n return null;\n };\n SurveyElement.prototype.getCssTitle = function (cssClasses) {\n var isExpandable = this.state !== \"default\";\n var numInlineLimit = 4;\n return new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_6__[\"CssClassBuilder\"]()\n .append(cssClasses.title)\n .append(cssClasses.titleNumInline, (this.no || \"\").length > numInlineLimit || isExpandable)\n .append(cssClasses.titleExpandable, isExpandable)\n .append(cssClasses.titleExpanded, this.isExpanded)\n .append(cssClasses.titleCollapsed, this.isCollapsed)\n .append(cssClasses.titleDisabled, this.isDisabledStyle)\n .append(cssClasses.titleReadOnly, this.isReadOnly)\n .append(cssClasses.titleOnError, this.containsErrors).toString();\n };\n Object.defineProperty(SurveyElement.prototype, \"isDisabledStyle\", {\n get: function () {\n return !this.isDefaultV2Theme && (this.isReadOnlyStyle || this.isPreviewStyle);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"isReadOnlyStyle\", {\n get: function () {\n return this.isReadOnly && !this.isPreviewStyle;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"isPreviewStyle\", {\n get: function () {\n return !!this.survey && this.survey.state === \"preview\";\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.localeChanged = function () {\n _super.prototype.localeChanged.call(this);\n this.updateDescriptionVisibility(this.description);\n if (this.errors.length > 0) {\n this.errors.forEach(function (err) {\n err.updateText();\n });\n }\n };\n SurveyElement.prototype.setWrapperElement = function (element) {\n this.wrapperElement = element;\n };\n SurveyElement.prototype.getWrapperElement = function () {\n return this.wrapperElement;\n };\n Object.defineProperty(SurveyElement.prototype, \"isAnimatingCollapseExpand\", {\n get: function () {\n return this._isAnimatingCollapseExpand || this._renderedIsExpanded != this.isExpanded;\n },\n set: function (val) {\n if (val !== this._isAnimatingCollapseExpand) {\n this._isAnimatingCollapseExpand = val;\n this.updateElementCss(false);\n }\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.getExpandCollapseAnimationOptions = function () {\n var _this = this;\n var beforeRunAnimation = function (el) {\n _this.isAnimatingCollapseExpand = true;\n el.style.setProperty(\"--animation-height\", el.offsetHeight + \"px\");\n };\n var afterRunAnimation = function (el) {\n _this.isAnimatingCollapseExpand = false;\n };\n return {\n getEnterOptions: function () {\n var cssClasses = _this.isPanel ? _this.cssClasses.panel : _this.cssClasses;\n return {\n cssClass: cssClasses.contentFadeIn,\n onBeforeRunAnimation: beforeRunAnimation,\n onAfterRunAnimation: afterRunAnimation,\n };\n },\n getLeaveOptions: function () {\n var cssClasses = _this.isPanel ? _this.cssClasses.panel : _this.cssClasses;\n return { cssClass: cssClasses.contentFadeOut,\n onBeforeRunAnimation: beforeRunAnimation,\n onAfterRunAnimation: afterRunAnimation\n };\n },\n getAnimatedElement: function () {\n var _a;\n var cssClasses = _this.isPanel ? _this.cssClasses.panel : _this.cssClasses;\n if (cssClasses.content) {\n var selector = Object(_utils_utils__WEBPACK_IMPORTED_MODULE_8__[\"classesToSelector\"])(cssClasses.content);\n if (selector) {\n return (_a = _this.getWrapperElement()) === null || _a === void 0 ? void 0 : _a.querySelector(selector);\n }\n }\n return undefined;\n },\n isAnimationEnabled: function () { return _settings__WEBPACK_IMPORTED_MODULE_4__[\"settings\"].animationEnabled && _this.animationAllowed && !_this.isDesignMode; }\n };\n };\n Object.defineProperty(SurveyElement.prototype, \"renderedIsExpanded\", {\n get: function () {\n return !!this._renderedIsExpanded;\n },\n set: function (val) {\n this.animationCollapsed.sync(val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyElement.prototype, \"animationAllowed\", {\n get: function () {\n return !this.isLoadingFromJson && !this.isDisposed && !!this.survey && this.animationAllowedValue;\n },\n set: function (val) {\n this.animationAllowedValue = val;\n },\n enumerable: false,\n configurable: true\n });\n SurveyElement.prototype.dispose = function () {\n _super.prototype.dispose.call(this);\n if (this.titleToolbarValue) {\n this.titleToolbarValue.dispose();\n }\n };\n SurveyElement.CreateDisabledDesignElements = false;\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_0__[\"property\"])({ defaultValue: null })\n ], SurveyElement.prototype, \"dragTypeOverMe\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_0__[\"property\"])({ defaultValue: false })\n ], SurveyElement.prototype, \"isDragMe\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_0__[\"property\"])({ defaultValue: false })\n ], SurveyElement.prototype, \"hasVisibleErrors\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_0__[\"property\"])({ defaultValue: true })\n ], SurveyElement.prototype, \"isSingleInRow\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_0__[\"property\"])({ defaultValue: true })\n ], SurveyElement.prototype, \"allowRootStyle\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_0__[\"property\"])()\n ], SurveyElement.prototype, \"_renderedIsExpanded\", void 0);\n return SurveyElement;\n}(SurveyElementCore));\n\n\n\n/***/ }),\n\n/***/ \"./src/survey-error.ts\":\n/*!*****************************!*\\\n !*** ./src/survey-error.ts ***!\n \\*****************************/\n/*! exports provided: SurveyError */\n/***/ (function(module, __webpack_exports__, __webpack_require__) {\n\n\"use strict\";\n__webpack_require__.r(__webpack_exports__);\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"SurveyError\", function() { return SurveyError; });\n/* harmony import */ var _localizablestring__WEBPACK_IMPORTED_MODULE_0__ = __webpack_require__(/*! ./localizablestring */ \"./src/localizablestring.ts\");\n/* harmony import */ var _surveyStrings__WEBPACK_IMPORTED_MODULE_1__ = __webpack_require__(/*! ./surveyStrings */ \"./src/surveyStrings.ts\");\n\n\nvar SurveyError = /** @class */ (function () {\n function SurveyError(text, errorOwner) {\n if (text === void 0) { text = null; }\n if (errorOwner === void 0) { errorOwner = null; }\n this.text = text;\n this.errorOwner = errorOwner;\n this.visible = true;\n this.onUpdateErrorTextCallback = undefined;\n }\n SurveyError.prototype.equals = function (error) {\n if (!error || !error.getErrorType)\n return false;\n if (this.getErrorType() !== error.getErrorType())\n return false;\n return this.text === error.text && this.visible === error.visible;\n };\n Object.defineProperty(SurveyError.prototype, \"locText\", {\n get: function () {\n if (!this.locTextValue) {\n this.locTextValue = new _localizablestring__WEBPACK_IMPORTED_MODULE_0__[\"LocalizableString\"](this.errorOwner, true);\n this.locTextValue.storeDefaultText = true;\n this.locTextValue.text = this.getText();\n }\n return this.locTextValue;\n },\n enumerable: false,\n configurable: true\n });\n SurveyError.prototype.getText = function () {\n var res = this.text;\n if (!res)\n res = this.getDefaultText();\n if (!!this.errorOwner) {\n res = this.errorOwner.getErrorCustomText(res, this);\n }\n return res;\n };\n SurveyError.prototype.getErrorType = function () {\n return \"base\";\n };\n SurveyError.prototype.getDefaultText = function () {\n return \"\";\n };\n SurveyError.prototype.getLocale = function () {\n return !!this.errorOwner ? this.errorOwner.getLocale() : \"\";\n };\n SurveyError.prototype.getLocalizationString = function (locStrName) {\n return _surveyStrings__WEBPACK_IMPORTED_MODULE_1__[\"surveyLocalization\"].getString(locStrName, this.getLocale());\n };\n SurveyError.prototype.updateText = function () {\n if (this.onUpdateErrorTextCallback) {\n this.onUpdateErrorTextCallback(this);\n }\n this.locText.text = this.getText();\n };\n return SurveyError;\n}());\n\n\n\n/***/ }),\n\n/***/ \"./src/survey-events-api.ts\":\n/*!**********************************!*\\\n !*** ./src/survey-events-api.ts ***!\n \\**********************************/\n/*! no exports provided */\n/***/ (function(module, __webpack_exports__, __webpack_require__) {\n\n\"use strict\";\n__webpack_require__.r(__webpack_exports__);\n\n\n\n/***/ }),\n\n/***/ \"./src/survey.ts\":\n/*!***********************!*\\\n !*** ./src/survey.ts ***!\n \\***********************/\n/*! exports provided: SurveyModel */\n/***/ (function(module, __webpack_exports__, __webpack_require__) {\n\n\"use strict\";\n__webpack_require__.r(__webpack_exports__);\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"SurveyModel\", function() { return SurveyModel; });\n/* harmony import */ var _helpers__WEBPACK_IMPORTED_MODULE_0__ = __webpack_require__(/*! ./helpers */ \"./src/helpers.ts\");\n/* harmony import */ var _jsonobject__WEBPACK_IMPORTED_MODULE_1__ = __webpack_require__(/*! ./jsonobject */ \"./src/jsonobject.ts\");\n/* harmony import */ var _base__WEBPACK_IMPORTED_MODULE_2__ = __webpack_require__(/*! ./base */ \"./src/base.ts\");\n/* harmony import */ var _survey_element__WEBPACK_IMPORTED_MODULE_3__ = __webpack_require__(/*! ./survey-element */ \"./src/survey-element.ts\");\n/* harmony import */ var _defaultCss_defaultV2Css__WEBPACK_IMPORTED_MODULE_4__ = __webpack_require__(/*! ./defaultCss/defaultV2Css */ \"./src/defaultCss/defaultV2Css.ts\");\n/* harmony import */ var _textPreProcessor__WEBPACK_IMPORTED_MODULE_5__ = __webpack_require__(/*! ./textPreProcessor */ \"./src/textPreProcessor.ts\");\n/* harmony import */ var _conditionProcessValue__WEBPACK_IMPORTED_MODULE_6__ = __webpack_require__(/*! ./conditionProcessValue */ \"./src/conditionProcessValue.ts\");\n/* harmony import */ var _dxSurveyService__WEBPACK_IMPORTED_MODULE_7__ = __webpack_require__(/*! ./dxSurveyService */ \"./src/dxSurveyService.ts\");\n/* harmony import */ var _surveyStrings__WEBPACK_IMPORTED_MODULE_8__ = __webpack_require__(/*! ./surveyStrings */ \"./src/surveyStrings.ts\");\n/* harmony import */ var _error__WEBPACK_IMPORTED_MODULE_9__ = __webpack_require__(/*! ./error */ \"./src/error.ts\");\n/* harmony import */ var _localizablestring__WEBPACK_IMPORTED_MODULE_10__ = __webpack_require__(/*! ./localizablestring */ \"./src/localizablestring.ts\");\n/* harmony import */ var _stylesmanager__WEBPACK_IMPORTED_MODULE_11__ = __webpack_require__(/*! ./stylesmanager */ \"./src/stylesmanager.ts\");\n/* harmony import */ var _surveyTimerModel__WEBPACK_IMPORTED_MODULE_12__ = __webpack_require__(/*! ./surveyTimerModel */ \"./src/surveyTimerModel.ts\");\n/* harmony import */ var _conditions__WEBPACK_IMPORTED_MODULE_13__ = __webpack_require__(/*! ./conditions */ \"./src/conditions.ts\");\n/* harmony import */ var _settings__WEBPACK_IMPORTED_MODULE_14__ = __webpack_require__(/*! ./settings */ \"./src/settings.ts\");\n/* harmony import */ var _utils_utils__WEBPACK_IMPORTED_MODULE_15__ = __webpack_require__(/*! ./utils/utils */ \"./src/utils/utils.ts\");\n/* harmony import */ var _actions_action__WEBPACK_IMPORTED_MODULE_16__ = __webpack_require__(/*! ./actions/action */ \"./src/actions/action.ts\");\n/* harmony import */ var _actions_container__WEBPACK_IMPORTED_MODULE_17__ = __webpack_require__(/*! ./actions/container */ \"./src/actions/container.ts\");\n/* harmony import */ var _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_18__ = __webpack_require__(/*! ./utils/cssClassBuilder */ \"./src/utils/cssClassBuilder.ts\");\n/* harmony import */ var _notifier__WEBPACK_IMPORTED_MODULE_19__ = __webpack_require__(/*! ./notifier */ \"./src/notifier.ts\");\n/* harmony import */ var _header__WEBPACK_IMPORTED_MODULE_20__ = __webpack_require__(/*! ./header */ \"./src/header.ts\");\n/* harmony import */ var _surveytimer__WEBPACK_IMPORTED_MODULE_21__ = __webpack_require__(/*! ./surveytimer */ \"./src/surveytimer.ts\");\n/* harmony import */ var _surveyTaskManager__WEBPACK_IMPORTED_MODULE_22__ = __webpack_require__(/*! ./surveyTaskManager */ \"./src/surveyTaskManager.ts\");\n/* harmony import */ var _progress_buttons__WEBPACK_IMPORTED_MODULE_23__ = __webpack_require__(/*! ./progress-buttons */ \"./src/progress-buttons.ts\");\n/* harmony import */ var _surveyToc__WEBPACK_IMPORTED_MODULE_24__ = __webpack_require__(/*! ./surveyToc */ \"./src/surveyToc.ts\");\n/* harmony import */ var _global_variables_utils__WEBPACK_IMPORTED_MODULE_25__ = __webpack_require__(/*! ./global_variables_utils */ \"./src/global_variables_utils.ts\");\nvar __extends = (undefined && undefined.__extends) || (function () {\n var extendStatics = function (d, b) {\n extendStatics = Object.setPrototypeOf ||\n ({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) ||\n function (d, b) { for (var p in b) if (Object.prototype.hasOwnProperty.call(b, p)) d[p] = b[p]; };\n return extendStatics(d, b);\n };\n return function (d, b) {\n if (typeof b !== \"function\" && b !== null)\n throw new TypeError(\"Class extends value \" + String(b) + \" is not a constructor or null\");\n extendStatics(d, b);\n function __() { this.constructor = d; }\n d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());\n };\n})();\nvar __decorate = (undefined && undefined.__decorate) || function (decorators, target, key, desc) {\n var c = arguments.length, r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc, d;\n if (typeof Reflect === \"object\" && typeof Reflect.decorate === \"function\") r = Reflect.decorate(decorators, target, key, desc);\n else for (var i = decorators.length - 1; i >= 0; i--) if (d = decorators[i]) r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;\n return c > 3 && r && Object.defineProperty(target, key, r), r;\n};\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n/**\n * The `SurveyModel` object contains properties and methods that allow you to control the survey and access its elements.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/nps-question/ (linkStyle))\n */\nvar SurveyModel = /** @class */ (function (_super) {\n __extends(SurveyModel, _super);\n //#endregion\n function SurveyModel(jsonObj, renderedElement) {\n if (jsonObj === void 0) { jsonObj = null; }\n if (renderedElement === void 0) { renderedElement = null; }\n var _this = _super.call(this) || this;\n _this.valuesHash = {};\n _this.variablesHash = {};\n //#region Event declarations\n /**\n * An event that is raised after a [trigger](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#triggers) is executed.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [Conditional Survey Logic (Triggers)](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#conditional-survey-logic-triggers (linkStyle)).\n * @see triggers\n * @see runTriggers\n */\n _this.onTriggerExecuted = _this.addEvent();\n /**\n * An event that is raised before the survey is completed. Use this event to prevent survey completion.\n * @see onComplete\n * @see doComplete\n * @see allowCompleteSurveyAutomatic\n */\n _this.onCompleting = _this.addEvent();\n /**\n * An event that is raised after the survey is completed. Use this event to send survey results to the server.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * For an example of how to use the methods described above, refer to the following help topic: [Store Survey Results in Your Own Database](https://surveyjs.io/form-library/documentation/handle-survey-results-store#store-survey-results-in-your-own-database).\n *\n * > Do not disable the [`showCompletedPage`](https://surveyjs.io/form-library/documentation/surveymodel#showCompletedPage) property if you call one of the `options.showSave...` methods. This is required because the UI that indicates data saving progress is integrated into the complete page. If you hide the complete page, the UI also becomes invisible.\n * @see onPartialSend\n * @see doComplete\n * @see allowCompleteSurveyAutomatic\n */\n _this.onComplete = _this.addEvent();\n /**\n * An event that is raised before the survey displays a [preview of given answers](https://surveyjs.io/form-library/documentation/design-survey/create-a-multi-page-survey#preview-page). Use this event to cancel the preview.\n * @see showPreviewBeforeComplete\n * @see showPreview\n * @see cancelPreview\n */\n _this.onShowingPreview = _this.addEvent();\n /**\n * An event that is raised before the survey navigates to a specified URL. Use this event to change the URL or cancel the navigation.\n * @see navigateToUrl\n * @see navigateToUrlOnCondition\n */\n _this.onNavigateToUrl = _this.addEvent();\n /**\n * An event that is raised when the survey [`state`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#state) changes to `\"running\"`.\n * @see firstPageIsStarted\n */\n _this.onStarted = _this.addEvent();\n /**\n * An event that is raised to save incomplete survey results. Enable the [`sendResultOnPageNext`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#sendResultOnPageNext) property for this event to occur.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * Alternatively, you can handle the [`onCurrentPageChanged`](#onCurrentPageChanged) and [`onValueChanged`](#onValueChanged) events, as shown in the following demo: [Continue an Incomplete Survey](https://surveyjs.io/form-library/examples/survey-editprevious/).\n */\n _this.onPartialSend = _this.addEvent();\n /**\n * An event that is raised before the current page is switched.\n *\n * @see currentPageNo\n * @see nextPage\n * @see prevPage\n **/\n _this.onCurrentPageChanging = _this.addEvent();\n /**\n * An event that is raised after the current page is switched.\n *\n * @see currentPageNo\n * @see nextPage\n * @see prevPage\n */\n _this.onCurrentPageChanged = _this.addEvent();\n /**\n * An event that is raised before a question value is changed.\n * @see setValue\n */\n _this.onValueChanging = _this.addEvent();\n /**\n * An event that is raised after a question value is changed.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * To handle value changes in matrix cells or panels within a [Dynamic Panel](https://surveyjs.io/form-library/documentation/api-reference/dynamic-panel-model), use the [`onMatrixCellValueChanged`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#onMatrixCellValueChanged) or [`onDynamicPanelItemValueChanged`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#onDynamicPanelItemValueChanged) event.\n * @see setValue\n */\n _this.onValueChanged = _this.addEvent();\n /**\n * An event that is raised after a [variable](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#variables) or [calculated value](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#calculated-values) is changed.\n *\n * @see setVariable\n * @see calculatedValues\n */\n _this.onVariableChanged = _this.addEvent();\n /**\n * An event that is raised after question visibility is changed.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * Refer to the following help topic for information on how to implement conditional visibility: [Conditional Visibility](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#conditional-visibility).\n */\n _this.onQuestionVisibleChanged = _this.addEvent();\n _this.onVisibleChanged = _this.onQuestionVisibleChanged;\n /**\n * An event that is raised after page visibility is changed.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * Refer to the following help topic for information on how to implement conditional visibility: [Conditional Visibility](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#conditional-visibility).\n */\n _this.onPageVisibleChanged = _this.addEvent();\n /**\n * An event that is raised after panel visibility is changed.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * Refer to the following help topic for information on how to implement conditional visibility: [Conditional Visibility](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#conditional-visibility).\n */\n _this.onPanelVisibleChanged = _this.addEvent();\n /**\n * An event that is raised when the survey creates any new object derived from [`Question`](https://surveyjs.io/form-library/documentation/api-reference/question).\n *\n * In a survey, complex elements ([Dynamic Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdynamic/), [Multiple Text](https://surveyjs.io/form-library/examples/questiontype-multipletext/), and [Dynamic Panel](https://surveyjs.io/form-library/examples/questiontype-paneldynamic/)) are composed of questions. Use this event to customize any question regardless of which survey element it belongs to.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * To use this event for questions loaded from JSON, create an empty survey model, add an event handler, and only then populate the model from the JSON object:\n *\n * ```js\n * import { Model } from \"survey-core\";\n *\n * const surveyJson = {\n * // ...\n * };\n * // Create an empty model\n * const survey = new Model();\n * // Add an event handler\n * survey.onQuestionCreated.add((sender, options) => {\n * //...\n * });\n * // Load the survey JSON schema\n * survey.fromJSON(surveyJson);\n * ```\n * @see onQuestionAdded\n */\n _this.onQuestionCreated = _this.addEvent();\n /**\n * An event that is raised when a new question is added to a panel or page.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * To use this event for questions loaded from JSON, create an empty survey model, add an event handler, and only then populate the model from the JSON object:\n *\n * ```js\n * import { Model } from \"survey-core\";\n *\n * const surveyJson = {\n * // ...\n * };\n * // Create an empty model\n * const survey = new Model();\n * // Add an event handler\n * survey.onQuestionAdded.add((sender, options) => {\n * //...\n * });\n * // Load the survey JSON schema\n * survey.fromJSON(surveyJson);\n * ```\n * @see onQuestionCreated\n */\n _this.onQuestionAdded = _this.addEvent();\n /**\n * An event that is raised after a question is deleted from the survey.\n */\n _this.onQuestionRemoved = _this.addEvent();\n /**\n * An event that is raised when a new panel is added to a page.\n */\n _this.onPanelAdded = _this.addEvent();\n /**\n * An event that is raised after a panel is deleted from the survey.\n */\n _this.onPanelRemoved = _this.addEvent();\n /**\n * An event that is raised when a new page is added to the survey.\n * @see PanelModel\n */\n _this.onPageAdded = _this.addEvent();\n /**\n * An event that is raised when a question value is being validated. Use this event to specify a custom error message.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/add-custom-input-validation/ (linkStyle))\n * @see onServerValidateQuestions\n * @see onValidatePanel\n * @see onMatrixCellValidate\n * @see onSettingQuestionErrors\n */\n _this.onValidateQuestion = _this.addEvent();\n /**\n * An event that is raised before errors are assigned to a question. Use this event to add/remove/modify errors.\n * @see onValidateQuestion\n */\n _this.onSettingQuestionErrors = _this.addEvent();\n /**\n * Use this event to validate data on your server.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/javascript-server-side-form-validation/ (linkStyle))\n * @see onValidateQuestion\n * @see onValidatePanel\n * @see isValidatingOnServer\n */\n _this.onServerValidateQuestions = _this.addEvent();\n /**\n * An event that is raised when a panel is being validated. Use this event to specify a custom error message.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/add-custom-input-validation/ (linkStyle))\n * @see onValidateQuestion\n * @see onServerValidateQuestions\n */\n _this.onValidatePanel = _this.addEvent();\n /**\n * An event that is raised to change default error messages.\n */\n _this.onErrorCustomText = _this.addEvent();\n /**\n * An event that is raised when the [current page](#currentPage) is being validated. Handle this event to be notified of current page validation.\n */\n _this.onValidatedErrorsOnCurrentPage = _this.addEvent();\n /**\n * An event that is raised when the survey processes HTML content. Handle this event to modify HTML content before displaying.\n * @see completedHtml\n * @see loadingHtml\n * @see QuestionHtmlModel.html\n */\n _this.onProcessHtml = _this.addEvent();\n /**\n * Use this event to change a question's display text.\n */\n _this.onGetQuestionDisplayValue = _this.addEvent();\n /**\n * An event that is raised before the survey displays a question title. Handle this event to modify question titles.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * If you want to modify question numbers, handle the [`onGetQuestionNo`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#onGetQuestionNo) event.\n * @see requiredText\n */\n _this.onGetQuestionTitle = _this.addEvent();\n /**\n * An event that is raised when the survey calculates heading levels (``, ``, etc.) for a survey, page, panel, and question title. Handle this event to change the heading level of individual titles.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * If you want to specify heading levels for all titles, use the [`titleTags`](https://surveyjs.io/form-library/documentation/api-reference/settings#titleTags) object in [global settings](https://surveyjs.io/form-library/documentation/api-reference/settings).\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-titletagnames/ (linkStyle))\n * @see onGetQuestionTitle\n * @see onGetQuestionNo\n */\n _this.onGetTitleTagName = _this.addEvent();\n /**\n * An event that is raised before the survey calculates a question number. Handle this event to modify question numbers.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * If you want to hide question numbers, disable the [`showQuestionNumbers`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#showQuestionNumbers) property.\n * @see onGetQuestionTitle\n * @see questionStartIndex\n */\n _this.onGetQuestionNo = _this.addEvent();\n /**\n * An event that is raised before the survey displays progress text. Handle this event to change the progress text in code.\n * @see showProgressBar\n * @see progressBarType\n */\n _this.onProgressText = _this.addEvent();\n /**\n * An event that is raised to convert Markdown content to HTML.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/edit-survey-questions-markdown/ (linkStyle))\n */\n _this.onTextMarkdown = _this.addEvent();\n _this.onTextRenderAs = _this.addEvent();\n /**\n * An event that is raised after a request to save survey results on [SurveyJS Service](https://api.surveyjs.io/) has been completed. Use this event to find out if the results have been saved successfully.\n */\n _this.onSendResult = _this.addEvent();\n /**\n * An event that is raised when the [`getResult(resultId, questionName)`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#getResult) method is called. Use this event to obtain answers to an individual question from [SurveyJS Service](https://api.surveyjs.io/).\n * @see getResult\n */\n _this.onGetResult = _this.addEvent();\n /**\n * An event that is raised when Survey Creator opens a dialog window for users to select files.\n * @see onUploadFile\n * @see uploadFiles\n */\n _this.onOpenFileChooser = _this.addEvent();\n /**\n * An event that is raised when a File Upload or Signature Pad question starts to upload a file. Applies only if [`storeDataAsText`](https://surveyjs.io/form-library/documentation/api-reference/file-model#storeDataAsText) is `false`. Use this event to upload files to your server.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/file-upload/ (linkStyle))\n * @see uploadFiles\n * @see onDownloadFile\n * @see onClearFiles\n */\n _this.onUploadFiles = _this.addEvent();\n /**\n * An event that is raised when a File Upload question starts to download a file. Use this event to implement file preview when your server stores only file names.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/store-file-names-in-survey-results/ (linkStyle))\n * @see downloadFile\n * @see onClearFiles\n * @see onUploadFiles\n */\n _this.onDownloadFile = _this.addEvent();\n /**\n * An event that is raised when users clear files in a [File Upload](https://surveyjs.io/form-library/documentation/api-reference/file-model) question or clear signature in a [Signature Pad](https://surveyjs.io/form-library/documentation/api-reference/signature-pad-model) question. Use this event to delete files from your server.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/file-delayed-upload/ (linkStyle))\n * @see clearFiles\n * @see onDownloadFile\n * @see onUploadFiles\n */\n _this.onClearFiles = _this.addEvent();\n /**\n * An event that is raised after choices are loaded from a server but before they are assigned to a choice-based question, such as [Dropdown](https://surveyjs.io/form-library/documentation/api-reference/dropdown-menu-model) or [Checkboxes](https://surveyjs.io/form-library/documentation/api-reference/checkbox-question-model). Handle this event if you need to modify the loaded choices.\n */\n _this.onLoadChoicesFromServer = _this.addEvent();\n /**\n * An event that is raised after a survey JSON schema is loaded from the [SurveyJS Service](https://api.surveyjs.io). Use this event to modify the loaded schema.\n * @see surveyId\n * @see clientId\n * @see loadSurveyFromService\n */\n _this.onLoadedSurveyFromService = _this.addEvent();\n /**\n * An event that is raised when the survey processes [dynamic texts](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#dynamic-texts) and any text in curly brackets. Use this event, for instance, to substitute parameters in a RESTful URL with real values when you [load choices by URL](https://surveyjs.io/form-library/documentation/api-reference/checkbox-question-model#choicesByUrl).\n */\n _this.onProcessTextValue = _this.addEvent();\n /**\n * An event that is raised before rendering a question. Use it to override default question CSS classes.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/customize-survey-with-css/ (linkStyle))\n * @see css\n */\n _this.onUpdateQuestionCssClasses = _this.addEvent();\n /**\n * An event that is raised before rendering a standalone panel and panels within [Dynamic Panel](https://surveyjs.io/form-library/examples/duplicate-group-of-fields-in-form/). Use it to override default panel CSS classes.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/customize-survey-with-css/ (linkStyle))\n * @see css\n */\n _this.onUpdatePanelCssClasses = _this.addEvent();\n /**\n * An event that is raised before rendering a page. Use it to override default page CSS classes.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/customize-survey-with-css/ (linkStyle))\n * @see css\n */\n _this.onUpdatePageCssClasses = _this.addEvent();\n /**\n * An event that is raised before rendering a choice item in Radio Button Group, Checkboxes, and Dropdown questions. Use it to override default CSS classes applied to choice items.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/customize-survey-with-css/ (linkStyle))\n * @see css\n */\n _this.onUpdateChoiceItemCss = _this.addEvent();\n /**\n * An event that is raised after the survey is rendered to the DOM. Use this event to modify survey markup.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-animation/ (linkStyle))\n */\n _this.onAfterRenderSurvey = _this.addEvent();\n _this.onAfterRenderHeader = _this.addEvent();\n /**\n * An event that is raised after a page is rendered to the DOM. Use it to modify page markup.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-afterrender/ (linkStyle))\n */\n _this.onAfterRenderPage = _this.addEvent();\n /**\n * An event that is raised after a question is rendered to the DOM. Use it to modify question markup.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-afterrender/ (linkStyle))\n */\n _this.onAfterRenderQuestion = _this.addEvent();\n /**\n * An event that is raised after a question with a single input field is rendered to the DOM. Use it to modify question markup.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * > This event is not raised for questions without input fields ([HTML](https://surveyjs.io/form-library/documentation/questionhtmlmodel), [Image](https://surveyjs.io/form-library/documentation/questionimagemodel)) or questions with multiple input fields ([Matrix](https://surveyjs.io/form-library/documentation/questionmatrixmodel), [Multiple Text](https://surveyjs.io/form-library/documentation/questionmultipletextmodel)).\n */\n _this.onAfterRenderQuestionInput = _this.addEvent();\n /**\n * An event that is raised after a panel is rendered to the DOM. Use it to modify panel markup.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * > This event is raised for static [Panels](https://surveyjs.io/form-library/examples/set-properties-on-multiple-questions-using-panel/) as well as panels within a [Dynamic Panel](https://surveyjs.io/form-library/examples/duplicate-group-of-fields-in-form/).\n */\n _this.onAfterRenderPanel = _this.addEvent();\n /**\n * An event that is raised when an element (input field, checkbox, radio button) within a question gets focus.\n * @see onFocusInPanel\n * @see focusFirstQuestionAutomatic\n * @see focusQuestion\n */\n _this.onFocusInQuestion = _this.addEvent();\n /**\n * An event that is raised when an element within a panel gets focus.\n * @see onFocusInQuestion\n * @see focusFirstQuestionAutomatic\n * @see focusQuestion\n */\n _this.onFocusInPanel = _this.addEvent();\n /**\n * An event that is raised before a [choice item](https://surveyjs.io/form-library/documentation/api-reference/questionselectbase#choices) is displayed. Use this event to change the visibility of individual choice items in [Checkboxes](https://surveyjs.io/form-library/documentation/api-reference/checkbox-question-model), [Dropdown](https://surveyjs.io/form-library/documentation/api-reference/dropdown-menu-model), [Radio Button Group](https://surveyjs.io/form-library/documentation/api-reference/radio-button-question-model), and other similar question types.\n */\n _this.onShowingChoiceItem = _this.addEvent();\n /**\n * Use this event to load choice items in [Dropdown](https://surveyjs.io/form-library/documentation/questiondropdownmodel) and [Tag Box](https://surveyjs.io/form-library/documentation/questiontagboxmodel) questions on demand.\n *\n * This event is raised only for those questions that have the [`choicesLazyLoadEnabled`](https://surveyjs.io/form-library/documentation/questiondropdownmodel#choicesLazyLoadEnabled) property set to `true`.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/lazy-loading-dropdown/ (linkStyle))\n */\n _this.onChoicesLazyLoad = _this.addEvent();\n /**\n * An event that is raised each time a search string in a [Dropdown](https://surveyjs.io/form-library/documentation/api-reference/dropdown-menu-model) or [Tag Box](https://surveyjs.io/form-library/documentation/api-reference/dropdown-tag-box-model) question changes. Use this event to implement custom filtering of choice options.\n * @see [QuestionDropdownModel.searchEnabled](https://surveyjs.io/form-library/documentation/api-reference/dropdown-menu-model#searchEnabled)\n * @see [QuestionDropdownModel.searchMode](https://surveyjs.io/form-library/documentation/api-reference/dropdown-menu-model#searchMode)\n */\n _this.onChoicesSearch = _this.addEvent();\n /**\n * Use this event to load a display text for the [default choice item](https://surveyjs.io/form-library/documentation/questiondropdownmodel#defaultValue) in [Dropdown](https://surveyjs.io/form-library/documentation/questiondropdownmodel) and [Tag Box](https://surveyjs.io/form-library/documentation/questiontagboxmodel) questions.\n *\n * If you load choices from a server (use [`choicesByUrl`](https://surveyjs.io/form-library/documentation/questiondropdownmodel#choicesByUrl) or [`onChoicesLazyLoad`](https://surveyjs.io/form-library/documentation/surveymodel#onChoicesLazyLoad)), display texts become available only when data is loaded, which does not happen until a user opens the drop-down menu. However, a display text for a default choice item is required before that. In this case, you can load data individually for the default item within the `onGetChoiceDisplayValue` event handler.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/lazy-loading-dropdown/ (linkStyle))\n */\n _this.onGetChoiceDisplayValue = _this.addEvent();\n /**\n * An event that is raised after a new row is added to a [Dynamic Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdynamic/).\n */\n _this.onMatrixRowAdded = _this.addEvent();\n /**\n * An event that is raised before a new row is added to a [Dynamic Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdynamic/).\n */\n _this.onMatrixRowAdding = _this.addEvent();\n /**\n * This event is obsolete. Use the [`onMatrixRowAdding`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#onMatrixRowAdding) event instead.\n */\n _this.onMatrixBeforeRowAdded = _this.onMatrixRowAdding;\n /**\n * An event that is raised before a row is deleted from a [Dynamic Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdynamic/). You can cancel row deletion and clear row data instead.\n * @see onMatrixRenderRemoveButton\n */\n _this.onMatrixRowRemoving = _this.addEvent();\n /**\n * An event that is raised after a row is deleted from a [Dynamic Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdynamic/).\n * @see onMatrixRenderRemoveButton\n */\n _this.onMatrixRowRemoved = _this.addEvent();\n /**\n * An event that is raised before rendering the Remove button in a row of a [Dynamic Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdynamic/). Use this event to hide the Remove button for individual matrix rows.\n * @see onMatrixRowRemoving\n * @see onMatrixRowRemoved\n */\n _this.onMatrixRenderRemoveButton = _this.addEvent();\n /**\n * This event is obsolete. Use the [`onMatrixRenderRemoveButton`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#onMatrixRenderRemoveButton) event instead.\n */\n _this.onMatrixAllowRemoveRow = _this.onMatrixRenderRemoveButton;\n /**\n * An event that is raised after the visibility of an [expandable detail section](https://surveyjs.io/form-library/examples/add-expandable-details-section-under-matrix-rows/) is changed. This event can be raised for [Multi-Select](https://surveyjs.io/form-library/documentation/api-reference/matrix-table-with-dropdown-list) and [Dynamic Matrix](https://surveyjs.io/form-library/documentation/api-reference/dynamic-matrix-table-question-model) questions.\n */\n _this.onMatrixDetailPanelVisibleChanged = _this.addEvent();\n /**\n * An event that is raised before a cell in a [Multi-Select Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdropdown/) or [Dynamic Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdynamic/) is created. Use this event to change the type of individual matrix cells.\n * @see onAfterRenderMatrixCell\n */\n _this.onMatrixCellCreating = _this.addEvent();\n /**\n * An event that is raised after a cell in a [Multi-Select Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdropdown/) or [Dynamic Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdynamic/) is created.\n * @see onAfterRenderMatrixCell\n */\n _this.onMatrixCellCreated = _this.addEvent();\n /**\n * An event that is raised for every matrix cell after it is rendered to the DOM.\n * @see onMatrixCellCreated\n */\n _this.onAfterRenderMatrixCell = _this.addEvent();\n /**\n * This event is obsolete. Use the [`onAfterRenderMatrixCell`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#onAfterRenderMatrixCell) event instead.\n */\n _this.onMatrixAfterCellRender = _this.onAfterRenderMatrixCell;\n /**\n * An event that is raised after a cell value is changed in a [Multi-Select Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdropdown/) or [Dynamic Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdynamic/).\n * @see onMatrixRowAdding\n */\n _this.onMatrixCellValueChanged = _this.addEvent();\n /**\n * An event that is raised before a cell value is changed in a [Multi-Select Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdropdown/) or [Dynamic Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdynamic/). Use this event to change the cell value.\n * @see onMatrixRowAdding\n */\n _this.onMatrixCellValueChanging = _this.addEvent();\n /**\n * An event that is raised for [Multi-Select Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdropdown/) and [Dynamic Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdynamic/) questions when they validate a cell value. Use this event to display a custom error message based on a condition.\n * @see onMatrixRowAdding\n */\n _this.onMatrixCellValidate = _this.addEvent();\n /**\n * An event that is raised after a new column is added to a [Multi-Select Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdropdown/) or [Dynamic Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdynamic/).\n */\n _this.onMatrixColumnAdded = _this.addEvent();\n /**\n * An event that is raised on adding a new item in Multiple Text question.\n */\n _this.onMultipleTextItemAdded = _this.addEvent();\n /**\n * An event that is raised after a new panel is added to a [Dynamic Panel](https://surveyjs.io/form-library/examples/questiontype-paneldynamic/) question.\n */\n _this.onDynamicPanelAdded = _this.addEvent();\n /**\n * An event that is raised after a panel is deleted from a [Dynamic Panel](https://surveyjs.io/form-library/examples/questiontype-paneldynamic/) question.\n */\n _this.onDynamicPanelRemoved = _this.addEvent();\n /**\n * An event that is raised before a panel is deleted from a [Dynamic Panel](https://surveyjs.io/form-library/examples/questiontype-paneldynamic/) question. Use this event to cancel the deletion.\n */\n _this.onDynamicPanelRemoving = _this.addEvent();\n /**\n * An event that is raised every second while the timer is running.\n *\n * Use the [`timeSpent`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#timeSpent) property to find out how many seconds have elapsed.\n * @see maxTimeToFinish\n * @see maxTimeToFinishPage\n * @see showTimerPanel\n * @see startTimer\n */\n _this.onTimer = _this.addEvent();\n _this.onTimerPanelInfoText = _this.addEvent();\n /**\n * An event that is raised after an item value is changed in a panel within a [Dynamic Panel](https://surveyjs.io/form-library/examples/questiontype-paneldynamic/) question.\n */\n _this.onDynamicPanelItemValueChanged = _this.addEvent();\n /**\n * An event that is raised before a [Dynamic Panel](https://surveyjs.io/form-library/examples/questiontype-paneldynamic/) renders [tab titles](https://surveyjs.io/form-library/documentation/api-reference/dynamic-panel-model#templateTabTitle). Use this event to change individual tab titles.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/tabbed-interface-for-duplicate-group-option/ (linkStyle))\n */\n _this.onGetDynamicPanelTabTitle = _this.addEvent();\n /**\n * An event that is raised after the current panel is changed in a [Dynamic Panel](https://surveyjs.io/form-library/examples/questiontype-paneldynamic/) question.\n */\n _this.onDynamicPanelCurrentIndexChanged = _this.addEvent();\n /**\n * An event that is raised to define whether a question answer is correct. Applies only to [quiz surveys](https://surveyjs.io/form-library/documentation/design-survey/create-a-quiz).\n */\n _this.onIsAnswerCorrect = _this.addEvent();\n /**\n * An event that is raised when users drag and drop survey elements while designing the survey in [Survey Creator](https://surveyjs.io/survey-creator/documentation/overview). Use this event to control drag and drop operations.\n * @see isDesignMode\n */\n _this.onDragDropAllow = _this.addEvent();\n /**\n * An event this is raised before a survey element (usually page) is scrolled to the top. Use this event to cancel the scroll operation.\n */\n _this.onScrollingElementToTop = _this.addEvent();\n _this.onLocaleChangedEvent = _this.addEvent();\n /**\n * An event that allows you to add, delete, or modify actions in a question title.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-titleactions/ (linkStyle))\n * @see [IAction](https://surveyjs.io/form-library/documentation/api-reference/iaction)\n */\n _this.onGetQuestionTitleActions = _this.addEvent();\n /**\n * An event that allows you to add, delete, or modify actions in a panel title.\n * @see [IAction](https://surveyjs.io/form-library/documentation/api-reference/iaction)\n */\n _this.onGetPanelTitleActions = _this.addEvent();\n /**\n * An event that allows you to add, delete, or modify actions in a page title.\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/modify-titles-of-survey-elements/ (linkStyle))\n * @see [IAction](https://surveyjs.io/form-library/documentation/api-reference/iaction)\n */\n _this.onGetPageTitleActions = _this.addEvent();\n /**\n * An event that allows you to add, delete, or modify actions in the footer of a [Panel](https://surveyjs.io/form-library/documentation/panelmodel).\n * @see [IAction](https://surveyjs.io/form-library/documentation/api-reference/iaction)\n */\n _this.onGetPanelFooterActions = _this.addEvent();\n /**\n * An event that allows you to add, delete, or modify actions in rows of a [Dynamic Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdynamic/).\n *\n * For information on event handler parameters, refer to descriptions within the interface.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/employee-information-form/ (linkStyle))\n * @see [IAction](https://surveyjs.io/form-library/documentation/api-reference/iaction)\n */\n _this.onGetMatrixRowActions = _this.addEvent();\n /**\n * An event that is raised after a survey element is [expanded or collapsed](https://surveyjs.io/form-library/documentation/api-reference/question#state).\n */\n _this.onElementContentVisibilityChanged = _this.addEvent();\n /**\n * An event that is raised before an [Expression](https://surveyjs.io/form-library/documentation/api-reference/expression-model) question displays a value. Use this event to override the display value.\n */\n _this.onGetExpressionDisplayValue = _this.addEvent();\n /**\n * An event that is raised after the visibility of a popup is changed. This event can be raised for [Single-](https://surveyjs.io/form-library/documentation/api-reference/dropdown-menu-model) and [Multi-Select Dropdown](https://surveyjs.io/form-library/documentation/api-reference/dropdown-tag-box-model) questions and [Rating](https://surveyjs.io/form-library/documentation/api-reference/rating-scale-question-model) questions [rendered as drop-down menus](https://surveyjs.io/form-library/documentation/api-reference/rating-scale-question-model#displayMode).\n */\n _this.onPopupVisibleChanged = _this.addEvent();\n /**\n * A list of errors in a survey JSON schema.\n * @see ensureUniqueNames\n */\n _this.jsonErrors = null;\n _this.cssValue = null;\n /**\n * Specifies whether to hide validation errors thrown by the Required validation in the UI.\n *\n * [Built-In Client-Side Validators](https://surveyjs.io/form-library/documentation/data-validation#built-in-client-side-validators (linkStyle))\n * @see validationEnabled\n * @see validationAllowSwitchPages\n */\n _this.hideRequiredErrors = false;\n //#endregion\n _this.cssVariables = {};\n _this._isMobile = false;\n _this._isCompact = false;\n _this._isDesignMode = false;\n /**\n * Specifies whether data validation is enabled.\n *\n * Default value: `true`\n * @see checkErrorsMode\n * @see hideRequiredErrors\n */\n _this.validationEnabled = true;\n /**\n * Specifies whether respondents can switch the current page even if it contains validation errors.\n *\n * Default value: `false`\n * @see checkErrorsMode\n */\n _this.validationAllowSwitchPages = false;\n /**\n * Specifies whether respondents can end a survey with validation errors.\n *\n * Default value: `false`\n * @see checkErrorsMode\n */\n _this.validationAllowComplete = false;\n _this.isNavigationButtonPressed = false;\n _this.mouseDownPage = null;\n _this.isCalculatingProgressText = false;\n _this.isFirstPageRendering = true;\n _this.isCurrentPageRendering = true;\n _this.isTriggerIsRunning = false;\n _this.triggerValues = null;\n _this.triggerKeys = null;\n _this.conditionValues = null;\n _this.isValueChangedOnRunningCondition = false;\n _this.conditionRunnerCounter = 0;\n _this.conditionUpdateVisibleIndexes = false;\n _this.conditionNotifyElementsOnAnyValueOrVariableChanged = false;\n _this.isEndLoadingFromJson = null;\n _this.questionHashes = {\n names: {},\n namesInsensitive: {},\n valueNames: {},\n valueNamesInsensitive: {},\n };\n _this.needRenderIcons = true;\n _this.skippedPages = [];\n _this.skeletonComponentName = \"sv-skeleton\";\n _this.taskManager = new _surveyTaskManager__WEBPACK_IMPORTED_MODULE_22__[\"SurveyTaskManagerModel\"]();\n if (_global_variables_utils__WEBPACK_IMPORTED_MODULE_25__[\"DomDocumentHelper\"].isAvailable()) {\n SurveyModel.stylesManager = new _stylesmanager__WEBPACK_IMPORTED_MODULE_11__[\"StylesManager\"]();\n }\n var htmlCallBack = function (str) { return \"\" + str + \"
\"; };\n _this.createHtmlLocString(\"completedHtml\", \"completingSurvey\", htmlCallBack);\n _this.createHtmlLocString(\"completedBeforeHtml\", \"completingSurveyBefore\", htmlCallBack, \"completed-before\");\n _this.createHtmlLocString(\"loadingHtml\", \"loadingSurvey\", htmlCallBack, \"loading\");\n _this.createLocalizableString(\"emptySurveyText\", _this, true, \"emptySurvey\");\n _this.createLocalizableString(\"logo\", _this, false);\n _this.createLocalizableString(\"startSurveyText\", _this, false, true);\n _this.createLocalizableString(\"pagePrevText\", _this, false, true);\n _this.createLocalizableString(\"pageNextText\", _this, false, true);\n _this.createLocalizableString(\"completeText\", _this, false, true);\n _this.createLocalizableString(\"previewText\", _this, false, true);\n _this.createLocalizableString(\"editText\", _this, false, true);\n _this.createLocalizableString(\"questionTitleTemplate\", _this, true);\n _this.timerModelValue = new _surveyTimerModel__WEBPACK_IMPORTED_MODULE_12__[\"SurveyTimerModel\"](_this);\n _this.timerModelValue.onTimer = function (page) {\n _this.doTimer(page);\n };\n _this.createNewArray(\"pages\", function (value) {\n _this.doOnPageAdded(value);\n }, function (value) {\n _this.doOnPageRemoved(value);\n });\n _this.createNewArray(\"triggers\", function (value) {\n value.setOwner(_this);\n });\n _this.createNewArray(\"calculatedValues\", function (value) {\n value.setOwner(_this);\n });\n _this.createNewArray(\"completedHtmlOnCondition\", function (value) {\n value.locOwner = _this;\n });\n _this.createNewArray(\"navigateToUrlOnCondition\", function (value) {\n value.locOwner = _this;\n });\n _this.registerPropertyChangedHandlers([\"locale\"], function () {\n _this.onSurveyLocaleChanged();\n });\n _this.registerPropertyChangedHandlers([\"firstPageIsStarted\"], function () {\n _this.onFirstPageIsStartedChanged();\n });\n _this.registerPropertyChangedHandlers([\"mode\"], function () {\n _this.onModeChanged();\n });\n _this.registerPropertyChangedHandlers([\"progressBarType\"], function () {\n _this.updateProgressText();\n });\n _this.registerPropertyChangedHandlers([\"questionStartIndex\", \"requiredText\", \"questionTitlePattern\"], function () {\n _this.resetVisibleIndexes();\n });\n _this.registerPropertyChangedHandlers([\"isLoading\", \"isCompleted\", \"isCompletedBefore\", \"mode\", \"isStartedState\", \"currentPage\", \"isShowingPreview\"], function () { _this.updateState(); });\n _this.registerPropertyChangedHandlers([\"state\", \"currentPage\", \"showPreviewBeforeComplete\"], function () { _this.onStateAndCurrentPageChanged(); });\n _this.registerPropertyChangedHandlers([\"logo\", \"logoPosition\"], function () { _this.updateHasLogo(); });\n _this.registerPropertyChangedHandlers([\"backgroundImage\"], function () { _this.updateRenderBackgroundImage(); });\n _this.registerPropertyChangedHandlers([\"renderBackgroundImage\", \"backgroundOpacity\", \"backgroundImageFit\", \"fitToContainer\", \"backgroundImageAttachment\"], function () {\n _this.updateBackgroundImageStyle();\n });\n _this.registerPropertyChangedHandlers([\"showPrevButton\", \"showCompleteButton\"], function () { _this.updateButtonsVisibility(); });\n _this.onGetQuestionNo.onCallbacksChanged = function () {\n _this.resetVisibleIndexes();\n };\n _this.onProgressText.onCallbacksChanged = function () {\n _this.updateProgressText();\n };\n _this.onTextMarkdown.onCallbacksChanged = function () {\n _this.locStrsChanged();\n };\n _this.onProcessHtml.onCallbacksChanged = function () {\n _this.locStrsChanged();\n };\n _this.onGetQuestionTitle.onCallbacksChanged = function () {\n _this.locStrsChanged();\n };\n _this.onUpdatePageCssClasses.onCallbacksChanged = function () {\n _this.currentPage && _this.currentPage.updateElementCss();\n };\n _this.onUpdatePanelCssClasses.onCallbacksChanged = function () {\n _this.currentPage && _this.currentPage.updateElementCss();\n };\n _this.onUpdateQuestionCssClasses.onCallbacksChanged = function () {\n _this.currentPage && _this.currentPage.updateElementCss();\n };\n _this.onShowingChoiceItem.onCallbacksChanged = function () {\n _this.rebuildQuestionChoices();\n };\n _this.navigationBarValue = _this.createNavigationBar();\n _this.navigationBar.locOwner = _this;\n _this.onBeforeCreating();\n if (jsonObj) {\n if (typeof jsonObj === \"string\" || jsonObj instanceof String) {\n jsonObj = JSON.parse(jsonObj);\n }\n if (jsonObj && jsonObj.clientId) {\n _this.clientId = jsonObj.clientId;\n }\n _this.fromJSON(jsonObj);\n if (_this.surveyId) {\n _this.loadSurveyFromService(_this.surveyId, _this.clientId);\n }\n }\n _this.onCreating();\n if (!!renderedElement) {\n _this.render(renderedElement);\n }\n _this.updateCss();\n _this.setCalculatedWidthModeUpdater();\n _this.notifier = new _notifier__WEBPACK_IMPORTED_MODULE_19__[\"Notifier\"](_this.css.saveData);\n _this.notifier.addAction(_this.createTryAgainAction(), \"error\");\n _this.onPopupVisibleChanged.add(function (_, opt) {\n if (opt.visible) {\n _this.onScrollCallback = function () {\n opt.popup.toggleVisibility();\n };\n }\n else {\n _this.onScrollCallback = undefined;\n }\n });\n _this.progressBarValue = new _progress_buttons__WEBPACK_IMPORTED_MODULE_23__[\"ProgressButtons\"](_this);\n _this.layoutElements.push({\n id: \"timerpanel\",\n template: \"survey-timerpanel\",\n component: \"sv-timerpanel\",\n data: _this.timerModel\n });\n _this.layoutElements.push({\n id: \"progress-buttons\",\n component: \"sv-progress-buttons\",\n data: _this.progressBar,\n processResponsiveness: function (width) { return _this.progressBar.processResponsiveness && _this.progressBar.processResponsiveness(width); }\n });\n _this.layoutElements.push({\n id: \"progress-questions\",\n component: \"sv-progress-questions\",\n data: _this\n });\n _this.layoutElements.push({\n id: \"progress-pages\",\n component: \"sv-progress-pages\",\n data: _this\n });\n _this.layoutElements.push({\n id: \"progress-correctquestions\",\n component: \"sv-progress-correctquestions\",\n data: _this\n });\n _this.layoutElements.push({\n id: \"progress-requiredquestions\",\n component: \"sv-progress-requiredquestions\",\n data: _this\n });\n _this.addLayoutElement({\n id: \"toc-navigation\",\n component: \"sv-navigation-toc\",\n data: new _surveyToc__WEBPACK_IMPORTED_MODULE_24__[\"TOCModel\"](_this)\n });\n _this.layoutElements.push({\n id: \"buttons-navigation\",\n component: \"sv-action-bar\",\n data: _this.navigationBar\n });\n _this.locTitle.onStringChanged.add(function () { return _this.titleIsEmpty = _this.locTitle.isEmpty; });\n return _this;\n }\n Object.defineProperty(SurveyModel, \"cssType\", {\n get: function () {\n return _defaultCss_defaultV2Css__WEBPACK_IMPORTED_MODULE_4__[\"surveyCss\"].currentType;\n },\n set: function (value) {\n _stylesmanager__WEBPACK_IMPORTED_MODULE_11__[\"StylesManager\"].applyTheme(value);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"platformName\", {\n get: function () {\n return SurveyModel.platform;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"commentSuffix\", {\n /**\n * A suffix added to the name of the property that stores comments.\n *\n * Default value: \"-Comment\"\n *\n * Many question types allow respondents to leave comments. To enable this functionality, set a question's [`showCommentArea`](https://surveyjs.io/form-library/documentation/api-reference/checkbox-question-model#showCommentArea) property to `true`. Comment values are saved in a separate property. The property name is composed of the question `name` and `commentSuffix`.\n *\n * Respondents can also leave comments when they select \"Other\" in choice-based questions, such as Dropdown or Checkboxes. The property name for the comment value is composed according to the same rules. However, you can use the question `name` as a key to store the comment value instead. Disable the [`storeOthersAsComment`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#storeOthersAsComment) property in this case.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/create-checkboxes-question-in-javascript/ (linkStyle))\n */\n get: function () {\n return _settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].commentSuffix;\n },\n set: function (val) {\n _settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].commentSuffix = val;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"commentPrefix\", {\n get: function () {\n return this.commentSuffix;\n },\n set: function (val) {\n this.commentSuffix = val;\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.processClosedPopup = function (question, popupModel) {\n throw new Error(\"Method not implemented.\");\n };\n SurveyModel.prototype.createTryAgainAction = function () {\n var _this = this;\n return {\n id: \"save-again\",\n title: this.getLocalizationString(\"saveAgainButton\"),\n action: function () {\n if (_this.isCompleted) {\n _this.saveDataOnComplete();\n }\n else {\n _this.doComplete();\n }\n }\n };\n };\n SurveyModel.prototype.createHtmlLocString = function (name, locName, func, reason) {\n var _this = this;\n var res = this.createLocalizableString(name, this, false, locName);\n res.onGetLocalizationTextCallback = func;\n if (reason) {\n res.onGetTextCallback = function (str) { return _this.processHtml(str, reason); };\n }\n };\n SurveyModel.prototype.getType = function () {\n return \"survey\";\n };\n SurveyModel.prototype.onPropertyValueChanged = function (name, oldValue, newValue) {\n if (name === \"questionsOnPageMode\") {\n this.onQuestionsOnPageModeChanged(oldValue);\n }\n };\n Object.defineProperty(SurveyModel.prototype, \"pages\", {\n /**\n * Returns an array of all pages in the survey.\n *\n * To get an array of only visible pages, use the [`visiblePages`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#visiblePages) array.\n * @see PageModel\n */\n get: function () {\n return this.getPropertyValue(\"pages\");\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.render = function (element) {\n if (element === void 0) { element = null; }\n if (this.renderCallback) {\n this.renderCallback();\n }\n };\n SurveyModel.prototype.updateSurvey = function (newProps, oldProps) {\n var _loop_1 = function () {\n if (key == \"model\" || key == \"children\")\n return \"continue\";\n if (key.indexOf(\"on\") == 0 && this_1[key] && this_1[key].add) {\n var funcBody_1 = newProps[key];\n var func = function (sender, options) {\n funcBody_1(sender, options);\n };\n this_1[key].add(func);\n }\n else {\n this_1[key] = newProps[key];\n }\n };\n var this_1 = this;\n for (var key in newProps) {\n _loop_1();\n }\n if (newProps && newProps.data)\n this.onValueChanged.add(function (sender, options) {\n newProps.data[options.name] = options.value;\n });\n };\n SurveyModel.prototype.getCss = function () {\n return this.css;\n };\n SurveyModel.prototype.updateCompletedPageCss = function () {\n this.containerCss = this.css.container;\n this.completedCss = new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_18__[\"CssClassBuilder\"]().append(this.css.body)\n .append(this.css.completedPage).toString(); // for completed page\n this.completedBeforeCss = new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_18__[\"CssClassBuilder\"]()\n .append(this.css.body)\n .append(this.css.completedBeforePage)\n .toString();\n this.loadingBodyCss = new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_18__[\"CssClassBuilder\"]()\n .append(this.css.body)\n .append(this.css.bodyLoading)\n .toString();\n };\n SurveyModel.prototype.updateCss = function () {\n this.rootCss = this.getRootCss();\n this.updateNavigationCss();\n this.updateCompletedPageCss();\n this.updateWrapperFormCss();\n };\n Object.defineProperty(SurveyModel.prototype, \"css\", {\n /**\n * Gets or sets an object in which keys are UI elements and values are CSS classes applied to them.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/customize-survey-with-css/ (linkStyle))\n */\n get: function () {\n if (!this.cssValue) {\n this.cssValue = {};\n this.copyCssClasses(this.cssValue, _defaultCss_defaultV2Css__WEBPACK_IMPORTED_MODULE_4__[\"surveyCss\"].getCss());\n }\n return this.cssValue;\n },\n set: function (value) {\n this.setCss(value);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.setCss = function (value, needMerge) {\n if (needMerge === void 0) { needMerge = true; }\n if (needMerge) {\n this.mergeValues(value, this.css);\n }\n else {\n this.cssValue = value;\n }\n this.updateCss();\n this.updateElementCss(false);\n };\n Object.defineProperty(SurveyModel.prototype, \"cssTitle\", {\n get: function () {\n return this.css.title;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"cssNavigationComplete\", {\n get: function () {\n return this.getNavigationCss(this.cssSurveyNavigationButton, this.css.navigation.complete);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"cssNavigationPreview\", {\n get: function () {\n return this.getNavigationCss(this.cssSurveyNavigationButton, this.css.navigation.preview);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"cssNavigationEdit\", {\n get: function () {\n return this.getNavigationCss(this.css.navigationButton, this.css.navigation.edit);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"cssNavigationPrev\", {\n get: function () {\n return this.getNavigationCss(this.cssSurveyNavigationButton, this.css.navigation.prev);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"cssNavigationStart\", {\n get: function () {\n return this.getNavigationCss(this.cssSurveyNavigationButton, this.css.navigation.start);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"cssNavigationNext\", {\n get: function () {\n return this.getNavigationCss(this.cssSurveyNavigationButton, this.css.navigation.next);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"cssSurveyNavigationButton\", {\n get: function () {\n return new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_18__[\"CssClassBuilder\"]().append(this.css.navigationButton).append(this.css.bodyNavigationButton).toString();\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"bodyCss\", {\n get: function () {\n return new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_18__[\"CssClassBuilder\"]().append(this.css.body)\n .append(this.css.bodyWithTimer, this.showTimerPanel != \"none\" && this.state === \"running\")\n .append(this.css.body + \"--\" + this.calculatedWidthMode).toString();\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"bodyContainerCss\", {\n get: function () {\n return this.css.bodyContainer;\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.insertAdvancedHeader = function (advHeader) {\n advHeader.survey = this;\n this.layoutElements.push({\n id: \"advanced-header\",\n container: \"header\",\n component: \"sv-header\",\n index: -100,\n data: advHeader,\n processResponsiveness: function (width) { return advHeader.processResponsiveness(width); }\n });\n };\n SurveyModel.prototype.getNavigationCss = function (main, btn) {\n return new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_18__[\"CssClassBuilder\"]().append(main)\n .append(btn).toString();\n };\n Object.defineProperty(SurveyModel.prototype, \"lazyRendering\", {\n /**\n * Specifies whether to enable lazy rendering.\n *\n * In default mode, a survey renders the entire current page. With lazy rendering, the survey renders the page gradually as a user scrolls it. This helps reduce survey startup time and optimizes large surveys for low-end devices.\n *\n * Default value: `false`\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-lazy/ (linkStyle))\n * @see [settings.lazyRender](https://surveyjs.io/form-library/documentation/api-reference/settings#lazyRender)\n */\n get: function () {\n return this.lazyRenderingValue === true;\n },\n set: function (val) {\n if (this.lazyRendering === val)\n return;\n this.lazyRenderingValue = val;\n var page = this.currentPage;\n if (!!page) {\n page.updateRows();\n }\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isLazyRendering\", {\n get: function () {\n return this.lazyRendering || _settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].lazyRender.enabled;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"lazyRenderingFirstBatchSize\", {\n get: function () {\n return this.lazyRenderingFirstBatchSizeValue || _settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].lazyRender.firstBatchSize;\n },\n set: function (val) {\n this.lazyRenderingFirstBatchSizeValue = val;\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.updateLazyRenderingRowsOnRemovingElements = function () {\n if (!this.isLazyRendering)\n return;\n var page = this.currentPage;\n if (!!page) {\n Object(_utils_utils__WEBPACK_IMPORTED_MODULE_15__[\"scrollElementByChildId\"])(page.id);\n }\n };\n Object.defineProperty(SurveyModel.prototype, \"triggers\", {\n /**\n * A list of triggers in the survey.\n *\n * [Conditional Survey Logic (Triggers)](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#conditional-survey-logic-triggers (linkStyle))\n * @see runTriggers\n * @see onTriggerExecuted\n */\n get: function () {\n return this.getPropertyValue(\"triggers\");\n },\n set: function (val) {\n this.setPropertyValue(\"triggers\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"calculatedValues\", {\n /**\n * An array of [calculated values](https://surveyjs.io/form-library/documentation/design-survey-conditional-logic#calculated-values).\n */\n get: function () {\n return this.getPropertyValue(\"calculatedValues\");\n },\n set: function (val) {\n this.setPropertyValue(\"calculatedValues\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"surveyId\", {\n /**\n * The identifier of a survey JSON schema to load from [SurveyJS Service](https://api.surveyjs.io).\n *\n * Refer to the following help topic for more information: [Store Survey Results in the SurveyJS Service](https://surveyjs.io/form-library/documentation/handle-survey-results-store#store-survey-results-in-the-surveyjs-service).\n * @see loadSurveyFromService\n * @see onLoadedSurveyFromService\n */\n get: function () {\n return this.getPropertyValue(\"surveyId\", \"\");\n },\n set: function (val) {\n this.setPropertyValue(\"surveyId\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"surveyPostId\", {\n /**\n * An identifier used to save survey results to [SurveyJS Service](https://api.surveyjs.io).\n *\n * Refer to the following help topic for more information: [Store Survey Results in the SurveyJS Service](https://surveyjs.io/form-library/documentation/handle-survey-results-store#store-survey-results-in-the-surveyjs-service).\n * @see onComplete\n * @see surveyShowDataSaving\n */\n get: function () {\n return this.getPropertyValue(\"surveyPostId\", \"\");\n },\n set: function (val) {\n this.setPropertyValue(\"surveyPostId\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"clientId\", {\n /**\n * A user identifier (e-mail or other unique ID).\n *\n * If your application works with [SurveyJS Service](https://api.surveyjs.io), the ID ensures that users do not pass the same survey twice. On the second run, they will see the [Completed Before page](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#completedBeforeHtml).\n * @see cookieName\n */\n get: function () {\n return this.getPropertyValue(\"clientId\", \"\");\n },\n set: function (val) {\n this.setPropertyValue(\"clientId\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"cookieName\", {\n /**\n * A cookie name used to save information about survey completion.\n *\n * When this property has a value, the survey creates a cookie with the specified name on completion. This cookie helps ensure that users do not pass the same survey twice. On the second run, they will see the [Completed Before page](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#completedBeforeHtml).\n * @see clientId\n */\n get: function () {\n return this.getPropertyValue(\"cookieName\", \"\");\n },\n set: function (val) {\n this.setPropertyValue(\"cookieName\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"sendResultOnPageNext\", {\n /**\n * Specifies whether to save survey results when respondents switch between pages. Handle the [`onPartialSend`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#onPartialSend) event to implement the save operation.\n *\n * Alternatively, you can handle the [`onCurrentPageChanged`](#onCurrentPageChanged) and [`onValueChanged`](#onValueChanged) events, as shown in the following demo: [Continue an Incomplete Survey](https://surveyjs.io/form-library/examples/survey-editprevious/).\n */\n get: function () {\n return this.getPropertyValue(\"sendResultOnPageNext\");\n },\n set: function (val) {\n this.setPropertyValue(\"sendResultOnPageNext\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"surveyShowDataSaving\", {\n /**\n * Specifies whether to show progress when the survey sends data to [SurveyJS Service](https://api.surveyjs.io).\n *\n * [View Demo](https://surveyjs.io/form-library/examples/save-survey-results-and-load-surveys-from-surveyjs-service/ (linkStyle))\n * @see surveyPostId\n */\n get: function () {\n return this.getPropertyValue(\"surveyShowDataSaving\");\n },\n set: function (val) {\n this.setPropertyValue(\"surveyShowDataSaving\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"focusFirstQuestionAutomatic\", {\n /**\n * Specifies whether to focus the first question on the page on survey startup or when users switch between pages.\n *\n * Default value: `false` in v1.9.114 and later, `true` in earlier versions\n * @see focusOnFirstError\n * @see focusFirstQuestion\n * @see focusQuestion\n */\n get: function () {\n return this.getPropertyValue(\"focusFirstQuestionAutomatic\");\n },\n set: function (val) {\n this.setPropertyValue(\"focusFirstQuestionAutomatic\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"focusOnFirstError\", {\n /**\n * Specifies whether to focus the first question with a validation error on the current page.\n *\n * Default value: `true`\n * @see validate\n * @see focusFirstQuestionAutomatic\n */\n get: function () {\n return this.getPropertyValue(\"focusOnFirstError\");\n },\n set: function (val) {\n this.setPropertyValue(\"focusOnFirstError\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"showNavigationButtons\", {\n /**\n * Gets or sets the position of the Start, Next, Previous, and Complete navigation buttons and controls their visibility.\n *\n * Possible values:\n *\n * - `\"bottom\"` (default) - Displays the navigation buttons below survey content.\n * - `\"top\"` - Displays the navigation buttons above survey content.\n * - `\"both\"` - Displays the navigation buttons above and below survey content.\n * - `\"none\"` - Hides the navigation buttons. This setting may be useful if you [implement custom external navigation](https://surveyjs.io/form-library/examples/external-form-navigation-system/).\n * @see goNextPageAutomatic\n * @see showPrevButton\n * @see showCompleteButton\n */\n get: function () {\n return this.getPropertyValue(\"showNavigationButtons\");\n },\n set: function (val) {\n if (val === true || val === undefined) {\n val = \"bottom\";\n }\n if (val === false) {\n val = \"none\";\n }\n this.setPropertyValue(\"showNavigationButtons\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"showPrevButton\", {\n /**\n * Specifies whether to display the Previous button. Set this property to `false` if respondents should not move backward along the survey.\n * @see showNavigationButtons\n * @see showCompleteButton\n */\n get: function () {\n return this.getPropertyValue(\"showPrevButton\");\n },\n set: function (val) {\n this.setPropertyValue(\"showPrevButton\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"showCompleteButton\", {\n /**\n * Specifies whether to display the Complete button. Set this property to `false` if respondents should not complete the survey.\n * @see showNavigationButtons\n * @see showPrevButton\n */\n get: function () {\n return this.getPropertyValue(\"showCompleteButton\", true);\n },\n set: function (val) {\n this.setPropertyValue(\"showCompleteButton\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"showTOC\", {\n /**\n * Gets or sets the visibility of the table of contents.\n *\n * Default value: `false`\n *\n * [View Demo](https://surveyjs.io/form-library/examples/toc-feature/ (linkStyle))\n * @see tocLocation\n */\n get: function () {\n return this.getPropertyValue(\"showTOC\");\n },\n set: function (val) {\n this.setPropertyValue(\"showTOC\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"tocLocation\", {\n /**\n * Gets or sets the position of the table of contents. Applies only when the table of contents is visible.\n *\n * Possible values:\n *\n * - `\"left\"` (default)\n * - `\"right\"`\n *\n * [View Demo](https://surveyjs.io/form-library/examples/toc-feature/ (linkStyle))\n * @see showTOC\n */\n get: function () {\n return this.getPropertyValue(\"tocLocation\");\n },\n set: function (val) {\n this.setPropertyValue(\"tocLocation\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"showTitle\", {\n /**\n * Specifies whether to display the [survey title](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#title).\n *\n * [View Demo](https://surveyjs.io/form-library/examples/brand-your-survey-header/ (linkStyle))\n * @see title\n */\n get: function () {\n return this.getPropertyValue(\"showTitle\");\n },\n set: function (val) {\n this.setPropertyValue(\"showTitle\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"showPageTitles\", {\n /**\n * Specifies whether to display [page titles](https://surveyjs.io/form-library/documentation/api-reference/page-model#title).\n */\n get: function () {\n return this.getPropertyValue(\"showPageTitles\");\n },\n set: function (val) {\n this.setPropertyValue(\"showPageTitles\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"showCompletedPage\", {\n /**\n * Specifies whether to show the [complete page](https://surveyjs.io/form-library/documentation/design-survey/create-a-multi-page-survey#complete-page).\n * @see onComplete\n * @see navigateToUrl\n */\n get: function () {\n return this.getPropertyValue(\"showCompletedPage\");\n },\n set: function (val) {\n this.setPropertyValue(\"showCompletedPage\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"navigateToUrl\", {\n /**\n * A URL to which respondents should be navigated after survey completion.\n * @see onNavigateToUrl\n * @see navigateToUrlOnCondition\n */\n get: function () {\n return this.getPropertyValue(\"navigateToUrl\");\n },\n set: function (val) {\n this.setPropertyValue(\"navigateToUrl\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"navigateToUrlOnCondition\", {\n /**\n * An array of objects that allows you to navigate respondents to different URLs after survey completion.\n *\n * Each object should include the [`expression`](https://surveyjs.io/form-library/documentation/api-reference/urlconditionitem#url) and [`url`](https://surveyjs.io/form-library/documentation/api-reference/urlconditionitem#expression) properties. When `expression` evaluates to `true`, the survey navigates to the corresponding `url`. Refer to the following help topic for more information about expressions: [Expressions](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#expressions).\n * @see onNavigateToUrl\n * @see navigateToUrl\n */\n get: function () {\n return this.getPropertyValue(\"navigateToUrlOnCondition\");\n },\n set: function (val) {\n this.setPropertyValue(\"navigateToUrlOnCondition\", val);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.getNavigateToUrl = function () {\n var item = this.getExpressionItemOnRunCondition(this.navigateToUrlOnCondition);\n var url = !!item ? item.url : this.navigateToUrl;\n if (!!url) {\n url = this.processText(url, false);\n }\n return url;\n };\n SurveyModel.prototype.navigateTo = function () {\n var url = this.getNavigateToUrl();\n var options = { url: url, allow: true };\n this.onNavigateToUrl.fire(this, options);\n if (!options.url || !options.allow)\n return;\n Object(_utils_utils__WEBPACK_IMPORTED_MODULE_15__[\"navigateToUrl\"])(options.url);\n };\n Object.defineProperty(SurveyModel.prototype, \"requiredText\", {\n /**\n * Specifies one or multiple characters that designate required questions.\n *\n * Default value: `*`\n *\n * [View Demo](https://surveyjs.io/form-library/examples/modify-question-title/ (linkStyle))\n */\n get: function () {\n return this.getPropertyValue(\"requiredText\", \"*\");\n },\n set: function (val) {\n this.setPropertyValue(\"requiredText\", val);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.beforeSettingQuestionErrors = function (question, errors) {\n this.makeRequiredErrorsInvisible(errors);\n this.onSettingQuestionErrors.fire(this, {\n question: question,\n errors: errors,\n });\n };\n SurveyModel.prototype.beforeSettingPanelErrors = function (question, errors) {\n this.makeRequiredErrorsInvisible(errors);\n };\n SurveyModel.prototype.makeRequiredErrorsInvisible = function (errors) {\n if (!this.hideRequiredErrors)\n return;\n for (var i = 0; i < errors.length; i++) {\n var erType = errors[i].getErrorType();\n if (erType == \"required\" || erType == \"requireoneanswer\") {\n errors[i].visible = false;\n }\n }\n };\n Object.defineProperty(SurveyModel.prototype, \"questionStartIndex\", {\n /**\n * Specifies the initial number or letter from which to start question numbering.\n *\n * [Question Numbers](https://surveyjs.io/form-library/documentation/design-survey/configure-question-titles#question-numbers (linkStyle))\n */\n get: function () {\n return this.getPropertyValue(\"questionStartIndex\", \"\");\n },\n set: function (val) {\n this.setPropertyValue(\"questionStartIndex\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"storeOthersAsComment\", {\n /**\n * Specifies whether to store the \"Other\" option response in a separate property.\n *\n * Default value: `true`\n *\n * Respondents can leave comments when they select \"Other\" in choice-based questions, such as Dropdown or Checkboxes. Comment values are saved in a separate property. The property name is composed of the question `name` and [`commentSuffix`](#commentSuffix). However, you can use the question `name` as a key to store the comment value instead. Disable the `storeOthersAsComment` property in this case.\n * @see maxOthersLength\n */\n get: function () {\n return this.getPropertyValue(\"storeOthersAsComment\");\n },\n set: function (val) {\n this.setPropertyValue(\"storeOthersAsComment\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"maxTextLength\", {\n /**\n * Specifies the maximum text length in textual questions ([Single-Line Input](https://surveyjs.io/form-library/examples/text-entry-question/), [Long Text](https://surveyjs.io/form-library/examples/add-open-ended-question-to-a-form/), [Multiple Textboxes](https://surveyjs.io/form-library/examples/multiple-text-box-question/)), measured in characters.\n *\n * Default value: 0 (unlimited)\n *\n * You can override this setting for individual questions if you specify their [`maxLength`](https://surveyjs.io/form-library/documentation/api-reference/text-entry-question-model#maxLength) property.\n * @see maxOthersLength\n */\n get: function () {\n return this.getPropertyValue(\"maxTextLength\");\n },\n set: function (val) {\n this.setPropertyValue(\"maxTextLength\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"maxOthersLength\", {\n /**\n * Specifies the maximum text length for question comments. Applies to questions with the [`showCommentArea`](https://surveyjs.io/form-library/documentation/api-reference/question#showCommentArea) or [`showOtherItem`](https://surveyjs.io/form-library/documentation/api-reference/question#showOtherItem) property set to `true`.\n *\n * Default value: 0 (unlimited)\n * @see maxTextLength\n */\n get: function () {\n return this.getPropertyValue(\"maxOthersLength\");\n },\n set: function (val) {\n this.setPropertyValue(\"maxOthersLength\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"goNextPageAutomatic\", {\n /**\n * Specifies whether the survey switches to the next page automatically after a user answers all questions on the current page.\n *\n * Default value: `false`\n *\n * If you enable this property, the survey is also completed automatically. Set the [`allowCompleteSurveyAutomatic`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#allowCompleteSurveyAutomatic) property to `false` if you want to disable this behavior.\n *\n * > If any of the following questions is answered last, the survey does not switch to the next page: Checkboxes, Yes/No (Boolean) (rendered as Checkbox), Long Text, Signature, Image Picker (with Multi Select), File Upload, Single-Select Matrix (not all rows are answered), Dynamic Matrix, Dynamic Panel.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/automatically-move-to-next-page-if-answer-selected/ (linkStyle))\n * @see [`settings.autoAdvanceDelay`](https://surveyjs.io/form-library/documentation/api-reference/settings#autoAdvanceDelay)\n */\n get: function () {\n return this.getPropertyValue(\"goNextPageAutomatic\");\n },\n set: function (val) {\n this.setPropertyValue(\"goNextPageAutomatic\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"allowCompleteSurveyAutomatic\", {\n /**\n * Specifies whether to complete the survey automatically after a user answers all questions on the last page. Applies only if the [`goNextPageAutomatic`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#goNextPageAutomatic) property is `true`.\n *\n * Default value: `true`\n * @see [`settings.autoAdvanceDelay`](https://surveyjs.io/form-library/documentation/api-reference/settings#autoAdvanceDelay)\n */\n get: function () {\n return this.getPropertyValue(\"allowCompleteSurveyAutomatic\");\n },\n set: function (val) {\n this.setPropertyValue(\"allowCompleteSurveyAutomatic\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"checkErrorsMode\", {\n /**\n * Specifies when the survey validates answers.\n *\n * Possible values:\n *\n * - `\"onNextPage\"` (default) - Triggers validation before the survey is switched to the next page or completed.\n * - `\"onValueChanged\"` - Triggers validation each time a question value is changed.\n * - `\"onComplete\"` - Triggers validation when a user clicks the Complete button. If previous pages contain errors, the survey switches to the page with the first error.\n *\n * Refer to the following help topic for more information: [Data Validation](https://surveyjs.io/form-library/documentation/data-validation).\n * @see validationEnabled\n * @see validationAllowSwitchPages\n * @see validationAllowComplete\n * @see validate\n */\n get: function () {\n return this.getPropertyValue(\"checkErrorsMode\");\n },\n set: function (val) {\n this.setPropertyValue(\"checkErrorsMode\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"autoGrowComment\", {\n /**\n * Specifies whether to increase the height of [Long Text](https://surveyjs.io/form-library/examples/add-open-ended-question-to-a-form/) questions and other text areas to accommodate multi-line text content.\n *\n * Default value: `false`\n *\n * You can override this property for individual Long Text questions: [`autoGrow`](https://surveyjs.io/form-library/documentation/api-reference/comment-field-model#autoGrow).\n * @see allowResizeComment\n */\n get: function () {\n return this.getPropertyValue(\"autoGrowComment\");\n },\n set: function (val) {\n this.setPropertyValue(\"autoGrowComment\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"allowResizeComment\", {\n /**\n * Specifies whether to display a resize handle for [Long Text](https://surveyjs.io/form-library/examples/add-open-ended-question-to-a-form/) questions and other text areas intended for multi-line text content.\n *\n * Default value: `true`\n *\n * You can override this property for individual Long Text questions: [`allowResize`](https://surveyjs.io/form-library/documentation/api-reference/comment-field-model#allowResize).\n * @see autoGrowComment\n */\n get: function () {\n return this.getPropertyValue(\"allowResizeComment\");\n },\n set: function (val) {\n this.setPropertyValue(\"allowResizeComment\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"textUpdateMode\", {\n /**\n * Specifies when to update the question value in questions with a text input field.\n *\n * Possible values:\n *\n * - `\"onBlur\"` (default) - Updates the value after the input field loses focus.\n * - `\"onTyping\"` - Updates the value on every key press.\n *\n * > Do not use the `\"onTyping\"` mode if your survey contains many expressions. Expressions are reevaluated each time a question value is changed. In `\"onTyping\"` mode, the question value changes frequently. This may cause performance degradation.\n *\n * You can override this setting for individual questions: [`textUpdateMode`](https://surveyjs.io/form-library/documentation/api-reference/text-entry-question-model#textUpdateMode).\n */\n get: function () {\n return this.getPropertyValue(\"textUpdateMode\");\n },\n set: function (val) {\n this.setPropertyValue(\"textUpdateMode\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"clearInvisibleValues\", {\n /**\n * Specifies when to remove values of invisible questions from [survey results](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#data).\n *\n * Possible values:\n *\n * - `\"onComplete\"` (default) - Clears invisible question values when the survey is complete.\n * - `\"onHidden\"` - Clears a question value when the question becomes invisible. If the question is invisible initially, its value is removed on survey completion.\n * - `\"onHiddenContainer\"` - Clears a question value when the question or its containter (page or panel) becomes invisible. If the question is invisible initially, its value is removed on survey completion.\n * - `\"none\"` - Keeps invisible values in survey results.\n * - `true` - Equivalent to `\"onComplete\"`.\n * - `false` - Equivalent to `\"none\"`.\n * @see [Conditional Visibility](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#conditional-visibility)\n * @see onComplete\n */\n get: function () {\n return this.getPropertyValue(\"clearInvisibleValues\");\n },\n set: function (val) {\n if (val === true)\n val = \"onComplete\";\n if (val === false)\n val = \"none\";\n this.setPropertyValue(\"clearInvisibleValues\", val);\n },\n enumerable: false,\n configurable: true\n });\n /**\n * Removes values that cannot be assigned to a question, for example, choices unlisted in the `choices` array.\n *\n * Call this method after you assign new question values in code to ensure that they are acceptable.\n *\n * > This method does not remove values that fail validation. Call the [`validate()`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#validate) method to validate newly assigned values.\n * @param removeNonExistingRootKeys Pass `true` to remove values that do not correspond to any question or [calculated value](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#calculated-values).\n */\n SurveyModel.prototype.clearIncorrectValues = function (removeNonExistingRootKeys) {\n if (removeNonExistingRootKeys === void 0) { removeNonExistingRootKeys = false; }\n for (var i = 0; i < this.pages.length; i++) {\n this.pages[i].clearIncorrectValues();\n }\n if (!removeNonExistingRootKeys)\n return;\n var data = this.data;\n var hasChanges = false;\n for (var key in data) {\n if (!!this.getQuestionByValueName(key))\n continue;\n if (this.iscorrectValueWithPostPrefix(key, _settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].commentSuffix) ||\n this.iscorrectValueWithPostPrefix(key, _settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].matrix.totalsSuffix))\n continue;\n var calcValue = this.getCalculatedValueByName(key);\n if (!!calcValue && calcValue.includeIntoResult)\n continue;\n hasChanges = true;\n delete data[key];\n }\n if (hasChanges) {\n this.data = data;\n }\n };\n SurveyModel.prototype.iscorrectValueWithPostPrefix = function (key, postPrefix) {\n if (key.indexOf(postPrefix) !== key.length - postPrefix.length)\n return false;\n return !!this.getQuestionByValueName(key.substring(0, key.indexOf(postPrefix)));\n };\n Object.defineProperty(SurveyModel.prototype, \"keepIncorrectValues\", {\n /**\n * Specifies whether to keep values that cannot be assigned to questions, for example, choices unlisted in the `choices` array.\n *\n * > This property cannot be specified in the survey JSON schema. Use dot notation to specify it.\n * @see clearIncorrectValues\n */\n get: function () {\n return this.getPropertyValue(\"keepIncorrectValues\");\n },\n set: function (val) {\n this.setPropertyValue(\"keepIncorrectValues\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"locale\", {\n /**\n * Specifies the survey's locale.\n *\n * Default value: `\"\"` (a default locale is used)\n *\n * [Localization & Globalization help topic](https://surveyjs.io/form-library/documentation/survey-localization (linkStyle))\n *\n * [Survey Localization demo](https://surveyjs.io/form-library/examples/survey-localization/ (linkStyle))\n */\n get: function () {\n return this.getPropertyValueWithoutDefault(\"locale\") || _surveyStrings__WEBPACK_IMPORTED_MODULE_8__[\"surveyLocalization\"].currentLocale;\n },\n set: function (value) {\n if (value === _surveyStrings__WEBPACK_IMPORTED_MODULE_8__[\"surveyLocalization\"].defaultLocale && !_surveyStrings__WEBPACK_IMPORTED_MODULE_8__[\"surveyLocalization\"].currentLocale) {\n value = \"\";\n }\n this.setPropertyValue(\"locale\", value);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.onSurveyLocaleChanged = function () {\n this.notifyElementsOnAnyValueOrVariableChanged(\"locale\");\n this.localeChanged();\n this.onLocaleChangedEvent.fire(this, this.locale);\n };\n /**\n * Returns an array of locales whose translations are used in the survey.\n *\n * [Localization & Globalization help topic](https://surveyjs.io/form-library/documentation/survey-localization (linkStyle))\n *\n * [Survey Localization demo](https://surveyjs.io/form-library/examples/survey-localization/ (linkStyle))\n */\n SurveyModel.prototype.getUsedLocales = function () {\n var locs = new Array();\n this.addUsedLocales(locs);\n //Replace the default locale with the real one\n var index = locs.indexOf(\"default\");\n if (index > -1) {\n var defaultLoc = _surveyStrings__WEBPACK_IMPORTED_MODULE_8__[\"surveyLocalization\"].defaultLocale;\n //Remove the defaultLoc\n var defIndex = locs.indexOf(defaultLoc);\n if (defIndex > -1) {\n locs.splice(defIndex, 1);\n }\n index = locs.indexOf(\"default\");\n locs[index] = defaultLoc;\n }\n return locs;\n };\n SurveyModel.prototype.localeChanged = function () {\n for (var i = 0; i < this.pages.length; i++) {\n this.pages[i].localeChanged();\n }\n };\n //ILocalizableOwner\n SurveyModel.prototype.getLocale = function () {\n return this.locale;\n };\n SurveyModel.prototype.locStrsChanged = function () {\n if (this.isClearingUnsedValues)\n return;\n _super.prototype.locStrsChanged.call(this);\n if (!this.currentPage)\n return;\n if (this.isDesignMode) {\n this.pages.forEach(function (page) { return page.locStrsChanged(); });\n }\n else {\n var page = this.activePage;\n if (!!page) {\n page.locStrsChanged();\n }\n var visPages = this.visiblePages;\n for (var i = 0; i < visPages.length; i++) {\n visPages[i].navigationLocStrChanged();\n }\n }\n if (!this.isShowStartingPage) {\n this.updateProgressText();\n }\n this.navigationBar.locStrsChanged();\n };\n SurveyModel.prototype.getMarkdownHtml = function (text, name) {\n return this.getSurveyMarkdownHtml(this, text, name);\n };\n SurveyModel.prototype.getRenderer = function (name) {\n return this.getRendererForString(this, name);\n };\n SurveyModel.prototype.getRendererContext = function (locStr) {\n return this.getRendererContextForString(this, locStr);\n };\n SurveyModel.prototype.getRendererForString = function (element, name) {\n var renderAs = this.getBuiltInRendererForString(element, name);\n var options = { element: element, name: name, renderAs: renderAs };\n this.onTextRenderAs.fire(this, options);\n return options.renderAs;\n };\n SurveyModel.prototype.getRendererContextForString = function (element, locStr) {\n return locStr;\n };\n SurveyModel.prototype.getExpressionDisplayValue = function (question, value, displayValue) {\n var options = {\n question: question,\n value: value,\n displayValue: displayValue,\n };\n this.onGetExpressionDisplayValue.fire(this, options);\n return options.displayValue;\n };\n SurveyModel.prototype.getBuiltInRendererForString = function (element, name) {\n if (this.isDesignMode)\n return _localizablestring__WEBPACK_IMPORTED_MODULE_10__[\"LocalizableString\"].editableRenderer;\n return undefined;\n };\n SurveyModel.prototype.getProcessedText = function (text) {\n return this.processText(text, true);\n };\n SurveyModel.prototype.getLocString = function (str) {\n return this.getLocalizationString(str);\n };\n //ISurveyErrorOwner\n SurveyModel.prototype.getErrorCustomText = function (text, error) {\n return this.getSurveyErrorCustomText(this, text, error);\n };\n SurveyModel.prototype.getSurveyErrorCustomText = function (obj, text, error) {\n var options = {\n text: text,\n name: error.getErrorType(),\n obj: obj,\n error: error\n };\n this.onErrorCustomText.fire(this, options);\n return options.text;\n };\n SurveyModel.prototype.getQuestionDisplayValue = function (question, displayValue) {\n var options = { question: question, displayValue: displayValue };\n this.onGetQuestionDisplayValue.fire(this, options);\n return options.displayValue;\n };\n Object.defineProperty(SurveyModel.prototype, \"emptySurveyText\", {\n /**\n * A message that is displayed when a survey does not contain visible pages or questions.\n * @see [Localization & Globalization](https://surveyjs.io/form-library/documentation/survey-localization)\n */\n get: function () {\n return this.getLocalizableStringText(\"emptySurveyText\");\n },\n set: function (val) {\n this.setLocalizableStringText(\"emptySurveyText\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"logo\", {\n //#region Title/Header options\n /**\n * An image URL or a Base64-encoded image to use as a survey logo.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-logo/ (linkStyle))\n * @see logoPosition\n * @see logoFit\n */\n get: function () {\n return this.getLocalizableStringText(\"logo\");\n },\n set: function (value) {\n this.setLocalizableStringText(\"logo\", value);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"locLogo\", {\n get: function () {\n return this.getLocalizableString(\"logo\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"logoWidth\", {\n /**\n * A logo width in CSS-accepted values.\n *\n * Default value: `300px`\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-logo/ (linkStyle))\n * @see logoHeight\n * @see logo\n * @see logoPosition\n * @see logoFit\n */\n get: function () {\n return this.getPropertyValue(\"logoWidth\");\n },\n set: function (value) {\n this.setPropertyValue(\"logoWidth\", value);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"renderedLogoWidth\", {\n get: function () {\n return this.logoWidth ? Object(_utils_utils__WEBPACK_IMPORTED_MODULE_15__[\"getRenderedSize\"])(this.logoWidth) : undefined;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"renderedStyleLogoWidth\", {\n get: function () {\n return this.logoWidth ? Object(_utils_utils__WEBPACK_IMPORTED_MODULE_15__[\"getRenderedStyleSize\"])(this.logoWidth) : undefined;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"logoHeight\", {\n /**\n * A logo height in CSS-accepted values.\n *\n * Default value: `200px`\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-logo/ (linkStyle))\n * @see logoHeight\n * @see logo\n * @see logoPosition\n * @see logoFit\n */\n get: function () {\n return this.getPropertyValue(\"logoHeight\");\n },\n set: function (value) {\n this.setPropertyValue(\"logoHeight\", value);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"renderedLogoHeight\", {\n get: function () {\n return this.logoHeight ? Object(_utils_utils__WEBPACK_IMPORTED_MODULE_15__[\"getRenderedSize\"])(this.logoHeight) : undefined;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"renderedStyleLogoHeight\", {\n get: function () {\n return this.logoHeight ? Object(_utils_utils__WEBPACK_IMPORTED_MODULE_15__[\"getRenderedStyleSize\"])(this.logoHeight) : undefined;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"logoPosition\", {\n /**\n * A logo position relative to the [survey title](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#title).\n *\n * Possible values:\n *\n * - `\"left\"` (default) - Places the logo to the left of the survey title.\n * - `\"right\"` - Places the logo to the right of the survey title.\n * - `\"none\"` - Hides the logo.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-logo/ (linkStyle))\n * @see logo\n * @see logoFit\n */\n get: function () {\n return this.getPropertyValue(\"logoPosition\");\n },\n set: function (value) {\n this.setPropertyValue(\"logoPosition\", value);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"hasLogo\", {\n get: function () {\n return this.getPropertyValue(\"hasLogo\", false);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.updateHasLogo = function () {\n this.setPropertyValue(\"hasLogo\", !!this.logo && this.logoPosition !== \"none\");\n };\n Object.defineProperty(SurveyModel.prototype, \"isLogoBefore\", {\n get: function () {\n if (this.isDesignMode)\n return false;\n return (this.renderedHasLogo &&\n (this.logoPosition === \"left\" || this.logoPosition === \"top\"));\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isLogoAfter\", {\n get: function () {\n if (this.isDesignMode)\n return this.renderedHasLogo;\n return (this.renderedHasLogo &&\n (this.logoPosition === \"right\" || this.logoPosition === \"bottom\"));\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"logoClassNames\", {\n get: function () {\n var logoClasses = {\n left: \"sv-logo--left\",\n right: \"sv-logo--right\",\n top: \"sv-logo--top\",\n bottom: \"sv-logo--bottom\",\n };\n return new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_18__[\"CssClassBuilder\"]().append(this.css.logo)\n .append(logoClasses[this.logoPosition]).toString();\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"renderedHasTitle\", {\n get: function () {\n if (this.isDesignMode)\n return this.isPropertyVisible(\"title\");\n return !this.titleIsEmpty && this.showTitle;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"renderedHasDescription\", {\n get: function () {\n if (this.isDesignMode)\n return this.isPropertyVisible(\"description\");\n return !!this.hasDescription;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"hasTitle\", {\n get: function () {\n return this.renderedHasTitle;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"renderedHasLogo\", {\n get: function () {\n if (this.isDesignMode)\n return this.isPropertyVisible(\"logo\");\n return this.hasLogo;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"renderedHasHeader\", {\n get: function () {\n return this.renderedHasTitle || this.renderedHasLogo;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"logoFit\", {\n /**\n * Specifies how to resize a logo to fit it into its container.\n *\n * Possible values:\n *\n * - `\"contain\"` (default)\n * - `\"cover\"`\n * - `\"fill\"`\n * - `\"none\"`\n *\n * Refer to the [`object-fit`](https://developer.mozilla.org/en-US/docs/Web/CSS/object-fit) CSS property description for information on the possible values.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-logo/ (linkStyle))\n * @see logo\n * @see logoPosition\n */\n get: function () {\n return this.getPropertyValue(\"logoFit\");\n },\n set: function (val) {\n this.setPropertyValue(\"logoFit\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"themeVariables\", {\n get: function () {\n return Object.assign({}, this.cssVariables);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.setIsMobile = function (newVal) {\n if (newVal === void 0) { newVal = true; }\n if (this._isMobile !== newVal) {\n this._isMobile = newVal;\n this.updateCss();\n this.getAllQuestions().forEach(function (q) { return q.setIsMobile(newVal); });\n }\n };\n Object.defineProperty(SurveyModel.prototype, \"isMobile\", {\n get: function () {\n return this._isMobile && !this.isDesignMode;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isCompact\", {\n get: function () {\n return this._isCompact;\n },\n set: function (newVal) {\n if (newVal !== this._isCompact) {\n this._isCompact = newVal;\n this.updateElementCss();\n this.triggerResponsiveness(true);\n }\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.isLogoImageChoosen = function () {\n return this.locLogo.renderedHtml;\n };\n Object.defineProperty(SurveyModel.prototype, \"titleMaxWidth\", {\n get: function () {\n if (!(Object(_utils_utils__WEBPACK_IMPORTED_MODULE_15__[\"isMobile\"])() || this.isMobile) &&\n !this.isValueEmpty(this.isLogoImageChoosen()) &&\n !_settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].supportCreatorV2) {\n var logoWidth = this.logoWidth;\n if (this.logoPosition === \"left\" || this.logoPosition === \"right\") {\n return \"calc(100% - 5px - 2em - \" + logoWidth + \")\";\n }\n }\n return \"\";\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.updateRenderBackgroundImage = function () {\n var path = this.backgroundImage;\n this.renderBackgroundImage = Object(_utils_utils__WEBPACK_IMPORTED_MODULE_15__[\"wrapUrlForBackgroundImage\"])(path);\n };\n Object.defineProperty(SurveyModel.prototype, \"backgroundOpacity\", {\n /**\n * A value from 0 to 1 that specifies how transparent the [background image](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#backgroundImage) should be: 0 makes the image completely transparent, and 1 makes it opaque.\n */\n get: function () {\n return this.getPropertyValue(\"backgroundOpacity\");\n },\n set: function (val) {\n this.setPropertyValue(\"backgroundOpacity\", val);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.updateBackgroundImageStyle = function () {\n this.backgroundImageStyle = {\n opacity: this.backgroundOpacity,\n backgroundImage: this.renderBackgroundImage,\n backgroundSize: this.backgroundImageFit,\n backgroundAttachment: !this.fitToContainer ? this.backgroundImageAttachment : undefined\n };\n };\n SurveyModel.prototype.updateWrapperFormCss = function () {\n this.wrapperFormCss = new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_18__[\"CssClassBuilder\"]()\n .append(this.css.rootWrapper)\n .append(this.css.rootWrapperFixed, this.backgroundImageAttachment === \"fixed\")\n .toString();\n };\n Object.defineProperty(SurveyModel.prototype, \"completedHtml\", {\n /**\n * HTML content displayed on the [complete page](https://surveyjs.io/form-library/documentation/design-survey/create-a-multi-page-survey#complete-page).\n *\n * [View Demo](https://surveyjs.io/form-library/examples/modify-survey-navigation-settings/ (linkStyle))\n * @see showCompletedPage\n * @see completedHtmlOnCondition\n */\n get: function () {\n return this.getLocalizableStringText(\"completedHtml\");\n },\n set: function (value) {\n this.setLocalizableStringText(\"completedHtml\", value);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"locCompletedHtml\", {\n get: function () {\n return this.getLocalizableString(\"completedHtml\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"completedHtmlOnCondition\", {\n /**\n * An array of objects that allows you to specify different HTML content for the [complete page](https://surveyjs.io/form-library/documentation/design-survey/create-a-multi-page-survey#complete-page).\n *\n * Each object should include the [`expression`](https://surveyjs.io/form-library/documentation/api-reference/htmlconditionitem#expression) and [`html`](https://surveyjs.io/form-library/documentation/api-reference/htmlconditionitem#html) properties. When `expression` evaluates to `true`, the survey uses the corresponding HTML markup instead of [`completedHtml`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#completedHtml). Refer to the following help topic for more information about expressions: [Expressions](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#expressions).\n *\n * [View Demo](https://surveyjs.io/form-library/examples/nps-question/ (linkStyle))\n */\n get: function () {\n return this.getPropertyValue(\"completedHtmlOnCondition\");\n },\n set: function (val) {\n this.setPropertyValue(\"completedHtmlOnCondition\", val);\n },\n enumerable: false,\n configurable: true\n });\n /**\n * Calculates a given [expression](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#expressions) and returns a result value.\n * @param expression An expression to calculate.\n */\n SurveyModel.prototype.runExpression = function (expression) {\n if (!expression)\n return null;\n var values = this.getFilteredValues();\n var properties = this.getFilteredProperties();\n return new _conditions__WEBPACK_IMPORTED_MODULE_13__[\"ExpressionRunner\"](expression).run(values, properties);\n };\n /**\n * Calculates a given [expression](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#expressions) and returns `true` or `false`.\n * @param expression An expression to calculate.\n */\n SurveyModel.prototype.runCondition = function (expression) {\n if (!expression)\n return false;\n var values = this.getFilteredValues();\n var properties = this.getFilteredProperties();\n return new _conditions__WEBPACK_IMPORTED_MODULE_13__[\"ConditionRunner\"](expression).run(values, properties);\n };\n /**\n * Executes [all triggers](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#triggers), except [\"complete\"](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#complete).\n *\n * [Conditional Survey Logic (Triggers)](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#conditional-survey-logic-triggers (linkStyle))\n * @see onTriggerExecuted\n */\n SurveyModel.prototype.runTriggers = function () {\n this.checkTriggers(this.getFilteredValues(), false);\n };\n Object.defineProperty(SurveyModel.prototype, \"renderedCompletedHtml\", {\n get: function () {\n var item = this.getExpressionItemOnRunCondition(this.completedHtmlOnCondition);\n return !!item ? item.html : this.completedHtml;\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.getExpressionItemOnRunCondition = function (items) {\n if (items.length == 0)\n return null;\n var values = this.getFilteredValues();\n var properties = this.getFilteredProperties();\n for (var i = 0; i < items.length; i++) {\n if (items[i].runCondition(values, properties)) {\n return items[i];\n }\n }\n return null;\n };\n Object.defineProperty(SurveyModel.prototype, \"completedBeforeHtml\", {\n /**\n * HTML content displayed to a user who has completed the survey before. To identify such users, the survey uses a [cookie name](#cookieName) or [client ID](#clientId).\n * @see processedCompletedBeforeHtml\n */\n get: function () {\n return this.getLocalizableStringText(\"completedBeforeHtml\");\n },\n set: function (value) {\n this.setLocalizableStringText(\"completedBeforeHtml\", value);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"locCompletedBeforeHtml\", {\n get: function () {\n return this.getLocalizableString(\"completedBeforeHtml\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"loadingHtml\", {\n /**\n * HTML content displayed while a survey JSON schema is being loaded from [SurveyJS Service](https://api.surveyjs.io).\n * @see surveyId\n * @see processedLoadingHtml\n */\n get: function () {\n return this.getLocalizableStringText(\"loadingHtml\");\n },\n set: function (value) {\n this.setLocalizableStringText(\"loadingHtml\", value);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"locLoadingHtml\", {\n get: function () {\n return this.getLocalizableString(\"loadingHtml\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"defaultLoadingHtml\", {\n get: function () {\n return \"\" + this.getLocalizationString(\"loadingSurvey\") + \"
\";\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"navigationBar\", {\n get: function () {\n return this.navigationBarValue;\n },\n enumerable: false,\n configurable: true\n });\n /**\n * Adds a custom navigation item similar to the Previous Page, Next Page, and Complete buttons.\n * Accepts an object described in the [IAction](https://surveyjs.io/Documentation/Library?id=IAction) help section.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-changenavigation/ (linkStyle))\n */\n SurveyModel.prototype.addNavigationItem = function (val) {\n if (!val.component) {\n val.component = \"sv-nav-btn\";\n }\n if (!val.innerCss) {\n val.innerCss = this.cssSurveyNavigationButton;\n }\n return this.navigationBar.addAction(val);\n };\n Object.defineProperty(SurveyModel.prototype, \"startSurveyText\", {\n /**\n * Gets or sets a caption for the Start button.\n * @see firstPageIsStarted\n * @see [Localization & Globalization](https://surveyjs.io/form-library/documentation/survey-localization)\n */\n get: function () {\n return this.getLocalizableStringText(\"startSurveyText\");\n },\n set: function (newValue) {\n this.setLocalizableStringText(\"startSurveyText\", newValue);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"locStartSurveyText\", {\n get: function () {\n return this.getLocalizableString(\"startSurveyText\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"pagePrevText\", {\n /**\n * Gets or sets a caption for the Previous button.\n * @see [Localization & Globalization](https://surveyjs.io/form-library/documentation/survey-localization)\n */\n get: function () {\n return this.getLocalizableStringText(\"pagePrevText\");\n },\n set: function (newValue) {\n this.setLocalizableStringText(\"pagePrevText\", newValue);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"locPagePrevText\", {\n get: function () {\n return this.getLocalizableString(\"pagePrevText\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"pageNextText\", {\n /**\n * Gets or sets a caption for the Next button.\n * @see [Localization & Globalization](https://surveyjs.io/form-library/documentation/survey-localization)\n */\n get: function () {\n return this.getLocalizableStringText(\"pageNextText\");\n },\n set: function (newValue) {\n this.setLocalizableStringText(\"pageNextText\", newValue);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"locPageNextText\", {\n get: function () {\n return this.getLocalizableString(\"pageNextText\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"completeText\", {\n /**\n * Gets or sets a caption for the Complete button.\n * @see [Localization & Globalization](https://surveyjs.io/form-library/documentation/survey-localization)\n */\n get: function () {\n return this.getLocalizableStringText(\"completeText\");\n },\n set: function (newValue) {\n this.setLocalizableStringText(\"completeText\", newValue);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"locCompleteText\", {\n get: function () {\n return this.getLocalizableString(\"completeText\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"previewText\", {\n /**\n * Gets or sets a caption for the Preview button.\n * @see showPreviewBeforeComplete\n * @see showPreview\n * @see editText\n */\n get: function () {\n return this.getLocalizableStringText(\"previewText\");\n },\n set: function (newValue) {\n this.setLocalizableStringText(\"previewText\", newValue);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"locPreviewText\", {\n get: function () {\n return this.getLocalizableString(\"previewText\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"editText\", {\n /**\n * Gets or sets a caption for the Edit button displayed when the survey shows a [preview of given answers](https://surveyjs.io/form-library/documentation/design-survey/create-a-multi-page-survey#preview-page).\n * @see showPreviewBeforeComplete\n * @see cancelPreview\n * @see previewText\n */\n get: function () {\n return this.getLocalizableStringText(\"editText\");\n },\n set: function (newValue) {\n this.setLocalizableStringText(\"editText\", newValue);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"locEditText\", {\n get: function () {\n return this.getLocalizableString(\"editText\");\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.getElementTitleTagName = function (element, tagName) {\n if (this.onGetTitleTagName.isEmpty)\n return tagName;\n var options = { element: element, tagName: tagName };\n this.onGetTitleTagName.fire(this, options);\n return options.tagName;\n };\n Object.defineProperty(SurveyModel.prototype, \"questionTitlePattern\", {\n /**\n * Specifies a pattern for question titles.\n *\n * Refer to the following help topic for more information: [Title Pattern](https://surveyjs.io/form-library/documentation/design-survey/configure-question-titles#title-pattern).\n */\n get: function () {\n return this.getPropertyValue(\"questionTitlePattern\", \"numTitleRequire\");\n },\n set: function (val) {\n if (val !== \"numRequireTitle\" &&\n val !== \"requireNumTitle\" &&\n val != \"numTitle\") {\n val = \"numTitleRequire\";\n }\n this.setPropertyValue(\"questionTitlePattern\", val);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.getQuestionTitlePatternOptions = function () {\n var res = new Array();\n var title = this.getLocalizationString(\"questionTitlePatternText\");\n var num = !!this.questionStartIndex ? this.questionStartIndex : \"1.\";\n res.push({\n value: \"numTitleRequire\",\n text: num + \" \" + title + \" \" + this.requiredText\n });\n res.push({\n value: \"numRequireTitle\",\n text: num + \" \" + this.requiredText + \" \" + title\n });\n res.push({\n value: \"requireNumTitle\",\n text: this.requiredText + \" \" + num + \" \" + title\n });\n res.push({\n value: \"numTitle\",\n text: num + \" \" + title\n });\n return res;\n };\n Object.defineProperty(SurveyModel.prototype, \"questionTitleTemplate\", {\n get: function () {\n return this.getLocalizableStringText(\"questionTitleTemplate\");\n },\n set: function (value) {\n this.setLocalizableStringText(\"questionTitleTemplate\", value);\n this.questionTitlePattern = this.getNewTitlePattern(value);\n this.questionStartIndex = this.getNewQuestionTitleElement(value, \"no\", this.questionStartIndex, \"1\");\n this.requiredText = this.getNewQuestionTitleElement(value, \"require\", this.requiredText, \"*\");\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.getNewTitlePattern = function (template) {\n if (!!template) {\n var strs = [];\n while (template.indexOf(\"{\") > -1) {\n template = template.substring(template.indexOf(\"{\") + 1);\n var ind = template.indexOf(\"}\");\n if (ind < 0)\n break;\n strs.push(template.substring(0, ind));\n template = template.substring(ind + 1);\n }\n if (strs.length > 1) {\n if (strs[0] == \"require\")\n return \"requireNumTitle\";\n if (strs[1] == \"require\" && strs.length == 3)\n return \"numRequireTitle\";\n if (strs.indexOf(\"require\") < 0)\n return \"numTitle\";\n }\n if (strs.length == 1 && strs[0] == \"title\") {\n return \"numTitle\";\n }\n }\n return \"numTitleRequire\";\n };\n SurveyModel.prototype.getNewQuestionTitleElement = function (template, name, currentValue, defaultValue) {\n name = \"{\" + name + \"}\";\n if (!template || template.indexOf(name) < 0)\n return currentValue;\n var ind = template.indexOf(name);\n var prefix = \"\";\n var postfix = \"\";\n var i = ind - 1;\n for (; i >= 0; i--) {\n if (template[i] == \"}\")\n break;\n }\n if (i < ind - 1) {\n prefix = template.substring(i + 1, ind);\n }\n ind += name.length;\n i = ind;\n for (; i < template.length; i++) {\n if (template[i] == \"{\")\n break;\n }\n if (i > ind) {\n postfix = template.substring(ind, i);\n }\n i = 0;\n while (i < prefix.length && prefix.charCodeAt(i) < 33)\n i++;\n prefix = prefix.substring(i);\n i = postfix.length - 1;\n while (i >= 0 && postfix.charCodeAt(i) < 33)\n i--;\n postfix = postfix.substring(0, i + 1);\n if (!prefix && !postfix)\n return currentValue;\n var value = !!currentValue ? currentValue : defaultValue;\n return prefix + value + postfix;\n };\n Object.defineProperty(SurveyModel.prototype, \"locQuestionTitleTemplate\", {\n get: function () {\n return this.getLocalizableString(\"questionTitleTemplate\");\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.getUpdatedQuestionTitle = function (question, title) {\n if (this.onGetQuestionTitle.isEmpty)\n return title;\n var options = { question: question, title: title };\n this.onGetQuestionTitle.fire(this, options);\n return options.title;\n };\n SurveyModel.prototype.getUpdatedQuestionNo = function (question, no) {\n if (this.onGetQuestionNo.isEmpty)\n return no;\n var options = { question: question, no: no };\n this.onGetQuestionNo.fire(this, options);\n return options.no;\n };\n Object.defineProperty(SurveyModel.prototype, \"showPageNumbers\", {\n /**\n * Specifies whether page titles contain page numbers.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/how-to-number-pages-and-questions/ (linkStyle))\n */\n get: function () {\n return this.getPropertyValue(\"showPageNumbers\");\n },\n set: function (value) {\n if (value === this.showPageNumbers)\n return;\n this.setPropertyValue(\"showPageNumbers\", value);\n this.updateVisibleIndexes();\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"showQuestionNumbers\", {\n /**\n * Specifies whether to display question numbers and how to calculate them.\n *\n * Possible values:\n *\n * - `true` or `\"on\"` - Displays question numbers.\n * - `\"onpage\"` - Displays question numbers and starts numbering on each page from scratch.\n * - `false` or `\"off\"` - Hides question numbers.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/how-to-number-pages-and-questions/ (linkStyle))\n *\n * If you want to hide the number of an individual question, enable its [`hideNumber`](https://surveyjs.io/form-library/documentation/api-reference/question#hideNumber) property.\n */\n get: function () {\n return this.getPropertyValue(\"showQuestionNumbers\");\n },\n set: function (value) {\n if (value === true) {\n value = \"on\";\n }\n if (value === false) {\n value = \"off\";\n }\n value = value.toLowerCase();\n value = value === \"onpage\" ? \"onPage\" : value;\n if (value === this.showQuestionNumbers)\n return;\n this.setPropertyValue(\"showQuestionNumbers\", value);\n this.updateVisibleIndexes();\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"progressBar\", {\n get: function () {\n return this.progressBarValue;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"showProgressBar\", {\n /**\n * Controls the visibility of the progress bar and specifies its position.\n *\n * Possible values:\n *\n * - `\"off\"` (default) - Hides the progress bar.\n * - `\"aboveHeader\"` - Displays the progress bar above the survey header.\n * - `\"belowHeader\"` - Displays the progress bar below the survey header.\n * - `\"bottom\"` - Displays the progress bar below survey content.\n * - `\"topBottom\"` - Displays the progress bar above and below survey content.\n * - `\"auto\"` - Displays the progress bar below the survey header if the header has a [background image](https://surveyjs.io/form-library/documentation/api-reference/iheader#backgroundImage) or color. Otherwise, the progress bar is displayed above the header.\n * - `\"top\"` - *(Obsolete)* Use the `\"aboveHeader\"` or `\"belowHeader\"` property value instead.\n * - `\"both\"` - *(Obsolete)* Use the `\"topBottom\"` property value instead.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/navigation-default/ (linkStyle))\n * @see progressBarType\n * @see progressValue\n */\n get: function () {\n return this.getPropertyValue(\"showProgressBar\");\n },\n set: function (newValue) {\n this.setPropertyValue(\"showProgressBar\", newValue.toLowerCase());\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"progressBarType\", {\n /**\n * Specifies the type of information displayed by the progress bar. Applies only when [`showProgressBar`](#showProgressBar) is not `\"off\"`.\n *\n * Possible values:\n *\n * - `\"pages\"` (default) - The number of completed pages.\n * - `\"questions\"` - The number of answered questions.\n * - `\"requiredQuestions\"` - The number of answered [required questions](https://surveyjs.io/form-library/documentation/api-reference/question#isRequired).\n * - `\"correctQuestions\"` - The number of correct questions in a [quiz](https://surveyjs.io/form-library/documentation/design-survey/create-a-quiz).\n * - `\"buttons\"` - *(Obsolete)* Use the `\"pages\"` property value with the [`progressBarShowPageTitles`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#progressBarShowPageTitles) property set to `true` instead.\n *\n * > When `progressBarType` is set to `\"pages\"`, you can also enable the [`progressBarShowPageNumbers`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#progressBarShowPageNumbers) and [`progressBarShowPageTitles`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#progressBarShowPageTitles) properties if you want to display page numbers and titles in the progress bar.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/navigation-buttons/ (linkStyle))\n * @see progressValue\n */\n get: function () {\n return this.getPropertyValue(\"progressBarType\");\n },\n set: function (newValue) {\n if (newValue === \"correctquestion\")\n newValue = \"correctQuestion\";\n if (newValue === \"requiredquestion\")\n newValue = \"requiredQuestion\";\n // if (newValue === \"buttons\") {\n // newValue = \"pages\";\n // this.progressBarShowPageTitles = true;\n // }\n this.setPropertyValue(\"progressBarType\", newValue);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"progressBarComponentName\", {\n get: function () {\n var actualProgressBarType = this.progressBarType;\n if (!_settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].legacyProgressBarView && _defaultCss_defaultV2Css__WEBPACK_IMPORTED_MODULE_4__[\"surveyCss\"].currentType === \"defaultV2\") {\n if (isStrCiEqual(actualProgressBarType, \"pages\")) {\n actualProgressBarType = \"buttons\";\n }\n }\n return \"progress-\" + actualProgressBarType;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isShowProgressBarOnTop\", {\n get: function () {\n if (!this.canShowProresBar())\n return false;\n return [\"auto\", \"aboveheader\", \"belowheader\", \"topbottom\", \"top\", \"both\"].indexOf(this.showProgressBar) !== -1;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isShowProgressBarOnBottom\", {\n get: function () {\n if (!this.canShowProresBar())\n return false;\n return this.showProgressBar === \"bottom\" || this.showProgressBar === \"both\" || this.showProgressBar === \"topbottom\";\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.getProgressTypeComponent = function () {\n return \"sv-progress-\" + this.progressBarType.toLowerCase();\n };\n SurveyModel.prototype.getProgressCssClasses = function (container) {\n if (container === void 0) { container = \"\"; }\n return new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_18__[\"CssClassBuilder\"]()\n .append(this.css.progress)\n .append(this.css.progressTop, this.isShowProgressBarOnTop && (!container || container == \"header\"))\n .append(this.css.progressBottom, this.isShowProgressBarOnBottom && (!container || container == \"footer\"))\n .toString();\n };\n SurveyModel.prototype.canShowProresBar = function () {\n return (!this.isShowingPreview ||\n this.showPreviewBeforeComplete != \"showAllQuestions\");\n };\n Object.defineProperty(SurveyModel.prototype, \"processedTitle\", {\n get: function () {\n return this.locTitle.renderedHtml;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"questionTitleLocation\", {\n /**\n * Gets or sets question title location relative to the input field: `\"top\"`, `\"bottom\"`, or `\"left\"`.\n *\n * > Certain question types (Matrix, Multiple Text) do not support the `\"left\"` value. For them, the `\"top\"` value is used.\n *\n * You can override this setting if you specify the `questionTitleLocation` property for an [individual page](https://surveyjs.io/form-library/documentation/pagemodel#questionTitleLocation) or [panel](https://surveyjs.io/form-library/documentation/panelmodel#questionTitleLocation) or set the `titleLocation` property for a [specific question](https://surveyjs.io/form-library/documentation/question#titleLocation).\n */\n get: function () {\n return this.getPropertyValue(\"questionTitleLocation\");\n },\n set: function (value) {\n this.setPropertyValue(\"questionTitleLocation\", value.toLowerCase());\n if (!this.isLoadingFromJson) {\n this.updateElementCss(true);\n }\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.updateElementCss = function (reNew) {\n if (!!this.startedPage) {\n this.startedPage.updateElementCss(reNew);\n }\n var pages = this.visiblePages;\n for (var i = 0; i < pages.length; i++) {\n pages[i].updateElementCss(reNew);\n }\n this.updateCss();\n };\n Object.defineProperty(SurveyModel.prototype, \"questionErrorLocation\", {\n /**\n * Specifies the error message position.\n *\n * Possible values:\n *\n * - `\"top\"` (default) - Displays error messages above questions.\n * - `\"bottom\"` - Displays error messages below questions.\n *\n * You can override this setting if you specify the `questionErrorLocation` property for an [individual page](https://surveyjs.io/form-library/documentation/pagemodel#questionErrorLocation) or [panel](https://surveyjs.io/form-library/documentation/panelmodel#questionErrorLocation) or set the `errorLocation` property for a [specific question](https://surveyjs.io/form-library/documentation/question#errorLocation).\n */\n get: function () {\n return this.getPropertyValue(\"questionErrorLocation\");\n },\n set: function (value) {\n this.setPropertyValue(\"questionErrorLocation\", value.toLowerCase());\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"questionDescriptionLocation\", {\n /**\n * Specifies where to display question descriptions.\n *\n * Possible values:\n *\n * - `\"underTitle\"` (default) - Displays descriptions under question titles.\n * - `\"underInput\"` - Displays descriptions under the interactive area.\n *\n * You can override this setting for individual questions if you specify their [`descriptionLocation`](https://surveyjs.io/form-library/documentation/api-reference/question#descriptionLocation) property.\n *\n */\n get: function () {\n return this.getPropertyValue(\"questionDescriptionLocation\");\n },\n set: function (value) {\n this.setPropertyValue(\"questionDescriptionLocation\", value);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"mode\", {\n /**\n * Specifies whether users can take the survey or only view it.\n *\n * Possible values:\n *\n * - `\"edit\"` (default) - Allows users to take the survey.\n * - `\"display\"` - Makes the survey read-only.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-displaymode/ (linkStyle))\n */\n get: function () {\n return this.getPropertyValue(\"mode\");\n },\n set: function (value) {\n value = value.toLowerCase();\n if (value == this.mode)\n return;\n if (value != \"edit\" && value != \"display\")\n return;\n this.setPropertyValue(\"mode\", value);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.onModeChanged = function () {\n for (var i = 0; i < this.pages.length; i++) {\n var page = this.pages[i];\n page.setPropertyValue(\"isReadOnly\", page.isReadOnly);\n }\n this.updateButtonsVisibility();\n this.updateCss();\n };\n Object.defineProperty(SurveyModel.prototype, \"data\", {\n /**\n * Gets or sets an object with survey results. You can set this property with an object of the following structure:\n *\n * ```js\n * {\n * question1Name: question1Value,\n * question2Name: question2Value,\n * // ...\n * }\n * ```\n *\n * When you set this property in code, the new object overrides the old object that may contain default question values and entered data. If you want to *merge* the new and old objects, call the [`mergeData(newDataObj)`](https://surveyjs.io/form-library/documentation/surveymodel#mergeData) method.\n *\n * If you assign a new object while a respondent takes the survey, set the [`currentPageNo`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#currentPageNo) property to 0 to start the survey from the beginning. This will also cause the survey to re-evaluate the [`visibleIf`](https://surveyjs.io/form-library/documentation/api-reference/question#visibleIf), [`enableIf`](https://surveyjs.io/form-library/documentation/api-reference/question#enableIf), and other [expressions](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#expressions).\n * @see setValue\n * @see getValue\n */\n get: function () {\n var result = {};\n var keys = this.getValuesKeys();\n for (var i = 0; i < keys.length; i++) {\n var key = keys[i];\n var dataValue = this.getDataValueCore(this.valuesHash, key);\n if (dataValue !== undefined) {\n result[key] = dataValue;\n }\n }\n this.setCalculatedValuesIntoResult(result);\n return result;\n },\n set: function (data) {\n this.valuesHash = {};\n this.setDataCore(data, !data);\n },\n enumerable: false,\n configurable: true\n });\n /**\n * Merges a specified data object with the object from the [`data`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#data) property.\n *\n * Refer to the following help topic for more information: [Populate Form Fields | Multiple Question Values](https://surveyjs.io/form-library/documentation/design-survey/pre-populate-form-fields#multiple-question-values).\n *\n * @param data A data object to merge. It should have the following structure: `{ questionName: questionValue, ... }`\n * @see setValue\n */\n SurveyModel.prototype.mergeData = function (data) {\n if (!data)\n return;\n var newData = this.data;\n this.mergeValues(data, newData);\n this.setDataCore(newData);\n };\n SurveyModel.prototype.setDataCore = function (data, clearData) {\n if (clearData === void 0) { clearData = false; }\n if (clearData) {\n this.valuesHash = {};\n }\n if (data) {\n for (var key in data) {\n this.setDataValueCore(this.valuesHash, key, data[key]);\n }\n }\n this.updateAllQuestionsValue(clearData);\n this.notifyAllQuestionsOnValueChanged();\n this.notifyElementsOnAnyValueOrVariableChanged(\"\");\n this.runConditions();\n this.updateAllQuestionsValue(clearData);\n };\n SurveyModel.prototype.getStructuredData = function (includePages, level) {\n if (includePages === void 0) { includePages = true; }\n if (level === void 0) { level = -1; }\n if (level === 0)\n return this.data;\n var data = {};\n this.pages.forEach(function (p) {\n if (includePages) {\n var pageValues = {};\n if (p.collectValues(pageValues, level - 1)) {\n data[p.name] = pageValues;\n }\n }\n else {\n p.collectValues(data, level);\n }\n });\n return data;\n };\n SurveyModel.prototype.setStructuredData = function (data, doMerge) {\n if (doMerge === void 0) { doMerge = false; }\n if (!data)\n return;\n var res = {};\n for (var key in data) {\n var q = this.getQuestionByValueName(key);\n if (q) {\n res[key] = data[key];\n }\n else {\n var panel = this.getPageByName(key);\n if (!panel) {\n panel = this.getPanelByName(key);\n }\n if (panel) {\n this.collectDataFromPanel(panel, res, data[key]);\n }\n }\n }\n if (doMerge) {\n this.mergeData(res);\n }\n else {\n this.data = res;\n }\n };\n SurveyModel.prototype.collectDataFromPanel = function (panel, output, data) {\n for (var key in data) {\n var el = panel.getElementByName(key);\n if (!el)\n continue;\n if (el.isPanel) {\n this.collectDataFromPanel(el, output, data[key]);\n }\n else {\n output[key] = data[key];\n }\n }\n };\n Object.defineProperty(SurveyModel.prototype, \"editingObj\", {\n get: function () {\n return this.editingObjValue;\n },\n set: function (val) {\n var _this = this;\n if (this.editingObj == val)\n return;\n if (!!this.editingObj) {\n this.editingObj.onPropertyChanged.remove(this.onEditingObjPropertyChanged);\n }\n this.editingObjValue = val;\n if (this.isDisposed)\n return;\n if (!val) {\n var questions = this.getAllQuestions();\n for (var i = 0; i < questions.length; i++) {\n questions[i].unbindValue();\n }\n }\n if (!!this.editingObj) {\n this.setDataCore({});\n this.onEditingObjPropertyChanged = function (sender, options) {\n if (!_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"Serializer\"].hasOriginalProperty(_this.editingObj, options.name))\n return;\n if (options.name === \"locale\") {\n _this.setDataCore({});\n }\n _this.updateOnSetValue(options.name, _this.editingObj[options.name], options.oldValue);\n };\n this.editingObj.onPropertyChanged.add(this.onEditingObjPropertyChanged);\n }\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isEditingSurveyElement\", {\n get: function () {\n return !!this.editingObj;\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.setCalculatedValuesIntoResult = function (result) {\n for (var i = 0; i < this.calculatedValues.length; i++) {\n var calValue = this.calculatedValues[i];\n if (calValue.includeIntoResult &&\n !!calValue.name &&\n this.getVariable(calValue.name) !== undefined) {\n result[calValue.name] = this.getVariable(calValue.name);\n }\n }\n };\n SurveyModel.prototype.getAllValues = function () {\n return this.data;\n };\n /**\n * Returns survey results as an array of objects in which the question name, title, value, and other parameters are stored as individual properties.\n *\n * If a question can have more than one value (Matrix, Multiple Text), its object enables the `isNode` flag and stores information about these values in the `data` property. Refer to the following help topic for more information: [Access Full Survey Results](https://surveyjs.io/form-library/documentation/handle-survey-results-access#access-full-survey-results).\n *\n * If you want to skip empty answers, pass an object with the `includeEmpty` property set to `false`.\n */\n SurveyModel.prototype.getPlainData = function (options) {\n if (!options) {\n options = { includeEmpty: true, includeQuestionTypes: false, includeValues: false };\n }\n var result = [];\n var questionValueNames = [];\n this.getAllQuestions().forEach(function (question) {\n var resultItem = question.getPlainData(options);\n if (!!resultItem) {\n result.push(resultItem);\n questionValueNames.push(question.valueName || question.name);\n }\n });\n if (!!options.includeValues) {\n var keys = this.getValuesKeys();\n for (var i = 0; i < keys.length; i++) {\n var key = keys[i];\n if (questionValueNames.indexOf(key) == -1) {\n var dataValue = this.getDataValueCore(this.valuesHash, key);\n if (!!dataValue) {\n result.push({\n name: key,\n title: key,\n value: dataValue,\n displayValue: dataValue,\n isNode: false,\n getString: function (val) {\n return typeof val === \"object\" ? JSON.stringify(val) : val;\n },\n });\n }\n }\n }\n }\n return result;\n };\n SurveyModel.prototype.getFilteredValues = function () {\n var values = {};\n for (var key in this.variablesHash)\n values[key] = this.variablesHash[key];\n this.addCalculatedValuesIntoFilteredValues(values);\n var keys = this.getValuesKeys();\n for (var i = 0; i < keys.length; i++) {\n var key = keys[i];\n values[key] = this.getDataValueCore(this.valuesHash, key);\n }\n this.getAllQuestions().forEach(function (q) {\n if (q.hasFilteredValue) {\n values[q.getValueName()] = q.getFilteredValue();\n }\n });\n return values;\n };\n SurveyModel.prototype.addCalculatedValuesIntoFilteredValues = function (values) {\n var caclValues = this.calculatedValues;\n for (var i = 0; i < caclValues.length; i++)\n values[caclValues[i].name] = caclValues[i].value;\n };\n SurveyModel.prototype.getFilteredProperties = function () {\n return { survey: this };\n };\n SurveyModel.prototype.getValuesKeys = function () {\n if (!this.editingObj)\n return Object.keys(this.valuesHash);\n var props = _jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"Serializer\"].getPropertiesByObj(this.editingObj);\n var res = [];\n for (var i = 0; i < props.length; i++) {\n res.push(props[i].name);\n }\n return res;\n };\n SurveyModel.prototype.getDataValueCore = function (valuesHash, key) {\n if (!!this.editingObj)\n return _jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"Serializer\"].getObjPropertyValue(this.editingObj, key);\n return this.getDataFromValueHash(valuesHash, key);\n };\n SurveyModel.prototype.setDataValueCore = function (valuesHash, key, value) {\n if (!!this.editingObj) {\n _jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"Serializer\"].setObjPropertyValue(this.editingObj, key, value);\n }\n else {\n this.setDataToValueHash(valuesHash, key, value);\n }\n };\n SurveyModel.prototype.deleteDataValueCore = function (valuesHash, key) {\n if (!!this.editingObj) {\n this.editingObj[key] = null;\n }\n else {\n this.deleteDataFromValueHash(valuesHash, key);\n }\n };\n SurveyModel.prototype.getDataFromValueHash = function (valuesHash, key) {\n if (!!this.valueHashGetDataCallback)\n return this.valueHashGetDataCallback(valuesHash, key);\n return valuesHash[key];\n };\n SurveyModel.prototype.setDataToValueHash = function (valuesHash, key, value) {\n if (!!this.valueHashSetDataCallback) {\n this.valueHashSetDataCallback(valuesHash, key, value);\n }\n else {\n valuesHash[key] = value;\n }\n };\n SurveyModel.prototype.deleteDataFromValueHash = function (valuesHash, key) {\n if (!!this.valueHashDeleteDataCallback) {\n this.valueHashDeleteDataCallback(valuesHash, key);\n }\n else {\n delete valuesHash[key];\n }\n };\n Object.defineProperty(SurveyModel.prototype, \"comments\", {\n /**\n * An object with all comment values.\n * @see Question.showCommentArea\n * @see storeOthersAsComment\n */\n get: function () {\n var result = {};\n var keys = this.getValuesKeys();\n for (var i = 0; i < keys.length; i++) {\n var key = keys[i];\n if (key.indexOf(this.commentSuffix) > 0) {\n result[key] = this.getDataValueCore(this.valuesHash, key);\n }\n }\n return result;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"visiblePages\", {\n /**\n * Returns an array of visible pages without the start page.\n *\n * To get an array of all pages, use the [`pages`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#pages) property. If all pages are visible, the `pages` and `visiblePages` arrays are identical.\n * @see [Conditional Visibility](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#conditional-visibility)\n */\n get: function () {\n if (this.isDesignMode)\n return this.pages;\n var result = new Array();\n for (var i = 0; i < this.pages.length; i++) {\n if (this.isPageInVisibleList(this.pages[i])) {\n result.push(this.pages[i]);\n }\n }\n return result;\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.isPageInVisibleList = function (page) {\n return this.isDesignMode || page.isVisible && !page.isStartPage;\n };\n Object.defineProperty(SurveyModel.prototype, \"isEmpty\", {\n /**\n * Returns `true` if the survey contains zero pages.\n * @see emptySurveyText\n */\n get: function () {\n return this.pages.length == 0;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"PageCount\", {\n get: function () {\n return this.pageCount;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"pageCount\", {\n /**\n * Returns a total number of survey pages.\n *\n * To get the number of visible pages, use the [`visiblePageCount`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#visiblePageCount) property.\n * @see pages\n */\n get: function () {\n return this.pages.length;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"visiblePageCount\", {\n /**\n * Returns the number of visible survey pages.\n *\n * To get a total number of survey pages, use the [`pageCount`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#pageCount) property.\n * @see visiblePages\n * @see [Conditional Visibility](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#conditional-visibility)\n */\n get: function () {\n return this.visiblePages.length;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"startedPage\", {\n /**\n * Returns the start page. Applies only if the [`firstPageIsStarted`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#firstPageIsStarted) property is set to `true`.\n *\n * Refer to the following help topic for more information: [Start Page](https://surveyjs.io/form-library/documentation/design-survey/create-a-multi-page-survey#start-page).\n * @see firstPageIsStarted\n * @see activePage\n */\n get: function () {\n var page = this.firstPageIsStarted && this.pages.length > 1 ? this.pages[0] : null;\n if (!!page) {\n page.onFirstRendering();\n page.setWasShown(true);\n }\n return page;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"currentPage\", {\n /**\n * Gets or sets the current page.\n *\n * If you want to change the current page, set this property to a `PageModel` object. You can get this object in different ways. For example, you can call the [`getPageByName()`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#getPageByName) method to obtain a `PageModel` object with a specific name:\n *\n * ```js\n * survey.currentPage = survey.getPageByName(\"my-page-name\");\n * ```\n *\n * Alternatively, you can change the current page if you set the [`currentPageNo`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#currentPageNo) property to the index of the required page.\n *\n * The `currentPage` property does not return the start page even if it is current. Use the [`activePage`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#activePage) property instead if your survey contains a start page.\n */\n get: function () {\n return this.getPropertyValue(\"currentPage\", null);\n },\n set: function (value) {\n if (this.isLoadingFromJson)\n return;\n var newPage = this.getPageByObject(value);\n if (!!value && !newPage)\n return;\n if (!newPage && this.isCurrentPageAvailable)\n return;\n var vPages = this.visiblePages;\n if (newPage != null && vPages.indexOf(newPage) < 0)\n return;\n if (newPage == this.currentPage)\n return;\n var oldValue = this.currentPage;\n if (!this.isShowingPreview && !this.currentPageChanging(newPage, oldValue))\n return;\n this.setPropertyValue(\"currentPage\", newPage);\n if (!!newPage) {\n newPage.onFirstRendering();\n newPage.updateCustomWidgets();\n newPage.setWasShown(true);\n }\n this.locStrsChanged();\n if (!this.isShowingPreview) {\n this.currentPageChanged(newPage, oldValue);\n }\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.tryNavigateToPage = function (page) {\n if (this.isDesignMode)\n return false;\n var index = this.visiblePages.indexOf(page);\n if (index < 0 || index >= this.visiblePageCount)\n return false;\n if (index === this.currentPageNo)\n return false;\n if (index < this.currentPageNo || this.isValidateOnComplete) {\n this.currentPageNo = index;\n return true;\n }\n for (var i = this.currentPageNo; i < index; i++) {\n var page_1 = this.visiblePages[i];\n if (!page_1.validate(true, true))\n return false;\n page_1.passed = true;\n }\n this.currentPage = page;\n return true;\n };\n SurveyModel.prototype.updateCurrentPage = function () {\n if (this.isCurrentPageAvailable)\n return;\n this.currentPage = this.firstVisiblePage;\n };\n Object.defineProperty(SurveyModel.prototype, \"isCurrentPageAvailable\", {\n get: function () {\n var page = this.currentPage;\n return !!page && this.isPageInVisibleList(page) && this.isPageExistsInSurvey(page);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.isPageExistsInSurvey = function (page) {\n if (this.pages.indexOf(page) > -1)\n return true;\n return !!this.onContainsPageCallback && this.onContainsPageCallback(page);\n };\n Object.defineProperty(SurveyModel.prototype, \"activePage\", {\n /**\n * Returns [`startedPage`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#startedPage) if the survey currently displays a start page; otherwise, returns [`currentPage`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#currentPage).\n * @see startedPage\n * @see currentPage\n * @see firstPageIsStarted\n */\n get: function () {\n return this.getPropertyValue(\"activePage\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isShowStartingPage\", {\n /**\n * A Boolean value that indicates whether the [start page](https://surveyjs.io/form-library/documentation/design-survey/create-a-multi-page-survey#start-page) is currently displayed.\n */\n get: function () {\n return this.state === \"starting\";\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"matrixDragHandleArea\", {\n /**\n * Specifies which part of a matrix row responds to a drag gesture in [Dynamic Matrix](https://surveyjs.io/form-library/examples/questiontype-matrixdynamic/) questions.\n *\n * Possible values:\n *\n * - `\"entireItem\"` (default) - Users can use the entire matrix row as a drag handle.\n * - `\"icon\"` - Users can only use a drag icon as a drag handle.\n */\n get: function () {\n return this.getPropertyValue(\"matrixDragHandleArea\", \"entireItem\");\n },\n set: function (val) {\n this.setPropertyValue(\"matrixDragHandleArea\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isShowingPage\", {\n get: function () {\n return this.state == \"running\" || this.state == \"preview\" || this.isShowStartingPage;\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.updateActivePage = function () {\n var newPage = this.isShowStartingPage ? this.startedPage : this.currentPage;\n this.setPropertyValue(\"activePage\", newPage);\n };\n SurveyModel.prototype.onStateAndCurrentPageChanged = function () {\n this.updateActivePage();\n this.updateButtonsVisibility();\n };\n SurveyModel.prototype.getPageByObject = function (value) {\n if (!value)\n return null;\n if (value.getType && value.getType() == \"page\")\n return value;\n if (typeof value === \"string\" || value instanceof String)\n return this.getPageByName(String(value));\n if (!isNaN(value)) {\n var index = Number(value);\n var vPages = this.visiblePages;\n if (value < 0 || value >= vPages.length)\n return null;\n return vPages[index];\n }\n return value;\n };\n Object.defineProperty(SurveyModel.prototype, \"currentPageNo\", {\n /**\n * A zero-based index of the current page in the [`visiblePages`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#visiblePages) array.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-editprevious/ (linkStyle))\n * @see visiblePages\n */\n get: function () {\n return this.visiblePages.indexOf(this.currentPage);\n },\n set: function (value) {\n var vPages = this.visiblePages;\n if (value < 0 || value >= vPages.length)\n return;\n this.currentPage = vPages[value];\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"questionsOrder\", {\n /**\n * Specifies the sort order of questions in the survey.\n *\n * Possible values:\n *\n * - `\"initial\"` (default) - Preserves the original order of questions.\n * - `\"random\"` - Displays questions in random order.\n *\n * You can override this property for individual pages and panels.\n * @see PageModel.questionsOrder\n * @see PanelModel.questionsOrder\n */\n get: function () {\n return this.getPropertyValue(\"questionsOrder\");\n },\n set: function (val) {\n this.setPropertyValue(\"questionsOrder\", val);\n },\n enumerable: false,\n configurable: true\n });\n /**\n * Focuses the first question on the current page.\n * @see focusQuestion\n * @see focusFirstQuestionAutomatic\n */\n SurveyModel.prototype.focusFirstQuestion = function () {\n if (this.focusingQuestionInfo)\n return;\n var page = this.activePage;\n if (page) {\n page.scrollToTop();\n page.focusFirstQuestion();\n }\n };\n SurveyModel.prototype.scrollToTopOnPageChange = function (doScroll) {\n if (doScroll === void 0) { doScroll = true; }\n var page = this.activePage;\n if (!page)\n return;\n if (doScroll) {\n page.scrollToTop();\n }\n if (this.isCurrentPageRendering && this.focusFirstQuestionAutomatic && !this.focusingQuestionInfo) {\n page.focusFirstQuestion();\n this.isCurrentPageRendering = false;\n }\n };\n Object.defineProperty(SurveyModel.prototype, \"state\", {\n /**\n * Returns the current survey state.\n *\n * Possible values:\n *\n * - `\"loading\"` - The survey is being loaded from a JSON schema.\n * - `\"empty\"` - The survey has no elements to display.\n * - `\"starting\"` - The survey displays a [start page](https://surveyjs.io/form-library/documentation/design-survey/create-a-multi-page-survey#start-page).\n * - `\"running\"` - A respondent is taking the survey.\n * - `\"preview\"` - A respondent is [previewing](https://surveyjs.io/form-library/examples/survey-showpreview/) answers before submitting them.\n * - `\"completed\"` - A respondent has completed the survey and submitted the results.\n */\n get: function () {\n return this.getPropertyValue(\"state\", \"empty\");\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.updateState = function () {\n this.setPropertyValue(\"state\", this.calcState());\n };\n SurveyModel.prototype.calcState = function () {\n if (this.isLoading)\n return \"loading\";\n if (this.isCompleted)\n return \"completed\";\n if (this.isCompletedBefore)\n return \"completedbefore\";\n if (!this.isDesignMode &&\n this.isEditMode &&\n this.isStartedState &&\n this.startedPage)\n return \"starting\";\n if (this.isShowingPreview)\n return this.currentPage ? \"preview\" : \"empty\";\n return this.currentPage ? \"running\" : \"empty\";\n };\n Object.defineProperty(SurveyModel.prototype, \"isCompleted\", {\n get: function () {\n return this.getPropertyValue(\"isCompleted\", false);\n },\n set: function (val) {\n this.setPropertyValue(\"isCompleted\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isShowingPreview\", {\n get: function () {\n return this.getPropertyValue(\"isShowingPreview\", false);\n },\n set: function (val) {\n if (this.isShowingPreview == val)\n return;\n this.setPropertyValue(\"isShowingPreview\", val);\n this.onShowingPreviewChanged();\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isStartedState\", {\n get: function () {\n return this.getPropertyValue(\"isStartedState\", false);\n },\n set: function (val) {\n this.setPropertyValue(\"isStartedState\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isCompletedBefore\", {\n get: function () {\n return this.getPropertyValue(\"isCompletedBefore\", false);\n },\n set: function (val) {\n this.setPropertyValue(\"isCompletedBefore\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isLoading\", {\n get: function () {\n return this.getPropertyValue(\"isLoading\", false);\n },\n set: function (val) {\n this.setPropertyValue(\"isLoading\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"completedState\", {\n get: function () {\n return this.getPropertyValue(\"completedState\", \"\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"completedStateText\", {\n get: function () {\n return this.getPropertyValue(\"completedStateText\", \"\");\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.setCompletedState = function (value, text) {\n this.setPropertyValue(\"completedState\", value);\n if (!text) {\n if (value == \"saving\")\n text = this.getLocalizationString(\"savingData\");\n if (value == \"error\")\n text = this.getLocalizationString(\"savingDataError\");\n if (value == \"success\")\n text = this.getLocalizationString(\"savingDataSuccess\");\n }\n this.setPropertyValue(\"completedStateText\", text);\n if (this.state === \"completed\" && this.showCompletedPage && !!this.completedState) {\n this.notify(this.completedStateText, this.completedState, value === \"error\");\n }\n };\n /**\n * Displays a toast notification with a specified message.\n *\n * Depending on the `type` argument, a survey can display the following notification types:\n *\n * 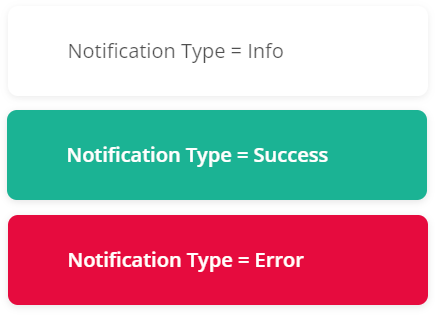\n * @param message A message to display.\n * @param type A notification type: `\"info\"` (default), `\"success\"`, or `\"error\"`.\n * @param showActions For internal use.\n */\n SurveyModel.prototype.notify = function (message, type, showActions) {\n if (showActions === void 0) { showActions = false; }\n this.notifier.showActions = showActions;\n this.notifier.notify(message, type, showActions);\n };\n /**\n * Resets the survey [`state`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#state) and, optionally, [`data`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#data). If `state` is `\"completed\"`, it becomes `\"running\"`.\n * @param clearData *(Optional)* Specifies whether to clear survey data. Default value: `true`.\n * @param goToFirstPage *(Optional)* Specifies whether to switch the survey to the first page. Default value: `true`.\n */\n SurveyModel.prototype.clear = function (clearData, goToFirstPage) {\n if (clearData === void 0) { clearData = true; }\n if (goToFirstPage === void 0) { goToFirstPage = true; }\n this.isCompleted = false;\n this.isCompletedBefore = false;\n this.isLoading = false;\n this.completedByTriggers = undefined;\n if (clearData) {\n this.setDataCore(null, true);\n }\n this.timerModel.spent = 0;\n for (var i = 0; i < this.pages.length; i++) {\n this.pages[i].timeSpent = 0;\n this.pages[i].setWasShown(false);\n this.pages[i].passed = false;\n }\n this.onFirstPageIsStartedChanged();\n if (goToFirstPage) {\n this.currentPage = this.firstVisiblePage;\n }\n if (clearData) {\n this.updateValuesWithDefaults();\n }\n };\n SurveyModel.prototype.mergeValues = function (src, dest) {\n Object(_utils_utils__WEBPACK_IMPORTED_MODULE_15__[\"mergeValues\"])(src, dest);\n };\n SurveyModel.prototype.updateValuesWithDefaults = function () {\n if (this.isDesignMode || this.isLoading)\n return;\n for (var i = 0; i < this.pages.length; i++) {\n var questions = this.pages[i].questions;\n for (var j = 0; j < questions.length; j++) {\n questions[j].updateValueWithDefaults();\n }\n }\n };\n SurveyModel.prototype.updateCustomWidgets = function (page) {\n if (!page)\n return;\n page.updateCustomWidgets();\n };\n SurveyModel.prototype.currentPageChanging = function (newValue, oldValue) {\n var options = this.createPageChangeEventOptions(newValue, oldValue);\n options.allow = true;\n options.allowChanging = true;\n this.onCurrentPageChanging.fire(this, options);\n var allow = options.allowChanging && options.allow;\n if (allow) {\n this.isCurrentPageRendering = true;\n }\n return allow;\n };\n SurveyModel.prototype.currentPageChanged = function (newValue, oldValue) {\n this.notifyQuestionsOnHidingContent(oldValue);\n var options = this.createPageChangeEventOptions(newValue, oldValue);\n if (oldValue && !oldValue.passed) {\n if (oldValue.validate(false)) {\n oldValue.passed = true;\n }\n }\n this.onCurrentPageChanged.fire(this, options);\n };\n SurveyModel.prototype.notifyQuestionsOnHidingContent = function (page) {\n if (!page)\n return;\n page.questions.forEach(function (q) { return q.onHidingContent(); });\n };\n SurveyModel.prototype.createPageChangeEventOptions = function (newValue, oldValue) {\n var diff = !!newValue && !!oldValue ? newValue.visibleIndex - oldValue.visibleIndex : 0;\n return {\n oldCurrentPage: oldValue,\n newCurrentPage: newValue,\n isNextPage: diff === 1,\n isPrevPage: diff === -1,\n isGoingForward: diff > 0,\n isGoingBackward: diff < 0,\n isAfterPreview: this.changeCurrentPageFromPreview === true\n };\n };\n SurveyModel.prototype.getProgress = function () {\n if (this.currentPage == null)\n return 0;\n if (this.progressBarType !== \"pages\") {\n var info = this.getProgressInfo();\n if (this.progressBarType === \"requiredQuestions\") {\n return info.requiredQuestionCount >= 1\n ? Math.ceil((info.requiredAnsweredQuestionCount * 100) /\n info.requiredQuestionCount)\n : 100;\n }\n return info.questionCount >= 1\n ? Math.ceil((info.answeredQuestionCount * 100) / info.questionCount)\n : 100;\n }\n var visPages = this.visiblePages;\n var index = visPages.indexOf(this.currentPage);\n return Math.ceil((index * 100) / visPages.length);\n };\n Object.defineProperty(SurveyModel.prototype, \"progressValue\", {\n /**\n * Returns a percentage value that indicates user progress in the survey.\n * @see showProgressBar\n * @see progressBarType\n * @see progressText\n */\n get: function () {\n return this.getPropertyValue(\"progressValue\", 0);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isNavigationButtonsShowing\", {\n get: function () {\n if (this.isDesignMode)\n return \"none\";\n var page = this.currentPage;\n if (!page)\n return \"none\";\n if (page.navigationButtonsVisibility === \"show\") {\n return this.showNavigationButtons === \"none\" ? \"bottom\" : this.showNavigationButtons;\n }\n if (page.navigationButtonsVisibility === \"hide\") {\n return \"none\";\n }\n return this.showNavigationButtons;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isNavigationButtonsShowingOnTop\", {\n get: function () {\n return this.getIsNavigationButtonsShowingOn(\"top\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isNavigationButtonsShowingOnBottom\", {\n get: function () {\n return this.getIsNavigationButtonsShowingOn(\"bottom\");\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.getIsNavigationButtonsShowingOn = function (buttonPosition) {\n var res = this.isNavigationButtonsShowing;\n return res == \"both\" || res == buttonPosition;\n };\n Object.defineProperty(SurveyModel.prototype, \"isEditMode\", {\n get: function () {\n return this.mode == \"edit\";\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isDisplayMode\", {\n get: function () {\n return this.mode == \"display\" && !this.isDesignMode || this.state == \"preview\";\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isUpdateValueTextOnTyping\", {\n get: function () {\n return this.textUpdateMode == \"onTyping\";\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isDesignMode\", {\n /**\n * Indicates whether the survey is being designed in [Survey Creator](https://surveyjs.io/survey-creator/documentation/overview).\n */\n get: function () {\n return this._isDesignMode;\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.setDesignMode = function (value) {\n if (!!this._isDesignMode != !!value) {\n this._isDesignMode = !!value;\n this.onQuestionsOnPageModeChanged(\"standard\");\n }\n };\n Object.defineProperty(SurveyModel.prototype, \"showInvisibleElements\", {\n /**\n * Specifies whether to show all survey elements, regardless of their visibility.\n *\n * Default value: `false`\n */\n get: function () {\n return this.getPropertyValue(\"showInvisibleElements\", false);\n },\n set: function (val) {\n var visPages = this.visiblePages;\n this.setPropertyValue(\"showInvisibleElements\", val);\n if (this.isLoadingFromJson)\n return;\n this.runConditions();\n this.updateAllElementsVisibility(visPages);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.updateAllElementsVisibility = function (visPages) {\n for (var i = 0; i < this.pages.length; i++) {\n var page = this.pages[i];\n page.updateElementVisibility();\n if (visPages.indexOf(page) > -1 != page.isVisible) {\n this.onPageVisibleChanged.fire(this, {\n page: page,\n visible: page.isVisible,\n });\n }\n }\n };\n Object.defineProperty(SurveyModel.prototype, \"areInvisibleElementsShowing\", {\n get: function () {\n return this.isDesignMode || this.showInvisibleElements;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"areEmptyElementsHidden\", {\n get: function () {\n return (this.isShowingPreview &&\n this.showPreviewBeforeComplete == \"showAnsweredQuestions\" && this.isAnyQuestionAnswered);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isAnyQuestionAnswered\", {\n get: function () {\n var questions = this.getAllQuestions(true);\n for (var i = 0; i < questions.length; i++) {\n if (!questions[i].isEmpty())\n return true;\n }\n return false;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"hasCookie\", {\n /**\n * Indicates whether the browser has a cookie with a specified [`cookieName`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#cookieName). If this property's value is `true`, the respondent has passed the survey previously.\n * @see setCookie\n * @see deleteCookie\n */\n get: function () {\n if (!this.cookieName)\n return false;\n var cookies = _global_variables_utils__WEBPACK_IMPORTED_MODULE_25__[\"DomDocumentHelper\"].getCookie();\n return cookies && cookies.indexOf(this.cookieName + \"=true\") > -1;\n },\n enumerable: false,\n configurable: true\n });\n /**\n * Sets a cookie with a specified [`cookieName`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#cookieName) in the browser. If the `cookieName` property value is defined, this method is automatically called on survey completion.\n * @see hasCookie\n * @see deleteCookie\n */\n SurveyModel.prototype.setCookie = function () {\n if (!this.cookieName)\n return;\n _global_variables_utils__WEBPACK_IMPORTED_MODULE_25__[\"DomDocumentHelper\"].setCookie(this.cookieName + \"=true; expires=Fri, 31 Dec 9999 0:0:0 GMT\");\n };\n /**\n * Deletes a cookie with a specified [`cookieName`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#cookieName) from the browser.\n * @see hasCookie\n * @see setCookie\n */\n SurveyModel.prototype.deleteCookie = function () {\n if (!this.cookieName)\n return;\n _global_variables_utils__WEBPACK_IMPORTED_MODULE_25__[\"DomDocumentHelper\"].setCookie(this.cookieName + \"=;\");\n };\n Object.defineProperty(SurveyModel.prototype, \"ignoreValidation\", {\n /**\n * This property is obsolete. Use the [`validationEnabled`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#validationEnabled) property instead.\n */\n get: function () { return !this.validationEnabled; },\n set: function (val) { this.validationEnabled = !val; },\n enumerable: false,\n configurable: true\n });\n /**\n * Switches the survey to the next page.\n *\n * This method returns a Boolean value that indicates whether the page was successfully switched. `false` is returned if the current page is the last page or if it contains validation errors.\n * @returns `true` if the page was successfully switched; `false` otherwise.\n * @see isLastPage\n * @see prevPage\n * @see completeLastPage\n */\n SurveyModel.prototype.nextPage = function () {\n if (this.isLastPage)\n return false;\n return this.doCurrentPageComplete(false);\n };\n SurveyModel.prototype.hasErrorsOnNavigate = function (doComplete) {\n var _this = this;\n if (!this.isEditMode || this.ignoreValidation)\n return false;\n var skipValidation = doComplete && this.validationAllowComplete || !doComplete && this.validationAllowSwitchPages;\n var func = function (hasErrors) {\n if (!hasErrors || skipValidation) {\n _this.doCurrentPageCompleteCore(doComplete);\n }\n };\n if (this.isValidateOnComplete) {\n if (!this.isLastPage)\n return false;\n return this.validate(true, true, func) !== true && !skipValidation;\n }\n return this.validateCurrentPage(func) !== true && !skipValidation;\n };\n SurveyModel.prototype.checkForAsyncQuestionValidation = function (questions, func) {\n var _this = this;\n this.clearAsyncValidationQuesitons();\n var _loop_2 = function () {\n if (questions[i].isRunningValidators) {\n var q_1 = questions[i];\n q_1.onCompletedAsyncValidators = function (hasErrors) {\n _this.onCompletedAsyncQuestionValidators(q_1, func, hasErrors);\n };\n this_2.asyncValidationQuesitons.push(questions[i]);\n }\n };\n var this_2 = this;\n for (var i = 0; i < questions.length; i++) {\n _loop_2();\n }\n return this.asyncValidationQuesitons.length > 0;\n };\n SurveyModel.prototype.clearAsyncValidationQuesitons = function () {\n if (!!this.asyncValidationQuesitons) {\n var asynQuestions = this.asyncValidationQuesitons;\n for (var i = 0; i < asynQuestions.length; i++) {\n asynQuestions[i].onCompletedAsyncValidators = null;\n }\n }\n this.asyncValidationQuesitons = [];\n };\n SurveyModel.prototype.onCompletedAsyncQuestionValidators = function (question, func, hasErrors) {\n if (hasErrors) {\n this.clearAsyncValidationQuesitons();\n func(true);\n if (this.focusOnFirstError && !!question && !!question.page && question.page === this.currentPage) {\n var questions = this.currentPage.questions;\n for (var i_1 = 0; i_1 < questions.length; i_1++) {\n if (questions[i_1] !== question && questions[i_1].errors.length > 0)\n return;\n }\n question.focus(true);\n }\n return;\n }\n var asynQuestions = this.asyncValidationQuesitons;\n for (var i = 0; i < asynQuestions.length; i++) {\n if (asynQuestions[i].isRunningValidators)\n return;\n }\n func(false);\n };\n Object.defineProperty(SurveyModel.prototype, \"isCurrentPageHasErrors\", {\n get: function () {\n return this.checkIsCurrentPageHasErrors();\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isCurrentPageValid\", {\n /**\n * Returns `true` if the current page does not contain errors.\n * @see currentPage\n */\n get: function () {\n return !this.checkIsCurrentPageHasErrors();\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.hasCurrentPageErrors = function (onAsyncValidation) {\n return this.hasPageErrors(undefined, onAsyncValidation);\n };\n /**\n * Validates all questions on the current page and returns `false` if the validation fails.\n *\n * If you use validation expressions and at least one of them calls an async function, the `validateCurrentPage` method returns `undefined`. In this case, you should pass a callback function as the `onAsyncValidation` parameter. The function's `hasErrors` Boolean parameter will contain the validation result.\n * @param onAsyncValidation *(Optional)* Pass a callback function. It accepts a Boolean `hasErrors` parameter that equals `true` if the validation fails or `false` otherwise.\n * @see currentPage\n * @see validate\n * @see validateCurrentPage\n */\n SurveyModel.prototype.validateCurrentPage = function (onAsyncValidation) {\n return this.validatePage(undefined, onAsyncValidation);\n };\n SurveyModel.prototype.hasPageErrors = function (page, onAsyncValidation) {\n var res = this.validatePage(page, onAsyncValidation);\n if (res === undefined)\n return res;\n return !res;\n };\n /**\n * Validates all questions on a specified page and returns `false` if the validation fails.\n *\n * If you use validation expressions and at least one of them calls an async function, the `validatePage` method returns `undefined`. In this case, you should pass a callback function as the `onAsyncValidation` parameter. The function's `hasErrors` Boolean parameter will contain the validation result.\n * @param page Pass the `PageModel` that you want to validate. You can pass `undefined` to validate the [`activePage`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#activePage).\n * @param onAsyncValidation *(Optional)* Pass a callback function. It accepts a Boolean `hasErrors` parameter that equals `true` if the validation fails or `false` otherwise.\n * @see validate\n * @see validateCurrentPage\n */\n SurveyModel.prototype.validatePage = function (page, onAsyncValidation) {\n if (!page) {\n page = this.activePage;\n }\n if (!page)\n return true;\n if (this.checkIsPageHasErrors(page))\n return false;\n if (!onAsyncValidation)\n return true;\n return this.checkForAsyncQuestionValidation(page.questions, function (hasErrors) { return onAsyncValidation(hasErrors); })\n ? undefined\n : true;\n };\n SurveyModel.prototype.hasErrors = function (fireCallback, focusOnFirstError, onAsyncValidation) {\n if (fireCallback === void 0) { fireCallback = true; }\n if (focusOnFirstError === void 0) { focusOnFirstError = false; }\n var res = this.validate(fireCallback, focusOnFirstError, onAsyncValidation);\n if (res === undefined)\n return res;\n return !res;\n };\n /**\n * Validates all questions and returns `false` if the validation fails.\n *\n * If you use validation expressions and at least one of them calls an async function, the `validate` method returns `undefined`. In this case, you should pass a callback function as the `onAsyncValidation` parameter. The function's `hasErrors` Boolean parameter will contain the validation result.\n * @param fireCallback *(Optional)* Pass `false` if you do not want to show validation errors in the UI.\n * @param focusOnFirstError *(Optional)* Pass `true` if you want to focus the first question with a validation error. The survey will be switched to the page that contains this question if required.\n * @param onAsyncValidation *(Optional)* Pass a callback function. It accepts a Boolean `hasErrors` parameter that equals `true` if the validation fails or `false` otherwise.\n * @see validateCurrentPage\n * @see validatePage\n */\n SurveyModel.prototype.validate = function (fireCallback, focusOnFirstError, onAsyncValidation) {\n if (fireCallback === void 0) { fireCallback = true; }\n if (focusOnFirstError === void 0) { focusOnFirstError = false; }\n if (!!onAsyncValidation) {\n fireCallback = true;\n }\n var visPages = this.visiblePages;\n var firstErrorPage = null;\n var res = true;\n var rec = { fireCallback: fireCallback, focuseOnFirstError: focusOnFirstError, firstErrorQuestion: null, result: false };\n for (var i = 0; i < visPages.length; i++) {\n if (!visPages[i].validate(fireCallback, focusOnFirstError, rec)) {\n if (!firstErrorPage)\n firstErrorPage = visPages[i];\n res = false;\n }\n }\n if (focusOnFirstError && !!firstErrorPage && !!rec.firstErrorQuestion) {\n rec.firstErrorQuestion.focus(true);\n }\n if (!res || !onAsyncValidation)\n return res;\n return this.checkForAsyncQuestionValidation(this.getAllQuestions(), function (hasErrors) { return onAsyncValidation(hasErrors); })\n ? undefined\n : true;\n };\n SurveyModel.prototype.ensureUniqueNames = function (element) {\n if (element === void 0) { element = null; }\n if (element == null) {\n for (var i = 0; i < this.pages.length; i++) {\n this.ensureUniqueName(this.pages[i]);\n }\n }\n else {\n this.ensureUniqueName(element);\n }\n };\n SurveyModel.prototype.ensureUniqueName = function (element) {\n if (element.isPage) {\n this.ensureUniquePageName(element);\n }\n if (element.isPanel) {\n this.ensureUniquePanelName(element);\n }\n if (element.isPage || element.isPanel) {\n var elements = element.elements;\n for (var i = 0; i < elements.length; i++) {\n this.ensureUniqueNames(elements[i]);\n }\n }\n else {\n this.ensureUniqueQuestionName(element);\n }\n };\n SurveyModel.prototype.ensureUniquePageName = function (element) {\n var _this = this;\n return this.ensureUniqueElementName(element, function (name) {\n return _this.getPageByName(name);\n });\n };\n SurveyModel.prototype.ensureUniquePanelName = function (element) {\n var _this = this;\n return this.ensureUniqueElementName(element, function (name) {\n return _this.getPanelByName(name);\n });\n };\n SurveyModel.prototype.ensureUniqueQuestionName = function (element) {\n var _this = this;\n return this.ensureUniqueElementName(element, function (name) {\n return _this.getQuestionByName(name);\n });\n };\n SurveyModel.prototype.ensureUniqueElementName = function (element, getElementByName) {\n var existingElement = getElementByName(element.name);\n if (!existingElement || existingElement == element)\n return;\n var newName = this.getNewName(element.name);\n while (!!getElementByName(newName)) {\n var newName = this.getNewName(element.name);\n }\n element.name = newName;\n };\n SurveyModel.prototype.getNewName = function (name) {\n var pos = name.length;\n while (pos > 0 && name[pos - 1] >= \"0\" && name[pos - 1] <= \"9\") {\n pos--;\n }\n var base = name.substring(0, pos);\n var num = 0;\n if (pos < name.length) {\n num = parseInt(name.substring(pos));\n }\n num++;\n return base + num;\n };\n SurveyModel.prototype.checkIsCurrentPageHasErrors = function (isFocuseOnFirstError) {\n if (isFocuseOnFirstError === void 0) { isFocuseOnFirstError = undefined; }\n return this.checkIsPageHasErrors(this.activePage, isFocuseOnFirstError);\n };\n SurveyModel.prototype.checkIsPageHasErrors = function (page, isFocuseOnFirstError) {\n if (isFocuseOnFirstError === void 0) { isFocuseOnFirstError = undefined; }\n if (isFocuseOnFirstError === undefined) {\n isFocuseOnFirstError = this.focusOnFirstError;\n }\n if (!page)\n return true;\n var res = !page.validate(true, isFocuseOnFirstError);\n this.fireValidatedErrorsOnPage(page);\n return res;\n };\n SurveyModel.prototype.fireValidatedErrorsOnPage = function (page) {\n if (this.onValidatedErrorsOnCurrentPage.isEmpty || !page)\n return;\n var questionsOnPage = page.questions;\n var questions = new Array();\n var errors = new Array();\n for (var i = 0; i < questionsOnPage.length; i++) {\n var q = questionsOnPage[i];\n if (q.errors.length > 0) {\n questions.push(q);\n for (var j = 0; j < q.errors.length; j++) {\n errors.push(q.errors[j]);\n }\n }\n }\n this.onValidatedErrorsOnCurrentPage.fire(this, {\n questions: questions,\n errors: errors,\n page: page,\n });\n };\n /**\n * Switches the survey to the previous page.\n *\n * This method returns a Boolean value that indicates whether the page was successfully switched. `false` is returned if the current page is the first page.\n * @returns `true` if the page was successfully switched; `false` otherwise.\n * @see isFirstPage\n * @see nextPage\n */\n SurveyModel.prototype.prevPage = function () {\n var _this = this;\n if (this.isFirstPage || this.state === \"starting\")\n return false;\n this.resetNavigationButton();\n var skipped = this.skippedPages.find(function (sp) { return sp.to == _this.currentPage; });\n if (skipped) {\n this.currentPage = skipped.from;\n this.skippedPages.splice(this.skippedPages.indexOf(skipped), 1);\n }\n else {\n var vPages = this.visiblePages;\n var index = vPages.indexOf(this.currentPage);\n this.currentPage = vPages[index - 1];\n }\n return true;\n };\n /**\n * Completes the survey if it currently displays the last page and the page contains no validation errors. If both these conditions are met, this method returns `true`; otherwise, `false`.\n *\n * If you want to complete the survey regardless of the current page and validation errors, use the [`doComplete()`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#completeLastPage) event.\n * @see isCurrentPageValid\n * @see nextPage\n */\n SurveyModel.prototype.completeLastPage = function () {\n if (this.isValidateOnComplete) {\n this.cancelPreview();\n }\n var res = this.doCurrentPageComplete(true);\n if (res) {\n this.cancelPreview();\n }\n return res;\n };\n SurveyModel.prototype.navigationMouseDown = function () {\n this.isNavigationButtonPressed = true;\n return true;\n };\n SurveyModel.prototype.resetNavigationButton = function () {\n this.isNavigationButtonPressed = false;\n };\n SurveyModel.prototype.nextPageUIClick = function () {\n if (!!this.mouseDownPage && this.mouseDownPage !== this.activePage)\n return;\n this.mouseDownPage = null;\n return this.nextPage();\n };\n SurveyModel.prototype.nextPageMouseDown = function () {\n this.mouseDownPage = this.activePage;\n return this.navigationMouseDown();\n };\n /**\n * Displays a [preview of given answers](https://surveyjs.io/form-library/documentation/design-survey/create-a-multi-page-survey#preview-page). Returns `false` if the preview cannot be displayed because of validation errors.\n * @see cancelPreview\n * @see showPreviewBeforeComplete\n * @see onShowingPreview\n * @see state\n */\n SurveyModel.prototype.showPreview = function () {\n this.resetNavigationButton();\n if (!this.isValidateOnComplete) {\n if (this.hasErrorsOnNavigate(true))\n return false;\n if (this.doServerValidation(true, true))\n return false;\n }\n this.showPreviewCore();\n return true;\n };\n SurveyModel.prototype.showPreviewCore = function () {\n var options = { allowShowPreview: true, allow: true };\n this.onShowingPreview.fire(this, options);\n this.isShowingPreview = options.allowShowPreview && options.allow;\n };\n /**\n * Cancels a [preview of given answers](https://surveyjs.io/form-library/documentation/design-survey/create-a-multi-page-survey#preview-page) and switches the survey to the page specified by the `currentPage` parameter.\n * @param currentPage A new current page. If you do not specify this parameter, the survey displays the last page.\n * @see showPreview\n * @see showPreviewBeforeComplete\n * @see state\n */\n SurveyModel.prototype.cancelPreview = function (currentPage) {\n if (currentPage === void 0) { currentPage = null; }\n if (!this.isShowingPreview)\n return;\n this.gotoPageFromPreview = currentPage;\n this.isShowingPreview = false;\n };\n SurveyModel.prototype.cancelPreviewByPage = function (panel) {\n this.cancelPreview(panel[\"originalPage\"]);\n };\n SurveyModel.prototype.doCurrentPageComplete = function (doComplete) {\n if (this.isValidatingOnServer)\n return false;\n this.resetNavigationButton();\n if (this.hasErrorsOnNavigate(doComplete))\n return false;\n return this.doCurrentPageCompleteCore(doComplete);\n };\n SurveyModel.prototype.doCurrentPageCompleteCore = function (doComplete) {\n if (this.doServerValidation(doComplete))\n return false;\n if (doComplete) {\n this.currentPage.passed = true;\n return this.doComplete(this.canBeCompletedByTrigger, this.completedTrigger);\n }\n this.doNextPage();\n return true;\n };\n Object.defineProperty(SurveyModel.prototype, \"isSinglePage\", {\n get: function () {\n return this.questionsOnPageMode == \"singlePage\";\n },\n set: function (val) {\n this.questionsOnPageMode = val ? \"singlePage\" : \"standard\";\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"questionsOnPageMode\", {\n /**\n * Specifies how to distribute survey elements between pages.\n *\n * Possible values:\n *\n * - `\"singlePage\"` - Combines all survey pages into a single page.\n * - `\"questionPerPage\"` - Creates a separate page for every question.\n * - `\"standard\"` (default) - Retains the original structure specified in the JSON schema.\n */\n get: function () {\n return this.getPropertyValue(\"questionsOnPageMode\");\n },\n set: function (val) {\n this.setPropertyValue(\"questionsOnPageMode\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"firstPageIsStarted\", {\n /**\n * Gets or sets a Boolean value that specifies whether the first page is a start page.\n *\n * Refer to the following help topic for more information: [Start Page](https://surveyjs.io/form-library/documentation/design-survey/create-a-multi-page-survey#start-page).\n * @see startedPage\n * @see activePage\n */\n get: function () {\n return this.getPropertyValue(\"firstPageIsStarted\");\n },\n set: function (val) {\n this.setPropertyValue(\"firstPageIsStarted\", val);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.isPageStarted = function (page) {\n return (this.firstPageIsStarted && this.pages.length > 1 && this.pages[0] === page);\n };\n Object.defineProperty(SurveyModel.prototype, \"showPreviewBeforeComplete\", {\n /**\n * Allows respondents to preview answers before they are submitted.\n *\n * Possible values:\n *\n * - `\"showAllQuestions\"` - Displays all questions in the preview.\n * - `\"showAnsweredQuestions\"` - Displays only answered questions in the preview.\n * - `\"noPreview\"` (default) - Hides the preview.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/survey-showpreview/ (linkStyle))\n * @see showPreview\n * @see cancelPreview\n */\n get: function () {\n return this.getPropertyValue(\"showPreviewBeforeComplete\");\n },\n set: function (val) {\n this.setPropertyValue(\"showPreviewBeforeComplete\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isShowPreviewBeforeComplete\", {\n get: function () {\n var preview = this.showPreviewBeforeComplete;\n return preview == \"showAllQuestions\" || preview == \"showAnsweredQuestions\";\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.onFirstPageIsStartedChanged = function () {\n this.isStartedState = this.firstPageIsStarted && this.pages.length > 1;\n this.pageVisibilityChanged(this.pages[0], !this.isStartedState);\n };\n SurveyModel.prototype.onShowingPreviewChanged = function () {\n if (this.isDesignMode)\n return;\n if (this.isShowingPreview) {\n this.runningPages = this.pages.slice(0, this.pages.length);\n this.setupPagesForPageModes(true, false);\n }\n else {\n if (this.runningPages) {\n this.restoreOriginalPages(this.runningPages);\n }\n this.runningPages = undefined;\n }\n this.runConditions();\n this.updateAllElementsVisibility(this.pages);\n this.updateVisibleIndexes();\n if (this.isShowingPreview) {\n this.currentPageNo = 0;\n }\n else {\n var curPage = this.gotoPageFromPreview;\n this.gotoPageFromPreview = null;\n if (_helpers__WEBPACK_IMPORTED_MODULE_0__[\"Helpers\"].isValueEmpty(curPage) && this.visiblePageCount > 0) {\n curPage = this.visiblePages[this.visiblePageCount - 1];\n }\n if (!!curPage) {\n this.changeCurrentPageFromPreview = true;\n this.currentPage = curPage;\n this.changeCurrentPageFromPreview = false;\n }\n }\n };\n SurveyModel.prototype.onQuestionsOnPageModeChanged = function (oldValue, isFirstLoad) {\n if (isFirstLoad === void 0) { isFirstLoad = false; }\n if (this.isShowingPreview)\n return;\n if (this.questionsOnPageMode == \"standard\" || this.isDesignMode) {\n if (this.originalPages) {\n this.restoreOriginalPages(this.originalPages);\n }\n this.originalPages = undefined;\n }\n else {\n if (!oldValue || oldValue == \"standard\") {\n this.originalPages = this.pages.slice(0, this.pages.length);\n }\n this.setupPagesForPageModes(this.isSinglePage, isFirstLoad);\n }\n this.runConditions();\n this.updateVisibleIndexes();\n };\n SurveyModel.prototype.restoreOriginalPages = function (originalPages) {\n this.questionHashesClear();\n this.pages.splice(0, this.pages.length);\n for (var i = 0; i < originalPages.length; i++) {\n var page = originalPages[i];\n page.setWasShown(false);\n this.pages.push(page);\n }\n };\n SurveyModel.prototype.getPageStartIndex = function () {\n return this.firstPageIsStarted && this.pages.length > 0 ? 1 : 0;\n };\n SurveyModel.prototype.setupPagesForPageModes = function (isSinglePage, isFirstLoad) {\n this.questionHashesClear();\n this.isLockingUpdateOnPageModes = !isFirstLoad;\n var startIndex = this.getPageStartIndex();\n _super.prototype.startLoadingFromJson.call(this);\n var newPages = this.createPagesForQuestionOnPageMode(isSinglePage, startIndex);\n var deletedLen = this.pages.length - startIndex;\n this.pages.splice(startIndex, deletedLen);\n for (var i = 0; i < newPages.length; i++) {\n this.pages.push(newPages[i]);\n }\n _super.prototype.endLoadingFromJson.call(this);\n for (var i = 0; i < newPages.length; i++) {\n newPages[i].setSurveyImpl(this, true);\n }\n this.doElementsOnLoad();\n this.updateCurrentPage();\n this.isLockingUpdateOnPageModes = false;\n };\n SurveyModel.prototype.createPagesForQuestionOnPageMode = function (isSinglePage, startIndex) {\n if (isSinglePage) {\n return [this.createSinglePage(startIndex)];\n }\n return this.createPagesForEveryQuestion(startIndex);\n };\n SurveyModel.prototype.createSinglePage = function (startIndex) {\n var single = this.createNewPage(\"all\");\n single.setSurveyImpl(this);\n for (var i = startIndex; i < this.pages.length; i++) {\n var page = this.pages[i];\n var panel = _jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"Serializer\"].createClass(\"panel\");\n panel.originalPage = page;\n single.addPanel(panel);\n var json = new _jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"JsonObject\"]().toJsonObject(page);\n new _jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"JsonObject\"]().toObject(json, panel);\n if (!this.showPageTitles) {\n panel.title = \"\";\n }\n }\n return single;\n };\n SurveyModel.prototype.createPagesForEveryQuestion = function (startIndex) {\n var res = [];\n for (var i = startIndex; i < this.pages.length; i++) {\n var originalPage = this.pages[i];\n // Initialize randomization\n originalPage.setWasShown(true);\n for (var j = 0; j < originalPage.elements.length; j++) {\n var originalElement = originalPage.elements[j];\n var element = _jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"Serializer\"].createClass(originalElement.getType());\n if (!element)\n continue;\n var jsonObj = new _jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"JsonObject\"]();\n //Deserialize page properties only, excluding elements\n jsonObj.lightSerializing = true;\n var pageJson = jsonObj.toJsonObject(originalPage);\n var page = _jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"Serializer\"].createClass(originalPage.getType());\n page.fromJSON(pageJson);\n page.name = originalElement.name;\n page.setSurveyImpl(this);\n res.push(page);\n var json = new _jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"JsonObject\"]().toJsonObject(originalElement);\n page.addElement(element);\n new _jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"JsonObject\"]().toObject(json, element);\n for (var k = 0; k < page.questions.length; k++) {\n this.questionHashesAdded(page.questions[k]);\n }\n }\n }\n return res;\n };\n Object.defineProperty(SurveyModel.prototype, \"isFirstPage\", {\n /**\n * Indicates whether the [current page](#currentPage) is the first page.\n *\n * > If the survey displays the [start page](https://surveyjs.io/form-library/documentation/design-survey/create-a-multi-page-survey#start-page), this property contains `false`. Use the [`isShowStartingPage`](#isShowStartingPage) property to find out whether the start page is currently displayed.\n */\n get: function () {\n return this.getPropertyValue(\"isFirstPage\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isLastPage\", {\n /**\n * Indicates whether the [current page](#currentPage) is the last page.\n */\n get: function () {\n return this.getPropertyValue(\"isLastPage\");\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.updateButtonsVisibility = function () {\n this.updateIsFirstLastPageState();\n this.setPropertyValue(\"isShowPrevButton\", this.calcIsShowPrevButton());\n this.setPropertyValue(\"isShowNextButton\", this.calcIsShowNextButton());\n this.setPropertyValue(\"isCompleteButtonVisible\", this.calcIsCompleteButtonVisible());\n this.setPropertyValue(\"isPreviewButtonVisible\", this.calcIsPreviewButtonVisible());\n this.setPropertyValue(\"isCancelPreviewButtonVisible\", this.calcIsCancelPreviewButtonVisible());\n };\n Object.defineProperty(SurveyModel.prototype, \"isShowPrevButton\", {\n get: function () {\n return this.getPropertyValue(\"isShowPrevButton\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isShowNextButton\", {\n get: function () {\n return this.getPropertyValue(\"isShowNextButton\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isCompleteButtonVisible\", {\n get: function () {\n return this.getPropertyValue(\"isCompleteButtonVisible\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isPreviewButtonVisible\", {\n get: function () {\n return this.getPropertyValue(\"isPreviewButtonVisible\");\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isCancelPreviewButtonVisible\", {\n get: function () {\n return this.getPropertyValue(\"isCancelPreviewButtonVisible\");\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.updateIsFirstLastPageState = function () {\n var curPage = this.currentPage;\n this.setPropertyValue(\"isFirstPage\", !!curPage && curPage === this.firstVisiblePage);\n this.setPropertyValue(\"isLastPage\", !!curPage && curPage === this.lastVisiblePage);\n };\n SurveyModel.prototype.calcIsShowPrevButton = function () {\n if (this.isFirstPage || !this.showPrevButton || this.state !== \"running\")\n return false;\n var page = this.visiblePages[this.currentPageNo - 1];\n return this.getPageMaxTimeToFinish(page) <= 0;\n };\n SurveyModel.prototype.calcIsShowNextButton = function () {\n return this.state === \"running\" && !this.isLastPage && !this.canBeCompletedByTrigger;\n };\n SurveyModel.prototype.calcIsCompleteButtonVisible = function () {\n var state = this.state;\n return this.isEditMode && (this.state === \"running\" &&\n (this.isLastPage && !this.isShowPreviewBeforeComplete || this.canBeCompletedByTrigger)\n || state === \"preview\") && this.showCompleteButton;\n };\n SurveyModel.prototype.calcIsPreviewButtonVisible = function () {\n return (this.isEditMode &&\n this.isShowPreviewBeforeComplete &&\n this.state == \"running\" && this.isLastPage);\n };\n SurveyModel.prototype.calcIsCancelPreviewButtonVisible = function () {\n return (this.isEditMode &&\n this.isShowPreviewBeforeComplete &&\n this.state == \"preview\");\n };\n Object.defineProperty(SurveyModel.prototype, \"firstVisiblePage\", {\n get: function () {\n var pages = this.pages;\n for (var i = 0; i < pages.length; i++) {\n if (this.isPageInVisibleList(pages[i]))\n return pages[i];\n }\n return null;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"lastVisiblePage\", {\n get: function () {\n var pages = this.pages;\n for (var i = pages.length - 1; i >= 0; i--) {\n if (this.isPageInVisibleList(pages[i]))\n return pages[i];\n }\n return null;\n },\n enumerable: false,\n configurable: true\n });\n /**\n * Completes the survey.\n *\n * When you call this method, Form Library performs the following actions:\n *\n * 1. Saves a cookie if the [`cookieName`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#cookieName) property is set.\n * 1. Switches the survey [`state`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#state) to `\"completed\"`.\n * 1. Raises the [`onComplete`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#onComplete) event.\n * 1. Navigates the user to a URL specified by the [`navigateToUrl`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#navigateToUrl) or [`navigateToUrlOnCondition`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#navigateToUrlOnCondition) property.\n * 1. Calls the [`sendResult()`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#sendResult) method if Form Library works with [SurveyJS Service](https://api.surveyjs.io/).\n *\n * The `doComplete()` method completes the survey regardless of validation errors and the current page. If you need to ensure that survey results are valid and full, call the [`completeLastPage()`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#completeLastPage) method instead.\n *\n * @param isCompleteOnTrigger For internal use.\n * @param completeTrigger For internal use.\n * @returns `false` if survey completion is cancelled within the [`onCompleting`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#onCompleting) event handler; otherwise, `true`.\n * @see surveyPostId\n */\n SurveyModel.prototype.doComplete = function (isCompleteOnTrigger, completeTrigger) {\n if (isCompleteOnTrigger === void 0) { isCompleteOnTrigger = false; }\n if (this.isCompleted)\n return;\n if (!this.checkOnCompletingEvent(isCompleteOnTrigger, completeTrigger)) {\n this.isCompleted = false;\n return false;\n }\n this.checkOnPageTriggers(true);\n this.stopTimer();\n this.notifyQuestionsOnHidingContent(this.currentPage);\n this.isCompleted = true;\n this.clearUnusedValues();\n this.saveDataOnComplete(isCompleteOnTrigger, completeTrigger);\n this.setCookie();\n return true;\n };\n SurveyModel.prototype.saveDataOnComplete = function (isCompleteOnTrigger, completeTrigger) {\n var _this = this;\n if (isCompleteOnTrigger === void 0) { isCompleteOnTrigger = false; }\n var previousCookie = this.hasCookie;\n var showSaveInProgress = function (text) {\n savingDataStarted = true;\n _this.setCompletedState(\"saving\", text);\n };\n var showSaveError = function (text) {\n _this.setCompletedState(\"error\", text);\n };\n var showSaveSuccess = function (text) {\n _this.setCompletedState(\"success\", text);\n _this.navigateTo();\n };\n var clearSaveMessages = function (text) {\n _this.setCompletedState(\"\", \"\");\n };\n var savingDataStarted = false;\n var onCompleteOptions = {\n isCompleteOnTrigger: isCompleteOnTrigger,\n completeTrigger: completeTrigger,\n showSaveInProgress: showSaveInProgress,\n showSaveError: showSaveError,\n showSaveSuccess: showSaveSuccess,\n clearSaveMessages: clearSaveMessages,\n //Obsolete functions\n showDataSaving: showSaveInProgress,\n showDataSavingError: showSaveError,\n showDataSavingSuccess: showSaveSuccess,\n showDataSavingClear: clearSaveMessages\n };\n this.onComplete.fire(this, onCompleteOptions);\n if (!previousCookie && this.surveyPostId) {\n this.sendResult();\n }\n if (!savingDataStarted) {\n this.navigateTo();\n }\n };\n SurveyModel.prototype.checkOnCompletingEvent = function (isCompleteOnTrigger, completeTrigger) {\n var options = {\n allowComplete: true,\n allow: true,\n isCompleteOnTrigger: isCompleteOnTrigger,\n completeTrigger: completeTrigger\n };\n this.onCompleting.fire(this, options);\n return options.allowComplete && options.allow;\n };\n /**\n * Starts the survey. Applies only if the survey has a [start page](https://surveyjs.io/form-library/documentation/design-survey/create-a-multi-page-survey#start-page).\n * @see firstPageIsStarted\n * @see completeLastPage\n */\n SurveyModel.prototype.start = function () {\n if (!this.firstPageIsStarted)\n return false;\n this.isCurrentPageRendering = true;\n if (this.checkIsPageHasErrors(this.startedPage, true))\n return false;\n this.isStartedState = false;\n this.notifyQuestionsOnHidingContent(this.pages[0]);\n this.startTimerFromUI();\n this.onStarted.fire(this, {});\n this.updateVisibleIndexes();\n if (!!this.currentPage) {\n this.currentPage.locStrsChanged();\n }\n return true;\n };\n Object.defineProperty(SurveyModel.prototype, \"isValidatingOnServer\", {\n /**\n * Indicates whether the current page is being [validated on a server](#onServerValidateQuestions).\n */\n get: function () {\n return this.getPropertyValue(\"isValidatingOnServer\", false);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.setIsValidatingOnServer = function (val) {\n if (val == this.isValidatingOnServer)\n return;\n this.setPropertyValue(\"isValidatingOnServer\", val);\n this.onIsValidatingOnServerChanged();\n };\n SurveyModel.prototype.createServerValidationOptions = function (doComplete, isPreview) {\n var self = this;\n var options = {\n data: {},\n errors: {},\n survey: this,\n complete: function () {\n self.completeServerValidation(options, isPreview);\n },\n };\n if (doComplete && this.isValidateOnComplete) {\n options.data = this.data;\n }\n else {\n var questions = this.activePage.questions;\n for (var i = 0; i < questions.length; i++) {\n var question = questions[i];\n if (!question.visible)\n continue;\n var value = this.getValue(question.getValueName());\n if (!this.isValueEmpty(value))\n options.data[question.getValueName()] = value;\n }\n }\n return options;\n };\n SurveyModel.prototype.onIsValidatingOnServerChanged = function () { };\n SurveyModel.prototype.doServerValidation = function (doComplete, isPreview) {\n var _this = this;\n if (isPreview === void 0) { isPreview = false; }\n if (!this.onServerValidateQuestions ||\n this.onServerValidateQuestions.isEmpty)\n return false;\n if (!doComplete && this.isValidateOnComplete)\n return false;\n this.setIsValidatingOnServer(true);\n var isFunc = typeof this.onServerValidateQuestions === \"function\";\n this.serverValidationEventCount = !isFunc ? this.onServerValidateQuestions.length : 1;\n if (isFunc) {\n this.onServerValidateQuestions(this, this.createServerValidationOptions(doComplete, isPreview));\n }\n else {\n this.onServerValidateQuestions.fireByCreatingOptions(this, function () { return _this.createServerValidationOptions(doComplete, isPreview); });\n }\n return true;\n };\n SurveyModel.prototype.completeServerValidation = function (options, isPreview) {\n if (this.serverValidationEventCount > 1) {\n this.serverValidationEventCount--;\n if (!!options && !!options.errors && Object.keys(options.errors).length === 0)\n return;\n }\n this.serverValidationEventCount = 0;\n this.setIsValidatingOnServer(false);\n if (!options && !options.survey)\n return;\n var self = options.survey;\n var hasErrors = false;\n if (options.errors) {\n var hasToFocus = this.focusOnFirstError;\n for (var name in options.errors) {\n var question = self.getQuestionByName(name);\n if (question && question[\"errors\"]) {\n hasErrors = true;\n question.addError(new _error__WEBPACK_IMPORTED_MODULE_9__[\"CustomError\"](options.errors[name], this));\n if (hasToFocus) {\n hasToFocus = false;\n if (!!question.page) {\n this.currentPage = question.page;\n }\n question.focus(true);\n }\n }\n }\n this.fireValidatedErrorsOnPage(this.currentPage);\n }\n if (!hasErrors) {\n if (isPreview) {\n this.showPreviewCore();\n }\n else {\n if (self.isLastPage)\n self.doComplete();\n else\n self.doNextPage();\n }\n }\n };\n SurveyModel.prototype.doNextPage = function () {\n var curPage = this.currentPage;\n this.checkOnPageTriggers(false);\n if (!this.isCompleted) {\n if (this.sendResultOnPageNext) {\n this.sendResult(this.surveyPostId, this.clientId, true);\n }\n if (curPage === this.currentPage) {\n var vPages = this.visiblePages;\n var index = vPages.indexOf(this.currentPage);\n this.currentPage = vPages[index + 1];\n }\n }\n else {\n this.doComplete(true);\n }\n };\n SurveyModel.prototype.setCompleted = function (trigger) {\n this.doComplete(true, trigger);\n };\n SurveyModel.prototype.canBeCompleted = function (trigger, isCompleted) {\n var _a;\n if (!_settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].triggers.changeNavigationButtonsOnComplete)\n return;\n var prevCanBeCompleted = this.canBeCompletedByTrigger;\n if (!this.completedByTriggers)\n this.completedByTriggers = {};\n if (isCompleted) {\n this.completedByTriggers[trigger.id] = { trigger: trigger, pageId: (_a = this.currentPage) === null || _a === void 0 ? void 0 : _a.id };\n }\n else {\n delete this.completedByTriggers[trigger.id];\n }\n if (prevCanBeCompleted !== this.canBeCompletedByTrigger) {\n this.updateButtonsVisibility();\n }\n };\n Object.defineProperty(SurveyModel.prototype, \"canBeCompletedByTrigger\", {\n get: function () {\n var _a;\n if (!this.completedByTriggers)\n return false;\n var keys = Object.keys(this.completedByTriggers);\n if (keys.length === 0)\n return false;\n var id = (_a = this.currentPage) === null || _a === void 0 ? void 0 : _a.id;\n if (!id)\n return true;\n for (var i = 0; i < keys.length; i++) {\n if (id === this.completedByTriggers[keys[i]].pageId)\n return true;\n }\n return false;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"completedTrigger\", {\n get: function () {\n if (!this.canBeCompletedByTrigger)\n return undefined;\n var key = Object.keys(this.completedByTriggers)[0];\n return this.completedByTriggers[key].trigger;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"processedCompletedHtml\", {\n /**\n * Returns HTML content displayed on the [complete page](https://surveyjs.io/form-library/documentation/design-survey/create-a-multi-page-survey#complete-page).\n *\n * To specify HTML content, use the [`completedHtml`](#completedHtml) property.\n */\n get: function () {\n var html = this.renderedCompletedHtml;\n return !!html ? this.processHtml(html, \"completed\") : \"\";\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"processedCompletedBeforeHtml\", {\n /**\n * Returns HTML content displayed to a user who has completed the survey before. To identify such users, the survey uses a [cookie name](#cookieName) or [client ID](#clientId).\n *\n * To specify HTML content, use the [`completedBeforeHtml`](#completedBeforeHtml) property.\n */\n get: function () {\n return this.locCompletedBeforeHtml.textOrHtml;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"processedLoadingHtml\", {\n /**\n * Returns HTML content displayed while a survey JSON schema is being loaded from [SurveyJS Service](https://api.surveyjs.io).\n *\n * To specify HTML content, use the [`loadingHtml`](#loadingHtml) property.\n */\n get: function () {\n return this.locLoadingHtml.textOrHtml;\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.getProgressInfo = function () {\n var pages = this.isDesignMode ? this.pages : this.visiblePages;\n return _survey_element__WEBPACK_IMPORTED_MODULE_3__[\"SurveyElement\"].getProgressInfoByElements(pages, false);\n };\n Object.defineProperty(SurveyModel.prototype, \"progressText\", {\n /**\n * Returns text displayed by the progress bar (for instance, \"Page 2 of 3\" or \"Answered 3/8 questions\"). Handle the [`onProgressText`](#onProgressText) event to change this text.\n * @see progressValue\n * @see showProgressBar\n * @see progressBarType\n */\n get: function () {\n var res = this.getPropertyValue(\"progressText\", \"\");\n if (!res) {\n this.updateProgressText();\n res = this.getPropertyValue(\"progressText\", \"\");\n }\n return res;\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.updateProgressText = function (onValueChanged) {\n if (onValueChanged === void 0) { onValueChanged = false; }\n if (this.isCalculatingProgressText || this.isShowingPreview || this.isLockingUpdateOnPageModes)\n return;\n if (onValueChanged &&\n this.progressBarType == \"pages\" &&\n this.onProgressText.isEmpty)\n return;\n this.isCalculatingProgressText = true;\n this.setPropertyValue(\"progressText\", this.getProgressText());\n this.setPropertyValue(\"progressValue\", this.getProgress());\n this.isCalculatingProgressText = false;\n };\n SurveyModel.prototype.getProgressText = function () {\n if (!this.isDesignMode && this.currentPage == null)\n return \"\";\n var options = {\n questionCount: 0,\n answeredQuestionCount: 0,\n requiredQuestionCount: 0,\n requiredAnsweredQuestionCount: 0,\n text: \"\",\n };\n var type = this.progressBarType.toLowerCase();\n if (type === \"questions\" ||\n type === \"requiredquestions\" ||\n type === \"correctquestions\" ||\n !this.onProgressText.isEmpty) {\n var info = this.getProgressInfo();\n options.questionCount = info.questionCount;\n options.answeredQuestionCount = info.answeredQuestionCount;\n options.requiredQuestionCount = info.requiredQuestionCount;\n options.requiredAnsweredQuestionCount =\n info.requiredAnsweredQuestionCount;\n }\n options.text = this.getProgressTextCore(options);\n this.onProgressText.fire(this, options);\n return options.text;\n };\n SurveyModel.prototype.getProgressTextCore = function (info) {\n var type = this.progressBarType.toLowerCase();\n if (type === \"questions\") {\n return this.getLocalizationFormatString(\"questionsProgressText\", info.answeredQuestionCount, info.questionCount);\n }\n if (type === \"requiredquestions\") {\n return this.getLocalizationFormatString(\"questionsProgressText\", info.requiredAnsweredQuestionCount, info.requiredQuestionCount);\n }\n if (type === \"correctquestions\") {\n var correctAnswersCount = this.getCorrectedAnswerCount();\n return this.getLocalizationFormatString(\"questionsProgressText\", correctAnswersCount, info.questionCount);\n }\n var vPages = this.isDesignMode ? this.pages : this.visiblePages;\n var index = vPages.indexOf(this.currentPage) + 1;\n return this.getLocalizationFormatString(\"progressText\", index, vPages.length);\n };\n SurveyModel.prototype.getRootCss = function () {\n return new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_18__[\"CssClassBuilder\"]()\n .append(this.css.root)\n .append(this.css.rootMobile, this.isMobile)\n .append(this.css.rootAnimationDisabled, !_settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].animationEnabled)\n .append(this.css.rootReadOnly, this.mode === \"display\" && !this.isDesignMode)\n .append(this.css.rootCompact, this.isCompact)\n .append(this.css.rootFitToContainer, this.fitToContainer)\n .toString();\n };\n SurveyModel.prototype.afterRenderSurvey = function (htmlElement) {\n var _this = this;\n this.destroyResizeObserver();\n if (Array.isArray(htmlElement)) {\n htmlElement = _survey_element__WEBPACK_IMPORTED_MODULE_3__[\"SurveyElement\"].GetFirstNonTextElement(htmlElement);\n }\n var observedElement = htmlElement;\n var cssVariables = this.css.variables;\n if (!!cssVariables) {\n var mobileWidth_1 = Number.parseFloat(_global_variables_utils__WEBPACK_IMPORTED_MODULE_25__[\"DomDocumentHelper\"].getComputedStyle(observedElement).getPropertyValue(cssVariables.mobileWidth));\n if (!!mobileWidth_1) {\n var isProcessed_1 = false;\n this.resizeObserver = new ResizeObserver(function (entries) {\n _global_variables_utils__WEBPACK_IMPORTED_MODULE_25__[\"DomWindowHelper\"].requestAnimationFrame(function () {\n if (isProcessed_1 || !Object(_utils_utils__WEBPACK_IMPORTED_MODULE_15__[\"isContainerVisible\"])(observedElement)) {\n isProcessed_1 = false;\n }\n else {\n isProcessed_1 = _this.processResponsiveness(observedElement.offsetWidth, mobileWidth_1);\n }\n });\n });\n this.resizeObserver.observe(observedElement);\n }\n }\n this.onAfterRenderSurvey.fire(this, {\n survey: this,\n htmlElement: htmlElement,\n });\n this.rootElement = htmlElement;\n this.addScrollEventListener();\n };\n SurveyModel.prototype.processResponsiveness = function (width, mobileWidth) {\n var isMobile = width < mobileWidth;\n var isMobileChanged = this.isMobile !== isMobile;\n if (isMobileChanged) {\n this.setIsMobile(isMobile);\n }\n this.layoutElements.forEach(function (layoutElement) { return layoutElement.processResponsiveness && layoutElement.processResponsiveness(width); });\n return isMobileChanged;\n };\n SurveyModel.prototype.triggerResponsiveness = function (hard) {\n this.getAllQuestions().forEach(function (question) {\n question.triggerResponsiveness(hard);\n });\n };\n SurveyModel.prototype.destroyResizeObserver = function () {\n if (!!this.resizeObserver) {\n this.resizeObserver.disconnect();\n this.resizeObserver = undefined;\n }\n };\n SurveyModel.prototype.updateQuestionCssClasses = function (question, cssClasses) {\n this.onUpdateQuestionCssClasses.fire(this, {\n question: question,\n cssClasses: cssClasses,\n });\n };\n SurveyModel.prototype.updatePanelCssClasses = function (panel, cssClasses) {\n this.onUpdatePanelCssClasses.fire(this, {\n panel: panel,\n cssClasses: cssClasses,\n });\n };\n SurveyModel.prototype.updatePageCssClasses = function (page, cssClasses) {\n this.onUpdatePageCssClasses.fire(this, {\n page: page,\n cssClasses: cssClasses,\n });\n };\n SurveyModel.prototype.updateChoiceItemCss = function (question, options) {\n options.question = question;\n this.onUpdateChoiceItemCss.fire(this, options);\n };\n SurveyModel.prototype.afterRenderPage = function (htmlElement) {\n var _this = this;\n if (!this.isDesignMode && !this.focusingQuestionInfo) {\n var doScroll_1 = !this.isFirstPageRendering;\n setTimeout(function () { return _this.scrollToTopOnPageChange(doScroll_1); }, 1);\n }\n this.focusQuestionInfo();\n this.isFirstPageRendering = false;\n if (this.onAfterRenderPage.isEmpty)\n return;\n this.onAfterRenderPage.fire(this, {\n page: this.activePage,\n htmlElement: htmlElement,\n });\n };\n SurveyModel.prototype.afterRenderHeader = function (htmlElement) {\n if (this.onAfterRenderHeader.isEmpty)\n return;\n this.onAfterRenderHeader.fire(this, {\n htmlElement: htmlElement,\n });\n };\n SurveyModel.prototype.afterRenderQuestion = function (question, htmlElement) {\n this.onAfterRenderQuestion.fire(this, {\n question: question,\n htmlElement: htmlElement,\n });\n };\n SurveyModel.prototype.afterRenderQuestionInput = function (question, htmlElement) {\n if (this.onAfterRenderQuestionInput.isEmpty)\n return;\n var id = question.inputId;\n var root = _settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].environment.root;\n if (!!id && htmlElement.id !== id && typeof root !== \"undefined\") {\n var el = root.getElementById(id);\n if (!!el) {\n htmlElement = el;\n }\n }\n this.onAfterRenderQuestionInput.fire(this, {\n question: question,\n htmlElement: htmlElement,\n });\n };\n SurveyModel.prototype.afterRenderPanel = function (panel, htmlElement) {\n this.onAfterRenderPanel.fire(this, {\n panel: panel,\n htmlElement: htmlElement,\n });\n };\n SurveyModel.prototype.whenQuestionFocusIn = function (question) {\n this.onFocusInQuestion.fire(this, {\n question: question\n });\n };\n SurveyModel.prototype.whenPanelFocusIn = function (panel) {\n this.onFocusInPanel.fire(this, {\n panel: panel\n });\n };\n SurveyModel.prototype.rebuildQuestionChoices = function () {\n this.getAllQuestions().forEach(function (q) { return q.surveyChoiceItemVisibilityChange(); });\n };\n SurveyModel.prototype.canChangeChoiceItemsVisibility = function () {\n return !this.onShowingChoiceItem.isEmpty;\n };\n SurveyModel.prototype.getChoiceItemVisibility = function (question, item, val) {\n var options = { question: question, item: item, visible: val };\n this.onShowingChoiceItem.fire(this, options);\n return options.visible;\n };\n SurveyModel.prototype.loadQuestionChoices = function (options) {\n this.onChoicesLazyLoad.fire(this, options);\n };\n SurveyModel.prototype.getChoiceDisplayValue = function (options) {\n if (this.onGetChoiceDisplayValue.isEmpty) {\n options.setItems(null);\n }\n else {\n this.onGetChoiceDisplayValue.fire(this, options);\n }\n };\n SurveyModel.prototype.matrixBeforeRowAdded = function (options) {\n this.onMatrixRowAdding.fire(this, options);\n };\n SurveyModel.prototype.matrixRowAdded = function (question, row) {\n this.onMatrixRowAdded.fire(this, { question: question, row: row });\n };\n SurveyModel.prototype.matrixColumnAdded = function (question, column) {\n this.onMatrixColumnAdded.fire(this, { question: question, column: column });\n };\n SurveyModel.prototype.multipleTextItemAdded = function (question, item) {\n this.onMultipleTextItemAdded.fire(this, { question: question, item: item });\n };\n SurveyModel.prototype.getQuestionByValueNameFromArray = function (valueName, name, index) {\n var questions = this.getQuestionsByValueName(valueName);\n if (!questions)\n return;\n for (var i = 0; i < questions.length; i++) {\n var res = questions[i].getQuestionFromArray(name, index);\n if (!!res)\n return res;\n }\n return null;\n };\n SurveyModel.prototype.matrixRowRemoved = function (question, rowIndex, row) {\n this.onMatrixRowRemoved.fire(this, {\n question: question,\n rowIndex: rowIndex,\n row: row,\n });\n };\n SurveyModel.prototype.matrixRowRemoving = function (question, rowIndex, row) {\n var options = {\n question: question,\n rowIndex: rowIndex,\n row: row,\n allow: true,\n };\n this.onMatrixRowRemoving.fire(this, options);\n return options.allow;\n };\n SurveyModel.prototype.matrixAllowRemoveRow = function (question, rowIndex, row) {\n var options = { question: question, rowIndex: rowIndex, row: row, allow: true };\n this.onMatrixRenderRemoveButton.fire(this, options);\n return options.allow;\n };\n SurveyModel.prototype.matrixDetailPanelVisibleChanged = function (question, rowIndex, row, visible) {\n var options = { question: question, rowIndex: rowIndex, row: row, visible: visible, detailPanel: row.detailPanel };\n this.onMatrixDetailPanelVisibleChanged.fire(this, options);\n };\n SurveyModel.prototype.matrixCellCreating = function (question, options) {\n options.question = question;\n this.onMatrixCellCreating.fire(this, options);\n };\n SurveyModel.prototype.matrixCellCreated = function (question, options) {\n options.question = question;\n this.onMatrixCellCreated.fire(this, options);\n };\n SurveyModel.prototype.matrixAfterCellRender = function (question, options) {\n options.question = question;\n this.onAfterRenderMatrixCell.fire(this, options);\n };\n SurveyModel.prototype.matrixCellValueChanged = function (question, options) {\n options.question = question;\n this.onMatrixCellValueChanged.fire(this, options);\n };\n SurveyModel.prototype.matrixCellValueChanging = function (question, options) {\n options.question = question;\n this.onMatrixCellValueChanging.fire(this, options);\n };\n Object.defineProperty(SurveyModel.prototype, \"isValidateOnValueChanging\", {\n get: function () {\n return this.checkErrorsMode === \"onValueChanging\";\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isValidateOnValueChanged\", {\n get: function () {\n return this.checkErrorsMode === \"onValueChanged\";\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isValidateOnComplete\", {\n get: function () {\n return this.checkErrorsMode === \"onComplete\" || this.validationAllowSwitchPages && !this.validationAllowComplete;\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.matrixCellValidate = function (question, options) {\n options.question = question;\n this.onMatrixCellValidate.fire(this, options);\n return options.error ? new _error__WEBPACK_IMPORTED_MODULE_9__[\"CustomError\"](options.error, this) : null;\n };\n SurveyModel.prototype.dynamicPanelAdded = function (question, panelIndex, panel) {\n if (!this.isLoadingFromJson) {\n this.updateVisibleIndexes();\n }\n if (this.onDynamicPanelAdded.isEmpty)\n return;\n var panels = question.panels;\n if (panelIndex === undefined) {\n panelIndex = panels.length - 1;\n panel = panels[panelIndex];\n }\n this.onDynamicPanelAdded.fire(this, { question: question, panel: panel, panelIndex: panelIndex });\n };\n SurveyModel.prototype.dynamicPanelRemoved = function (question, panelIndex, panel) {\n var questions = !!panel ? panel.questions : [];\n for (var i = 0; i < questions.length; i++) {\n questions[i].clearOnDeletingContainer();\n }\n this.updateVisibleIndexes();\n this.onDynamicPanelRemoved.fire(this, {\n question: question,\n panelIndex: panelIndex,\n panel: panel,\n });\n };\n SurveyModel.prototype.dynamicPanelRemoving = function (question, panelIndex, panel) {\n var options = {\n question: question,\n panelIndex: panelIndex,\n panel: panel,\n allow: true\n };\n this.onDynamicPanelRemoving.fire(this, options);\n return options.allow;\n };\n SurveyModel.prototype.dynamicPanelItemValueChanged = function (question, options) {\n options.question = question;\n options.panelIndex = options.itemIndex;\n options.panelData = options.itemValue;\n this.onDynamicPanelItemValueChanged.fire(this, options);\n };\n SurveyModel.prototype.dynamicPanelGetTabTitle = function (question, options) {\n options.question = question;\n this.onGetDynamicPanelTabTitle.fire(this, options);\n };\n SurveyModel.prototype.dynamicPanelCurrentIndexChanged = function (question, options) {\n options.question = question;\n this.onDynamicPanelCurrentIndexChanged.fire(this, options);\n };\n SurveyModel.prototype.dragAndDropAllow = function (options) {\n this.onDragDropAllow.fire(this, options);\n return options.allow;\n };\n SurveyModel.prototype.elementContentVisibilityChanged = function (element) {\n if (this.currentPage) {\n this.currentPage.ensureRowsVisibility();\n }\n this.onElementContentVisibilityChanged.fire(this, { element: element });\n };\n SurveyModel.prototype.getUpdatedPanelFooterActions = function (panel, actions, question) {\n var options = {\n question: question,\n panel: panel,\n actions: actions,\n };\n this.onGetPanelFooterActions.fire(this, options);\n return options.actions;\n };\n SurveyModel.prototype.getUpdatedElementTitleActions = function (element, titleActions) {\n if (element.isPage)\n return this.getUpdatedPageTitleActions(element, titleActions);\n if (element.isPanel)\n return this.getUpdatedPanelTitleActions(element, titleActions);\n return this.getUpdatedQuestionTitleActions(element, titleActions);\n };\n SurveyModel.prototype.getUpdatedQuestionTitleActions = function (question, titleActions) {\n var options = {\n question: question,\n titleActions: titleActions,\n };\n this.onGetQuestionTitleActions.fire(this, options);\n return options.titleActions;\n };\n SurveyModel.prototype.getUpdatedPanelTitleActions = function (panel, titleActions) {\n var options = {\n panel: panel,\n titleActions: titleActions,\n };\n this.onGetPanelTitleActions.fire(this, options);\n return options.titleActions;\n };\n SurveyModel.prototype.getUpdatedPageTitleActions = function (page, titleActions) {\n var options = {\n page: page,\n titleActions: titleActions,\n };\n this.onGetPageTitleActions.fire(this, options);\n return options.titleActions;\n };\n SurveyModel.prototype.getUpdatedMatrixRowActions = function (question, row, actions) {\n var options = {\n question: question,\n actions: actions,\n row: row,\n };\n this.onGetMatrixRowActions.fire(this, options);\n return options.actions;\n };\n SurveyModel.prototype.scrollElementToTop = function (element, question, page, id, scrollIfVisible) {\n var options = {\n element: element,\n question: question,\n page: page,\n elementId: id,\n cancel: false,\n };\n this.onScrollingElementToTop.fire(this, options);\n if (!options.cancel) {\n _survey_element__WEBPACK_IMPORTED_MODULE_3__[\"SurveyElement\"].ScrollElementToTop(options.elementId, scrollIfVisible);\n }\n };\n /**\n * Opens a dialog window for users to select files.\n * @param input A [file input HTML element](https://developer.mozilla.org/en-US/docs/Web/API/HTMLInputElement).\n * @param callback A callback function that you can use to process selected files. Accepts an array of JavaScript File objects.\n * @see onOpenFileChooser\n * @see onUploadFile\n */\n SurveyModel.prototype.chooseFiles = function (input, callback, context) {\n if (this.onOpenFileChooser.isEmpty) {\n Object(_utils_utils__WEBPACK_IMPORTED_MODULE_15__[\"chooseFiles\"])(input, callback);\n }\n else {\n this.onOpenFileChooser.fire(this, {\n input: input,\n element: context && context.element || this.survey,\n elementType: context && context.elementType,\n item: context && context.item,\n propertyName: context && context.propertyName,\n callback: callback,\n context: context\n });\n }\n };\n /**\n * Uploads files to a server.\n *\n * The following code shows how to call this method:\n *\n * ```js\n * const question = survey.getQuestionByName(\"myFileQuestion\");\n * survey.uploadFiles(\n * question,\n * question.name,\n * question.value,\n * (data, errors) => {\n * // ...\n * }\n * );\n * ```\n * @param question A [File Upload question instance](https://surveyjs.io/form-library/documentation/api-reference/file-model) or [Signature Pad question instance](https://surveyjs.io/form-library/documentation/api-reference/signature-pad-model).\n * @param name The File Upload question's [`name`](https://surveyjs.io/form-library/documentation/api-reference/file-model#name) or Signature Pad question's [`name`](https://surveyjs.io/form-library/documentation/api-reference/signature-pad-model#name).\n * @param files An array of JavaScript File objects that represent files to upload.\n * @param callback A callback function that allows you to access successfully uploaded files as the first argument. If any files fail to upload, the second argument contains an array of error messages.\n * @see onUploadFiles\n * @see downloadFile\n */\n SurveyModel.prototype.uploadFiles = function (question, name, files, callback) {\n var _this = this;\n if (this.onUploadFiles.isEmpty) {\n callback(\"error\", this.getLocString(\"noUploadFilesHandler\"));\n }\n else {\n this.taskManager.runTask(\"file\", function (done) {\n _this.onUploadFiles.fire(_this, {\n question: question,\n name: name,\n files: files || [],\n callback: function (status, data) {\n callback(status, data);\n done();\n },\n });\n });\n }\n if (this.surveyPostId) {\n this.uploadFilesCore(name, files, callback);\n }\n };\n /**\n * Downloads a file from a server.\n *\n * The following code shows how to call this method:\n *\n * ```js\n * const question = survey.getQuestionByName(\"myFileQuestion\");\n * survey.downloadFile(\n * question,\n * question.name,\n * // Download the first uploaded file\n * question.value[0],\n * (status, data) => {\n * if (status === \"success\") {\n * // Use `data` to retrieve the file\n * }\n * if (status === \"error\") {\n * // Handle error\n * }\n * }\n * );\n * ```\n *\n * @param question A [File Upload question instance](https://surveyjs.io/form-library/documentation/api-reference/file-model).\n * @param questionName The File Upload question's [`name`](https://surveyjs.io/form-library/documentation/api-reference/file-model#name).\n * @param fileValue An object from File Upload's [`value`](https://surveyjs.io/form-library/documentation/api-reference/file-model#value) array. This object contains metadata about the file you want to download.\n * @param callback A callback function that allows you to get the download status (`\"success\"` or `\"error\"`) and the file identifier (URL, file name, etc.) that you can use to retrieve the file.\n * @see onDownloadFile\n * @see uploadFiles\n */\n SurveyModel.prototype.downloadFile = function (question, questionName, fileValue, callback) {\n if (this.onDownloadFile.isEmpty) {\n !!callback && callback(\"success\", fileValue.content || fileValue);\n }\n this.onDownloadFile.fire(this, {\n question: question,\n name: questionName,\n content: fileValue.content || fileValue,\n fileValue: fileValue,\n callback: callback,\n });\n };\n SurveyModel.prototype.clearFiles = function (question, name, value, fileName, callback) {\n if (this.onClearFiles.isEmpty) {\n !!callback && callback(\"success\", value);\n }\n this.onClearFiles.fire(this, {\n question: question,\n name: name,\n value: value,\n fileName: fileName,\n callback: callback,\n });\n };\n SurveyModel.prototype.updateChoicesFromServer = function (question, choices, serverResult) {\n var options = {\n question: question,\n choices: choices,\n serverResult: serverResult,\n };\n this.onLoadChoicesFromServer.fire(this, options);\n return options.choices;\n };\n SurveyModel.prototype.loadedChoicesFromServer = function (question) {\n this.locStrsChanged();\n };\n SurveyModel.prototype.createSurveyService = function () {\n return new _dxSurveyService__WEBPACK_IMPORTED_MODULE_7__[\"dxSurveyService\"]();\n };\n SurveyModel.prototype.uploadFilesCore = function (name, files, uploadingCallback) {\n var _this = this;\n var responses = [];\n files.forEach(function (file) {\n if (uploadingCallback)\n uploadingCallback(\"uploading\", file);\n _this.createSurveyService().sendFile(_this.surveyPostId, file, function (success, response) {\n if (success) {\n responses.push({ content: response, file: file });\n if (responses.length === files.length) {\n if (uploadingCallback)\n uploadingCallback(\"success\", responses);\n }\n }\n else {\n if (uploadingCallback)\n uploadingCallback(\"error\", {\n response: response,\n file: file,\n });\n }\n });\n });\n };\n SurveyModel.prototype.getPage = function (index) {\n return this.pages[index];\n };\n /**\n * Adds an existing page to the survey.\n * @param page A page to add.\n * @param index An index at which to insert the page. If you do not specify this parameter, the page will be added to the end.\n * @see addNewPage\n * @see createNewPage\n */\n SurveyModel.prototype.addPage = function (page, index) {\n if (index === void 0) { index = -1; }\n if (page == null)\n return;\n if (index < 0 || index >= this.pages.length) {\n this.pages.push(page);\n }\n else {\n this.pages.splice(index, 0, page);\n }\n };\n /**\n * Creates a new page and adds it to the survey.\n *\n * If you want to switch a survey to the newly added page, assign its index to the [`currentPageNo`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#currentPageNo) property or assign the entire page to the [`currentPage`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#currentPage) property.\n *\n * @param name A page name. If you do not specify this parameter, it will be generated automatically.\n * @param index An index at which to insert the page. If you do not specify this parameter, the page will be added to the end.\n * @returns The created and added page.\n * @see addPage\n * @see createNewPage\n */\n SurveyModel.prototype.addNewPage = function (name, index) {\n if (name === void 0) { name = null; }\n if (index === void 0) { index = -1; }\n var page = this.createNewPage(name);\n this.addPage(page, index);\n return page;\n };\n /**\n * Removes a page from the survey.\n *\n * Pass a `PageModel` object to this method. You can get this object in different ways. For example, you can call the [`getPageByName()`](#getPageByName) method to obtain a `PageModel` object with a specific name or use the [`currentPage`](#currentPage) property to access and delete the current page, as shown in the code below.\n *\n * ```js\n * // Delete the current page\n * survey.removePage(survey.currentPage);\n * ```\n * @param page A page to remove.\n * @see addNewPage\n */\n SurveyModel.prototype.removePage = function (page) {\n var index = this.pages.indexOf(page);\n if (index < 0)\n return;\n this.pages.splice(index, 1);\n if (this.currentPage == page) {\n this.currentPage = this.pages.length > 0 ? this.pages[0] : null;\n }\n };\n /**\n * Returns a question with a specified [`name`](https://surveyjs.io/form-library/documentation/api-reference/question#name).\n * @param name A question name\n * @param caseInsensitive *(Optional)* A Boolean value that specifies case sensitivity when searching for the question. Default value: `false` (uppercase and lowercase letters are treated as distinct).\n * @returns A question with a specified name.\n * @see getAllQuestions\n * @see getQuestionByValueName\n */\n SurveyModel.prototype.getQuestionByName = function (name, caseInsensitive) {\n if (caseInsensitive === void 0) { caseInsensitive = false; }\n if (!name)\n return null;\n if (caseInsensitive) {\n name = name.toLowerCase();\n }\n var hash = !!caseInsensitive\n ? this.questionHashes.namesInsensitive\n : this.questionHashes.names;\n var res = hash[name];\n if (!res)\n return null;\n return res[0];\n };\n SurveyModel.prototype.findQuestionByName = function (name) {\n return this.getQuestionByName(name);\n };\n /**\n * Returns a question with a specified [`valueName`](https://surveyjs.io/form-library/documentation/api-reference/question#valueName).\n *\n * > Since `valueName` does not have to be unique, multiple questions can have the same `valueName` value. In this case, the `getQuestionByValueName()` method returns the first such question. If you need to get all questions with the same `valueName`, call the `getQuestionsByValueName()` method.\n * @param valueName A question's `valueName` property value.\n * @param caseInsensitive *(Optional)* A Boolean value that specifies case sensitivity when searching for the question. Default value: `false` (uppercase and lowercase letters are treated as distinct).\n * @returns A question with a specified `valueName`.\n * @see getAllQuestions\n * @see getQuestionByName\n */\n SurveyModel.prototype.getQuestionByValueName = function (valueName, caseInsensitive) {\n if (caseInsensitive === void 0) { caseInsensitive = false; }\n var res = this.getQuestionsByValueName(valueName, caseInsensitive);\n return !!res ? res[0] : null;\n };\n /**\n * Returns all questions with a specified [`valueName`](https://surveyjs.io/form-library/documentation/api-reference/question#valueName). If a question's `valueName` is undefined, its [`name`](https://surveyjs.io/form-library/documentation/api-reference/question#name) property is used.\n * @param valueName A question's `valueName` property value.\n * @param caseInsensitive *(Optional)* A Boolean value that specifies case sensitivity when searching for the questions. Default value: `false` (uppercase and lowercase letters are treated as distinct).\n * @returns An array of questions with a specified `valueName`.\n * @see getAllQuestions\n * @see getQuestionByName\n */\n SurveyModel.prototype.getQuestionsByValueName = function (valueName, caseInsensitive) {\n if (caseInsensitive === void 0) { caseInsensitive = false; }\n var hash = !!caseInsensitive\n ? this.questionHashes.valueNamesInsensitive\n : this.questionHashes.valueNames;\n var res = hash[valueName];\n if (!res)\n return null;\n return res;\n };\n SurveyModel.prototype.getCalculatedValueByName = function (name) {\n for (var i = 0; i < this.calculatedValues.length; i++) {\n if (name == this.calculatedValues[i].name)\n return this.calculatedValues[i];\n }\n return null;\n };\n /**\n * Returns an array of questions with specified [names](https://surveyjs.io/form-library/documentation/api-reference/question#name).\n * @param names An array of question names.\n * @param caseInsensitive *(Optional)* A Boolean value that specifies case sensitivity when searching for the questions. Default value: `false` (uppercase and lowercase letters are treated as distinct).\n * @returns An array of questions with specified names\n * @see getAllQuestions\n */\n SurveyModel.prototype.getQuestionsByNames = function (names, caseInsensitive) {\n if (caseInsensitive === void 0) { caseInsensitive = false; }\n var result = [];\n if (!names)\n return result;\n for (var i = 0; i < names.length; i++) {\n if (!names[i])\n continue;\n var question = this.getQuestionByName(names[i], caseInsensitive);\n if (question)\n result.push(question);\n }\n return result;\n };\n /**\n * Returns a page to which a specified survey element (question or panel) belongs.\n * @param element A question or panel instance.\n */\n SurveyModel.prototype.getPageByElement = function (element) {\n for (var i = 0; i < this.pages.length; i++) {\n var page = this.pages[i];\n if (page.containsElement(element))\n return page;\n }\n return null;\n };\n /**\n * Returns a page to which a specified question belongs.\n * @param question A question instance.\n */\n SurveyModel.prototype.getPageByQuestion = function (question) {\n return this.getPageByElement(question);\n };\n /**\n * Returns a page with a specified name.\n * @param name A page [name](https://surveyjs.io/form-library/documentation/api-reference/page-model#name).\n */\n SurveyModel.prototype.getPageByName = function (name) {\n for (var i = 0; i < this.pages.length; i++) {\n if (this.pages[i].name == name)\n return this.pages[i];\n }\n return null;\n };\n /**\n * Returns an array of pages with specified names.\n * @param names An array of page names.\n */\n SurveyModel.prototype.getPagesByNames = function (names) {\n var result = [];\n if (!names)\n return result;\n for (var i = 0; i < names.length; i++) {\n if (!names[i])\n continue;\n var page = this.getPageByName(names[i]);\n if (page)\n result.push(page);\n }\n return result;\n };\n /**\n * Returns a list of all [questions](https://surveyjs.io/form-library/documentation/api-reference/question) in the survey.\n * @param visibleOnly A Boolean value that specifies whether to include only visible questions.\n * @param includeDesignTime For internal use.\n * @param includeNested A Boolean value that specifies whether to include nested questions, such as questions within matrix cells.\n * @returns An array of questions.\n * @see getQuestionByName\n */\n SurveyModel.prototype.getAllQuestions = function (visibleOnly, includeDesignTime, includeNested) {\n if (visibleOnly === void 0) { visibleOnly = false; }\n if (includeDesignTime === void 0) { includeDesignTime = false; }\n if (includeNested === void 0) { includeNested = false; }\n if (includeNested)\n includeDesignTime = false;\n var res = [];\n for (var i = 0; i < this.pages.length; i++) {\n this.pages[i].addQuestionsToList(res, visibleOnly, includeDesignTime);\n }\n if (!includeNested)\n return res;\n var res2 = [];\n res.forEach(function (q) {\n res2.push(q);\n q.getNestedQuestions(visibleOnly).forEach(function (nQ) { return res2.push(nQ); });\n });\n return res2;\n };\n /**\n * Returns an array of quiz questions. A question counts if it is visible, has an input field, and specifies [`correctAnswer`](https://surveyjs.io/form-library/documentation/api-reference/checkbox-question-model#correctAnswer).\n *\n * For more information about quizzes, refer to the following tutorial: [Create a Quiz](https://surveyjs.io/form-library/documentation/design-survey/create-a-quiz).\n * @returns An array of quiz questions.\n * @see getQuizQuestionCount\n */\n SurveyModel.prototype.getQuizQuestions = function () {\n var result = new Array();\n var startIndex = this.getPageStartIndex();\n for (var i = startIndex; i < this.pages.length; i++) {\n if (!this.pages[i].isVisible)\n continue;\n var questions = this.pages[i].questions;\n for (var j = 0; j < questions.length; j++) {\n var q = questions[j];\n if (q.quizQuestionCount > 0) {\n result.push(q);\n }\n }\n }\n return result;\n };\n /**\n * Returns a [panel](https://surveyjs.io/form-library/documentation/api-reference/panel-model) with a specified [`name`](https://surveyjs.io/form-library/documentation/api-reference/panel-model#name).\n * @param name A panel name.\n * @param caseInsensitive *(Optional)* A Boolean value that specifies case sensitivity when searching for the panel. Default value: `false` (uppercase and lowercase letters are treated as distinct).\n * @returns A panel with a specified name.\n * @see getAllPanels\n */\n SurveyModel.prototype.getPanelByName = function (name, caseInsensitive) {\n if (caseInsensitive === void 0) { caseInsensitive = false; }\n var panels = this.getAllPanels();\n if (caseInsensitive)\n name = name.toLowerCase();\n for (var i = 0; i < panels.length; i++) {\n var panelName = panels[i].name;\n if (caseInsensitive)\n panelName = panelName.toLowerCase();\n if (panelName == name)\n return panels[i];\n }\n return null;\n };\n /**\n * Returns a list of all [panels](https://surveyjs.io/form-library/documentation/api-reference/panel-model) in the survey.\n * @param visibleOnly A Boolean value that specifies whether to include only visible panels.\n * @param includeDesignTime For internal use.\n * @returns An array of panels.\n * @see getPanelByName\n */\n SurveyModel.prototype.getAllPanels = function (visibleOnly, includeDesignTime) {\n if (visibleOnly === void 0) { visibleOnly = false; }\n if (includeDesignTime === void 0) { includeDesignTime = false; }\n var result = new Array();\n for (var i = 0; i < this.pages.length; i++) {\n this.pages[i].addPanelsIntoList(result, visibleOnly, includeDesignTime);\n }\n return result;\n };\n /**\n * Creates and returns a new page but does not add it to the survey.\n *\n * Call the [`addPage(page)`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#addPage) method to add the created page to the survey later or the [`addNewPage(name, index)`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#addNewPage) method to create _and_ add a page to the survey.\n * @see addPage\n * @see addNewPage\n */\n SurveyModel.prototype.createNewPage = function (name) {\n var page = _jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"Serializer\"].createClass(\"page\");\n page.name = name;\n return page;\n };\n SurveyModel.prototype.questionOnValueChanging = function (valueName, newValue) {\n if (!!this.editingObj) {\n var prop = _jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"Serializer\"].findProperty(this.editingObj.getType(), valueName);\n if (!!prop)\n newValue = prop.settingValue(this.editingObj, newValue);\n }\n if (this.onValueChanging.isEmpty)\n return newValue;\n var options = {\n name: valueName,\n question: this.getQuestionByValueName(valueName),\n value: this.getUnbindValue(newValue),\n oldValue: this.getValue(valueName),\n };\n this.onValueChanging.fire(this, options);\n return options.value;\n };\n SurveyModel.prototype.updateQuestionValue = function (valueName, newValue) {\n if (this.isLoadingFromJson)\n return;\n var questions = this.getQuestionsByValueName(valueName);\n if (!!questions) {\n for (var i = 0; i < questions.length; i++) {\n var qValue = questions[i].value;\n if ((qValue === newValue && Array.isArray(qValue) && !!this.editingObj) ||\n !this.isTwoValueEquals(qValue, newValue)) {\n questions[i].updateValueFromSurvey(newValue, false);\n }\n }\n }\n };\n SurveyModel.prototype.checkQuestionErrorOnValueChanged = function (question) {\n if (!this.isNavigationButtonPressed &&\n (this.isValidateOnValueChanged ||\n question.getAllErrors().length > 0)) {\n this.checkQuestionErrorOnValueChangedCore(question);\n }\n };\n SurveyModel.prototype.checkQuestionErrorOnValueChangedCore = function (question) {\n var oldErrorCount = question.getAllErrors().length;\n var res = !question.validate(true, {\n isOnValueChanged: !this.isValidateOnValueChanging,\n });\n var isCheckErrorOnChanged = this.checkErrorsMode.indexOf(\"Value\") > -1;\n if (!!question.page && isCheckErrorOnChanged &&\n (oldErrorCount > 0 || question.getAllErrors().length > 0)) {\n this.fireValidatedErrorsOnPage(question.page);\n }\n return res;\n };\n SurveyModel.prototype.checkErrorsOnValueChanging = function (valueName, newValue) {\n if (this.isLoadingFromJson)\n return false;\n var questions = this.getQuestionsByValueName(valueName);\n if (!questions)\n return false;\n var res = false;\n for (var i = 0; i < questions.length; i++) {\n var q = questions[i];\n if (!this.isTwoValueEquals(q.valueForSurvey, newValue)) {\n q.value = newValue;\n }\n if (this.checkQuestionErrorOnValueChangedCore(q))\n res = true;\n res = res || q.errors.length > 0;\n }\n return res;\n };\n SurveyModel.prototype.notifyQuestionOnValueChanged = function (valueName, newValue, questionName) {\n if (this.isLoadingFromJson)\n return;\n var questions = this.getQuestionsByValueName(valueName);\n if (!!questions) {\n for (var i = 0; i < questions.length; i++) {\n var question = questions[i];\n this.checkQuestionErrorOnValueChanged(question);\n question.onSurveyValueChanged(newValue);\n this.onValueChanged.fire(this, {\n name: valueName,\n question: question,\n value: newValue,\n });\n }\n }\n else {\n this.onValueChanged.fire(this, {\n name: valueName,\n question: null,\n value: newValue,\n });\n }\n if (this.isDisposed)\n return;\n this.checkElementsBindings(valueName, newValue);\n this.notifyElementsOnAnyValueOrVariableChanged(valueName, questionName);\n };\n SurveyModel.prototype.checkElementsBindings = function (valueName, newValue) {\n this.isRunningElementsBindings = true;\n for (var i = 0; i < this.pages.length; i++) {\n this.pages[i].checkBindings(valueName, newValue);\n }\n this.isRunningElementsBindings = false;\n if (this.updateVisibleIndexAfterBindings) {\n this.updateVisibleIndexes();\n this.updateVisibleIndexAfterBindings = false;\n }\n };\n SurveyModel.prototype.notifyElementsOnAnyValueOrVariableChanged = function (name, questionName) {\n if (this.isEndLoadingFromJson === \"processing\")\n return;\n if (this.isRunningConditions) {\n this.conditionNotifyElementsOnAnyValueOrVariableChanged = true;\n return;\n }\n for (var i = 0; i < this.pages.length; i++) {\n this.pages[i].onAnyValueChanged(name, questionName);\n }\n if (!this.isEndLoadingFromJson) {\n this.locStrsChanged();\n }\n };\n SurveyModel.prototype.updateAllQuestionsValue = function (clearData) {\n var questions = this.getAllQuestions();\n for (var i = 0; i < questions.length; i++) {\n var q = questions[i];\n var valName = q.getValueName();\n q.updateValueFromSurvey(this.getValue(valName), clearData);\n if (q.requireUpdateCommentValue) {\n q.updateCommentFromSurvey(this.getComment(valName));\n }\n }\n };\n SurveyModel.prototype.notifyAllQuestionsOnValueChanged = function () {\n var questions = this.getAllQuestions();\n for (var i = 0; i < questions.length; i++) {\n questions[i].onSurveyValueChanged(this.getValue(questions[i].getValueName()));\n }\n };\n SurveyModel.prototype.checkOnPageTriggers = function (isOnComplete) {\n var questions = this.getCurrentPageQuestions(true);\n var values = {};\n for (var i = 0; i < questions.length; i++) {\n var question = questions[i];\n var name = question.getValueName();\n values[name] = this.getValue(name);\n }\n this.addCalculatedValuesIntoFilteredValues(values);\n this.checkTriggers(values, true, isOnComplete);\n };\n SurveyModel.prototype.getCurrentPageQuestions = function (includeInvsible) {\n if (includeInvsible === void 0) { includeInvsible = false; }\n var result = [];\n var page = this.currentPage;\n if (!page)\n return result;\n for (var i = 0; i < page.questions.length; i++) {\n var question = page.questions[i];\n if ((!includeInvsible && !question.visible) || !question.name)\n continue;\n result.push(question);\n }\n return result;\n };\n SurveyModel.prototype.checkTriggers = function (key, isOnNextPage, isOnComplete, name) {\n if (isOnComplete === void 0) { isOnComplete = false; }\n if (this.isCompleted || this.triggers.length == 0 || this.isDisplayMode)\n return;\n if (this.isTriggerIsRunning) {\n this.triggerValues = this.getFilteredValues();\n for (var k in key) {\n this.triggerKeys[k] = key[k];\n }\n return;\n }\n var isQuestionInvalid = false;\n if (!isOnComplete && name && this.hasRequiredValidQuestionTrigger) {\n var question = this.getQuestionByValueName(name);\n isQuestionInvalid = question && !question.validate(false);\n }\n this.isTriggerIsRunning = true;\n this.triggerKeys = key;\n this.triggerValues = this.getFilteredValues();\n var properties = this.getFilteredProperties();\n var prevCanBeCompleted = this.canBeCompletedByTrigger;\n for (var i = 0; i < this.triggers.length; i++) {\n var trigger = this.triggers[i];\n if (isQuestionInvalid && trigger.requireValidQuestion)\n continue;\n trigger.checkExpression(isOnNextPage, isOnComplete, this.triggerKeys, this.triggerValues, properties);\n }\n if (prevCanBeCompleted !== this.canBeCompletedByTrigger) {\n this.updateButtonsVisibility();\n }\n this.isTriggerIsRunning = false;\n };\n Object.defineProperty(SurveyModel.prototype, \"hasRequiredValidQuestionTrigger\", {\n get: function () {\n for (var i = 0; i < this.triggers.length; i++) {\n if (this.triggers[i].requireValidQuestion)\n return true;\n }\n return false;\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.doElementsOnLoad = function () {\n for (var i = 0; i < this.pages.length; i++) {\n this.pages[i].onSurveyLoad();\n }\n };\n Object.defineProperty(SurveyModel.prototype, \"isRunningConditions\", {\n get: function () {\n return !!this.conditionValues;\n },\n enumerable: false,\n configurable: true\n });\n /**\n * Recalculates all [expressions](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#expressions) in the survey.\n */\n SurveyModel.prototype.runExpressions = function () {\n this.runConditions();\n };\n SurveyModel.prototype.runConditions = function () {\n if (this.isCompleted ||\n this.isEndLoadingFromJson === \"processing\" ||\n this.isRunningConditions)\n return;\n this.conditionValues = this.getFilteredValues();\n var properties = this.getFilteredProperties();\n var oldCurrentPageIndex = this.pages.indexOf(this.currentPage);\n this.runConditionsCore(properties);\n this.checkIfNewPagesBecomeVisible(oldCurrentPageIndex);\n this.conditionValues = null;\n if (this.isValueChangedOnRunningCondition &&\n this.conditionRunnerCounter <\n _settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].maxConditionRunCountOnValueChanged) {\n this.isValueChangedOnRunningCondition = false;\n this.conditionRunnerCounter++;\n this.runConditions();\n }\n else {\n this.isValueChangedOnRunningCondition = false;\n this.conditionRunnerCounter = 0;\n if (this.conditionUpdateVisibleIndexes) {\n this.conditionUpdateVisibleIndexes = false;\n this.updateVisibleIndexes();\n }\n if (this.conditionNotifyElementsOnAnyValueOrVariableChanged) {\n this.conditionNotifyElementsOnAnyValueOrVariableChanged = false;\n this.notifyElementsOnAnyValueOrVariableChanged(\"\");\n }\n }\n };\n SurveyModel.prototype.runConditionOnValueChanged = function (name, value) {\n if (this.isRunningConditions) {\n this.conditionValues[name] = value;\n this.isValueChangedOnRunningCondition = true;\n }\n else {\n this.runConditions();\n this.runQuestionsTriggers(name, value);\n }\n };\n SurveyModel.prototype.runConditionsCore = function (properties) {\n var pages = this.pages;\n for (var i = 0; i < this.calculatedValues.length; i++) {\n this.calculatedValues[i].resetCalculation();\n }\n for (var i = 0; i < this.calculatedValues.length; i++) {\n this.calculatedValues[i].doCalculation(this.calculatedValues, this.conditionValues, properties);\n }\n _super.prototype.runConditionCore.call(this, this.conditionValues, properties);\n for (var i_2 = 0; i_2 < pages.length; i_2++) {\n pages[i_2].runCondition(this.conditionValues, properties);\n }\n };\n SurveyModel.prototype.runQuestionsTriggers = function (name, value) {\n if (this.isDisplayMode || this.isDesignMode)\n return;\n var questions = this.getAllQuestions();\n questions.forEach(function (q) { return q.runTriggers(name, value); });\n };\n SurveyModel.prototype.checkIfNewPagesBecomeVisible = function (oldCurrentPageIndex) {\n var newCurrentPageIndex = this.pages.indexOf(this.currentPage);\n if (newCurrentPageIndex <= oldCurrentPageIndex + 1)\n return;\n for (var i = oldCurrentPageIndex + 1; i < newCurrentPageIndex; i++) {\n if (this.pages[i].isVisible) {\n this.currentPage = this.pages[i];\n break;\n }\n }\n };\n /**\n * Posts a survey result to [SurveyJS Service](https://api.surveyjs.io/).\n * @param postId An identifier used to save survey results. You can find it on the [My Surveys](https://surveyjs.io/service/mysurveys) page. If you do not specify this parameter, the survey uses the [`surveyPostId`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#surveyPostId) property value.\n * @param clientId A respondent identifier (e-mail or other unique ID). This ID ensures that the respondent does not pass the same survey twice.\n * @param isPartial Pass `true` to save partial survey results (see [Continue an Incomplete Survey](https://surveyjs.io/form-library/documentation/handle-survey-results-continue-incomplete)).\n */\n SurveyModel.prototype.sendResult = function (postId, clientId, isPartial) {\n if (postId === void 0) { postId = null; }\n if (clientId === void 0) { clientId = null; }\n if (isPartial === void 0) { isPartial = false; }\n if (!this.isEditMode)\n return;\n if (isPartial && this.onPartialSend) {\n this.onPartialSend.fire(this, null);\n }\n if (!postId && this.surveyPostId) {\n postId = this.surveyPostId;\n }\n if (!postId)\n return;\n if (clientId) {\n this.clientId = clientId;\n }\n if (isPartial && !this.clientId)\n return;\n var self = this;\n if (this.surveyShowDataSaving) {\n this.setCompletedState(\"saving\", \"\");\n }\n this.createSurveyService().sendResult(postId, this.data, function (success, response, request) {\n if (self.surveyShowDataSaving) {\n if (success) {\n self.setCompletedState(\"success\", \"\");\n }\n else {\n self.setCompletedState(\"error\", response);\n }\n }\n self.onSendResult.fire(self, {\n success: success,\n response: response,\n request: request,\n });\n }, this.clientId, isPartial);\n };\n /**\n * Requests [SurveyJS Service](https://api.surveyjs.io/) to retrieve all answers to a specified question. Handle the [`onGetResult`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#onGetResult) event to access the answers.\n * @param resultId A result ID that identifies the required survey. You can find it on the [My Surveys](https://surveyjs.io/service/mysurveys) page.\n * @param questionName A question name.\n */\n SurveyModel.prototype.getResult = function (resultId, questionName) {\n var self = this;\n this.createSurveyService().getResult(resultId, questionName, function (success, data, dataList, response) {\n self.onGetResult.fire(self, {\n success: success,\n data: data,\n dataList: dataList,\n response: response,\n });\n });\n };\n /**\n * Loads a survey JSON schema from the [SurveyJS Service](https://api.surveyjs.io). You can handle the [`onLoadedSurveyFromService`](#onLoadedSurveyFromService) event to modify the schema after loading if required.\n * @param surveyId The identifier of a survey JSON schema to load. Refer to the following help topic for more information: [Store Survey Results in the SurveyJS Service](https://surveyjs.io/form-library/documentation/handle-survey-results-store#store-survey-results-in-the-surveyjs-service).\n * @param clientId A user identifier (e-mail or other unique ID) used to determine whether the user has already taken the survey.\n */\n SurveyModel.prototype.loadSurveyFromService = function (surveyId, clientId) {\n if (surveyId === void 0) { surveyId = null; }\n if (clientId === void 0) { clientId = null; }\n if (surveyId) {\n this.surveyId = surveyId;\n }\n if (clientId) {\n this.clientId = clientId;\n }\n var self = this;\n this.isLoading = true;\n this.onLoadingSurveyFromService();\n if (clientId) {\n this.createSurveyService().getSurveyJsonAndIsCompleted(this.surveyId, this.clientId, function (success, json, isCompleted, response) {\n self.isLoading = false;\n if (success) {\n self.isCompletedBefore = isCompleted == \"completed\";\n self.loadSurveyFromServiceJson(json);\n }\n });\n }\n else {\n this.createSurveyService().loadSurvey(this.surveyId, function (success, result, response) {\n self.isLoading = false;\n if (success) {\n self.loadSurveyFromServiceJson(result);\n }\n });\n }\n };\n SurveyModel.prototype.loadSurveyFromServiceJson = function (json) {\n if (!json)\n return;\n this.fromJSON(json);\n this.notifyAllQuestionsOnValueChanged();\n this.onLoadSurveyFromService();\n this.onLoadedSurveyFromService.fire(this, {});\n };\n SurveyModel.prototype.onLoadingSurveyFromService = function () { };\n SurveyModel.prototype.onLoadSurveyFromService = function () { };\n SurveyModel.prototype.resetVisibleIndexes = function () {\n var questions = this.getAllQuestions(true);\n for (var i = 0; i < questions.length; i++) {\n questions[i].setVisibleIndex(-1);\n }\n this.updateVisibleIndexes();\n };\n SurveyModel.prototype.updateVisibleIndexes = function () {\n if (this.isLoadingFromJson || !!this.isEndLoadingFromJson || this.isLockingUpdateOnPageModes)\n return;\n if (this.isRunningConditions &&\n this.onQuestionVisibleChanged.isEmpty &&\n this.onPageVisibleChanged.isEmpty) {\n //Run update visible index only one time on finishing running conditions\n this.conditionUpdateVisibleIndexes = true;\n return;\n }\n if (this.isRunningElementsBindings) {\n this.updateVisibleIndexAfterBindings = true;\n return;\n }\n this.updatePageVisibleIndexes(this.showPageNumbers);\n if (this.showQuestionNumbers == \"onPage\") {\n var visPages = this.visiblePages;\n for (var i = 0; i < visPages.length; i++) {\n visPages[i].setVisibleIndex(0);\n }\n }\n else {\n var index = this.showQuestionNumbers == \"on\" ? 0 : -1;\n for (var i = 0; i < this.pages.length; i++) {\n index += this.pages[i].setVisibleIndex(index);\n }\n }\n this.updateProgressText(true);\n };\n SurveyModel.prototype.updatePageVisibleIndexes = function (showIndex) {\n this.updateButtonsVisibility();\n var index = 0;\n for (var i = 0; i < this.pages.length; i++) {\n var page = this.pages[i];\n var isPageVisible = page.isVisible && (i > 0 || !page.isStartPage);\n page.visibleIndex = isPageVisible ? index++ : -1;\n page.num = isPageVisible ? page.visibleIndex + 1 : -1;\n }\n };\n SurveyModel.prototype.fromJSON = function (json, options) {\n if (!json)\n return;\n this.questionHashesClear();\n this.jsonErrors = null;\n var jsonConverter = new _jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"JsonObject\"]();\n jsonConverter.toObject(json, this, options);\n if (jsonConverter.errors.length > 0) {\n this.jsonErrors = jsonConverter.errors;\n }\n this.onStateAndCurrentPageChanged();\n this.updateState();\n };\n SurveyModel.prototype.startLoadingFromJson = function (json) {\n _super.prototype.startLoadingFromJson.call(this, json);\n if (json && json.locale) {\n this.locale = json.locale;\n }\n };\n SurveyModel.prototype.setJsonObject = function (jsonObj) {\n this.fromJSON(jsonObj);\n };\n SurveyModel.prototype.endLoadingFromJson = function () {\n this.isEndLoadingFromJson = \"processing\";\n this.onFirstPageIsStartedChanged();\n this.onQuestionsOnPageModeChanged(\"standard\", true);\n _super.prototype.endLoadingFromJson.call(this);\n if (this.hasCookie) {\n this.isCompletedBefore = true;\n }\n this.doElementsOnLoad();\n this.isEndLoadingFromJson = \"conditions\";\n this.runConditions();\n this.notifyElementsOnAnyValueOrVariableChanged(\"\");\n this.isEndLoadingFromJson = null;\n this.updateVisibleIndexes();\n this.updateHasLogo();\n this.updateRenderBackgroundImage();\n this.updateCurrentPage();\n this.hasDescription = !!this.description;\n this.titleIsEmpty = this.locTitle.isEmpty;\n this.setCalculatedWidthModeUpdater();\n };\n SurveyModel.prototype.updateNavigationCss = function () {\n if (!!this.navigationBar) {\n this.updateNavigationBarCss();\n !!this.updateNavigationItemCssCallback && this.updateNavigationItemCssCallback();\n }\n };\n SurveyModel.prototype.updateNavigationBarCss = function () {\n var val = this.navigationBar;\n val.cssClasses = this.css.actionBar;\n val.containerCss = this.css.footer;\n };\n SurveyModel.prototype.createNavigationBar = function () {\n var res = new _actions_container__WEBPACK_IMPORTED_MODULE_17__[\"ActionContainer\"]();\n res.setItems(this.createNavigationActions());\n return res;\n };\n SurveyModel.prototype.createNavigationActions = function () {\n var _this = this;\n var defaultComponent = \"sv-nav-btn\";\n var navStart = new _actions_action__WEBPACK_IMPORTED_MODULE_16__[\"Action\"]({\n id: \"sv-nav-start\",\n visible: new _base__WEBPACK_IMPORTED_MODULE_2__[\"ComputedUpdater\"](function () { return _this.isShowStartingPage; }),\n visibleIndex: 10,\n locTitle: this.locStartSurveyText,\n action: function () { return _this.start(); },\n component: defaultComponent\n });\n var navPrev = new _actions_action__WEBPACK_IMPORTED_MODULE_16__[\"Action\"]({\n id: \"sv-nav-prev\",\n visible: new _base__WEBPACK_IMPORTED_MODULE_2__[\"ComputedUpdater\"](function () { return _this.isShowPrevButton; }),\n visibleIndex: 20,\n data: {\n mouseDown: function () { return _this.navigationMouseDown(); },\n },\n locTitle: this.locPagePrevText,\n action: function () { return _this.prevPage(); },\n component: defaultComponent\n });\n var navNext = new _actions_action__WEBPACK_IMPORTED_MODULE_16__[\"Action\"]({\n id: \"sv-nav-next\",\n visible: new _base__WEBPACK_IMPORTED_MODULE_2__[\"ComputedUpdater\"](function () { return _this.isShowNextButton; }),\n visibleIndex: 30,\n data: {\n mouseDown: function () { return _this.nextPageMouseDown(); },\n },\n locTitle: this.locPageNextText,\n action: function () { return _this.nextPageUIClick(); },\n component: defaultComponent\n });\n var navPreview = new _actions_action__WEBPACK_IMPORTED_MODULE_16__[\"Action\"]({\n id: \"sv-nav-preview\",\n visible: new _base__WEBPACK_IMPORTED_MODULE_2__[\"ComputedUpdater\"](function () { return _this.isPreviewButtonVisible; }),\n visibleIndex: 40,\n data: {\n mouseDown: function () { return _this.navigationMouseDown(); },\n },\n locTitle: this.locPreviewText,\n action: function () { return _this.showPreview(); },\n component: defaultComponent\n });\n var navComplete = new _actions_action__WEBPACK_IMPORTED_MODULE_16__[\"Action\"]({\n id: \"sv-nav-complete\",\n visible: new _base__WEBPACK_IMPORTED_MODULE_2__[\"ComputedUpdater\"](function () { return _this.isCompleteButtonVisible; }),\n visibleIndex: 50,\n data: {\n mouseDown: function () { return _this.navigationMouseDown(); },\n },\n locTitle: this.locCompleteText,\n action: function () { return _this.taskManager.waitAndExecute(function () { return _this.completeLastPage(); }); },\n component: defaultComponent\n });\n this.updateNavigationItemCssCallback = function () {\n navStart.innerCss = _this.cssNavigationStart;\n navPrev.innerCss = _this.cssNavigationPrev;\n navNext.innerCss = _this.cssNavigationNext;\n navPreview.innerCss = _this.cssNavigationPreview;\n navComplete.innerCss = _this.cssNavigationComplete;\n };\n return [navStart, navPrev, navNext, navPreview, navComplete];\n };\n SurveyModel.prototype.onBeforeCreating = function () { };\n SurveyModel.prototype.onCreating = function () { };\n SurveyModel.prototype.getProcessedTextValue = function (textValue) {\n this.getProcessedTextValueCore(textValue);\n if (!this.onProcessTextValue.isEmpty) {\n var wasEmpty = this.isValueEmpty(textValue.value);\n this.onProcessTextValue.fire(this, textValue);\n textValue.isExists =\n textValue.isExists || (wasEmpty && !this.isValueEmpty(textValue.value));\n }\n };\n SurveyModel.prototype.getBuiltInVariableValue = function (name) {\n if (name === \"pageno\") {\n var page = this.currentPage;\n return page != null ? this.visiblePages.indexOf(page) + 1 : 0;\n }\n if (name === \"pagecount\") {\n return this.visiblePageCount;\n }\n if (name === \"correctedanswers\" || name === \"correctanswers\" || name === \"correctedanswercount\") {\n return this.getCorrectedAnswerCount();\n }\n if (name === \"incorrectedanswers\" || name === \"incorrectanswers\" || name === \"incorrectedanswercount\") {\n return this.getInCorrectedAnswerCount();\n }\n if (name === \"questioncount\") {\n return this.getQuizQuestionCount();\n }\n return undefined;\n };\n SurveyModel.prototype.getProcessedTextValueCore = function (textValue) {\n var name = textValue.name.toLocaleLowerCase();\n if ([\"no\", \"require\", \"title\"].indexOf(name) !== -1) {\n return;\n }\n var builtInVar = this.getBuiltInVariableValue(name);\n if (builtInVar !== undefined) {\n textValue.isExists = true;\n textValue.value = builtInVar;\n return;\n }\n if (name === \"locale\") {\n textValue.isExists = true;\n textValue.value = !!this.locale\n ? this.locale\n : _surveyStrings__WEBPACK_IMPORTED_MODULE_8__[\"surveyLocalization\"].defaultLocale;\n return;\n }\n var variable = this.getVariable(name);\n if (variable !== undefined) {\n textValue.isExists = true;\n textValue.value = variable;\n return;\n }\n var question = this.getFirstName(name);\n if (question) {\n var questionUseDisplayText = question.useDisplayValuesInDynamicTexts;\n textValue.isExists = true;\n var firstName = question.getValueName().toLowerCase();\n name = firstName + name.substring(firstName.length);\n name = name.toLocaleLowerCase();\n var values = {};\n values[firstName] = textValue.returnDisplayValue && questionUseDisplayText\n ? question.getDisplayValue(false, undefined)\n : question.value;\n textValue.value = new _conditionProcessValue__WEBPACK_IMPORTED_MODULE_6__[\"ProcessValue\"]().getValue(name, values);\n return;\n }\n this.getProcessedValuesWithoutQuestion(textValue);\n };\n SurveyModel.prototype.getProcessedValuesWithoutQuestion = function (textValue) {\n var value = this.getValue(textValue.name);\n if (value !== undefined) {\n textValue.isExists = true;\n textValue.value = value;\n return;\n }\n var processor = new _conditionProcessValue__WEBPACK_IMPORTED_MODULE_6__[\"ProcessValue\"]();\n var firstName = processor.getFirstName(textValue.name);\n if (firstName === textValue.name)\n return;\n var data = {};\n var val = this.getValue(firstName);\n if (_helpers__WEBPACK_IMPORTED_MODULE_0__[\"Helpers\"].isValueEmpty(val)) {\n val = this.getVariable(firstName);\n }\n if (_helpers__WEBPACK_IMPORTED_MODULE_0__[\"Helpers\"].isValueEmpty(val))\n return;\n data[firstName] = val;\n textValue.value = processor.getValue(textValue.name, data);\n textValue.isExists = processor.hasValue(textValue.name, data);\n };\n SurveyModel.prototype.getFirstName = function (name) {\n name = name.toLowerCase();\n var question;\n do {\n question = this.getQuestionByValueName(name, true);\n name = this.reduceFirstName(name);\n } while (!question && !!name);\n return question;\n };\n SurveyModel.prototype.reduceFirstName = function (name) {\n var pos1 = name.lastIndexOf(\".\");\n var pos2 = name.lastIndexOf(\"[\");\n if (pos1 < 0 && pos2 < 0)\n return \"\";\n var pos = Math.max(pos1, pos2);\n return name.substring(0, pos);\n };\n SurveyModel.prototype.clearUnusedValues = function () {\n this.isClearingUnsedValues = true;\n var questions = this.getAllQuestions();\n for (var i = 0; i < questions.length; i++) {\n questions[i].clearUnusedValues();\n }\n this.clearInvisibleQuestionValues();\n this.isClearingUnsedValues = false;\n };\n SurveyModel.prototype.hasVisibleQuestionByValueName = function (valueName) {\n var questions = this.getQuestionsByValueName(valueName);\n if (!questions)\n return false;\n for (var i = 0; i < questions.length; i++) {\n var q = questions[i];\n if (q.isVisible && q.isParentVisible && !q.parentQuestion)\n return true;\n }\n return false;\n };\n SurveyModel.prototype.questionsByValueName = function (valueName) {\n var questions = this.getQuestionsByValueName(valueName);\n return !!questions ? questions : [];\n };\n SurveyModel.prototype.clearInvisibleQuestionValues = function () {\n var reason = this.clearInvisibleValues === \"none\" ? \"none\" : \"onComplete\";\n var questions = this.getAllQuestions();\n for (var i = 0; i < questions.length; i++) {\n questions[i].clearValueIfInvisible(reason);\n }\n };\n /**\n * Returns a variable value.\n *\n * [Variables help topic](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#variables (linkStyle))\n * @param name A variable name.\n * @return A variable value.\n * @see setVariable\n * @see getVariableNames\n */\n SurveyModel.prototype.getVariable = function (name) {\n if (!name)\n return null;\n name = name.toLowerCase();\n var res = this.variablesHash[name];\n if (!this.isValueEmpty(res))\n return res;\n if (name.indexOf(\".\") > -1 || name.indexOf(\"[\") > -1) {\n if (new _conditionProcessValue__WEBPACK_IMPORTED_MODULE_6__[\"ProcessValue\"]().hasValue(name, this.variablesHash))\n return new _conditionProcessValue__WEBPACK_IMPORTED_MODULE_6__[\"ProcessValue\"]().getValue(name, this.variablesHash);\n }\n return res;\n };\n /**\n * Sets a variable value.\n *\n * [Variables help topic](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#variables (linkStyle))\n * @param name A variable name.\n * @param newValue A new variable value.\n * @see getVariable\n * @see getVariableNames\n */\n SurveyModel.prototype.setVariable = function (name, newValue) {\n if (!name)\n return;\n if (!!this.valuesHash) {\n delete this.valuesHash[name];\n }\n name = name.toLowerCase();\n this.variablesHash[name] = newValue;\n this.notifyElementsOnAnyValueOrVariableChanged(name);\n this.runConditionOnValueChanged(name, newValue);\n this.onVariableChanged.fire(this, { name: name, value: newValue });\n };\n /**\n * Returns the names of all variables in the survey.\n *\n * [Variables help topic](https://surveyjs.io/form-library/documentation/design-survey/conditional-logic#variables (linkStyle))\n * @returns An array of variable names.\n * @see getVariable\n * @see setVariable\n */\n SurveyModel.prototype.getVariableNames = function () {\n var res = [];\n for (var key in this.variablesHash) {\n res.push(key);\n }\n return res;\n };\n //ISurvey data\n SurveyModel.prototype.getUnbindValue = function (value) {\n if (!!this.editingObj)\n return value;\n return _helpers__WEBPACK_IMPORTED_MODULE_0__[\"Helpers\"].getUnbindValue(value);\n };\n /**\n * Returns a value (answer) for a question with a specified `name`.\n * @param name A question name.\n * @returns A question value (answer).\n * @see data\n * @see setValue\n */\n SurveyModel.prototype.getValue = function (name) {\n if (!name || name.length == 0)\n return null;\n var value = this.getDataValueCore(this.valuesHash, name);\n return this.getUnbindValue(value);\n };\n /**\n * Sets a question value (answer).\n *\n * > This method executes all triggers and reevaluates conditions (`visibleIf`, `requiredId`, and others). It also switches the survey to the next page if the [`goNextPageAutomatic`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#goNextPageAutomatic) property is enabled and all questions on the current page have correct answers.\n * @param name A question name.\n * @param newValue A new question value.\n * @param locNotification For internal use.\n * @param allowNotifyValueChanged For internal use.\n * @see data\n * @see getValue\n */\n SurveyModel.prototype.setValue = function (name, newQuestionValue, locNotification, allowNotifyValueChanged, questionName) {\n if (locNotification === void 0) { locNotification = false; }\n if (allowNotifyValueChanged === void 0) { allowNotifyValueChanged = true; }\n if (this.isLockingUpdateOnPageModes)\n return;\n var newValue = newQuestionValue;\n if (allowNotifyValueChanged) {\n newValue = this.questionOnValueChanging(name, newQuestionValue);\n }\n if (this.isValidateOnValueChanging &&\n this.checkErrorsOnValueChanging(name, newValue))\n return;\n if (!this.editingObj &&\n this.isValueEqual(name, newValue) &&\n this.isTwoValueEquals(newValue, newQuestionValue))\n return;\n var oldValue = this.getValue(name);\n if (this.isValueEmpyOnSetValue(name, newValue)) {\n this.deleteDataValueCore(this.valuesHash, name);\n }\n else {\n newValue = this.getUnbindValue(newValue);\n this.setDataValueCore(this.valuesHash, name, newValue);\n }\n this.updateOnSetValue(name, newValue, oldValue, locNotification, allowNotifyValueChanged, questionName);\n };\n SurveyModel.prototype.isValueEmpyOnSetValue = function (name, val) {\n if (!this.isValueEmpty(val, false))\n return false;\n if (!this.editingObj || val === null || val === undefined)\n return true;\n return this.editingObj.getDefaultPropertyValue(name) === val;\n };\n SurveyModel.prototype.updateOnSetValue = function (name, newValue, oldValue, locNotification, allowNotifyValueChanged, questionName) {\n if (locNotification === void 0) { locNotification = false; }\n if (allowNotifyValueChanged === void 0) { allowNotifyValueChanged = true; }\n this.updateQuestionValue(name, newValue);\n if (locNotification === true || this.isDisposed || this.isRunningElementsBindings)\n return;\n questionName = questionName || name;\n var triggerKeys = {};\n triggerKeys[name] = { newValue: newValue, oldValue: oldValue };\n this.runConditionOnValueChanged(name, newValue);\n this.checkTriggers(triggerKeys, false, false, name);\n if (allowNotifyValueChanged)\n this.notifyQuestionOnValueChanged(name, newValue, questionName);\n if (locNotification !== \"text\") {\n this.tryGoNextPageAutomatic(name);\n }\n };\n SurveyModel.prototype.isValueEqual = function (name, newValue) {\n if (newValue === \"\" || newValue === undefined)\n newValue = null;\n var oldValue = this.getValue(name);\n if (oldValue === \"\" || oldValue === undefined)\n oldValue = null;\n if (newValue === null || oldValue === null)\n return newValue === oldValue;\n return this.isTwoValueEquals(newValue, oldValue);\n };\n SurveyModel.prototype.doOnPageAdded = function (page) {\n page.setSurveyImpl(this);\n if (!page.name)\n page.name = this.generateNewName(this.pages, \"page\");\n this.questionHashesPanelAdded(page);\n this.updateVisibleIndexes();\n if (!!this.runningPages)\n return;\n if (!this.isLoadingFromJson) {\n this.updateProgressText();\n this.updateCurrentPage();\n }\n var options = { page: page };\n this.onPageAdded.fire(this, options);\n };\n SurveyModel.prototype.doOnPageRemoved = function (page) {\n page.setSurveyImpl(null);\n if (!!this.runningPages)\n return;\n if (page === this.currentPage) {\n this.updateCurrentPage();\n }\n this.updateVisibleIndexes();\n this.updateProgressText();\n this.updateLazyRenderingRowsOnRemovingElements();\n };\n SurveyModel.prototype.generateNewName = function (elements, baseName) {\n var keys = {};\n for (var i = 0; i < elements.length; i++)\n keys[elements[i][\"name\"]] = true;\n var index = 1;\n while (keys[baseName + index])\n index++;\n return baseName + index;\n };\n SurveyModel.prototype.tryGoNextPageAutomatic = function (name) {\n var _this = this;\n if (!!this.isEndLoadingFromJson ||\n !this.goNextPageAutomatic ||\n !this.currentPage)\n return;\n var question = this.getQuestionByValueName(name);\n if (!question ||\n (!!question &&\n (!question.visible || !question.supportGoNextPageAutomatic())))\n return;\n if (!question.validate(false) && !question.supportGoNextPageError())\n return;\n var questions = this.getCurrentPageQuestions();\n if (questions.indexOf(question) < 0)\n return;\n for (var i = 0; i < questions.length; i++) {\n if (questions[i].hasInput && questions[i].isEmpty())\n return;\n }\n if (this.isLastPage && (this.goNextPageAutomatic !== true || !this.allowCompleteSurveyAutomatic))\n return;\n if (this.checkIsCurrentPageHasErrors(false))\n return;\n var goNextPage = function () {\n if (!_this.isLastPage) {\n _this.nextPage();\n }\n else {\n if (_this.isShowPreviewBeforeComplete) {\n _this.showPreview();\n }\n else {\n _this.completeLastPage();\n }\n }\n };\n _surveytimer__WEBPACK_IMPORTED_MODULE_21__[\"surveyTimerFunctions\"].safeTimeOut(goNextPage, _settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].autoAdvanceDelay);\n };\n /**\n * Returns a comment value from a question with a specified `name`.\n * @param name A question name.\n * @returns A comment.\n * @see setComment\n */\n SurveyModel.prototype.getComment = function (name) {\n var res = this.getValue(name + this.commentSuffix);\n return res || \"\";\n };\n /**\n * Sets a comment value to a question with a specified `name`.\n * @param name A question name.\n * @param newValue A new comment value.\n * @param locNotification For internal use.\n * @see getComment\n */\n SurveyModel.prototype.setComment = function (name, newValue, locNotification) {\n if (locNotification === void 0) { locNotification = false; }\n if (!newValue)\n newValue = \"\";\n if (this.isTwoValueEquals(newValue, this.getComment(name)))\n return;\n var commentName = name + this.commentSuffix;\n if (this.isValueEmpty(newValue)) {\n this.deleteDataValueCore(this.valuesHash, commentName);\n }\n else {\n this.setDataValueCore(this.valuesHash, commentName, newValue);\n }\n var questions = this.getQuestionsByValueName(name);\n if (!!questions) {\n for (var i = 0; i < questions.length; i++) {\n questions[i].updateCommentFromSurvey(newValue);\n this.checkQuestionErrorOnValueChanged(questions[i]);\n }\n }\n if (!locNotification) {\n this.runConditionOnValueChanged(name, this.getValue(name));\n }\n if (locNotification !== \"text\") {\n this.tryGoNextPageAutomatic(name);\n }\n var question = this.getQuestionByName(name);\n if (question) {\n this.onValueChanged.fire(this, {\n name: commentName,\n question: question,\n value: newValue,\n });\n }\n };\n /**\n * Deletes an answer from survey results.\n * @param {string} name An object property that stores the answer to delete. Pass a question's [`valueName`](https://surveyjs.io/form-library/documentation/api-reference/question#valueName) or [`name`](https://surveyjs.io/form-library/documentation/api-reference/question#name).\n */\n SurveyModel.prototype.clearValue = function (name) {\n this.setValue(name, null);\n this.setComment(name, null);\n };\n Object.defineProperty(SurveyModel.prototype, \"clearValueOnDisableItems\", {\n /**\n * Specifies whether to remove disabled choices from the value in [Dropdown](https://surveyjs.io/form-library/documentation/api-reference/dropdown-menu-model), [Checkboxes](https://surveyjs.io/form-library/documentation/api-reference/checkbox-question-model), and [Radio Button Group](https://surveyjs.io/form-library/documentation/api-reference/radio-button-question-model) questions.\n *\n * Default value: `false`\n *\n * > This property cannot be specified in the survey JSON schema. Use dot notation to specify it.\n */\n get: function () {\n return this.getPropertyValue(\"clearValueOnDisableItems\", false);\n },\n set: function (val) {\n this.setPropertyValue(\"clearValueOnDisableItems\", val);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.getQuestionClearIfInvisible = function (questionClearIf) {\n if (this.isShowingPreview || this.runningPages)\n return \"none\";\n if (questionClearIf !== \"default\")\n return questionClearIf;\n return this.clearInvisibleValues;\n };\n SurveyModel.prototype.questionVisibilityChanged = function (question, newValue, resetIndexes) {\n if (resetIndexes) {\n this.updateVisibleIndexes();\n }\n this.onQuestionVisibleChanged.fire(this, {\n question: question,\n name: question.name,\n visible: newValue,\n });\n };\n SurveyModel.prototype.pageVisibilityChanged = function (page, newValue) {\n if (this.isLoadingFromJson)\n return;\n if (newValue && !this.currentPage || page === this.currentPage) {\n this.updateCurrentPage();\n }\n this.updateVisibleIndexes();\n this.onPageVisibleChanged.fire(this, {\n page: page,\n visible: newValue,\n });\n };\n SurveyModel.prototype.panelVisibilityChanged = function (panel, newValue) {\n this.updateVisibleIndexes();\n this.onPanelVisibleChanged.fire(this, {\n panel: panel,\n visible: newValue,\n });\n };\n SurveyModel.prototype.questionCreated = function (question) {\n this.onQuestionCreated.fire(this, { question: question });\n };\n SurveyModel.prototype.questionAdded = function (question, index, parentPanel, rootPanel) {\n if (!question.name) {\n question.name = this.generateNewName(this.getAllQuestions(false, true), \"question\");\n }\n if (!!question.page) {\n this.questionHashesAdded(question);\n }\n if (!this.currentPage) {\n this.updateCurrentPage();\n }\n this.updateVisibleIndexes();\n this.setCalculatedWidthModeUpdater();\n if (this.canFireAddElement()) {\n this.onQuestionAdded.fire(this, {\n question: question,\n name: question.name,\n index: index,\n parent: parentPanel,\n page: rootPanel,\n parentPanel: parentPanel,\n rootPanel: rootPanel,\n });\n }\n };\n SurveyModel.prototype.canFireAddElement = function () {\n return !this.isMovingQuestion || this.isDesignMode && !_settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].supportCreatorV2;\n };\n SurveyModel.prototype.questionRemoved = function (question) {\n this.questionHashesRemoved(question, question.name, question.getValueName());\n this.updateVisibleIndexes();\n this.onQuestionRemoved.fire(this, {\n question: question,\n name: question.name,\n });\n this.updateLazyRenderingRowsOnRemovingElements();\n };\n SurveyModel.prototype.questionRenamed = function (question, oldName, oldValueName) {\n this.questionHashesRemoved(question, oldName, oldValueName);\n this.questionHashesAdded(question);\n };\n SurveyModel.prototype.questionHashesClear = function () {\n this.questionHashes.names = {};\n this.questionHashes.namesInsensitive = {};\n this.questionHashes.valueNames = {};\n this.questionHashes.valueNamesInsensitive = {};\n };\n SurveyModel.prototype.questionHashesPanelAdded = function (panel) {\n if (this.isLoadingFromJson)\n return;\n var questions = panel.questions;\n for (var i = 0; i < questions.length; i++) {\n this.questionHashesAdded(questions[i]);\n }\n };\n SurveyModel.prototype.questionHashesAdded = function (question) {\n this.questionHashAddedCore(this.questionHashes.names, question, question.name);\n this.questionHashAddedCore(this.questionHashes.namesInsensitive, question, question.name.toLowerCase());\n this.questionHashAddedCore(this.questionHashes.valueNames, question, question.getValueName());\n this.questionHashAddedCore(this.questionHashes.valueNamesInsensitive, question, question.getValueName().toLowerCase());\n };\n SurveyModel.prototype.questionHashesRemoved = function (question, name, valueName) {\n if (!!name) {\n this.questionHashRemovedCore(this.questionHashes.names, question, name);\n this.questionHashRemovedCore(this.questionHashes.namesInsensitive, question, name.toLowerCase());\n }\n if (!!valueName) {\n this.questionHashRemovedCore(this.questionHashes.valueNames, question, valueName);\n this.questionHashRemovedCore(this.questionHashes.valueNamesInsensitive, question, valueName.toLowerCase());\n }\n };\n SurveyModel.prototype.questionHashAddedCore = function (hash, question, name) {\n var res = hash[name];\n if (!!res) {\n var res = hash[name];\n if (res.indexOf(question) < 0) {\n res.push(question);\n }\n }\n else {\n hash[name] = [question];\n }\n };\n SurveyModel.prototype.questionHashRemovedCore = function (hash, question, name) {\n var res = hash[name];\n if (!res)\n return;\n var index = res.indexOf(question);\n if (index > -1) {\n res.splice(index, 1);\n }\n if (res.length == 0) {\n delete hash[name];\n }\n };\n SurveyModel.prototype.panelAdded = function (panel, index, parentPanel, rootPanel) {\n if (!panel.name) {\n panel.name = this.generateNewName(this.getAllPanels(false, true), \"panel\");\n }\n this.questionHashesPanelAdded(panel);\n this.updateVisibleIndexes();\n if (this.canFireAddElement()) {\n this.onPanelAdded.fire(this, {\n panel: panel,\n name: panel.name,\n index: index,\n parent: parentPanel,\n page: rootPanel,\n parentPanel: parentPanel,\n rootPanel: rootPanel,\n });\n }\n };\n SurveyModel.prototype.panelRemoved = function (panel) {\n this.updateVisibleIndexes();\n this.onPanelRemoved.fire(this, { panel: panel, name: panel.name });\n this.updateLazyRenderingRowsOnRemovingElements();\n };\n SurveyModel.prototype.validateQuestion = function (question) {\n if (this.onValidateQuestion.isEmpty)\n return null;\n var options = {\n name: question.name,\n question: question,\n value: question.value,\n error: null,\n };\n this.onValidateQuestion.fire(this, options);\n return options.error ? new _error__WEBPACK_IMPORTED_MODULE_9__[\"CustomError\"](options.error, this) : null;\n };\n SurveyModel.prototype.validatePanel = function (panel) {\n if (this.onValidatePanel.isEmpty)\n return null;\n var options = {\n name: panel.name,\n panel: panel,\n error: null,\n };\n this.onValidatePanel.fire(this, options);\n return options.error ? new _error__WEBPACK_IMPORTED_MODULE_9__[\"CustomError\"](options.error, this) : null;\n };\n SurveyModel.prototype.processHtml = function (html, reason) {\n if (!reason)\n reason = \"\";\n var options = { html: html, reason: reason };\n this.onProcessHtml.fire(this, options);\n return this.processText(options.html, true);\n };\n SurveyModel.prototype.processText = function (text, returnDisplayValue) {\n return this.processTextEx(text, returnDisplayValue, false).text;\n };\n SurveyModel.prototype.processTextEx = function (text, returnDisplayValue, doEncoding) {\n var res = {\n text: this.processTextCore(text, returnDisplayValue, doEncoding),\n hasAllValuesOnLastRun: true,\n };\n res.hasAllValuesOnLastRun = this.textPreProcessor.hasAllValuesOnLastRun;\n return res;\n };\n Object.defineProperty(SurveyModel.prototype, \"textPreProcessor\", {\n get: function () {\n var _this = this;\n if (!this.textPreProcessorValue) {\n this.textPreProcessorValue = new _textPreProcessor__WEBPACK_IMPORTED_MODULE_5__[\"TextPreProcessor\"]();\n this.textPreProcessorValue.onProcess = function (textValue) {\n _this.getProcessedTextValue(textValue);\n };\n }\n return this.textPreProcessorValue;\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.processTextCore = function (text, returnDisplayValue, doEncoding) {\n if (doEncoding === void 0) { doEncoding = false; }\n if (this.isDesignMode)\n return text;\n return this.textPreProcessor.process(text, returnDisplayValue, doEncoding);\n };\n SurveyModel.prototype.getSurveyMarkdownHtml = function (element, text, name) {\n var options = {\n element: element,\n text: text,\n name: name,\n html: null,\n };\n this.onTextMarkdown.fire(this, options);\n return options.html;\n };\n SurveyModel.prototype.getCorrectedAnswerCount = function () {\n return this.getCorrectAnswerCount();\n };\n /**\n * Returns the number of correct answers in a quiz.\n *\n * For more information about quizzes, refer to the following tutorial: [Create a Quiz](https://surveyjs.io/form-library/documentation/design-survey/create-a-quiz).\n * @returns The number of correct answers in a quiz.\n * @see getQuizQuestionCount\n * @see getInCorrectAnswerCount\n */\n SurveyModel.prototype.getCorrectAnswerCount = function () {\n return this.getCorrectedAnswerCountCore(true);\n };\n /**\n * Returns the number of quiz questions. A question counts if it is visible, has an input field, and specifies [`correctAnswer`](https://surveyjs.io/form-library/documentation/api-reference/checkbox-question-model#correctAnswer).\n *\n * This number may be different from `getQuizQuestions().length` because certain question types (for instance, matrix-like types) include more than one question.\n *\n * For more information about quizzes, refer to the following tutorial: [Create a Quiz](https://surveyjs.io/form-library/documentation/design-survey/create-a-quiz).\n * @returns The number of quiz questions.\n * @see getQuizQuestions\n */\n SurveyModel.prototype.getQuizQuestionCount = function () {\n var questions = this.getQuizQuestions();\n var res = 0;\n for (var i = 0; i < questions.length; i++) {\n res += questions[i].quizQuestionCount;\n }\n return res;\n };\n SurveyModel.prototype.getInCorrectedAnswerCount = function () {\n return this.getInCorrectAnswerCount();\n };\n /**\n * Returns the number of incorrect answers in a quiz.\n *\n * For more information about quizzes, refer to the following tutorial: [Create a Quiz](https://surveyjs.io/form-library/documentation/design-survey/create-a-quiz).\n * @returns The number of incorrect answers in a quiz.\n * @see getCorrectAnswerCount\n */\n SurveyModel.prototype.getInCorrectAnswerCount = function () {\n return this.getCorrectedAnswerCountCore(false);\n };\n SurveyModel.prototype.onCorrectQuestionAnswer = function (question, options) {\n if (this.onIsAnswerCorrect.isEmpty)\n return;\n options.question = question;\n this.onIsAnswerCorrect.fire(this, options);\n };\n SurveyModel.prototype.getCorrectedAnswerCountCore = function (isCorrect) {\n var questions = this.getQuizQuestions();\n var counter = 0;\n for (var i = 0; i < questions.length; i++) {\n var q = questions[i];\n var correctCount = q.correctAnswerCount;\n if (isCorrect) {\n counter += correctCount;\n }\n else {\n counter += q.quizQuestionCount - correctCount;\n }\n }\n return counter;\n };\n SurveyModel.prototype.getCorrectedAnswers = function () {\n return this.getCorrectedAnswerCount();\n };\n SurveyModel.prototype.getInCorrectedAnswers = function () {\n return this.getInCorrectedAnswerCount();\n };\n Object.defineProperty(SurveyModel.prototype, \"showTimerPanel\", {\n /**\n * Displays the timer panel and specifies its position. Applies only to [quiz surveys](https://surveyjs.io/form-library/documentation/design-survey-create-a-quiz).\n *\n * Possible values:\n *\n * - `\"top\"` - Displays the timer panel at the top of the survey.\n * - `\"bottom\"` - Displays the timer panel at the bottom of the survey.\n * - `\"none\"` (default) - Hides the timer panel.\n *\n * If the timer panel is displayed, the timer starts automatically when the survey begins. To specify time limits, use the [`maxTimeToFinish`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#maxTimeToFinish) and [`maxTimeToFinishPage`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#maxTimeToFinishPage) properties.\n *\n * The timer panel displays information about time spent on an individual page and the entire survey. If you want to display only the page timer or the survey timer, set the [`showTimerPanelMode`](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#showTimerPanelMode) property to `\"page\"` or `\"survey\"`.\n * @see startTimer\n * @see stopTimer\n * @see timeSpent\n * @see onTimer\n */\n get: function () {\n return this.getPropertyValue(\"showTimerPanel\");\n },\n set: function (val) {\n this.setPropertyValue(\"showTimerPanel\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isTimerPanelShowingOnTop\", {\n get: function () {\n return this.showTimerPanel == \"top\";\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"isTimerPanelShowingOnBottom\", {\n get: function () {\n return this.showTimerPanel == \"bottom\";\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"showTimerPanelMode\", {\n /**\n * Specifies whether the timer panel displays timers for the current page, the entire survey, or both. Applies only if the timer panel is [visible](https://surveyjs.io/form-library/documentation/api-reference/survey-data-model#showTimerPanel).\n *\n * Possible values:\n *\n * - `\"survey\"` - Displays only the survey timer.\n * - `\"page\"` - Displays only the page timer.\n * - `\"all\"` (default) - Displays both the survey and page timers.\n * @see timeSpent\n * @see onTimer\n * @see startTimer\n * @see stopTimer\n */\n get: function () {\n return this.getPropertyValue(\"showTimerPanelMode\");\n },\n set: function (val) {\n this.setPropertyValue(\"showTimerPanelMode\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"widthMode\", {\n /**\n * Specifies how to calculate the survey width.\n *\n * Possible values:\n *\n * - `\"static\"` - A survey has a [fixed width](#width).\n * - `\"responsive\"` - A survey occupies all available horizontal space and stretches or shrinks horizontally to fit in the screen size.\n * - `\"auto\"` (default) - Survey width depends on a question type and corresponds to the `\"static\"` or `\"responsive\"` mode.\n */\n // `custom/precise` - The survey width is specified by the width property. // in-future\n get: function () {\n return this.getPropertyValue(\"widthMode\");\n },\n set: function (val) {\n this.setPropertyValue(\"widthMode\", val);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.setCalculatedWidthModeUpdater = function () {\n var _this = this;\n if (this.calculatedWidthModeUpdater)\n this.calculatedWidthModeUpdater.dispose();\n this.calculatedWidthModeUpdater = new _base__WEBPACK_IMPORTED_MODULE_2__[\"ComputedUpdater\"](function () { return _this.calculateWidthMode(); });\n this.calculatedWidthMode = this.calculatedWidthModeUpdater;\n };\n SurveyModel.prototype.calculateWidthMode = function () {\n if (this.widthMode == \"auto\") {\n var isResponsive_1 = false;\n this.pages.forEach(function (page) {\n if (page.needResponsiveWidth())\n isResponsive_1 = true;\n });\n return isResponsive_1 ? \"responsive\" : \"static\";\n }\n return this.widthMode;\n };\n Object.defineProperty(SurveyModel.prototype, \"width\", {\n /**\n * A survey width in CSS values.\n *\n * Default value: `undefined` (the survey inherits the width from its container)\n */\n get: function () {\n return this.getPropertyValue(\"width\");\n },\n set: function (val) {\n this.setPropertyValue(\"width\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"renderedWidth\", {\n get: function () {\n var width = this.getPropertyValue(\"width\");\n if (width && !isNaN(width))\n width = width + \"px\";\n return this.getPropertyValue(\"calculatedWidthMode\") == \"static\" && width || undefined;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"timerInfo\", {\n get: function () {\n return this.getTimerInfo();\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"timerClock\", {\n get: function () {\n var major;\n var minor;\n if (!!this.currentPage) {\n var _a = this.getTimerInfo(), spent = _a.spent, limit = _a.limit, minorSpent = _a.minorSpent, minorLimit = _a.minorLimit;\n if (limit > 0)\n major = this.getDisplayClockTime(limit - spent);\n else {\n major = this.getDisplayClockTime(spent);\n }\n if (minorSpent !== undefined) {\n if (minorLimit > 0) {\n minor = this.getDisplayClockTime(minorLimit - minorSpent);\n }\n else {\n minor = this.getDisplayClockTime(minorSpent);\n }\n }\n }\n return { majorText: major, minorText: minor };\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"timerInfoText\", {\n get: function () {\n var options = { text: this.getTimerInfoText() };\n this.onTimerPanelInfoText.fire(this, options);\n var loc = new _localizablestring__WEBPACK_IMPORTED_MODULE_10__[\"LocalizableString\"](this, true);\n loc.text = options.text;\n return loc.textOrHtml;\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.getTimerInfo = function () {\n var page = this.currentPage;\n if (!page)\n return { spent: 0, limit: 0 };\n var pageSpent = page.timeSpent;\n var surveySpent = this.timeSpent;\n var pageLimitSec = this.getPageMaxTimeToFinish(page);\n var surveyLimit = this.maxTimeToFinish;\n if (this.showTimerPanelMode == \"page\") {\n return { spent: pageSpent, limit: pageLimitSec };\n }\n if (this.showTimerPanelMode == \"survey\") {\n return { spent: surveySpent, limit: surveyLimit };\n }\n else {\n if (pageLimitSec > 0 && surveyLimit > 0) {\n return { spent: pageSpent, limit: pageLimitSec, minorSpent: surveySpent, minorLimit: surveyLimit };\n }\n else if (pageLimitSec > 0) {\n return { spent: pageSpent, limit: pageLimitSec, minorSpent: surveySpent };\n }\n else if (surveyLimit > 0) {\n return { spent: surveySpent, limit: surveyLimit, minorSpent: pageSpent };\n }\n else {\n return { spent: pageSpent, minorSpent: surveySpent };\n }\n }\n };\n SurveyModel.prototype.getTimerInfoText = function () {\n var page = this.currentPage;\n if (!page)\n return \"\";\n var pageSpent = this.getDisplayTime(page.timeSpent);\n var surveySpent = this.getDisplayTime(this.timeSpent);\n var pageLimitSec = this.getPageMaxTimeToFinish(page);\n var pageLimit = this.getDisplayTime(pageLimitSec);\n var surveyLimit = this.getDisplayTime(this.maxTimeToFinish);\n if (this.showTimerPanelMode == \"page\")\n return this.getTimerInfoPageText(page, pageSpent, pageLimit);\n if (this.showTimerPanelMode == \"survey\")\n return this.getTimerInfoSurveyText(surveySpent, surveyLimit);\n if (this.showTimerPanelMode == \"all\") {\n if (pageLimitSec <= 0 && this.maxTimeToFinish <= 0) {\n return this.getLocalizationFormatString(\"timerSpentAll\", pageSpent, surveySpent);\n }\n if (pageLimitSec > 0 && this.maxTimeToFinish > 0) {\n return this.getLocalizationFormatString(\"timerLimitAll\", pageSpent, pageLimit, surveySpent, surveyLimit);\n }\n var pageText = this.getTimerInfoPageText(page, pageSpent, pageLimit);\n var surveyText = this.getTimerInfoSurveyText(surveySpent, surveyLimit);\n return pageText + \" \" + surveyText;\n }\n return \"\";\n };\n SurveyModel.prototype.getTimerInfoPageText = function (page, pageSpent, pageLimit) {\n return this.getPageMaxTimeToFinish(page) > 0\n ? this.getLocalizationFormatString(\"timerLimitPage\", pageSpent, pageLimit)\n : this.getLocalizationFormatString(\"timerSpentPage\", pageSpent, pageLimit);\n };\n SurveyModel.prototype.getTimerInfoSurveyText = function (surveySpent, surveyLimit) {\n var strName = this.maxTimeToFinish > 0 ? \"timerLimitSurvey\" : \"timerSpentSurvey\";\n return this.getLocalizationFormatString(strName, surveySpent, surveyLimit);\n };\n SurveyModel.prototype.getDisplayClockTime = function (val) {\n if (val < 0) {\n val = 0;\n }\n var min = Math.floor(val / 60);\n var sec = val % 60;\n var secStr = sec.toString();\n if (sec < 10) {\n secStr = \"0\" + secStr;\n }\n return min + \":\" + secStr;\n };\n SurveyModel.prototype.getDisplayTime = function (val) {\n var min = Math.floor(val / 60);\n var sec = val % 60;\n var res = \"\";\n if (min > 0) {\n res += min + \" \" + this.getLocalizationString(\"timerMin\");\n }\n if (res && sec == 0)\n return res;\n if (res)\n res += \" \";\n return res + sec + \" \" + this.getLocalizationString(\"timerSec\");\n };\n Object.defineProperty(SurveyModel.prototype, \"timerModel\", {\n get: function () { return this.timerModelValue; },\n enumerable: false,\n configurable: true\n });\n /**\n * Starts a timer that calculates how many seconds a respondent has spent on the survey. Applies only to [quiz surveys](https://surveyjs.io/form-library/documentation/design-survey-create-a-quiz).\n * @see stopTimer\n * @see maxTimeToFinish\n * @see maxTimeToFinishPage\n * @see timeSpent\n * @see onTimer\n */\n SurveyModel.prototype.startTimer = function () {\n if (this.isEditMode) {\n this.timerModel.start();\n }\n };\n SurveyModel.prototype.startTimerFromUI = function () {\n if (this.showTimerPanel != \"none\" && this.state === \"running\") {\n this.startTimer();\n }\n };\n /**\n * Stops the timer. Applies only to [quiz surveys](https://surveyjs.io/form-library/documentation/design-survey-create-a-quiz).\n * @see startTimer\n * @see maxTimeToFinish\n * @see maxTimeToFinishPage\n * @see timeSpent\n * @see onTimer\n */\n SurveyModel.prototype.stopTimer = function () {\n this.timerModel.stop();\n };\n Object.defineProperty(SurveyModel.prototype, \"timeSpent\", {\n /**\n * A time period that a respondent has spent on the survey so far; measured in seconds. Applies only to [quiz surveys](https://surveyjs.io/form-library/documentation/design-survey-create-a-quiz).\n *\n * Assign a number to this property if you need to start the quiz timer from a specific time (for instance, if you want to continue an interrupted quiz).\n *\n * You can also find out how many seconds a respondent has spent on an individual survey page. To do this, use the [`timeSpent`](https://surveyjs.io/form-library/documentation/api-reference/page-model#timeSpent) property of a [`PageModel`](https://surveyjs.io/form-library/documentation/api-reference/page-model) object.\n * @see maxTimeToFinish\n * @see maxTimeToFinishPage\n * @see startTimer\n */\n get: function () { return this.timerModel.spent; },\n set: function (val) { this.timerModel.spent = val; },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"maxTimeToFinish\", {\n /**\n * A time period that a respondent has to complete the survey; measured in seconds. Applies only to [quiz surveys](https://surveyjs.io/form-library/documentation/design-survey-create-a-quiz).\n *\n * A negative value or 0 sets an unlimited time period.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/make-quiz-javascript/ (linkStyle))\n * @see maxTimeToFinishPage\n * @see startTimer\n * @see timeSpent\n */\n get: function () {\n return this.getPropertyValue(\"maxTimeToFinish\", 0);\n },\n set: function (val) {\n this.setPropertyValue(\"maxTimeToFinish\", val);\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyModel.prototype, \"maxTimeToFinishPage\", {\n /**\n * A time period that a respondent has to complete each survey page; measured in seconds. Applies only to [quiz surveys](https://surveyjs.io/form-library/documentation/design-survey-create-a-quiz).\n *\n * A negative value or 0 sets an unlimited time period.\n *\n * You can also use `PageModel`'s [`maxTimeToFinish`](https://surveyjs.io/form-library/documentation/api-reference/page-model#maxTimeToFinish) property to specify a time period for an individual survey page.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/make-quiz-javascript/ (linkStyle))\n * @see maxTimeToFinish\n * @see startTimer\n * @see timeSpent\n */\n get: function () {\n return this.getPropertyValue(\"maxTimeToFinishPage\", 0);\n },\n set: function (val) {\n this.setPropertyValue(\"maxTimeToFinishPage\", val);\n },\n enumerable: false,\n configurable: true\n });\n SurveyModel.prototype.getPageMaxTimeToFinish = function (page) {\n if (!page || page.maxTimeToFinish < 0)\n return 0;\n return page.maxTimeToFinish > 0\n ? page.maxTimeToFinish\n : this.maxTimeToFinishPage;\n };\n SurveyModel.prototype.doTimer = function (page) {\n this.onTimer.fire(this, {});\n if (this.maxTimeToFinish > 0 && this.maxTimeToFinish == this.timeSpent) {\n this.completeLastPage();\n }\n if (page) {\n var pageLimit = this.getPageMaxTimeToFinish(page);\n if (pageLimit > 0 && pageLimit == page.timeSpent) {\n if (this.isLastPage) {\n this.completeLastPage();\n }\n else {\n this.nextPage();\n }\n }\n }\n };\n Object.defineProperty(SurveyModel.prototype, \"inSurvey\", {\n get: function () {\n return true;\n },\n enumerable: false,\n configurable: true\n });\n //ISurveyImplementor\n SurveyModel.prototype.getSurveyData = function () {\n return this;\n };\n SurveyModel.prototype.getSurvey = function () {\n return this;\n };\n SurveyModel.prototype.getTextProcessor = function () {\n return this;\n };\n //ISurveyTriggerOwner\n SurveyModel.prototype.getObjects = function (pages, questions) {\n var result = [];\n Array.prototype.push.apply(result, this.getPagesByNames(pages));\n Array.prototype.push.apply(result, this.getQuestionsByNames(questions));\n return result;\n };\n SurveyModel.prototype.setTriggerValue = function (name, value, isVariable) {\n if (!name)\n return;\n if (isVariable) {\n this.setVariable(name, value);\n }\n else {\n var question = this.getQuestionByName(name);\n if (!!question) {\n question.value = value;\n }\n else {\n var processor = new _conditionProcessValue__WEBPACK_IMPORTED_MODULE_6__[\"ProcessValue\"]();\n var firstName = processor.getFirstName(name);\n if (firstName == name) {\n this.setValue(name, value);\n }\n else {\n if (!this.getQuestionByName(firstName))\n return;\n var data = this.getUnbindValue(this.getFilteredValues());\n processor.setValue(data, name, value);\n this.setValue(firstName, data[firstName]);\n }\n }\n }\n };\n SurveyModel.prototype.copyTriggerValue = function (name, fromName, copyDisplayValue) {\n if (!name || !fromName)\n return;\n var value;\n if (copyDisplayValue) {\n value = this.processText(\"{\" + fromName + \"}\", true);\n }\n else {\n var processor = new _conditionProcessValue__WEBPACK_IMPORTED_MODULE_6__[\"ProcessValue\"]();\n value = processor.getValue(fromName, this.getFilteredValues());\n }\n this.setTriggerValue(name, value, false);\n };\n SurveyModel.prototype.triggerExecuted = function (trigger) {\n this.onTriggerExecuted.fire(this, { trigger: trigger });\n };\n SurveyModel.prototype.startMovingQuestion = function () {\n this.isMovingQuestion = true;\n };\n SurveyModel.prototype.stopMovingQuestion = function () {\n this.isMovingQuestion = false;\n };\n Object.defineProperty(SurveyModel.prototype, \"isQuestionDragging\", {\n get: function () { return this.isMovingQuestion; },\n enumerable: false,\n configurable: true\n });\n /**\n * Focuses a question with a specified name. Switches the current page if needed.\n * @param name A question name.\n * @returns `false` if the survey does not contain a question with a specified name or this question is hidden; otherwise, `true`.\n * @see focusFirstQuestion\n * @see focusFirstQuestionAutomatic\n */\n SurveyModel.prototype.focusQuestion = function (name) {\n return this.focusQuestionByInstance(this.getQuestionByName(name, true));\n };\n SurveyModel.prototype.focusQuestionByInstance = function (question, onError) {\n var _a;\n if (onError === void 0) { onError = false; }\n if (!question || !question.isVisible || !question.page)\n return false;\n var oldQuestion = (_a = this.focusingQuestionInfo) === null || _a === void 0 ? void 0 : _a.question;\n if (oldQuestion === question)\n return false;\n this.focusingQuestionInfo = { question: question, onError: onError };\n this.skippedPages.push({ from: this.currentPage, to: question.page });\n var isNeedWaitForPageRendered = this.activePage !== question.page && !question.page.isStartPage;\n if (isNeedWaitForPageRendered) {\n this.currentPage = question.page;\n }\n if (!isNeedWaitForPageRendered) {\n this.focusQuestionInfo();\n }\n return true;\n };\n SurveyModel.prototype.focusQuestionInfo = function () {\n var _a;\n var question = (_a = this.focusingQuestionInfo) === null || _a === void 0 ? void 0 : _a.question;\n if (!!question && !question.isDisposed) {\n question.focus(this.focusingQuestionInfo.onError);\n }\n this.focusingQuestionInfo = undefined;\n };\n SurveyModel.prototype.questionEditFinishCallback = function (question, event) {\n var enterKeyAction = this.enterKeyAction || _settings__WEBPACK_IMPORTED_MODULE_14__[\"settings\"].enterKeyAction;\n if (enterKeyAction == \"loseFocus\")\n event.target.blur();\n if (enterKeyAction == \"moveToNextEditor\") {\n var allQuestions = this.currentPage.questions;\n var questionIndex = allQuestions.indexOf(question);\n if (questionIndex > -1 && questionIndex < allQuestions.length - 1) {\n allQuestions[questionIndex + 1].focus();\n }\n else {\n event.target.blur();\n }\n }\n };\n SurveyModel.prototype.getElementWrapperComponentName = function (element, reason) {\n if (reason === \"logo-image\") {\n return \"sv-logo-image\";\n }\n return SurveyModel.TemplateRendererComponentName;\n };\n SurveyModel.prototype.getQuestionContentWrapperComponentName = function (element) {\n return SurveyModel.TemplateRendererComponentName;\n };\n SurveyModel.prototype.getRowWrapperComponentName = function (row) {\n return SurveyModel.TemplateRendererComponentName;\n };\n SurveyModel.prototype.getElementWrapperComponentData = function (element, reason) {\n return element;\n };\n SurveyModel.prototype.getRowWrapperComponentData = function (row) {\n return row;\n };\n SurveyModel.prototype.getItemValueWrapperComponentName = function (item, question) {\n return SurveyModel.TemplateRendererComponentName;\n };\n SurveyModel.prototype.getItemValueWrapperComponentData = function (item, question) {\n return item;\n };\n SurveyModel.prototype.getMatrixCellTemplateData = function (cell) {\n return cell.question;\n };\n SurveyModel.prototype.searchText = function (text) {\n if (!!text)\n text = text.toLowerCase();\n var res = [];\n for (var i = 0; i < this.pages.length; i++) {\n this.pages[i].searchText(text, res);\n }\n return res;\n };\n SurveyModel.prototype.getSkeletonComponentName = function (element) {\n return this.skeletonComponentName;\n };\n /**\n * Adds an element to the survey layout.\n *\n * This method accepts an object with the following layout element properties:\n *\n * - `id`: `string` | `\"timerpanel\"` | `\"progress-buttons\"` | `\"progress-questions\"` | `\"progress-pages\"` | `\"progress-correctquestions\"` | `\"progress-requiredquestions\"` | `\"toc-navigation\"` | `\"buttons-navigation\"`\\\n * A layout element identifier. You can use possible values to access and relocate or customize predefined layout elements.\n *\n * - `container`: `\"header\"` | `\"footer\"` | `\"left\"` | `\"right\"` | `\"contentTop\"` | `\"contentBottom\"`\\\n * A layout container that holds the element. If you want to display the element within multiple containers, set this property to an array of possible values.\n *\n * - `component`: `string`\\\n * The name of the component that renders the layout element.\n *\n * - `data`: `any`\\\n * Data passed as props to `component`.\n *\n * [View Demo](https://surveyjs.io/form-library/examples/progress-bar-with-percentage/ (linkStyle))\n * @param layoutElement A layout element configuration.\n * @returns The configuration of the previous layout element with the same `id`.\n */\n SurveyModel.prototype.addLayoutElement = function (layoutElement) {\n var existingLayoutElement = this.removeLayoutElement(layoutElement.id);\n this.layoutElements.push(layoutElement);\n return existingLayoutElement;\n };\n SurveyModel.prototype.findLayoutElement = function (layoutElementId) {\n var layoutElement = this.layoutElements.filter(function (a) { return a.id === layoutElementId; })[0];\n return layoutElement;\n };\n SurveyModel.prototype.removeLayoutElement = function (layoutElementId) {\n var layoutElement = this.findLayoutElement(layoutElementId);\n if (!!layoutElement) {\n var layoutElementIndex = this.layoutElements.indexOf(layoutElement);\n this.layoutElements.splice(layoutElementIndex, 1);\n }\n return layoutElement;\n };\n SurveyModel.prototype.getContainerContent = function (container) {\n var containerLayoutElements = [];\n for (var _i = 0, _a = this.layoutElements; _i < _a.length; _i++) {\n var layoutElement = _a[_i];\n if (this.mode !== \"display\" && isStrCiEqual(layoutElement.id, \"timerpanel\")) {\n if (container === \"header\") {\n if (this.isTimerPanelShowingOnTop && !this.isShowStartingPage) {\n containerLayoutElements.push(layoutElement);\n }\n }\n if (container === \"footer\") {\n if (this.isTimerPanelShowingOnBottom && !this.isShowStartingPage) {\n containerLayoutElements.push(layoutElement);\n }\n }\n }\n else if (this.state === \"running\" && isStrCiEqual(layoutElement.id, this.progressBarComponentName)) {\n var headerLayoutElement = this.findLayoutElement(\"advanced-header\");\n var advHeader = headerLayoutElement && headerLayoutElement.data;\n var isBelowHeader = !advHeader || advHeader.hasBackground;\n if (isStrCiEqual(this.showProgressBar, \"aboveHeader\")) {\n isBelowHeader = false;\n }\n if (isStrCiEqual(this.showProgressBar, \"belowHeader\")) {\n isBelowHeader = true;\n }\n if (container === \"header\" && !isBelowHeader) {\n layoutElement.index = -150;\n if (this.isShowProgressBarOnTop && !this.isShowStartingPage) {\n containerLayoutElements.push(layoutElement);\n }\n }\n if (container === \"center\" && isBelowHeader) {\n if (!!layoutElement.index) {\n delete layoutElement.index;\n }\n if (this.isShowProgressBarOnTop && !this.isShowStartingPage) {\n containerLayoutElements.push(layoutElement);\n }\n }\n if (container === \"footer\") {\n if (this.isShowProgressBarOnBottom && !this.isShowStartingPage) {\n containerLayoutElements.push(layoutElement);\n }\n }\n }\n else if (isStrCiEqual(layoutElement.id, \"buttons-navigation\")) {\n if (container === \"contentTop\") {\n if ([\"top\", \"both\"].indexOf(this.isNavigationButtonsShowing) !== -1) {\n containerLayoutElements.push(layoutElement);\n }\n }\n if (container === \"contentBottom\") {\n if ([\"bottom\", \"both\"].indexOf(this.isNavigationButtonsShowing) !== -1) {\n containerLayoutElements.push(layoutElement);\n }\n }\n }\n else if (this.state === \"running\" && isStrCiEqual(layoutElement.id, \"toc-navigation\") && this.showTOC) {\n if (container === \"left\") {\n if ([\"left\", \"both\"].indexOf(this.tocLocation) !== -1) {\n containerLayoutElements.push(layoutElement);\n }\n }\n if (container === \"right\") {\n if ([\"right\", \"both\"].indexOf(this.tocLocation) !== -1) {\n containerLayoutElements.push(layoutElement);\n }\n }\n }\n else if (isStrCiEqual(layoutElement.id, \"advanced-header\")) {\n if ((this.state === \"running\" || this.state === \"starting\") && layoutElement.container === container) {\n containerLayoutElements.push(layoutElement);\n }\n }\n else {\n if (Array.isArray(layoutElement.container) && layoutElement.container.indexOf(container) !== -1 || layoutElement.container === container) {\n containerLayoutElements.push(layoutElement);\n }\n }\n }\n containerLayoutElements.sort(function (a, b) { return (a.index || 0) - (b.index || 0); });\n return containerLayoutElements;\n };\n SurveyModel.prototype.processPopupVisiblityChanged = function (question, popup, visible) {\n this.onPopupVisibleChanged.fire(this, { question: question, popup: popup, visible: visible });\n };\n /**\n * Applies a specified theme to the survey.\n *\n * [Themes & Styles](https://surveyjs.io/form-library/documentation/manage-default-themes-and-styles (linkStyle))\n * @param theme An [`ITheme`](https://surveyjs.io/form-library/documentation/api-reference/itheme) object with theme settings.\n */\n SurveyModel.prototype.applyTheme = function (theme) {\n var _this = this;\n if (!theme)\n return;\n Object.keys(theme).forEach(function (key) {\n if (key === \"header\") {\n _this.removeLayoutElement(\"advanced-header\");\n var advHeader = new _header__WEBPACK_IMPORTED_MODULE_20__[\"Cover\"]();\n advHeader.fromTheme(theme);\n _this.insertAdvancedHeader(advHeader);\n }\n if (key === \"isPanelless\") {\n _this.isCompact = theme[key];\n }\n else {\n _this[key] = theme[key];\n }\n });\n this.themeChanged(theme);\n };\n SurveyModel.prototype.themeChanged = function (theme) {\n this.getAllQuestions().forEach(function (q) { return q.themeChanged(theme); });\n };\n /**\n * Disposes of the survey model.\n *\n * Call this method to release resources if your application contains multiple survey models or if you re-create a survey model at runtime.\n */\n SurveyModel.prototype.dispose = function () {\n this.removeScrollEventListener();\n this.destroyResizeObserver();\n this.rootElement = undefined;\n if (this.layoutElements) {\n for (var i = 0; i < this.layoutElements.length; i++) {\n if (!!this.layoutElements[i].data && this.layoutElements[i].data !== this && this.layoutElements[i].data.dispose) {\n this.layoutElements[i].data.dispose();\n }\n }\n this.layoutElements.splice(0, this.layoutElements.length);\n }\n _super.prototype.dispose.call(this);\n this.editingObj = null;\n if (!this.pages)\n return;\n this.currentPage = null;\n for (var i = 0; i < this.pages.length; i++) {\n this.pages[i].setSurveyImpl(undefined);\n this.pages[i].dispose();\n }\n this.pages.splice(0, this.pages.length);\n if (this.disposeCallback) {\n this.disposeCallback();\n }\n };\n SurveyModel.prototype.onScroll = function () {\n if (this.onScrollCallback) {\n this.onScrollCallback();\n }\n };\n SurveyModel.prototype.addScrollEventListener = function () {\n var _this = this;\n var _a;\n this.scrollHandler = function () { _this.onScroll(); };\n this.rootElement.addEventListener(\"scroll\", this.scrollHandler);\n if (!!this.rootElement.getElementsByTagName(\"form\")[0]) {\n this.rootElement.getElementsByTagName(\"form\")[0].addEventListener(\"scroll\", this.scrollHandler);\n }\n if (!!this.css.rootWrapper) {\n (_a = this.rootElement.getElementsByClassName(this.css.rootWrapper)[0]) === null || _a === void 0 ? void 0 : _a.addEventListener(\"scroll\", this.scrollHandler);\n }\n };\n SurveyModel.prototype.removeScrollEventListener = function () {\n var _a;\n if (!!this.rootElement && !!this.scrollHandler) {\n this.rootElement.removeEventListener(\"scroll\", this.scrollHandler);\n if (!!this.rootElement.getElementsByTagName(\"form\")[0]) {\n this.rootElement.getElementsByTagName(\"form\")[0].removeEventListener(\"scroll\", this.scrollHandler);\n }\n if (!!this.css.rootWrapper) {\n (_a = this.rootElement.getElementsByClassName(this.css.rootWrapper)[0]) === null || _a === void 0 ? void 0 : _a.removeEventListener(\"scroll\", this.scrollHandler);\n }\n }\n };\n SurveyModel.TemplateRendererComponentName = \"sv-template-renderer\";\n SurveyModel.stylesManager = null;\n SurveyModel.platform = \"unknown\";\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"completedCss\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"completedBeforeCss\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"loadingBodyCss\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"containerCss\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])({ onSet: function (newValue, target) { target.updateCss(); } })\n ], SurveyModel.prototype, \"fitToContainer\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])({\n onSet: function (newValue, target) {\n if (newValue === \"advanced\") {\n var layoutElement = target.findLayoutElement(\"advanced-header\");\n if (!layoutElement) {\n var advHeader = new _header__WEBPACK_IMPORTED_MODULE_20__[\"Cover\"]();\n advHeader.logoPositionX = target.logoPosition === \"right\" ? \"right\" : \"left\";\n advHeader.logoPositionY = \"middle\";\n advHeader.titlePositionX = target.logoPosition === \"right\" ? \"left\" : \"right\";\n advHeader.titlePositionY = \"middle\";\n advHeader.descriptionPositionX = target.logoPosition === \"right\" ? \"left\" : \"right\";\n advHeader.descriptionPositionY = \"middle\";\n target.insertAdvancedHeader(advHeader);\n }\n }\n else {\n target.removeLayoutElement(\"advanced-header\");\n }\n }\n })\n ], SurveyModel.prototype, \"headerView\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"showBrandInfo\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"enterKeyAction\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"lazyRenderingFirstBatchSizeValue\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])({ defaultValue: true })\n ], SurveyModel.prototype, \"titleIsEmpty\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])({ defaultValue: {} })\n ], SurveyModel.prototype, \"cssVariables\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"_isMobile\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"_isCompact\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"backgroundImage\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"renderBackgroundImage\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"backgroundImageFit\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])({\n onSet: function (newValue, target) {\n target.updateCss();\n }\n })\n ], SurveyModel.prototype, \"backgroundImageAttachment\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"backgroundImageStyle\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"wrapperFormCss\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])({\n getDefaultValue: function (self) {\n return self.progressBarType === \"buttons\";\n },\n })\n ], SurveyModel.prototype, \"progressBarShowPageTitles\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"progressBarShowPageNumbers\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"progressBarInheritWidthFrom\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"rootCss\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])()\n ], SurveyModel.prototype, \"calculatedWidthMode\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"propertyArray\"])()\n ], SurveyModel.prototype, \"layoutElements\", void 0);\n return SurveyModel;\n}(_survey_element__WEBPACK_IMPORTED_MODULE_3__[\"SurveyElementCore\"]));\n\nfunction isStrCiEqual(a, b) {\n if (!a)\n return false;\n if (!b)\n return false;\n return a.toUpperCase() === b.toUpperCase();\n}\n_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"Serializer\"].addClass(\"survey\", [\n {\n name: \"locale\",\n choices: function () {\n return _surveyStrings__WEBPACK_IMPORTED_MODULE_8__[\"surveyLocalization\"].getLocales(true);\n },\n onGetValue: function (obj) {\n return obj.locale == _surveyStrings__WEBPACK_IMPORTED_MODULE_8__[\"surveyLocalization\"].defaultLocale ? null : obj.locale;\n },\n },\n { name: \"title\", serializationProperty: \"locTitle\", dependsOn: \"locale\" },\n {\n name: \"description:text\",\n serializationProperty: \"locDescription\",\n dependsOn: \"locale\",\n },\n { name: \"logo:file\", serializationProperty: \"locLogo\" },\n { name: \"logoWidth\", default: \"300px\", minValue: 0 },\n { name: \"logoHeight\", default: \"200px\", minValue: 0 },\n {\n name: \"logoFit\",\n default: \"contain\",\n choices: [\"none\", \"contain\", \"cover\", \"fill\"],\n },\n {\n name: \"logoPosition\",\n default: \"left\",\n choices: [\"none\", \"left\", \"right\", \"top\", \"bottom\"],\n },\n { name: \"focusFirstQuestionAutomatic:boolean\" },\n { name: \"focusOnFirstError:boolean\", default: true },\n { name: \"completedHtml:html\", serializationProperty: \"locCompletedHtml\" },\n {\n name: \"completedBeforeHtml:html\",\n serializationProperty: \"locCompletedBeforeHtml\",\n },\n {\n name: \"completedHtmlOnCondition:htmlconditions\",\n className: \"htmlconditionitem\", isArray: true\n },\n { name: \"loadingHtml:html\", serializationProperty: \"locLoadingHtml\" },\n { name: \"pages:surveypages\", className: \"page\", isArray: true, onSerializeValue: function (obj) { return obj.originalPages || obj.pages; } },\n {\n name: \"elements\",\n alternativeName: \"questions\",\n baseClassName: \"question\",\n visible: false,\n isLightSerializable: false,\n onGetValue: function (obj) {\n return null;\n },\n onSetValue: function (obj, value, jsonConverter) {\n obj.pages.splice(0, obj.pages.length);\n var page = obj.addNewPage(\"\");\n jsonConverter.toObject({ questions: value }, page, jsonConverter === null || jsonConverter === void 0 ? void 0 : jsonConverter.options);\n },\n },\n {\n name: \"triggers:triggers\",\n baseClassName: \"surveytrigger\",\n classNamePart: \"trigger\",\n },\n {\n name: \"calculatedValues:calculatedvalues\",\n className: \"calculatedvalue\", isArray: true\n },\n { name: \"surveyId\", visible: false },\n { name: \"surveyPostId\", visible: false },\n { name: \"surveyShowDataSaving:boolean\", visible: false },\n \"cookieName\",\n \"sendResultOnPageNext:boolean\",\n {\n name: \"showNavigationButtons\",\n default: \"bottom\",\n choices: [\"none\", \"top\", \"bottom\", \"both\"],\n },\n {\n name: \"showPrevButton:boolean\",\n default: true,\n visibleIf: function (obj) { return obj.showNavigationButtons !== \"none\"; }\n },\n { name: \"showTitle:boolean\", default: true },\n { name: \"showPageTitles:boolean\", default: true },\n { name: \"showCompletedPage:boolean\", default: true },\n \"navigateToUrl\",\n {\n name: \"navigateToUrlOnCondition:urlconditions\",\n className: \"urlconditionitem\", isArray: true\n },\n {\n name: \"questionsOrder\",\n default: \"initial\",\n choices: [\"initial\", \"random\"],\n },\n {\n name: \"matrixDragHandleArea\",\n visible: false,\n default: \"entireItem\",\n choices: [\"entireItem\", \"icon\"]\n },\n \"showPageNumbers:boolean\",\n {\n name: \"showQuestionNumbers\",\n default: \"on\",\n choices: [\"on\", \"onPage\", \"off\"],\n },\n {\n name: \"questionTitleLocation\",\n default: \"top\",\n choices: [\"top\", \"bottom\", \"left\"],\n },\n {\n name: \"questionDescriptionLocation\",\n default: \"underTitle\",\n choices: [\"underInput\", \"underTitle\"],\n },\n { name: \"questionErrorLocation\", default: \"top\", choices: [\"top\", \"bottom\"] },\n {\n name: \"showProgressBar\",\n default: \"off\",\n choices: [\"off\", \"auto\", \"aboveHeader\", \"belowHeader\", \"bottom\", \"topBottom\"],\n },\n {\n name: \"progressBarType\",\n default: \"pages\",\n choices: [\n \"pages\",\n \"questions\",\n \"requiredQuestions\",\n \"correctQuestions\",\n ],\n visibleIf: function (obj) { return obj.showProgressBar !== \"off\"; }\n },\n {\n name: \"progressBarShowPageTitles:switch\",\n category: \"navigation\",\n visibleIf: function (obj) { return obj.showProgressBar !== \"off\" && obj.progressBarType === \"pages\"; }\n },\n {\n name: \"progressBarShowPageNumbers:switch\",\n default: false,\n category: \"navigation\",\n visibleIf: function (obj) { return obj.showProgressBar !== \"off\" && obj.progressBarType === \"pages\"; }\n },\n {\n name: \"progressBarInheritWidthFrom\",\n default: \"container\",\n choices: [\"container\", \"survey\"],\n category: \"navigation\",\n visibleIf: function (obj) { return obj.showProgressBar !== \"off\" && obj.progressBarType === \"pages\"; }\n },\n {\n name: \"showTOC:switch\",\n default: false\n },\n {\n name: \"tocLocation\", default: \"left\", choices: [\"left\", \"right\"],\n dependsOn: [\"showTOC\"],\n visibleIf: function (survey) { return !!survey && survey.showTOC; }\n },\n { name: \"mode\", default: \"edit\", choices: [\"edit\", \"display\"] },\n { name: \"storeOthersAsComment:boolean\", default: true },\n { name: \"maxTextLength:number\", default: 0, minValue: 0 },\n { name: \"maxOthersLength:number\", default: 0, minValue: 0 },\n {\n name: \"goNextPageAutomatic:boolean\",\n onSetValue: function (obj, value) {\n if (value !== \"autogonext\") {\n value = _helpers__WEBPACK_IMPORTED_MODULE_0__[\"Helpers\"].isTwoValueEquals(value, true);\n }\n obj.setPropertyValue(\"goNextPageAutomatic\", value);\n }\n },\n {\n name: \"allowCompleteSurveyAutomatic:boolean\", default: true,\n visibleIf: function (obj) { return obj.goNextPageAutomatic === true; }\n },\n {\n name: \"clearInvisibleValues\",\n default: \"onComplete\",\n choices: [\"none\", \"onComplete\", \"onHidden\", \"onHiddenContainer\"],\n },\n {\n name: \"checkErrorsMode\",\n default: \"onNextPage\",\n choices: [\"onNextPage\", \"onValueChanged\", \"onComplete\"],\n },\n {\n name: \"textUpdateMode\",\n default: \"onBlur\",\n choices: [\"onBlur\", \"onTyping\"],\n },\n { name: \"autoGrowComment:boolean\", default: false },\n { name: \"allowResizeComment:boolean\", default: true },\n {\n name: \"startSurveyText\",\n serializationProperty: \"locStartSurveyText\",\n visibleIf: function (obj) { return obj.firstPageIsStarted; }\n },\n {\n name: \"pagePrevText\",\n serializationProperty: \"locPagePrevText\",\n visibleIf: function (obj) { return obj.showNavigationButtons !== \"none\" && obj.showPrevButton; }\n },\n {\n name: \"pageNextText\",\n serializationProperty: \"locPageNextText\",\n visibleIf: function (obj) { return obj.showNavigationButtons !== \"none\"; }\n },\n {\n name: \"completeText\",\n serializationProperty: \"locCompleteText\",\n visibleIf: function (obj) { return obj.showNavigationButtons !== \"none\"; }\n },\n {\n name: \"previewText\",\n serializationProperty: \"locPreviewText\",\n visibleIf: function (obj) { return obj.showPreviewBeforeComplete !== \"noPreview\"; }\n },\n {\n name: \"editText\",\n serializationProperty: \"locEditText\",\n visibleIf: function (obj) { return obj.showPreviewBeforeComplete !== \"noPreview\"; }\n },\n { name: \"requiredText\", default: \"*\" },\n {\n name: \"questionStartIndex\",\n dependsOn: [\"showQuestionNumbers\"],\n visibleIf: function (survey) { return !survey || survey.showQuestionNumbers !== \"off\"; }\n },\n {\n name: \"questionTitlePattern\",\n default: \"numTitleRequire\",\n dependsOn: [\"questionStartIndex\", \"requiredText\"],\n choices: function (obj) {\n if (!obj)\n return [];\n return obj.getQuestionTitlePatternOptions();\n },\n },\n {\n name: \"questionTitleTemplate\",\n visible: false,\n isSerializable: false,\n serializationProperty: \"locQuestionTitleTemplate\",\n },\n { name: \"firstPageIsStarted:boolean\", default: false },\n {\n name: \"isSinglePage:boolean\",\n default: false,\n visible: false,\n isSerializable: false,\n },\n {\n name: \"questionsOnPageMode\",\n default: \"standard\",\n choices: [\"standard\", \"singlePage\", \"questionPerPage\"],\n },\n {\n name: \"showPreviewBeforeComplete\",\n default: \"noPreview\",\n choices: [\"noPreview\", \"showAllQuestions\", \"showAnsweredQuestions\"],\n },\n { name: \"maxTimeToFinish:number\", default: 0, minValue: 0 },\n { name: \"maxTimeToFinishPage:number\", default: 0, minValue: 0 },\n {\n name: \"showTimerPanel\",\n default: \"none\",\n choices: [\"none\", \"top\", \"bottom\"],\n },\n {\n name: \"showTimerPanelMode\",\n default: \"all\",\n choices: [\"page\", \"survey\", \"all\"],\n },\n {\n name: \"widthMode\",\n default: \"auto\",\n choices: [\"auto\", \"static\", \"responsive\"],\n },\n { name: \"width\", visibleIf: function (obj) { return obj.widthMode === \"static\"; } },\n { name: \"fitToContainer:boolean\", default: true, visible: false },\n { name: \"headerView\", default: \"basic\", choices: [\"basic\", \"advanced\"], visible: false },\n { name: \"backgroundImage:file\", visible: false },\n { name: \"backgroundImageFit\", default: \"cover\", choices: [\"auto\", \"contain\", \"cover\"], visible: false },\n { name: \"backgroundImageAttachment\", default: \"scroll\", choices: [\"scroll\", \"fixed\"], visible: false },\n { name: \"backgroundOpacity:number\", minValue: 0, maxValue: 1, default: 1, visible: false },\n { name: \"showBrandInfo:boolean\", default: false, visible: false }\n]);\n\n\n/***/ }),\n\n/***/ \"./src/surveyProgress.ts\":\n/*!*******************************!*\\\n !*** ./src/surveyProgress.ts ***!\n \\*******************************/\n/*! exports provided: SurveyProgressModel */\n/***/ (function(module, __webpack_exports__, __webpack_require__) {\n\n\"use strict\";\n__webpack_require__.r(__webpack_exports__);\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"SurveyProgressModel\", function() { return SurveyProgressModel; });\n/* harmony import */ var _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_0__ = __webpack_require__(/*! ./utils/cssClassBuilder */ \"./src/utils/cssClassBuilder.ts\");\n\nvar SurveyProgressModel = /** @class */ (function () {\n function SurveyProgressModel() {\n }\n SurveyProgressModel.getProgressTextInBarCss = function (css) {\n return new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_0__[\"CssClassBuilder\"]()\n .append(css.progressText)\n .append(css.progressTextInBar)\n .toString();\n };\n SurveyProgressModel.getProgressTextUnderBarCss = function (css) {\n return new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_0__[\"CssClassBuilder\"]()\n .append(css.progressText)\n .append(css.progressTextUnderBar)\n .toString();\n };\n return SurveyProgressModel;\n}());\n\n\n\n/***/ }),\n\n/***/ \"./src/surveyStrings.ts\":\n/*!******************************!*\\\n !*** ./src/surveyStrings.ts ***!\n \\******************************/\n/*! exports provided: surveyLocalization, surveyStrings */\n/***/ (function(module, __webpack_exports__, __webpack_require__) {\n\n\"use strict\";\n__webpack_require__.r(__webpack_exports__);\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"surveyLocalization\", function() { return surveyLocalization; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"surveyStrings\", function() { return surveyStrings; });\n/* harmony import */ var _localization_english__WEBPACK_IMPORTED_MODULE_0__ = __webpack_require__(/*! ./localization/english */ \"./src/localization/english.ts\");\n\nvar surveyLocalization = {\n currentLocaleValue: \"\",\n defaultLocaleValue: \"en\",\n locales: {},\n localeNames: {},\n supportedLocales: [],\n get currentLocale() {\n return this.currentLocaleValue === this.defaultLocaleValue ? \"\" : this.currentLocaleValue;\n },\n set currentLocale(val) {\n if (val === \"cz\")\n val = \"cs\";\n this.currentLocaleValue = val;\n },\n get defaultLocale() {\n return this.defaultLocaleValue;\n },\n set defaultLocale(val) {\n if (val === \"cz\")\n val = \"cs\";\n this.defaultLocaleValue = val;\n },\n getLocaleStrings: function (loc) {\n return this.locales[loc];\n },\n getString: function (strName, locale) {\n var _this = this;\n if (locale === void 0) { locale = null; }\n var locs = new Array();\n var addLocaleCore = function (locName) {\n var strs = _this.locales[locName];\n if (!!strs)\n locs.push(strs);\n };\n var addLocale = function (locName) {\n if (!locName)\n return;\n addLocaleCore(locName);\n var index = locName.indexOf(\"-\");\n if (index < 1)\n return;\n locName = locName.substring(0, index);\n addLocaleCore(locName);\n };\n addLocale(locale);\n addLocale(this.currentLocale);\n addLocale(this.defaultLocale);\n if (this.defaultLocale !== \"en\") {\n addLocaleCore(\"en\");\n }\n for (var i = 0; i < locs.length; i++) {\n var res = locs[i][strName];\n if (res !== undefined)\n return res;\n }\n return this.onGetExternalString(strName, locale);\n },\n getLocales: function (removeDefaultLoc) {\n if (removeDefaultLoc === void 0) { removeDefaultLoc = false; }\n var res = [];\n res.push(\"\");\n var locs = this.locales;\n if (this.supportedLocales && this.supportedLocales.length > 0) {\n locs = {};\n for (var i = 0; i < this.supportedLocales.length; i++) {\n locs[this.supportedLocales[i]] = true;\n }\n }\n for (var key in locs) {\n if (removeDefaultLoc && key == this.defaultLocale)\n continue;\n res.push(key);\n }\n var locName = function (loc) {\n if (!loc)\n return \"\";\n var res = surveyLocalization.localeNames[loc];\n if (!res)\n res = loc;\n return res.toLowerCase();\n };\n res.sort(function (a, b) {\n var str1 = locName(a);\n var str2 = locName(b);\n if (str1 === str2)\n return 0;\n return str1 < str2 ? -1 : 1;\n });\n return res;\n },\n onGetExternalString: function (name, locale) { return undefined; }\n};\nvar surveyStrings = _localization_english__WEBPACK_IMPORTED_MODULE_0__[\"englishStrings\"];\nsurveyLocalization.locales[\"en\"] = _localization_english__WEBPACK_IMPORTED_MODULE_0__[\"englishStrings\"];\nsurveyLocalization.localeNames[\"en\"] = \"english\";\n\n\n/***/ }),\n\n/***/ \"./src/surveyTaskManager.ts\":\n/*!**********************************!*\\\n !*** ./src/surveyTaskManager.ts ***!\n \\**********************************/\n/*! exports provided: SurveyTaskManagerModel */\n/***/ (function(module, __webpack_exports__, __webpack_require__) {\n\n\"use strict\";\n__webpack_require__.r(__webpack_exports__);\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"SurveyTaskManagerModel\", function() { return SurveyTaskManagerModel; });\n/* harmony import */ var _base__WEBPACK_IMPORTED_MODULE_0__ = __webpack_require__(/*! ./base */ \"./src/base.ts\");\n/* harmony import */ var _jsonobject__WEBPACK_IMPORTED_MODULE_1__ = __webpack_require__(/*! ./jsonobject */ \"./src/jsonobject.ts\");\nvar __extends = (undefined && undefined.__extends) || (function () {\n var extendStatics = function (d, b) {\n extendStatics = Object.setPrototypeOf ||\n ({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) ||\n function (d, b) { for (var p in b) if (Object.prototype.hasOwnProperty.call(b, p)) d[p] = b[p]; };\n return extendStatics(d, b);\n };\n return function (d, b) {\n if (typeof b !== \"function\" && b !== null)\n throw new TypeError(\"Class extends value \" + String(b) + \" is not a constructor or null\");\n extendStatics(d, b);\n function __() { this.constructor = d; }\n d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());\n };\n})();\nvar __decorate = (undefined && undefined.__decorate) || function (decorators, target, key, desc) {\n var c = arguments.length, r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc, d;\n if (typeof Reflect === \"object\" && typeof Reflect.decorate === \"function\") r = Reflect.decorate(decorators, target, key, desc);\n else for (var i = decorators.length - 1; i >= 0; i--) if (d = decorators[i]) r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;\n return c > 3 && r && Object.defineProperty(target, key, r), r;\n};\n\n\nvar SurveyTaskModel = /** @class */ (function () {\n function SurveyTaskModel(type) {\n this.type = type;\n this.timestamp = new Date();\n }\n return SurveyTaskModel;\n}());\nvar SurveyTaskManagerModel = /** @class */ (function (_super) {\n __extends(SurveyTaskManagerModel, _super);\n function SurveyTaskManagerModel() {\n var _this = _super.call(this) || this;\n _this.taskList = [];\n _this.onAllTasksCompleted = _this.addEvent();\n return _this;\n }\n SurveyTaskManagerModel.prototype.runTask = function (type, func) {\n var _this = this;\n var task = new SurveyTaskModel(type);\n this.taskList.push(task);\n this.hasActiveTasks = true;\n func(function () { return _this.taskFinished(task); });\n return task;\n };\n SurveyTaskManagerModel.prototype.waitAndExecute = function (action) {\n if (!this.hasActiveTasks) {\n action();\n return;\n }\n this.onAllTasksCompleted.add(function () { action(); });\n };\n SurveyTaskManagerModel.prototype.taskFinished = function (task) {\n var index = this.taskList.indexOf(task);\n if (index > -1) {\n this.taskList.splice(index, 1);\n }\n if (this.hasActiveTasks && this.taskList.length == 0) {\n this.hasActiveTasks = false;\n this.onAllTasksCompleted.fire(this, {});\n }\n };\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_1__[\"property\"])({ defaultValue: false })\n ], SurveyTaskManagerModel.prototype, \"hasActiveTasks\", void 0);\n return SurveyTaskManagerModel;\n}(_base__WEBPACK_IMPORTED_MODULE_0__[\"Base\"]));\n\n\n\n/***/ }),\n\n/***/ \"./src/surveyTimerModel.ts\":\n/*!*********************************!*\\\n !*** ./src/surveyTimerModel.ts ***!\n \\*********************************/\n/*! exports provided: SurveyTimerModel */\n/***/ (function(module, __webpack_exports__, __webpack_require__) {\n\n\"use strict\";\n__webpack_require__.r(__webpack_exports__);\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"SurveyTimerModel\", function() { return SurveyTimerModel; });\n/* harmony import */ var _base__WEBPACK_IMPORTED_MODULE_0__ = __webpack_require__(/*! ./base */ \"./src/base.ts\");\n/* harmony import */ var _surveytimer__WEBPACK_IMPORTED_MODULE_1__ = __webpack_require__(/*! ./surveytimer */ \"./src/surveytimer.ts\");\n/* harmony import */ var _jsonobject__WEBPACK_IMPORTED_MODULE_2__ = __webpack_require__(/*! ./jsonobject */ \"./src/jsonobject.ts\");\n/* harmony import */ var _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_3__ = __webpack_require__(/*! ./utils/cssClassBuilder */ \"./src/utils/cssClassBuilder.ts\");\nvar __extends = (undefined && undefined.__extends) || (function () {\n var extendStatics = function (d, b) {\n extendStatics = Object.setPrototypeOf ||\n ({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) ||\n function (d, b) { for (var p in b) if (Object.prototype.hasOwnProperty.call(b, p)) d[p] = b[p]; };\n return extendStatics(d, b);\n };\n return function (d, b) {\n if (typeof b !== \"function\" && b !== null)\n throw new TypeError(\"Class extends value \" + String(b) + \" is not a constructor or null\");\n extendStatics(d, b);\n function __() { this.constructor = d; }\n d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());\n };\n})();\nvar __decorate = (undefined && undefined.__decorate) || function (decorators, target, key, desc) {\n var c = arguments.length, r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc, d;\n if (typeof Reflect === \"object\" && typeof Reflect.decorate === \"function\") r = Reflect.decorate(decorators, target, key, desc);\n else for (var i = decorators.length - 1; i >= 0; i--) if (d = decorators[i]) r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;\n return c > 3 && r && Object.defineProperty(target, key, r), r;\n};\n\n\n\n\nvar SurveyTimerModel = /** @class */ (function (_super) {\n __extends(SurveyTimerModel, _super);\n function SurveyTimerModel(survey) {\n var _this = _super.call(this) || this;\n _this.timerFunc = null;\n _this.surveyValue = survey;\n _this.onCreating();\n return _this;\n }\n Object.defineProperty(SurveyTimerModel.prototype, \"survey\", {\n get: function () { return this.surveyValue; },\n enumerable: false,\n configurable: true\n });\n SurveyTimerModel.prototype.onCreating = function () { };\n SurveyTimerModel.prototype.start = function () {\n var _this = this;\n if (!this.survey)\n return;\n if (this.isRunning || this.isDesignMode)\n return;\n this.survey.onCurrentPageChanged.add(function () {\n _this.update();\n });\n this.timerFunc = function () { _this.doTimer(); };\n this.setIsRunning(true);\n this.update();\n _surveytimer__WEBPACK_IMPORTED_MODULE_1__[\"SurveyTimer\"].instance.start(this.timerFunc);\n };\n SurveyTimerModel.prototype.stop = function () {\n if (!this.isRunning)\n return;\n this.setIsRunning(false);\n _surveytimer__WEBPACK_IMPORTED_MODULE_1__[\"SurveyTimer\"].instance.stop(this.timerFunc);\n };\n Object.defineProperty(SurveyTimerModel.prototype, \"isRunning\", {\n get: function () {\n return this.getPropertyValue(\"isRunning\", false);\n },\n enumerable: false,\n configurable: true\n });\n SurveyTimerModel.prototype.setIsRunning = function (val) {\n this.setPropertyValue(\"isRunning\", val);\n };\n SurveyTimerModel.prototype.update = function () {\n this.updateText();\n this.updateProgress();\n };\n SurveyTimerModel.prototype.doTimer = function () {\n var page = this.survey.currentPage;\n if (page) {\n page.timeSpent = page.timeSpent + 1;\n }\n this.spent = this.spent + 1;\n this.update();\n if (this.onTimer) {\n this.onTimer(page);\n }\n };\n SurveyTimerModel.prototype.updateProgress = function () {\n var _this = this;\n var _a = this.survey.timerInfo, spent = _a.spent, limit = _a.limit;\n if (!limit) {\n this.progress = undefined;\n }\n else {\n if (spent == 0) {\n this.progress = 0;\n setTimeout(function () {\n _this.progress = Math.floor((spent + 1) / limit * 100) / 100;\n }, 0);\n }\n else if (spent <= limit) {\n this.progress = Math.floor((spent + 1) / limit * 100) / 100;\n }\n if (this.progress > 1) {\n this.progress = undefined;\n }\n }\n };\n SurveyTimerModel.prototype.updateText = function () {\n var timerClock = this.survey.timerClock;\n this.clockMajorText = timerClock.majorText;\n this.clockMinorText = timerClock.minorText;\n this.text = this.survey.timerInfoText;\n };\n Object.defineProperty(SurveyTimerModel.prototype, \"showProgress\", {\n get: function () {\n return this.progress !== undefined;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyTimerModel.prototype, \"showTimerAsClock\", {\n get: function () {\n return !!this.survey.getCss().clockTimerRoot;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyTimerModel.prototype, \"rootCss\", {\n get: function () {\n return new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_3__[\"CssClassBuilder\"]()\n .append(this.survey.getCss().clockTimerRoot)\n .append(this.survey.getCss().clockTimerRootTop, this.survey.isTimerPanelShowingOnTop)\n .append(this.survey.getCss().clockTimerRootBottom, this.survey.isTimerPanelShowingOnBottom)\n .toString();\n },\n enumerable: false,\n configurable: true\n });\n SurveyTimerModel.prototype.getProgressCss = function () {\n return new _utils_cssClassBuilder__WEBPACK_IMPORTED_MODULE_3__[\"CssClassBuilder\"]()\n .append(this.survey.getCss().clockTimerProgress)\n .append(this.survey.getCss().clockTimerProgressAnimation, this.progress > 0)\n .toString();\n };\n Object.defineProperty(SurveyTimerModel.prototype, \"textContainerCss\", {\n get: function () {\n return this.survey.getCss().clockTimerTextContainer;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyTimerModel.prototype, \"minorTextCss\", {\n get: function () {\n return this.survey.getCss().clockTimerMinorText;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(SurveyTimerModel.prototype, \"majorTextCss\", {\n get: function () {\n return this.survey.getCss().clockTimerMajorText;\n },\n enumerable: false,\n configurable: true\n });\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_2__[\"property\"])()\n ], SurveyTimerModel.prototype, \"text\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_2__[\"property\"])()\n ], SurveyTimerModel.prototype, \"progress\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_2__[\"property\"])()\n ], SurveyTimerModel.prototype, \"clockMajorText\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_2__[\"property\"])()\n ], SurveyTimerModel.prototype, \"clockMinorText\", void 0);\n __decorate([\n Object(_jsonobject__WEBPACK_IMPORTED_MODULE_2__[\"property\"])({ defaultValue: 0 })\n ], SurveyTimerModel.prototype, \"spent\", void 0);\n return SurveyTimerModel;\n}(_base__WEBPACK_IMPORTED_MODULE_0__[\"Base\"]));\n\n\n\n/***/ }),\n\n/***/ \"./src/surveyToc.ts\":\n/*!**************************!*\\\n !*** ./src/surveyToc.ts ***!\n \\**************************/\n/*! exports provided: tryFocusPage, createTOCListModel, getTocRootCss, TOCModel */\n/***/ (function(module, __webpack_exports__, __webpack_require__) {\n\n\"use strict\";\n__webpack_require__.r(__webpack_exports__);\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"tryFocusPage\", function() { return tryFocusPage; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"createTOCListModel\", function() { return createTOCListModel; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"getTocRootCss\", function() { return getTocRootCss; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"TOCModel\", function() { return TOCModel; });\n/* harmony import */ var _actions_action__WEBPACK_IMPORTED_MODULE_0__ = __webpack_require__(/*! ./actions/action */ \"./src/actions/action.ts\");\n/* harmony import */ var _base__WEBPACK_IMPORTED_MODULE_1__ = __webpack_require__(/*! ./base */ \"./src/base.ts\");\n/* harmony import */ var _global_variables_utils__WEBPACK_IMPORTED_MODULE_2__ = __webpack_require__(/*! ./global_variables_utils */ \"./src/global_variables_utils.ts\");\n/* harmony import */ var _list__WEBPACK_IMPORTED_MODULE_3__ = __webpack_require__(/*! ./list */ \"./src/list.ts\");\n/* harmony import */ var _page__WEBPACK_IMPORTED_MODULE_4__ = __webpack_require__(/*! ./page */ \"./src/page.ts\");\n/* harmony import */ var _popup__WEBPACK_IMPORTED_MODULE_5__ = __webpack_require__(/*! ./popup */ \"./src/popup.ts\");\n\n\n\n\n\n\nfunction tryFocusPage(survey, panel) {\n if (survey.isDesignMode)\n return true;\n panel.focusFirstQuestion();\n return true;\n}\nfunction createTOCListModel(survey, onAction) {\n var _a;\n var pagesSource = survey.questionsOnPageMode === \"singlePage\" ? (_a = survey.pages[0]) === null || _a === void 0 ? void 0 : _a.elements : survey.pages;\n var items = (pagesSource || []).map(function (page) {\n var _a, _b;\n return new _actions_action__WEBPACK_IMPORTED_MODULE_0__[\"Action\"]({\n id: page.name,\n locTitle: ((_a = page.locNavigationTitle) === null || _a === void 0 ? void 0 : _a.text) ? page.locNavigationTitle : (((_b = page.locTitle) === null || _b === void 0 ? void 0 : _b.text) ? page.locTitle : undefined),\n title: page.renderedNavigationTitle,\n action: function () {\n _global_variables_utils__WEBPACK_IMPORTED_MODULE_2__[\"DomDocumentHelper\"].activeElementBlur();\n !!onAction && onAction();\n if (page instanceof _page__WEBPACK_IMPORTED_MODULE_4__[\"PageModel\"]) {\n return survey.tryNavigateToPage(page);\n }\n return tryFocusPage(survey, page);\n },\n visible: new _base__WEBPACK_IMPORTED_MODULE_1__[\"ComputedUpdater\"](function () { return page.isVisible && !(page[\"isStartPage\"]); })\n });\n });\n var listModel = new _list__WEBPACK_IMPORTED_MODULE_3__[\"ListModel\"](items, function (item) {\n if (!!item.action()) {\n listModel.selectedItem = item;\n }\n }, true, items.filter(function (i) { return !!survey.currentPage && i.id === survey.currentPage.name; })[0] || items.filter(function (i) { return i.id === pagesSource[0].name; })[0]);\n listModel.allowSelection = false;\n listModel.locOwner = survey;\n listModel.searchEnabled = false;\n survey.onCurrentPageChanged.add(function (s, o) {\n listModel.selectedItem = items.filter(function (i) { return !!survey.currentPage && i.id === survey.currentPage.name; })[0];\n });\n return listModel;\n}\nfunction getTocRootCss(survey, isMobile) {\n if (isMobile === void 0) { isMobile = false; }\n if (isMobile) {\n return \"sv_progress-toc sv_progress-toc--mobile\";\n }\n return \"sv_progress-toc\" + (\" sv_progress-toc--\" + (survey.tocLocation || \"\").toLowerCase());\n}\nvar TOCModel = /** @class */ (function () {\n function TOCModel(survey) {\n var _this = this;\n this.survey = survey;\n this.icon = \"icon-navmenu_24x24\";\n this.togglePopup = function () {\n _this.popupModel.toggleVisibility();\n };\n this.listModel = createTOCListModel(survey, function () { _this.popupModel.isVisible = false; });\n this.popupModel = new _popup__WEBPACK_IMPORTED_MODULE_5__[\"PopupModel\"](\"sv-list\", { model: this.listModel });\n this.popupModel.overlayDisplayMode = \"overlay\";\n this.popupModel.displayMode = new _base__WEBPACK_IMPORTED_MODULE_1__[\"ComputedUpdater\"](function () { return _this.isMobile ? \"overlay\" : \"popup\"; });\n }\n Object.defineProperty(TOCModel.prototype, \"isMobile\", {\n get: function () {\n return this.survey.isMobile;\n },\n enumerable: false,\n configurable: true\n });\n Object.defineProperty(TOCModel.prototype, \"containerCss\", {\n get: function () {\n return getTocRootCss(this.survey, this.isMobile);\n },\n enumerable: false,\n configurable: true\n });\n TOCModel.prototype.dispose = function () {\n this.popupModel.dispose();\n this.listModel.dispose();\n };\n return TOCModel;\n}());\n\n\n\n/***/ }),\n\n/***/ \"./src/surveytimer.ts\":\n/*!****************************!*\\\n !*** ./src/surveytimer.ts ***!\n \\****************************/\n/*! exports provided: surveyTimerFunctions, SurveyTimer */\n/***/ (function(module, __webpack_exports__, __webpack_require__) {\n\n\"use strict\";\n__webpack_require__.r(__webpack_exports__);\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"surveyTimerFunctions\", function() { return surveyTimerFunctions; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"SurveyTimer\", function() { return SurveyTimer; });\n/* harmony import */ var _base__WEBPACK_IMPORTED_MODULE_0__ = __webpack_require__(/*! ./base */ \"./src/base.ts\");\n\nvar surveyTimerFunctions = {\n setTimeout: function (func) {\n return surveyTimerFunctions.safeTimeOut(func, 1000);\n },\n clearTimeout: function (timerId) {\n clearTimeout(timerId);\n },\n safeTimeOut: function (func, delay) {\n if (delay <= 0) {\n func();\n return 0;\n }\n else {\n return setTimeout(func, delay);\n }\n }\n};\nvar SurveyTimer = /** @class */ (function () {\n function SurveyTimer() {\n this.listenerCounter = 0;\n this.timerId = -1;\n this.onTimer = new _base__WEBPACK_IMPORTED_MODULE_0__[\"Event\"]();\n }\n Object.defineProperty(SurveyTimer, \"instance\", {\n get: function () {\n if (!SurveyTimer.instanceValue) {\n SurveyTimer.instanceValue = new SurveyTimer();\n }\n return SurveyTimer.instanceValue;\n },\n enumerable: false,\n configurable: true\n });\n SurveyTimer.prototype.start = function (func) {\n var _this = this;\n if (func === void 0) { func = null; }\n if (func) {\n this.onTimer.add(func);\n }\n if (this.timerId < 0) {\n this.timerId = surveyTimerFunctions.setTimeout(function () {\n _this.doTimer();\n });\n }\n this.listenerCounter++;\n };\n SurveyTimer.prototype.stop = function (func) {\n if (func === void 0) { func = null; }\n if (func) {\n this.onTimer.remove(func);\n }\n this.listenerCounter--;\n if (this.listenerCounter == 0 && this.timerId > -1) {\n surveyTimerFunctions.clearTimeout(this.timerId);\n this.timerId = -1;\n }\n };\n SurveyTimer.prototype.doTimer = function () {\n var _this = this;\n if (this.onTimer.isEmpty || this.listenerCounter == 0) {\n this.timerId = -1;\n }\n if (this.timerId < 0)\n return;\n var prevItem = this.timerId;\n this.onTimer.fire(this, {});\n //We have to check that we have the same timerId\n //It could be changed during events execution and it will lead to double timer events\n if (prevItem !== this.timerId)\n return;\n this.timerId = surveyTimerFunctions.setTimeout(function () {\n _this.doTimer();\n });\n };\n SurveyTimer.instanceValue = null;\n return SurveyTimer;\n}());\n\n\n\n/***/ }),\n\n/***/ \"./src/svgbundle.ts\":\n/*!**************************!*\\\n !*** ./src/svgbundle.ts ***!\n \\**************************/\n/*! exports provided: SvgIconRegistry, SvgRegistry, SvgBundleViewModel */\n/***/ (function(module, __webpack_exports__, __webpack_require__) {\n\n\"use strict\";\n__webpack_require__.r(__webpack_exports__);\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"SvgIconRegistry\", function() { return SvgIconRegistry; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"SvgRegistry\", function() { return SvgRegistry; });\n/* harmony export (binding) */ __webpack_require__.d(__webpack_exports__, \"SvgBundleViewModel\", function() { return SvgBundleViewModel; });\n/* harmony import */ var _global_variables_utils__WEBPACK_IMPORTED_MODULE_0__ = __webpack_require__(/*! ./global_variables_utils */ \"./src/global_variables_utils.ts\");\n\nvar SvgIconData = /** @class */ (function () {\n function SvgIconData() {\n }\n return SvgIconData;\n}());\nvar SvgIconRegistry = /** @class */ (function () {\n function SvgIconRegistry() {\n this.icons = {};\n this.iconPrefix = \"icon-\";\n }\n SvgIconRegistry.prototype.processId = function (iconId, iconPrefix) {\n if (iconId.indexOf(iconPrefix) == 0)\n iconId = iconId.substring(iconPrefix.length);\n return iconId;\n };\n SvgIconRegistry.prototype.registerIconFromSymbol = function (iconId, iconSymbolSvg) {\n this.icons[iconId] = iconSymbolSvg;\n };\n SvgIconRegistry.prototype.registerIconFromSvgViaElement = function (iconId, iconSvg, iconPrefix) {\n if (iconPrefix === void 0) { iconPrefix = this.iconPrefix; }\n if (!_global_variables_utils__WEBPACK_IMPORTED_MODULE_0__[\"DomDocumentHelper\"].isAvailable())\n return;\n iconId = this.processId(iconId, iconPrefix);\n var divSvg = _global_variables_utils__WEBPACK_IMPORTED_MODULE_0__[\"DomDocumentHelper\"].createElement(\"div\");\n divSvg.innerHTML = iconSvg;\n var symbol = _global_variables_utils__WEBPACK_IMPORTED_MODULE_0__[\"DomDocumentHelper\"].createElement(\"symbol\");\n var svg = divSvg.querySelector(\"svg\");\n symbol.innerHTML = svg.innerHTML;\n for (var i = 0; i < svg.attributes.length; i++) {\n symbol.setAttributeNS(\"http://www.w3.org/2000/svg\", svg.attributes[i].name, svg.attributes[i].value);\n }\n symbol.id = iconPrefix + iconId;\n this.registerIconFromSymbol(iconId, symbol.outerHTML);\n };\n SvgIconRegistry.prototype.registerIconFromSvg = function (iconId, iconSvg, iconPrefix) {\n if (iconPrefix === void 0) { iconPrefix = this.iconPrefix; }\n iconId = this.processId(iconId, iconPrefix);\n var startStr = \"